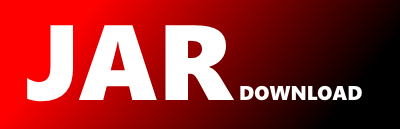
com.sun.jbi.ui.client.JMXConnectionImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jbi-admin-common Show documentation
Show all versions of jbi-admin-common Show documentation
The "jbi-admin-common" Common CLI code
The newest version!
/*
* BEGIN_HEADER - DO NOT EDIT
*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the "License"). You may not use this file except
* in compliance with the License.
*
* You can obtain a copy of the license at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* HEADER in each file and include the License file at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* If applicable add the following below this CDDL HEADER,
* with the fields enclosed by brackets "[]" replaced with
* your own identifying information: Portions Copyright
* [year] [name of copyright owner]
*/
/*
* @(#)JMXConnectionImpl.java
* Copyright 2004-2007 Sun Microsystems, Inc. All Rights Reserved.
*
* END_HEADER - DO NOT EDIT
*/
package com.sun.jbi.ui.client;
import com.sun.jbi.ui.common.I18NBundle;
import com.sun.jbi.ui.common.JBIRemoteException;
import com.sun.jbi.ui.common.JBIResultXmlBuilder;
import com.sun.jbi.ui.common.JMXConnectionException;
import java.io.IOException;
import java.util.Properties;
import javax.management.Attribute;
import javax.management.InstanceNotFoundException;
import javax.management.MBeanException;
import javax.management.MBeanServerConnection;
import javax.management.ObjectName;
import javax.management.ReflectionException;
import javax.management.RuntimeMBeanException;
import javax.management.RuntimeOperationsException;
/**
* This class is a base class to implement the jmx connection interface.
* apis.
* @author Sun Microsystems, Inc.
*/
public abstract class JMXConnectionImpl implements JMXConnection
{
/** i18n */
private static I18NBundle sI18NBundle = null;
/** connection to Mbeanserver */
protected MBeanServerConnection mMBeanServerConnection;
/**
* base implementation constructor
*/
public JMXConnectionImpl()
{
this.mMBeanServerConnection = null;
}
/** gives the I18N bundle
*@return I18NBundle object
*/
protected static I18NBundle getI18NBundle()
{
// lazzy initialize the JBI Client
if ( sI18NBundle == null )
{
sI18NBundle = new I18NBundle("com.sun.jbi.ui.client");
}
return sI18NBundle;
}
/**
* returns mbean server connection.
* @throws IllegalStateException on error
* @return mbeanserver interface
*/
public MBeanServerConnection getMBeanServerConnection()
throws IllegalStateException
{
if ( this.mMBeanServerConnection == null )
{
throw new IllegalStateException(
JBIResultXmlBuilder.createFailedJbiResultXml(
getI18NBundle(), "jbi.ui.jmx.connection.not.open", null)
);
}
return this.mMBeanServerConnection;
}
/**
* invokes operation
* @param name name fo mbean
* @param operationName operation name
* @param params parameters
* @param signature parameter signatures
* @throws JMXConnectionException on error
* @throws JBIRemoteException on error
* @return result object
*/
public Object invokeMBeanOperation(ObjectName name, String operationName,
Object[] params, String[] signature)
throws JMXConnectionException, JBIRemoteException
{
try
{
Object resultObject =
getMBeanServerConnection().invoke(name,operationName, params, signature);
return resultObject;
}
catch (InstanceNotFoundException notFoundEx)
{
// pass null to the cause as you have already serialized the cause to jbi mgmt xml
throw new JMXConnectionException(
JBIResultXmlBuilder.createJbiResultXml(
getI18NBundle(), "jbi.ui.jmx.invoke.error", null, notFoundEx),
null);
}
catch ( ReflectionException rEx)
{
// pass null to the cause as you have already serialized the cause to jbi mgmt xml
throw new JMXConnectionException(
JBIResultXmlBuilder.createJbiResultXml(
getI18NBundle(),"jbi.ui.jmx.invoke.error", null, rEx),
null);
}
catch ( IOException ioEx )
{
// pass null to the cause as you have already serialized the cause to jbi mgmt xml
throw new JMXConnectionException(
JBIResultXmlBuilder.createJbiResultXml(
getI18NBundle(),"jbi.ui.jmx.invoke.error", null, ioEx),
null);
}
catch ( MBeanException mbeanEx )
{
throw JBIRemoteException.filterJmxExceptions(mbeanEx);
}
catch (RuntimeMBeanException rtEx)
{
throw JBIRemoteException.filterJmxExceptions(rtEx);
}
catch (RuntimeOperationsException rtOpEx)
{
throw JBIRemoteException.filterJmxExceptions(rtOpEx);
}
catch ( Exception ex )
{
// pass null to the cause as you have already serialized the cause to jbi mgmt xml
throw new JBIRemoteException(
JBIResultXmlBuilder.createJbiResultXml(
getI18NBundle(),"jbi.ui.jmx.unknown.error", null, ex),
null);
}
}
/**
* set the attribute on mbean
* @param name mbean jmx object name
* @param attribute attribute
* @throws JMXConnectionException on error
* @throws JBIRemoteException on error
*/
public void setMBeanAttribute(ObjectName name, Attribute attribute)
throws JMXConnectionException, JBIRemoteException
{
try
{
getMBeanServerConnection().setAttribute(name,attribute);
}
catch ( Exception allEx )
{
//TODO: make explicit catch for better error reporting
// all other exceptions
throw JBIRemoteException.filterJmxExceptions(allEx);
}
}
/**
* return the attribute value
* @param name jmx object name
* @param attribute attribute name
* @throws JMXConnectionException on error
* @throws JBIRemoteException on error
* @return attribute value
*/
public Object getMBeanAttribute(ObjectName name, String attribute)
throws JMXConnectionException, JBIRemoteException
{
try
{
Object resultObject =
getMBeanServerConnection().getAttribute(name,attribute);
return resultObject;
}
catch ( Exception allEx )
{
//TODO: make explicit catch for better error reporting
// all other exceptions
throw JBIRemoteException.filterJmxExceptions(allEx);
}
}
/**
* creates jmx connection
* @return jmx connection
* @param username username
* @param password password
* @param host host name
* @param port port number
* @throws JMXConnectionException on error
*/
public static JMXConnection createJmxConnection(String host, String port,
String username, String password)
throws JMXConnectionException
{
Properties props = JMXConnectionProperties
.getJMXConnectionPropertyMap(null, host,port,username,password);
return createJmxConnection(props);
}
/**
* creates jmx connection
* @return jmx connection
* @param connProps properties
* @throws JMXConnectionException on error
*/
public static JMXConnection createJmxConnection(Properties connProps)
throws JMXConnectionException
{
return JMXConnectionFactory.newInstance("").getConnection(connProps);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy