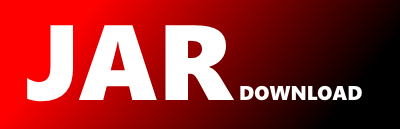
com.sun.jbi.ui.client.JMXConnectionProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jbi-admin-common Show documentation
Show all versions of jbi-admin-common Show documentation
The "jbi-admin-common" Common CLI code
The newest version!
/*
* BEGIN_HEADER - DO NOT EDIT
*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the "License"). You may not use this file except
* in compliance with the License.
*
* You can obtain a copy of the license at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* HEADER in each file and include the License file at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* If applicable add the following below this CDDL HEADER,
* with the fields enclosed by brackets "[]" replaced with
* your own identifying information: Portions Copyright
* [year] [name of copyright owner]
*/
/*
* @(#)JMXConnectionProperties.java
* Copyright 2004-2007 Sun Microsystems, Inc. All Rights Reserved.
*
* END_HEADER - DO NOT EDIT
*/
package com.sun.jbi.ui.client;
import com.sun.jbi.ui.common.ToolsLogManager;
import com.sun.jbi.ui.common.Util;
import java.io.IOException;
import java.text.MessageFormat;
import java.util.Properties;
import java.util.logging.Level;
/**
* This class manages the JMX Connection properties
*
* @author Sun Microsystems, Inc.
*/
public class JMXConnectionProperties
{
/**
* property name
*/
public static final String USERNAME_PROP =
"com.sun.jbi.tools.remote.jmx.username";
/**
* property name
*/
public static final String PASSWORD_PROP =
"com.sun.jbi.tools.remote.jmx.password";
/**
* property name
*/
public static final String HOST_PROP = "com.sun.jbi.tools.remote.jmx.host";
/**
* property name
*/
public static final String PORT_PROP = "com.sun.jbi.tools.remote.jmx.port";
/**
* property name
*/
public static final String URL_PROP = "com.sun.jbi.tools.remote.jmx.url";
/**
* property name
*/
public static final String CONNECTION_FACTORY_PROP =
"com.sun.jbi.tools.jmx.connection.factory";
/**
* signleton
*/
private static JMXConnectionProperties sConnProps;
/**
* defaults
*/
private static Properties sDefProps;
/**
* Creates a new instance of JMXConnectionProps
*/
private JMXConnectionProperties()
{
// don't allow others to create instance
}
/**
* loads default props
*
* @return Default Properties
*/
private Properties getDefaultProperties()
{
if (sDefProps == null)
{
try
{
sDefProps = new Properties();
sDefProps.load(this.getClass().getResourceAsStream("JMXConnection.properties"));
}
catch (IOException ex)
{
Util.logDebug(ToolsLogManager.getClientLogger(), ex);
}
}
return sDefProps;
}
/**
* gets the property value. Search order is first schemaorg_apache_xmlbeans.system props and then
* defults
*
* @param property Property to look for
*
* @return Property value
*/
private String getProperty(String property)
{
// Search order
// System setup
// default
String defValue = getDefaultProperties().getProperty(property);
String value = System.getProperty(property, defValue);
return value;
}
/**
* create instance if not created
*
* @return object
*/
public static JMXConnectionProperties getInstance()
{
if (sConnProps == null)
{
sConnProps = new JMXConnectionProperties();
}
return sConnProps;
}
/**
* username
*
* @return username
*/
public String getDefaultUserName()
{
String value = getProperty(USERNAME_PROP);
return value;
}
/**
* password
*
* @return password
*/
public String getDefaultPassword()
{
String value = getProperty(PASSWORD_PROP);
return value;
}
/**
* con factory
*
* @return connection factory
*/
public String getDefaultConnectionFactory()
{
String value = getProperty(CONNECTION_FACTORY_PROP);
return value;
}
/**
* host
*
* @return host
*/
public String getHost()
{
String value = getProperty(HOST_PROP);
return value;
}
/**
* port
*
* @return port
*/
public String getPort()
{
String value = getProperty(PORT_PROP);
return value;
}
/**
* returns url format
*
* @return url format
*/
public String getURLFormat()
{
String value = getProperty(URL_PROP);
return value;
}
/**
* constructs the jmx url
*
* @return url
*/
public String getConnectionURL()
{
return getConnectionURL(null, null, null);
}
/**
* constructs the jmx url. any or all params can be null.
*
* @param aHost host
* @param aPort port
*
* @return url
*/
public String getConnectionURL(
String aHost,
String aPort)
{
return getConnectionURL(null, aHost, aPort);
}
/**
* constructs the jmx url. any or all params can be null.
*
* @param aUrl url format
* @param aHost host
* @param aPort port
*
* @return url
*/
public String getConnectionURL(
String aUrl,
String aHost,
String aPort)
{
String urlFormat = null;
String host = null;
String port = null;
if (aHost == null)
{
host = getHost();
}
else
{
host = aHost;
}
if (aPort == null)
{
port = getPort();
}
else
{
port = aPort;
}
if (aUrl == null)
{
urlFormat = getURLFormat();
}
else
{
urlFormat = aUrl;
}
String [] args = {host, port};
String url = null;
try
{
MessageFormat mf = new MessageFormat(urlFormat);
url = mf.format(args);
}
catch (Exception ex)
{
Util.logDebug(ToolsLogManager.getClientLogger(), ex);
}
return url;
}
/**
* creates the Properties object that contains the name/value pair of the
* connection properties from the user input. Adds onlu non null values
* passed to the properties object. if user want to use the schemaorg_apache_xmlbeans.system
* defaults, they should pass null for the corresponding property.
*
* @param url url
* @param host host
* @param port port
* @param username username
* @param password password
*
* @return Property map.
*/
public static Properties getJMXConnectionPropertyMap(
String url,
String host,
String port,
String username,
String password)
{
Properties props = new Properties();
if (username != null)
{
props.setProperty(JMXConnectionProperties.USERNAME_PROP, username);
}
if (password != null)
{
props.setProperty(JMXConnectionProperties.PASSWORD_PROP, password);
}
if (host != null)
{
props.setProperty(JMXConnectionProperties.HOST_PROP, host);
}
if (port != null)
{
props.setProperty(JMXConnectionProperties.PORT_PROP, port);
}
if (url != null)
{
props.setProperty(JMXConnectionProperties.URL_PROP, url);
}
return props;
}
/**
*
*
* @param confac
* @param provprops
* @param url
* @param host
* @param port
* @param username
* @param password
*
* @return
*/
public static Properties getJMXConnectionPropertyMap(
String confac,
Properties provprops,
String url,
String host,
String port,
String username,
String password)
{
Properties props =
getJMXConnectionPropertyMap(url, host, port, username, password);
if (provprops != null)
{
try
{
props.putAll(provprops);
}
catch (Exception e)
{
;
}
}
if (confac != null)
{
props.put(CONNECTION_FACTORY_PROP, confac);
}
return props;
}
/**
*
*
* @param provprops
* @param url
* @param host
* @param port
* @param username
* @param password
*
* @return
*/
public static Properties getJMXConnectionPropertyMap(
Properties provprops,
String url,
String host,
String port,
String username,
String password)
{
Properties props =
getJMXConnectionPropertyMap(url, host, port, username, password);
if (provprops != null)
{
try
{
props.putAll(provprops);
}
catch (Exception e)
{
;
}
}
return props;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy