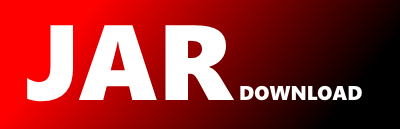
com.sun.jbi.ui.common.FileTransferManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jbi-admin-common Show documentation
Show all versions of jbi-admin-common Show documentation
The "jbi-admin-common" Common CLI code
The newest version!
/*
* BEGIN_HEADER - DO NOT EDIT
*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the "License"). You may not use this file except
* in compliance with the License.
*
* You can obtain a copy of the license at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* HEADER in each file and include the License file at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* If applicable add the following below this CDDL HEADER,
* with the fields enclosed by brackets "[]" replaced with
* your own identifying information: Portions Copyright
* [year] [name of copyright owner]
*/
/*
* @(#)FileTransferManager.java
* Copyright 2004-2007 Sun Microsystems, Inc. All Rights Reserved.
*
* END_HEADER - DO NOT EDIT
*/
package com.sun.jbi.ui.common;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.util.logging.Logger;
import javax.management.MBeanServerConnection;
import javax.management.ObjectName;
/**
* Provides access to the ESB Repository for remote clients. The scope of
* this class is limited to operations which require the transfer of
* archive data to/from the ESB Central Administration Server. All other
* repository operations can be accessed through the appropriate ESB MBean.
*
* The default chunk size for archive transfer is 8K. This setting can be
* adjusted using setChunkSize
if necessary.
*/
public class FileTransferManager {
/** Name of the ArchiveDownload MBean */
private static final String DOWNLOAD_MBEAN_NAME =
//"com.sun.jbi.esb:ServiceType=ArchiveDownload";
"com.sun.jbi:Target=domain,ServiceName=FileTransferService,ServiceType=Download";
/** Name of the ArchiveUpload MBean */
private static final String UPLOAD_MBEAN_NAME =
//"com.sun.jbi.esb:ServiceType=ArchiveUpload";
"com.sun.jbi:Target=domain,ServiceName=FileTransferService,ServiceType=Upload";
/** Default chunk size for archive transfer. */
private static final int DEFAULT_CHUNK_SIZE = 8 * 1024;
/** Upload MBean operations. */
private static final String UPLOAD_INITIATE = "initiateUpload";
private static final String UPLOAD_TRANSFER = "uploadBytes";
private static final String GET_ARCHIVE_FILE_PATH = "getArchiveFilePath";
private static final String UPLOAD_TERMINATE = "terminateUpload";
private static final String REMOVE_ARCHIVE = "removeArchive";
/** Download MBean operations. */
private static final String DOWNLOAD_INITIATE = "initiateDownload";
private static final String DOWNLOAD_TRANSFER = "downloadBytes";
private static final String DOWNLOAD_TERMINATE = "terminateDownload";
private MBeanServerConnection mServer;
private ObjectName mDownloadMBean;
private ObjectName mUploadMBean;
private int mChunkSize = DEFAULT_CHUNK_SIZE;
private Logger mLog = Logger.getLogger("com.sun.jbi.management.repository");
private Object mLastUploadId = null;
public FileTransferManager(MBeanServerConnection server) {
mServer = server;
try {
mDownloadMBean = new ObjectName(DOWNLOAD_MBEAN_NAME);
mUploadMBean = new ObjectName(UPLOAD_MBEAN_NAME);
} catch (javax.management.MalformedObjectNameException monEx) {
mLog.severe(monEx.getMessage());
}
}
/** Uploads the specified archive to a temporary store in the ESB repository.
* @return CAS-local path to the archive
*/
public String uploadArchive(File archive) throws Exception {
Object uploadId;
FileInputStream fis;
// setup our stream before initiating the upload session
fis = new FileInputStream(archive);
// initiate an upload session
uploadId = mServer.invoke(mUploadMBean, UPLOAD_INITIATE,
new Object[] { archive.getName() },
new String[] { "java.lang.String" });
this.mLastUploadId = uploadId; // remember the last uploaded id as it can be used for remove archive later
// get the upload url from the sesssion
Object uploadFilePathObj = mServer.invoke(mUploadMBean,
GET_ARCHIVE_FILE_PATH, new Object[] { uploadId },
new String[] { "java.lang.Object" });
// transfer the archive content to the server, chunk by chunk
byte[] chunk = new byte[mChunkSize];
int count = 0;
while ((count = fis.read(chunk)) != -1) {
mServer.invoke(mUploadMBean, UPLOAD_TRANSFER, new Object[] {
uploadId, trim(chunk, count) }, new String[] {
"java.lang.Object", "[B" });
}
// transfer complete, terminate the session
mServer.invoke(mUploadMBean, UPLOAD_TERMINATE,
new Object[] { uploadId, (long) 0}, new String[] { "java.lang.Object", "java.lang.Long" });
String uploadFilePath = null;
if (uploadFilePathObj != null) {
uploadFilePath = uploadFilePathObj.toString();
}
return uploadFilePath;
}
public Object getLastUploadId() {
return this.mLastUploadId;
}
/**
* this will remove any previously uploaded archive by using its uploaded id
*/
public void removeUploadedArchive(Object uploadId) {
if (uploadId == null) {
return;
}
try {
mServer.invoke(mUploadMBean, REMOVE_ARCHIVE,
new Object[] { uploadId },
new String[] { "java.lang.Object" });
} catch (Exception ex) {
// ignore any exception as the removal of the archive file is not important
// and the server during restart anyway cleans it up.
//TODO log the exception if you want.
mLog.warning(ex.getMessage());
}
}
public void downloadArchive(String archiveLocation, File target)
throws Exception {
Object downloadId;
FileOutputStream fos;
// setup our stream before initiating the download session
fos = new FileOutputStream(target);
// initiate a download session
downloadId = mServer.invoke(mDownloadMBean, DOWNLOAD_INITIATE,
new Object[] { archiveLocation },
new String[] { "java.lang.Object" });
// transfer the archive content from the server, chunk by chunk
byte[] chunk;
do {
chunk = (byte[]) mServer.invoke(mDownloadMBean, DOWNLOAD_TRANSFER,
new Object[] { downloadId, mChunkSize},
new String[] { "java.lang.Object", "int" });
fos.write(chunk);
fos.flush();
} while (chunk.length > 0);
// transfer complete, terminate the session
mServer.invoke(mDownloadMBean, DOWNLOAD_TERMINATE,
new Object[] { downloadId },
new String[] { "java.lang.Object" });
fos.close();
}
/** Return the chunk size for archive transfers. */
public int getChunkSize() {
return mChunkSize;
}
/** Sets the chuck size for archive transfers. */
public void setChunkSize(int size) {
mChunkSize = size;
}
/** Returns a byte array of the specified size, using the source array
* as input. If the length of the source array is equal to the desired
* size, the original array is returned.
*/
private byte[] trim(byte[] source, int size) {
byte[] trimmed;
if (source.length == size) {
trimmed = source;
} else {
trimmed = new byte[size];
System.arraycopy(source, 0, trimmed, 0, size);
}
return trimmed;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy