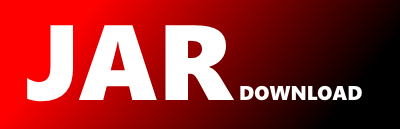
com.sun.jbi.ui.common.JBIAdminCommands Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jbi-admin-common Show documentation
Show all versions of jbi-admin-common Show documentation
The "jbi-admin-common" Common CLI code
The newest version!
/*
* BEGIN_HEADER - DO NOT EDIT
*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the "License"). You may not use this file except
* in compliance with the License.
*
* You can obtain a copy of the license at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* HEADER in each file and include the License file at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* If applicable add the following below this CDDL HEADER,
* with the fields enclosed by brackets "[]" replaced with
* your own identifying information: Portions Copyright
* [year] [name of copyright owner]
*/
/*
* @(#)JBIAdminCommands.java
* Copyright 2004-2007 Sun Microsystems, Inc. All Rights Reserved.
*
* END_HEADER - DO NOT EDIT
*/
package com.sun.jbi.ui.common;
import java.util.Map;
import java.util.Properties;
import java.util.logging.Level;
import javax.management.ObjectName;
import javax.management.openmbean.CompositeData;
import javax.management.openmbean.TabularData;
/**
* @(#)JBIAdminCommands.java - ver 2.0 - 08/22/2006
*
* This interface defines the methods corresponding to jbi administration tool
* commands and helper methods using which ant, cli, web admin tools and other
* management clients execute remote jbi administration commands on the jbi
* runtime.
*
* Client side implementations of this interface invoke the server side
* implemenation through JMX to execute commands on the server.
*
* The Server side implements this interface as an MBean so that management
* clients could invoke this interface implementation through JMX.
*
* @author graj
*/
public interface JBIAdminCommands {
public static final String DOMAIN_TARGET_KEY = "domain";
public static final String SERVER_TARGET_KEY = "server";
public static final String CUSTOM_CONFIGURATION_NAME_KEY = "Configuration";
public static final String CUSTOM_LOGGER_NAME_KEY = "Logger";
/**
* installs component ( service engine, binding component)
*
* @param paramProps
* Properties object contains name/value pair.
* @param zipFilePath
* archive file in a zip format
* @param targetName
* name of the target for this operation
* @return Map of targetName and component name strings.
* @throws JBIRemoteException
* on error
*/
public String installComponent(String zipFilePath, Properties paramProps,
String targetName) throws JBIRemoteException;
/**
* installs component ( service engine, binding component)
*
* @param zipFilePath
* archive file in a zip format
* @param targetName
* name of the target for this operation
* @return Map of targetName and component name strings.
* @throws JBIRemoteException
* on error
*/
public String installComponent(String zipFilePath, String targetName)
throws JBIRemoteException;
/**
* uninstalls component ( service engine, binding component)
*
* @param componentName
* name of the component
* @param targetName
* name of the target for this operation
* @return Map of targetName and component name strings.
* @throws JBIRemoteException
* on error
*/
public String uninstallComponent(String componentName, String targetName)
throws JBIRemoteException;
/**
* installs shared library
*
* @param zipFilePath
* archive file in a zip format
* @param targetName
* name of the target for this operation
* @return Map of targetName and shared library name strings.
* @throws JBIRemoteException
* on error
*/
public String installSharedLibrary(String zipFilePath, String targetName)
throws JBIRemoteException;
/**
* uninstalls shared library
*
* @param sharedLibraryName
* name of the shared library
* @param targetName
* name of the target for this operation
* @return Map of targetName and shared library name strings.
* @throws JBIRemoteException
* on error
*/
public String uninstallSharedLibrary(String sharedLibraryName,
String targetName) throws JBIRemoteException;
/**
* starts component ( service engine, binding component)
*
* @param componentName
* name of the component
* @param targetName
* name of the target for this operation
* @return Map of targetName and component name strings.
* @throws JBIRemoteException
* on error
*/
public String startComponent(String componentName, String targetName)
throws JBIRemoteException;
/**
* stops component ( service engine, binding component)
*
* @param componentName
* name of the component
* @param targetName
* name of the target for this operation
* @return Map of targetName and component name strings.
* @throws JBIRemoteException
* on error
*/
public String stopComponent(String componentName, String targetName)
throws JBIRemoteException;
/**
* shuts down component ( service engine, binding component)
*
* @param componentName
* name of the component
* @param targetName
* name of the target for this operation
* @return Map of targetName and component name strings.
* @throws JBIRemoteException
* on error
*/
public String shutdownComponent(String componentName, String targetName)
throws JBIRemoteException;
/**
* shuts down component ( service engine, binding component)
*
* @param componentName
* name of the component
* @param force
* true if component should be shutdown in any case, else false.
* @param targetName
* name of the target for this operation
* @return Map of targetName and component name strings.
* @throws JBIRemoteException
* on error
*/
public String shutdownComponent(String componentName, boolean force,
String targetName) throws JBIRemoteException;
/**
* deploys service assembly
*
* @param zipFilePath
* fie path
* @param targetName
* name of the target for this operation
* @return Map of targetName and management message xml text strings.
* @throws JBIRemoteException
* on error
*/
public String deployServiceAssembly(String zipFilePath, String targetName)
throws JBIRemoteException;
/**
* undeploys service assembly
*
* @param serviceAssemblyName
* name of the service assembly
* @param targetName
* name of the target for this operation
* @return Map of targetName and result as a management message xml text
* strings.
* @throws JBIRemoteException
* on error
*/
public String undeployServiceAssembly(String serviceAssemblyName,
String targetName) throws JBIRemoteException;
/**
* starts service assembly
*
* @param serviceAssemblyName
* name of the service assembly
* @param targetName
* name of the target for this operation
* @return Map of targetName and result as a management message xml text
* strings.
* @throws JBIRemoteException
* on error
*/
public String startServiceAssembly(String serviceAssemblyName,
String targetName) throws JBIRemoteException;
/**
* stops service assembly
*
* @param serviceAssemblyName
* name of the service assembly
* @param targetName
* name of the target for this operation
* @return Map of targetName and result as a management message xml text
* strings.
* @throws JBIRemoteException
* on error
*/
public String stopServiceAssembly(String serviceAssemblyName,
String targetName) throws JBIRemoteException;
/**
* shuts down service assembly
*
* @param serviceAssemblyName
* name of the service assembly
* @param targetName
* name of the target for this operation
* @return Map of targetName and result as a management message xml text
* strings.
* @throws JBIRemoteException
* on error
*/
public String shutdownServiceAssembly(String serviceAssemblyName,
String targetName) throws JBIRemoteException;
/**
* shuts down service assembly
*
* @param serviceAssemblyName
* name of the service assembly
* @param forceShutdown
* @param targetName
* name of the target for this operation
* @return Map of targetName and result as a management message xml text
* strings.
* @throws JBIRemoteException
* on error
*/
public String shutdownServiceAssembly(String serviceAssemblyName,
boolean forceShutdown, String targetName) throws JBIRemoteException;
/**
* return component info xml text that has only service engine infos.
*
* @param targetName
* name of the target for this operation
* @return the component info xml text.
* @throws JBIRemoteException
* on error
*/
public String listServiceEngines(String targetName)
throws JBIRemoteException;
/**
* return component info xml text that has only service engine infos which
* satisfies the options passed to the method.
*
* @param state
* return all the service engines that are in the specified
* state. valid states are JBIComponentInfo.STARTED, STOPPED,
* INSTALLED or null for ANY state
* @param sharedLibraryName
* return all the service engines that have a dependency on the
* specified shared library. null value to ignore this option.
* @param serviceAssemblyName
* return all the service engines that have the specified service
* assembly deployed on them. null value to ignore this option.
* @param targetName
* name of the target for this operation
* @return xml text contain the list of service engine component infos
* @throws JBIRemoteException
* if error or exception occurs.
*/
public String listServiceEngines(String state, String sharedLibraryName,
String serviceAssemblyName, String targetName)
throws JBIRemoteException;
/**
* return component info xml text that has only binding component infos.
*
* @param targetName
* name of the target for this operation
* @return the component info xml text.
* @throws JBIRemoteException
* on error
*/
public String listBindingComponents(String targetName)
throws JBIRemoteException;
/**
* return component info xml text that has only binding component infos
* which satisfies the options passed to the method.
*
* @param state
* return all the binding components that are in the specified
* state. valid states are JBIComponentInfo.STARTED, STOPPED,
* INSTALLED or null for ANY state
* @param sharedLibraryName
* return all the binding components that have a dependency on
* the specified shared library. null value to ignore this
* option.
* @param serviceAssemblyName
* return all the binding components that have the specified
* service assembly deployed on them. null value to ignore this
* option.
* @param targetName
* name of the target for this operation
* @return xml text contain the list of binding component infos
* @throws JBIRemoteException
* if error or exception occurs.
*/
public String listBindingComponents(String state, String sharedLibraryName,
String serviceAssemblyName, String targetName)
throws JBIRemoteException;
/**
* returns a list of Binding Component and Service Engine infos in xml
* format, that are dependent upon a specified Shared Library
*
* @param sharedLibraryName
* the shared library name
* @param targetName
* name of the target for this operation
* @return xml string containing the list of componentInfos
* @throws JBIRemoteException
* on error
*/
public String listSharedLibraryDependents(String sharedLibraryName,
String targetName) throws JBIRemoteException;
/**
* return component info xml text that has only shared library infos.
*
* @param targetName
* name of the target for this operation
* @return the component info xml text.
* @throws JBIRemoteException
* on error
*/
public String listSharedLibraries(String targetName)
throws JBIRemoteException;
/**
* returns the list of Shared Library infos in the in a xml format
*
* @param componentName
* to return only the shared libraries that are this component
* dependents. null for listing all the shared libraries in the
* schemaorg_apache_xmlbeans.system.
* @param targetName
* name of the target for this operation
* @return xml string contain the list of componentinfos for shared
* libraries.
* @throws JBIRemoteException
* if error or exception occurs.
*/
public String listSharedLibraries(String componentName, String targetName)
throws JBIRemoteException;
/**
* returns a list of Service Assembly Infos in a xml format.
*
* @param targetName
* name of the target for this operation
* @return xml text containing the Service Assembly infos
* @throws JBIRemoteException
* on error
*/
public String listServiceAssemblies(String targetName)
throws JBIRemoteException;
/**
* returns the list of service asssembly infos in a xml format that have the
* service unit deployed on the specified component.
*
* @param componentName
* to list all the service assemblies that have some deployments
* on this component.
* @param targetName
* name of the target for this operation
* @return xml string contain the list of service assembly infos
* @throws JBIRemoteException
* if error or exception occurs.
*/
public String listServiceAssemblies(String componentName, String targetName)
throws JBIRemoteException;
/**
* returns the list of service asssembly infos in a xml format that have the
* service unit deployed on the specified component.
*
* @param state
* to return all the service assemblies that are in the specified
* state. JBIServiceAssemblyInfo.STARTED, STOPPED, SHUTDOWN or
* null for ANY state
* @param componentName
* to list all the service assemblies that have some deployments
* on this component.
* @param targetName
* name of the target for this operation
* @return xml string contain the list of service assembly infos
* @throws JBIRemoteException
* if error or exception occurs.
*/
public String listServiceAssemblies(String state, String componentName,
String targetName) throws JBIRemoteException;
/**
* return component info xml text for the specified service engine if
* exists. If no service engine with that name exists, it returns the xml
* with empty list.
*
* @param name
* name of the service engine to lookup
* @param state
* return service engine that is in the specified state. valid
* states are JBIComponentInfo.STARTED, STOPPED, INSTALLED or
* null for ANY state
* @param sharedLibraryName
* return service engine that has a dependency on the specified
* shared library. null value to ignore this option.
* @param serviceAssemblyName
* return the service engine that has the specified service
* assembly deployed on it. null value to ignore this option.
* @param targetName
* name of the target for this operation
* @return xml text contain the service engine component info that confirms
* to the component info list xml grammer.
* @throws JBIRemoteException
* if error or exception occurs.
*/
public String showServiceEngine(String name, String state,
String sharedLibraryName, String serviceAssemblyName,
String targetName) throws JBIRemoteException;
/**
* return component info xml text for the specified binding component if
* exists. If no binding component with that name exists, it returns the xml
* with empty list.
*
* @param name
* name of the binding component to lookup
* @param state
* return the binding component that is in the specified state.
* valid states are JBIComponentInfo.STARTED, STOPPED, INSTALLED
* or null for ANY state
* @param sharedLibraryName
* return the binding component that has a dependency on the
* specified shared library. null value to ignore this option.
* @param serviceAssemblyName
* return the binding component that has the specified service
* assembly deployed on it. null value to ignore this option.
* @param targetName
* name of the target for this operation
* @return xml text contain the binding component info that confirms to the
* component info list xml grammer.
* @throws JBIRemoteException
* if error or exception occurs.
*/
public String showBindingComponent(String name, String state,
String sharedLibraryName, String serviceAssemblyName,
String targetName) throws JBIRemoteException;
/**
* return component info xml text for the specified shared library if
* exists. If no shared library with that name exists, it returns the xml
* with empty list.
*
* @param name
* name of the shared library to lookup
* @param componentName
* return the shared library that is this component dependents.
* null to ignore this option.
* @param targetName
* name of the target for this operation
* @return xml string contain shared library component info that confirms to
* the component info list xml grammer.
* @throws JBIRemoteException
* if error or exception occurs.
*/
public String showSharedLibrary(String name, String componentName,
String targetName) throws JBIRemoteException;
/**
* return service assembly info xml text for the specified service assembly
* if exists. If no service assembly with that name exists, it returns the
* xml with empty list.
*
* @param name
* name of the service assembly to lookup
* @param state
* return the service assembly that is in the specified state.
* JBIServiceAssemblyInfo.STARTED, STOPPED, SHUTDOWN or null for
* ANY state
* @param componentName
* return the service assembly that has service units on this
* component.
* @param targetName
* name of the target for this operation
* @return xml string contain service assembly info that confirms to the
* service assembly list xml grammer.
* @throws JBIRemoteException
* if error or exception occurs.
*/
public String showServiceAssembly(String name, String state,
String componentName, String targetName) throws JBIRemoteException;
/**
* installs component ( service engine, binding component)
*
* @param paramProps
* Properties object contains name/value pair.
* @param zipFilePath
* archive file in a zip format
* @param targetName
* name of the target for this operation
* @return Map of targetName and component name strings.
* @throws JBIRemoteException
* on error
*/
public Map installComponent(
String zipFilePath, Properties paramProps, String[] targetNames)
throws JBIRemoteException;
/**
* installs component ( service engine, binding component)
*
* @param zipFilePath
* archive file in a zip format
* @param targetName
* name of the target for this operation
* @return Map of targetName and component name strings.
* @throws JBIRemoteException
* on error
*/
public Map installComponent(
String zipFilePath, String[] targetNames) throws JBIRemoteException;
/**
* uninstalls component ( service engine, binding component)
*
* @param componentName
* name of the component
* @param targetName
* name of the target for this operation
* @return Map of targetName and component name strings.
* @throws JBIRemoteException
* on error
*/
public Map uninstallComponent(
String componentName, String[] targetNames)
throws JBIRemoteException;
/**
* installs shared library
*
* @param zipFilePath
* archive file in a zip format
* @param targetName
* name of the target for this operation
* @return Map of targetName and shared library name strings.
* @throws JBIRemoteException
* on error
*/
public Map installSharedLibrary(
String zipFilePath, String[] targetNames) throws JBIRemoteException;
/**
* uninstalls shared library
*
* @param sharedLibraryName
* name of the shared library
* @param targetName
* name of the target for this operation
* @return Map of targetName and shared library name strings.
* @throws JBIRemoteException
* on error
*/
public Map uninstallSharedLibrary(
String sharedLibraryName, String[] targetNames)
throws JBIRemoteException;
/**
* starts component ( service engine, binding component)
*
* @param componentName
* name of the component
* @param targetName
* name of the target for this operation
* @return Map of targetName and component name strings.
* @throws JBIRemoteException
* on error
*/
public Map startComponent(
String componentName, String[] targetNames)
throws JBIRemoteException;
/**
* stops component ( service engine, binding component)
*
* @param componentName
* name of the component
* @param targetName
* name of the target for this operation
* @return Map of targetName and component name strings.
* @throws JBIRemoteException
* on error
*/
public Map stopComponent(
String componentName, String[] targetNames)
throws JBIRemoteException;
/**
* shuts down component ( service engine, binding component)
*
* @param componentName
* name of the component
* @param targetName
* name of the target for this operation
* @return Map of targetName and component name strings.
* @throws JBIRemoteException
* on error
*/
public Map shutdownComponent(
String componentName, String[] targetNames)
throws JBIRemoteException;
/**
* shuts down component ( service engine, binding component)
*
* @param componentName
* name of the component
* @param force
* true if component should be shutdown in any case, else false.
* @param targetName
* name of the target for this operation
* @return Map of targetName and component name strings.
* @throws JBIRemoteException
* on error
*/
public Map shutdownComponent(
String componentName, boolean force, String[] targetNames)
throws JBIRemoteException;
/**
* deploys service assembly
*
* @param zipFilePath
* fie path
* @param targetName
* name of the target for this operation
* @return Map of targetName and management message xml text strings.
* @throws JBIRemoteException
* on error
*/
public Map deployServiceAssembly(
String zipFilePath, String[] targetNames) throws JBIRemoteException;
/**
* undeploys service assembly
*
* @param serviceAssemblyName
* name of the service assembly
* @param targetName
* name of the target for this operation
* @return Map of targetName and result as a management message xml text
* strings.
* @throws JBIRemoteException
* on error
*/
public Map undeployServiceAssembly(
String serviceAssemblyName, String[] targetNames)
throws JBIRemoteException;
/**
* starts service assembly
*
* @param serviceAssemblyName
* name of the service assembly
* @param targetName
* name of the target for this operation
* @return Map of targetName and result as a management message xml text
* strings.
* @throws JBIRemoteException
* on error
*/
public Map startServiceAssembly(
String serviceAssemblyName, String[] targetNames)
throws JBIRemoteException;
/**
* stops service assembly
*
* @param serviceAssemblyName
* name of the service assembly
* @param targetName
* name of the target for this operation
* @return Map of targetName and result as a management message xml text
* strings.
* @throws JBIRemoteException
* on error
*/
public Map stopServiceAssembly(
String serviceAssemblyName, String[] targetNames)
throws JBIRemoteException;
/**
* shuts down service assembly
*
* @param serviceAssemblyName
* name of the service assembly
* @param targetName
* name of the target for this operation
* @return Map of targetName and result as a management message xml text
* strings.
* @throws JBIRemoteException
* on error
*/
public Map shutdownServiceAssembly(
String serviceAssemblyName, String[] targetNames)
throws JBIRemoteException;
/**
* shuts down service assembly
*
* @param serviceAssemblyName
* name of the service assembly
* @param forceShutdown
* @param targetName
* name of the target for this operation
* @return Map of targetName and result as a management message xml text
* strings.
* @throws JBIRemoteException
* on error
*/
public Map shutdownServiceAssembly(
String serviceAssemblyName, boolean forceShutdown,
String[] targetNames) throws JBIRemoteException;
/**
* return component info xml text that has only service engine infos.
*
* @param targetName
* name of the target for this operation
* @return the component info xml text.
* @throws JBIRemoteException
* on error
*/
public Map listServiceEngines(
String[] targetNames) throws JBIRemoteException;
/**
* return component info xml text that has only service engine infos which
* satisfies the options passed to the method.
*
* @param state
* return all the service engines that are in the specified
* state. valid states are JBIComponentInfo.STARTED, STOPPED,
* INSTALLED or null for ANY state
* @param sharedLibraryName
* return all the service engines that have a dependency on the
* specified shared library. null value to ignore this option.
* @param serviceAssemblyName
* return all the service engines that have the specified service
* assembly deployed on them. null value to ignore this option.
* @param targetName
* name of the target for this operation
* @return xml text contain the list of service engine component infos
* @throws JBIRemoteException
* if error or exception occurs.
*/
public Map listServiceEngines(
String state, String sharedLibraryName, String serviceAssemblyName,
String[] targetNames) throws JBIRemoteException;
/**
* return component info xml text that has only binding component infos.
*
* @param targetName
* name of the target for this operation
* @return the component info xml text.
* @throws JBIRemoteException
* on error
*/
public Map listBindingComponents(
String[] targetNames) throws JBIRemoteException;
/**
* return component info xml text that has only binding component infos
* which satisfies the options passed to the method.
*
* @param state
* return all the binding components that are in the specified
* state. valid states are JBIComponentInfo.STARTED, STOPPED,
* INSTALLED or null for ANY state
* @param sharedLibraryName
* return all the binding components that have a dependency on
* the specified shared library. null value to ignore this
* option.
* @param serviceAssemblyName
* return all the binding components that have the specified
* service assembly deployed on them. null value to ignore this
* option.
* @param targetName
* name of the target for this operation
* @return xml text contain the list of binding component infos
* @throws JBIRemoteException
* if error or exception occurs.
*/
public Map listBindingComponents(
String state, String sharedLibraryName, String serviceAssemblyName,
String[] targetNames) throws JBIRemoteException;
/**
* returns a list of Binding Component and Service Engine infos in xml
* format, that are dependent upon a specified Shared Library
*
* @param sharedLibraryName
* the shared library name
* @param targetName
* name of the target for this operation
* @return xml string containing the list of componentInfos
* @throws JBIRemoteException
* on error
*/
public Map listSharedLibraryDependents(
String sharedLibraryName, String[] targetNames)
throws JBIRemoteException;
/**
* return component info xml text that has only shared library infos.
*
* @param targetName
* name of the target for this operation
* @return the component info xml text.
* @throws JBIRemoteException
* on error
*/
public Map listSharedLibraries(
String[] targetNames) throws JBIRemoteException;
/**
* returns the list of Shared Library infos in the in a xml format
*
* @param componentName
* to return only the shared libraries that are this component
* dependents. null for listing all the shared libraries in the
* schemaorg_apache_xmlbeans.system.
* @param targetName
* name of the target for this operation
* @return xml string contain the list of componentinfos for shared
* libraries.
* @throws JBIRemoteException
* if error or exception occurs.
*/
public Map listSharedLibraries(
String componentName, String[] targetNames)
throws JBIRemoteException;
/**
* returns a list of Service Assembly Infos in a xml format.
*
* @param targetName
* name of the target for this operation
* @return xml text containing the Service Assembly infos
* @throws JBIRemoteException
* on error
*/
public Map listServiceAssemblies(
String[] targetNames) throws JBIRemoteException;
/**
* returns the list of service asssembly infos in a xml format that have the
* service unit deployed on the specified component.
*
* @param componentName
* to list all the service assemblies that have some deployments
* on this component.
* @param targetName
* name of the target for this operation
* @return xml string contain the list of service assembly infos
* @throws JBIRemoteException
* if error or exception occurs.
*/
public Map listServiceAssemblies(
String componentName, String[] targetNames)
throws JBIRemoteException;
/**
* returns the list of service asssembly infos in a xml format that have the
* service unit deployed on the specified component.
*
* @param state
* to return all the service assemblies that are in the specified
* state. JBIServiceAssemblyInfo.STARTED, STOPPED, SHUTDOWN or
* null for ANY state
* @param componentName
* to list all the service assemblies that have some deployments
* on this component.
* @param targetName
* name of the target for this operation
* @return xml string contain the list of service assembly infos
* @throws JBIRemoteException
* if error or exception occurs.
*/
public Map listServiceAssemblies(
String state, String componentName, String[] targetNames)
throws JBIRemoteException;
/**
* return component info xml text for the specified service engine if
* exists. If no service engine with that name exists, it returns the xml
* with empty list.
*
* @param name
* name of the service engine to lookup
* @param state
* return service engine that is in the specified state. valid
* states are JBIComponentInfo.STARTED, STOPPED, INSTALLED or
* null for ANY state
* @param sharedLibraryName
* return service engine that has a dependency on the specified
* shared library. null value to ignore this option.
* @param serviceAssemblyName
* return the service engine that has the specified service
* assembly deployed on it. null value to ignore this option.
* @param targetName
* name of the target for this operation
* @return xml text contain the service engine component info that confirms
* to the component info list xml grammer.
* @throws JBIRemoteException
* if error or exception occurs.
*/
public Map showServiceEngine(
String name, String state, String sharedLibraryName,
String serviceAssemblyName, String[] targetNames)
throws JBIRemoteException;
/**
* return component info xml text for the specified binding component if
* exists. If no binding component with that name exists, it returns the xml
* with empty list.
*
* @param name
* name of the binding component to lookup
* @param state
* return the binding component that is in the specified state.
* valid states are JBIComponentInfo.STARTED, STOPPED, INSTALLED
* or null for ANY state
* @param sharedLibraryName
* return the binding component that has a dependency on the
* specified shared library. null value to ignore this option.
* @param serviceAssemblyName
* return the binding component that has the specified service
* assembly deployed on it. null value to ignore this option.
* @param targetName
* name of the target for this operation
* @return xml text contain the binding component info that confirms to the
* component info list xml grammer.
* @throws JBIRemoteException
* if error or exception occurs.
*/
public Map showBindingComponent(
String name, String state, String sharedLibraryName,
String serviceAssemblyName, String[] targetNames)
throws JBIRemoteException;
/**
* return component info xml text for the specified shared library if
* exists. If no shared library with that name exists, it returns the xml
* with empty list.
*
* @param name
* name of the shared library to lookup
* @param componentName
* return the shared library that is this component dependents.
* null to ignore this option.
* @param targetName
* name of the target for this operation
* @return xml string contain shared library component info that confirms to
* the component info list xml grammer.
* @throws JBIRemoteException
* if error or exception occurs.
*/
public Map showSharedLibrary(
String name, String componentName, String[] targetNames)
throws JBIRemoteException;
/**
* return service assembly info xml text for the specified service assembly
* if exists. If no service assembly with that name exists, it returns the
* xml with empty list.
*
* @param name
* name of the service assembly to lookup
* @param state
* return the service assembly that is in the specified state.
* JBIServiceAssemblyInfo.STARTED, STOPPED, SHUTDOWN or null for
* ANY state
* @param componentName
* return the service assembly that has service units on this
* component.
* @param targetName
* name of the target for this operation
* @return xml string contain service assembly info that confirms to the
* service assembly list xml grammer.
* @throws JBIRemoteException
* if error or exception occurs.
*/
public Map showServiceAssembly(
String name, String state, String componentName,
String[] targetNames) throws JBIRemoteException;
// ////////////////////////////////////////////////////////
// -- Operations on the Domain Administration Server --
// ////////////////////////////////////////////////////////
/**
* Checks to see if the JBI Runtime is enabled.
*
* @return true if JBI Runtime Framework is available, false if not
* @throws JBIRemoteException
* if error or exception occurs.
*/
public boolean isJBIRuntimeEnabled() throws JBIRemoteException;
/**
* Returns the jbi.xml Installation Descriptor for a Service Engine/Binding
* Component.
*
* @param the
* name of the ServiceEngine or Binding Component
* @return the jbi.xml deployment descriptor of the archive
* @throws JBIRemoteException
* if error or exception occurs.
*/
public String getComponentInstallationDescriptor(String componentName)
throws JBIRemoteException;
/**
* Returns the jbi.xml Installation Descriptor for a Shared Library.
*
* @param the
* name of the Shared Library
* @return the jbi.xml deployment descriptor of the archive
* @throws JBIRemoteException
* if error or exception occurs.
*/
public String getSharedLibraryInstallationDescriptor(
String sharedLibraryName) throws JBIRemoteException;
/**
* Returns the jbi.xml Deployment Descriptor for a Service Assembly.
*
* @param the
* name of the Service Assembly
* @return the jbi.xml deployment descriptor of the archive
* @throws JBIRemoteException
* if error or exception occurs.
*/
public String getServiceAssemblyDeploymentDescriptor(
String serviceAssemblyName) throws JBIRemoteException;
/**
* Returns the jbi.xml Deployment Descriptor for a Service Unit.
*
* @param the
* name of the Service Assembly
* @param the
* name of the Service Unit
* @return the jbi.xml deployment descriptor of the archive
* @throws JBIRemoteException
* if error or exception occurs.
*/
public String getServiceUnitDeploymentDescriptor(
String serviceAssemblyName, String serviceUnitName)
throws JBIRemoteException;
/**
* installs component ( service engine, binding component)
*
* @param componentName
* name of the component.
* @param targetName
* name of the target for this operation
* @return result.
* @throws JBIRemoteException
* on error
*/
public String installComponentFromDomain(String componentName,
String targetName) throws JBIRemoteException;
/**
* installs component ( service engine, binding component)
*
* @param componentName
* name of the component.
* @param component
* configuration properties
* @param targetName
* name of the target for this operation
* @return result.
* @throws JBIRemoteException
* on error
*/
public String installComponentFromDomain(String componentName,
Properties properties, String targetName) throws JBIRemoteException;
/**
* uninstalls component ( service engine, binding component)
*
* @param componentName
* name of the component
* @param forceDelete
* true to delete, false to not
* @param targetName
* name of the target for this operation
* @return Map of targetName and component name strings.
* @throws JBIRemoteException
* on error
*/
public String uninstallComponent(String componentName, boolean forceDelete,
String targetName) throws JBIRemoteException;
/**
* installs shared library
*
* @param libraryName
* Shared Library Name
* @param targetName
* name of the target for this operation
* @return Map of targetName and shared library name strings.
* @throws JBIRemoteException
* on error
*/
public String installSharedLibraryFromDomain(String libraryName,
String targetName) throws JBIRemoteException;
/**
* uninstalls shared library
*
* @param sharedLibraryName
* name of the shared library
* @param forceDelete
* true to delete, false to not
* @param targetName
* name of the target for this operation
* @return Map of targetName and shared library name strings.
* @throws JBIRemoteException
* on error
*/
public String uninstallSharedLibrary(String sharedLibraryName,
boolean forceDelete, String targetName) throws JBIRemoteException;
/**
* deploys service assembly
*
* @param assemblyName
* service assembly name
* @param targetName
* name of the target for this operation
* @return Map of targetName and management message xml text strings.
* @throws JBIRemoteException
* on error
*/
public String deployServiceAssemblyFromDomain(String assemblyName,
String targetName) throws JBIRemoteException;
/**
* undeploys service assembly
*
* @param serviceAssemblyName
* name of the service assembly
* @param forceDelete
* forces deletion of the assembly if true, false if not
* @param targetName
* name of the target for this operation
* @return Map of targetName and result as a management message xml text
* strings.
* @throws JBIRemoteException
* on error
*/
public String undeployServiceAssembly(String serviceAssemblyName,
boolean forceDelete, String targetName) throws JBIRemoteException;
/**
* installs component ( service engine, binding component)
*
* @param componentName
* name of the component
* @param targetNames
* array of targets for this operation
* @return Map of targetName and component name strings.
* @throws JBIRemoteException
* on error
*/
public Map installComponentFromDomain(
String componentName, String[] targetNames)
throws JBIRemoteException;
/**
* installs component ( service engine, binding component)
*
* @param componentName
* name of the component
* @param targetNames
* array of targets for this operation
* @param component
* configuration properties
* @return Map of targetName and component name strings.
* @throws JBIRemoteException
* on error
*/
public Map installComponentFromDomain(
String componentName, Properties properties, String[] targetNames)
throws JBIRemoteException;
/**
* uninstalls component ( service engine, binding component)
*
* @param componentName
* name of the component
* @param forceDelete
* true to delete, false to not
* @param targetName
* name of the target for this operation
* @return Map of targetName and component name strings.
* @throws JBIRemoteException
* on error
*/
public Map uninstallComponent(
String componentName, boolean forceDelete, String[] targetNames)
throws JBIRemoteException;
/**
* installs shared library
*
* @param libraryName
* name of the library
* @param targetName
* name of the target for this operation
* @return Map of targetName and shared library name strings.
* @throws JBIRemoteException
* on error
*/
public Map installSharedLibraryFromDomain(
String libraryName, String[] targetNames) throws JBIRemoteException;
/**
* uninstalls shared library
*
* @param sharedLibraryName
* name of the shared library
* @param forceDelete
* true to delete, false to not
* @param targetName
* name of the target for this operation
* @return Map of targetName and shared library name strings.
* @throws JBIRemoteException
* on error
*/
public Map uninstallSharedLibrary(
String sharedLibraryName, boolean forceDelete, String[] targetNames)
throws JBIRemoteException;
/**
* deploys service assembly
*
* @param assemblyName
* name of the service assembly
* @param targetName
* name of the target for this operation
* @return Map of targetName and management message xml text strings.
* @throws JBIRemoteException
* on error
*/
public Map deployServiceAssemblyFromDomain(
String assemblyName, String[] targetNames)
throws JBIRemoteException;
/**
* undeploys service assembly
*
* @param serviceAssemblyName
* name of the service assembly
* @param forceDelete
* true to delete, false to not
* @param targetName
* name of the target for this operation
* @return Map of targetName and result as a management message xml text
* strings.
* @throws JBIRemoteException
* on error
*/
public Map undeployServiceAssembly(
String serviceAssemblyName, boolean forceDelete,
String[] targetNames) throws JBIRemoteException;
// //////////////////////////////////
// Retain in Domain
// //////////////////////////////////
/**
* uninstalls component ( service engine, binding component)
*
* @param componentName
* name of the component
* @param forceDelete
* true to delete, false to not
* @param retainInDomain
* true to not delete it from the domain target, false to also
* delete it from the domain target.
* @param targetName
* name of the target for this operation
* @return component name string.
* @throws JBIRemoteException
* on error
*/
public String uninstallComponent(String componentName, boolean forceDelete,
boolean retainInDomain, String targetName)
throws JBIRemoteException;
/**
* uninstalls shared library
*
* @param sharedLibraryName
* name of the shared library
* @param forceDelete
* true to delete, false to not
* @param retainInDomain
* true to not delete it from the domain target, false to also
* delete it from the domain target.
* @param targetName
* name of the target for this operation
* @return shared library name string.
* @throws JBIRemoteException
* on error
*/
public String uninstallSharedLibrary(String sharedLibraryName,
boolean forceDelete, boolean retainInDomain, String targetName)
throws JBIRemoteException;
/**
* undeploys service assembly
*
* @param serviceAssemblyName
* name of the service assembly
* @param forceDelete
* forces deletion of the assembly if true, false if not
* @param retainInDomain
* true to not delete it from the domain target, false to also
* delete it from the domain target.
* @param targetName
* name of the target for this operation
* @return result as a management message xml text string.
* @throws JBIRemoteException
* on error
*/
public String undeployServiceAssembly(String serviceAssemblyName,
boolean forceDelete, boolean retainInDomain, String targetName)
throws JBIRemoteException;
/**
* uninstalls component ( service engine, binding component)
*
* @param componentName
* name of the component
* @param forceDelete
* true to delete, false to not
* @param retainInDomain
* true to not delete it from the domain target, false to also
* delete it from the domain target.
* @param targetNames
* array of targets for this operation
* @return Map of targetName and component name strings.
* @throws JBIRemoteException
* on error
*/
public Map uninstallComponent(
String componentName, boolean forceDelete, boolean retainInDomain,
String[] targetNames) throws JBIRemoteException;
/**
* uninstalls shared library
*
* @param sharedLibraryName
* name of the shared library
* @param forceDelete
* true to delete, false to not
* @param retainInDomain
* true to not delete it from the domain target, false to also
* delete it from the domain target.
* @param targetNames
* array of targets for this operation
* @return Map of targetName and shared library name strings.
* @throws JBIRemoteException
* on error
*/
public Map uninstallSharedLibrary(
String sharedLibraryName, boolean forceDelete,
boolean retainInDomain, String[] targetNames)
throws JBIRemoteException;
/**
* undeploys service assembly
*
* @param serviceAssemblyName
* name of the service assembly
* @param forceDelete
* forces deletion of the assembly if true, false if not
* @param retainInDomain
* true to not delete it from the domain target, false to also
* delete it from the domain target.
* @param targetNames
* array of targets for this operation
* @return Map of targetName and result as a management message xml text
* strings.
* @throws JBIRemoteException
* on error
*/
public Map undeployServiceAssembly(
String serviceAssemblyName, boolean forceDelete,
boolean retainInDomain, String[] targetNames)
throws JBIRemoteException;
/**
* Gets the component custom loggers and their levels
*
* @param componentName
* name of the component
* @param targetName
* name of the target (e.g., cluster1, server, etc.)
* @param targetInstanceName
* name of the target instance (e.g., cluster1-instance1, etc.)
* @return a Map of loggerCustomName to their log levels
* @throws JBIRemoteException
* on error
*/
public Map getComponentLoggerLevels(
String componentName, String targetName, String targetInstanceName)
throws JBIRemoteException;
/**
* Gets the component custom loggers and their display names.
*
* @param componentName
* name of the component
* @param targetName
* name of the target (e.g., cluster1, server, etc.)
* @param targetInstanceName
* name of the target instance (e.g., cluster1-instance1, etc.)
* @return a Map of loggerCustomName to their log levels
* @throws JBIRemoteException
* on error
*/
public Map getComponentLoggerDisplayNames(
String componentName, String targetName, String targetInstanceName)
throws JBIRemoteException;
/**
* Sets the component log level for a given custom logger
*
* @param componentName
* name of the component
* @param loggerCustomName
* the custom logger name
* @param logLevel
* the level to set the logger
* @param targetName
* name of the target (e.g., cluster1, server, etc.)
* @param targetInstanceName
* name of the target instance (e.g., cluster1-instance1, etc.)
* @throws JBIRemoteException
* on error
*/
public void setComponentLoggerLevel(String componentName,
String loggerCustomName, Level logLevel, String targetName,
String targetInstanceName) throws JBIRemoteException;
/**
* Gets all the runtime loggers and their levels
*
* @param targetName
* name of the target (e.g., cluster1, server, etc.)
* @param targetInstanceName
* name of the target instance (e.g., cluster1-instance1, etc.)
* @return a Map of loggerCustomName to their log levels
* @throws JBIRemoteException
* on error
*/
public Map getRuntimeLoggerLevels(
String targetName, String targetInstanceName)
throws JBIRemoteException;
/**
* Lookup the level of one runtime logger
*
* @param runtimeLoggerName
* name of the runtime logger (e.g. com.sun.jbi.framework
* @param targetName
* name of the target (e.g., cluster1, server, etc.)
* @param targetInstanceName
* name of the target instance (e.g., cluster1-instance1, etc.)
* @return a Map of loggerCustomName to their log levels
* @throws JBIRemoteException
* on error
*/
public Level getRuntimeLoggerLevel(
String runtimeLoggerName,
String targetName, String targetInstanceName)
throws JBIRemoteException;
/**
* Sets the log level for a given runtime logger
*
* @param runtimeLoggerName
* name of the runtime logger
* @param logLevel
* the level to set the logger
* @param targetName
* name of the target (e.g., cluster1, server, etc.)
* @param targetInstanceName
* name of the target instance (e.g., cluster1-instance1, etc.)
* @throws JBIRemoteException
* on error
*/
public void setRuntimeLoggerLevel(String runtimeLoggerName,
Level logLevel, String targetName, String targetInstanceName)
throws JBIRemoteException;
/**
* Gets all the runtime loggers and their display names
*
* @param targetName
* name of the target (e.g., cluster1, server, etc.)
* @param targetInstanceName
* name of the target instance (e.g., cluster1-instance1, etc.)
* @return a Map of display names to their logger names
* @throws JBIRemoteException
* on error
*/
public Map getRuntimeLoggerNames(
String targetName, String targetInstanceName)
throws JBIRemoteException;
/**
* Return the display name for a runtime logger
*
* @param runtimeLoggerName
* name of the logger (e.g. com.sun.jbi.framework)
* @param targetName
* name of the target (e.g., cluster1, server, etc.)
* @param targetInstanceName
* name of the target instance (e.g., cluster1-instance1, etc.)
* @return the display name for the given logger
* @throws JBIRemoteException
* on error
*/
public String getRuntimeLoggerDisplayName(String runtimeLoggerName,
String targetName, String targetInstanceName)
throws JBIRemoteException;
/**
* Gets all the runtime loggers and their levels
*
* @param targetName
* name of the target (e.g., cluster1, server, etc.)
* @return a Map of loggerCustomName to their log levels
* @throws JBIRemoteException
* on error
*/
public Map getRuntimeLoggerLevels(
String targetName)
throws JBIRemoteException;
/**
* Lookup the level of one runtime logger
*
* @param runtimeLoggerName
* name of the runtime logger (e.g. com.sun.jbi.framework
* @param targetName
* name of the target (e.g., cluster1, server, etc.)
* @return a Map of loggerCustomName to their log levels
* @throws JBIRemoteException
* on error
*/
public Level getRuntimeLoggerLevel(
String runtimeLoggerName,
String targetName)
throws JBIRemoteException;
/**
* Sets the log level for a given runtime logger
*
* @param runtimeLoggerName
* name of the runtime logger
* @param logLevel
* the level to set the logger
* @param targetName
* name of the target (e.g., cluster1, server, etc.)
* @throws JBIRemoteException
* on error
*/
public void setRuntimeLoggerLevel(String runtimeLoggerName,
Level logLevel, String targetName)
throws JBIRemoteException;
/**
* Gets all the runtime loggers and their display names
*
* @param targetName
* name of the target (e.g., cluster1, server, etc.)
* @return a Map of display names to their logger names
* @throws JBIRemoteException
* on error
*/
public Map getRuntimeLoggerNames(
String targetName)
throws JBIRemoteException;
/**
* Return the display name for a runtime logger
*
* @param runtimeLoggerName
* name of the logger (e.g. com.sun.jbi.framework)
* @param targetName
* name of the target (e.g., cluster1, server, etc.)
* @return the display name for the given logger
* @throws JBIRemoteException
* on error
*/
public String getRuntimeLoggerDisplayName(String runtimeLoggerName,
String targetName)
throws JBIRemoteException;
/**
* Gets the extension MBean object names
*
* @param componentName
* name of the component
* @param extensionName
* the name of the extension (e.g., Configuration, Logger, etc.)
* @param targetName
* name of the target (e.g., cluster1, server, etc.)
* @return
* @throws JBIRemoteException
* on error
*/
public Map getComponentExtensionMBeanObjectNames(
String componentName, String extensionName, String targetName)
throws JBIRemoteException;
/**
* Gets the extension MBean object names
*
* @param componentName
* name of the component
* @param extensionName
* the name of the extension (e.g., Configuration, Logger, etc.)
* @param targetName
* name of the target (e.g., cluster1, server, etc.)
* @param targetInstanceName
* name of the target instance (e.g., cluster1-instance1, etc.)
* @return an array of ObjectName(s)
* @throws JBIRemoteException
* on error
*/
public ObjectName[] getComponentExtensionMBeanObjectNames(
String componentName, String extensionName, String targetName,
String targetInstanceName) throws JBIRemoteException;
/**
* Retrieve component configuration
*
* @param componentName
* @param targetName
* @return the targetName as key and the name/value pairs as properties
*/
Properties getComponentConfiguration(
String componentName, String targetName) throws JBIRemoteException;
/**
* Will return jbi mgmt message with success, failure, or partial success
* per instance. The entry per instance will have value as part of the
* management message (XML) String.
*
* @param targetName the name of the JBI target
* @param componentName the component name
* @param configurationValues the configuration properties
* @return
*/
public String setComponentConfiguration(String targetName,
String componentName, Properties configurationValues)
throws JBIRemoteException;
/**
* updates component ( service engine, binding component)
*
* @param componentName
* Name of the component to update.
* @param zipFilePath
* archive file in a zip format
* @return The name of the component if successful
* @throws JBIRemoteException
* on error
*/
public String updateComponent(String componentName, String zipFilePath)
throws JBIRemoteException;
/**
* Checks to see if the Target (server, cluster) is up or down.
*
* @param targetName
* name of the target (e.g., cluster1, server, etc.)
* @return true if Target is up, false if not
* @throws JBIRemoteException
* if error or exception occurs.
*/
public boolean isTargetUp(String targetName) throws JBIRemoteException;
/**
* This method returns the runtime configuration metadata associated with
* the specified property. The metadata contain name-value pairs like:
* default, descriptionID, descriptorType, displayName, displayNameId,
* isStatic, name, resourceBundleName, tooltip, tooltipId, etc.
*
* @param propertyKeyName
* @return Properties that represent runtime configuration metadata
* @throws JBIRemoteException
*/
Properties getRuntimeConfigurationMetaData(String propertyKeyName)
throws JBIRemoteException;
/**
* This method sets one or more configuration parameters on the runtime with
* a list of name/value pairs passed as a properties object. The property
* name in the properties object should be an existing configuration
* parameter name. If user try to set the parameter that is not in the
* configuration parameters list, this method will throw an exception.
*
* The value of the property can be any object. If the value is non string
* object, its string value (Object.toString()) will be used as a value that
* will be set on the configuration.
*
* This method first validates whether all the paramters passed in
* properties object exist in the runtime configuration or not. If any one
* the parameters passed is not existing, it will return an error without
* settings the parameters that are passed in the properties including a
* valid parameters.
*
* If there is an error in setting a paramter, this method throws an
* exception with the list of parameters that were not set.
*
* @param params
* Properties object that contains name/value pairs corresponding
* to the configuration parameters to be set on the runtime.
*
* @param targetName
* cluster or instance name ( e.g. cluster1, instance1 ) on which
* configuration parameters will be set. null to represent the
* default instance which is admin server
*
* @return true if server restart is required, false if not
*
* @throws JBIRemoteException
* if there is a jmx error or a invalid parameter is passed in
* the params properties object. In case of an error setting the
* a particular parameter, the error message should list the
* invalid parameters.
*/
public boolean setRuntimeConfiguration(Properties parameters,
String targetName) throws JBIRemoteException;
/**
* This method returns a tabular data of a complex open data objects that
* represent the runtime configuration parameter descriptor. The parameter
* descriptor should contain the following data that represents the
* parameter.
*
* name : name of the parameter value : value of the parameter as a String
* type. type : type of the parameter. Basic data types only. description:
* (optional) description of the parameter. displayName: (optional) display
* name of the parameter readOnly : true/false validValues : (optional) list
* of string values with ',' as delimiter. or a range value with - with a
* '-' as delimiter. min and max strings will be converted to the parameter
* type and then used to validate the value of the parameter.
*
* @param targetName
* cluster or instance name ( e.g. cluster1, instance1 ) on which
* configuration parameters will be set. null to represent the
* default instance which is admin server
*
* @return Properties that represents the list of configuration parameter
* descriptors.
*
* @throws JBIRemoteException
* if there is a jmx error accessing the instance
*/
public Properties getRuntimeConfiguration(String targetName)
throws JBIRemoteException;
/**
* This method returns a tabular data of a complex open data objects that
* represent the runtime configuration parameter descriptor. The parameter
* descriptor should contain the following data that represents the
* parameter.
*
* name : name of the parameter value : value of the parameter as a String
* type. type : type of the parameter. Basic data types only. description:
* (optional) description of the parameter. displayName: (optional) display
* name of the parameter readOnly : true/false validValues : (optional) list
* of string values with ',' as delimiter. or a range value with - with a
* '-' as delimiter. min and max strings will be converted to the parameter
* type and then used to validate the value of the parameter.
*
* @param targetName
* cluster or instance name ( e.g. cluster1, instance1 ) on which
* configuration parameters will be set. null to represent the
* default instance which is admin server
*
* @return Properties that represents the list of configuration parameter
* descriptors.
*
* @throws JBIRemoteException
* if there is a jmx error accessing the instance
*/
public Properties getDefaultRuntimeConfiguration()
throws JBIRemoteException;
/**
* checks if the server need to be restarted to apply the changes made to
* some of the configuration parameters.
*
* @return true if server needs to be restarted for updated configuration to
* take effect. false if no server restart is needed.
*/
public boolean isServerRestartRequired() throws JBIRemoteException;
/**
* This method is used to verify if the application variables and
* application configuration objects used in the given
* application are available in JBI runtime in the specified target.
* Also this method verifies if all necessary components are installed.
* If generateTemplates is true templates for missing application variables
* and application configurations are generated. A command script that uses
* the template files to set configuration objects is generated.
*
* @param applicationURL the URL for the application zip file
* @param targetName the target on which the application has to be verified
* @param generateTemplates true if templates have to be generated
* @param templateDir the dir to store the generated templates
* @param includeDeployCommand true if the generated script should include
* deploy command
*
* @returns CompositeData the verification report
*
* CompositeType of verification report
* String - "ServiceAssemblyName",
* String - "ServiceAssemblyDescription",
* Integer - "NumServiceUnits",
* Boolean - "AllComponentsInstalled",
* String[] - "MissingComponentsList",
* CompositeData[] - "EndpointInfo",
* CompositeData[] - "JavaEEVerifierReport"
* String - "TemplateZIPID"
*
* CompositeType of each EndpointInfo
* String - "EndpointName",
* String - "ServiceUnitName",
* String - "ComponentName",
* String - "Status"
* String[] - "MissingApplicationVariables"
* String[] - "MissingApplicationConfigurations"
*
* CompositeType of each JavaEEVerifierReport
* String - "ServiceUnitName"
* TabularData - "JavaEEVerifierReport"
*
* TabularType of each JavaEEVerifierReport
*
* SimpleType.STRING - "Ear Filename"
* SimpleType.STRING - "Referrence By"
* SimpleType.STRING - "Referrence Class"
* SimpleType.STRING - "JNDI Name"
* SimpleType.STRING - "JNDI Class Type"
* SimpleType.STRING - "Message"
* SimpleType.INTEGER - "Status"
*
* @throws JBIRemoteException if the application could not be verified
*
* Note: param templateDir is used between ant/cli and common client client
* TemplateZIPID is used between common client server and common client client
*/
public CompositeData verifyApplication(
String applicationURL,
String targetName,
boolean generateTemplates,
String templateDir,
boolean includeDeployCommand)
throws JBIRemoteException;
/**
* This method is used to export the application variables and
* application configuration objects used by the given
* application in the specified target.
*
* @param applicationName the name of the application
* @param targetName the target whose configuration has to be exported
* @param configDir the dir to store the configurations
* @return String the id for the zip file with exported configurations
*
* @throws JBIRemoteException if the application configuration could not be exported
*
* Note: param configDir is used between ant/cli and common client client.
* The return value is used between common client server and common client client.
*/
public String exportApplicationConfiguration(
String applicationName,
String targetName,
String configDir)
throws JBIRemoteException;
/*----------------------------------------------------------------------------------*\
* Operations for Application Variable Management *
\*----------------------------------------------------------------------------------*/
/**
* Detect the components support for application variables. This method
* returns true if the component has a configuration MBean and implements
* all the operations for application variable management.
*
* @param componentName
* component identification
* @param targetName
* identification of the target. Can be a standalone server,
* cluster or clustered instance.
* @return true if the components configuration MBean implements all the
* operations for application variables.
* @throws JBIRemoteException if the component is not installed or is not
* in the Started state.
*
*/
public boolean isAppVarsSupported(String componentName, String targetName)
throws JBIRemoteException;
/**
* Add application variables to a component installed on a given target. If even a
* variable from the set is already set on the component, this operation fails.
*
* @param componentName
* component identification
* @param targetName
* identification of the target. Can be a standalone server,
* cluster or clustered instance.
* @param appVariables - set of application variables to add. The values of the
* application variables have the application variable type and
* value and the format is "[type]value"
* @return
* a JBI Management message indicating the status of the operation.
* In case a variable is not added the management message has a
* ERROR task status message giving the details of the failure.
* @throws JBIRemoteException
* if there is a jmx error accessing the instance
*/
public String addApplicationVariables (String componentName, String targetName,
Properties appVariables) throws JBIRemoteException;
/**
* Set application variables on a component installed on a given target. If even a
* variable from the set has not been added to the component, this operation fails.
*
* @param componentName
* component identification
* @param targetName
* identification of the target. Can be a standalone server,
* cluster or clustered instance.
* @param appVariables - set of application variables to update. The values of the
* application variables have the application variable type and
* value and the format is "[type]value"
* @return
* a JBI Management message indicating the status of the operation.
* In case a variable is not set the management message has a
* ERROR task status message giving the details of the failure.
* @throws JBIRemoteException
* if there is a jmx error accessing the instance
*/
public String setApplicationVariables (String componentName, String targetName,
Properties appVariables) throws JBIRemoteException;
/**
* Delete application variables from a component installed on a given target. If even a
* variable from the set has not been added to the component, this operation fails.
*
* @param componentName
* component identification
* @param targetName
* identification of the target. Can be a standalone server,
* cluster or clustered instance.
* @param appVariableNames - names of application variables to delete.
* @return
* a JBI Management message indicating the status of the operation.
* In case a variable is not deleted the management message has a
* ERROR task status message giving the details of the failure.
* @throws JBIRemoteException
* if there is a jmx error accessing the instance
*/
public String deleteApplicationVariables (String componentName, String targetName,
String[] appVariableNames) throws JBIRemoteException;
/**
* Get all the application variables set on a component.
*
* @return all the application variables et on the component. The return proerties
* set has the name="[type]value" pairs for the application variables.
* @return
* a JBI Management message indicating the status of the operation.
* @throws JBIRemoteException
* if there is a jmx error accessing the instance
*/
public Properties getApplicationVariables (String componentName, String targetName)
throws JBIRemoteException;
/*----------------------------------------------------------------------------------*\
* Operations for Application Configuration Management *
\*----------------------------------------------------------------------------------*/
/**
* Detect the components support for application configuration. This method
* returns true if the component has a configuration MBean and implements
* all the operations for application configuration management.
*
* @param componentName
* component identification
* @param targetName
* identification of the target. Can be a standalone server,
* cluster or clustered instance.
* @return true if the components configuration MBean implements all the
* operations for application configuration.
* @throws JBIRemoteException if the component is not installed or is not
* in the Started state.
*
*/
public boolean isAppConfigSupported(String componentName, String targetName)
throws JBIRemoteException;
/**
* Get the CompositeType definition for the components application configuration
*
* @param componentName
* component identification
* @param targetName
* identification of the target. Can be a standalone server,
* cluster or clustered instance.
* @return the CompositeType for the components application configuration.
*/
public javax.management.openmbean.CompositeType queryApplicationConfigurationType
(String componentName, String targetName)
throws JBIRemoteException;
/**
* Add a named application configuration to a component installed on a given target.
*
* @param componentName
* component identification
* @param targetName
* identification of the target. Can be a standalone server,
* cluster or clustered instance.
* @param name
* application configuration name
* @param config
* application configuration represented as a set of properties.
* @return
* a JBI Management message indicating the status of the operation.
* @throws JBIRemoteException
* if there is a jmx error accessing the instance
*/
public String addApplicationConfiguration (String componentName,
String targetName, String name, Properties config)
throws JBIRemoteException;
/**
* Update a named application configuration in a component installed on a given target.
*
* @param componentName
* component identification
* @param targetName
* identification of the target. Can be a standalone server,
* cluster or clustered instance.
* @param name
* application configuration name
* @param config
* application configuration represented as a set of properties.
* @return
* a JBI Management message indicating the status of the operation.
* @throws JBIRemoteException
* if there is a jmx error accessing the instance
*/
public String setApplicationConfiguration (String componentName,
String targetName, String name, Properties config)
throws JBIRemoteException;
/**
* Delete a named application configuration in a component installed on a given target.
*
* @param componentName
* component identification
* @param targetName
* identification of the target. Can be a standalone server,
* cluster or clustered instance.
* @param name
* name of application configuration to be deleted
* @return
* a JBI Management message indicating the status of the operation.
* @throws JBIRemoteException
* if there is a jmx error accessing the instance
*/
public String deleteApplicationConfiguration (String componentName,
String targetName, String name)
throws JBIRemoteException;
/**
* List all the application configurations in a component.
*
* @param componentName
* component identification
* @param targetName
* identification of the target. Can be a standalone server,
* cluster or clustered instance.
* @return
* an array of names of all the application configurations.
* @throws JBIRemoteException
* if there is a jmx error accessing the instance
*/
public String[] listApplicationConfigurationNames (String componentName, String targetName)
throws JBIRemoteException;
/**
* Get a specific named configuration. If the named configuration does not exist
* in the component the returned properties is an empty set.
*
* @param componentName
* component identification
* @param targetName
* identification of the target. Can be a standalone server,
* cluster or clustered instance.
* @return the application configuration represented as a set of properties.
* @throws JBIRemoteException
* if there is a jmx error accessing the instance
*/
public Properties getApplicationConfiguration (String componentName, String targetName, String name)
throws JBIRemoteException;
/**
* Get all the application configurations set on a component.
*
* @param componentName
* component identification
* @param targetName
* identification of the target. Can be a standalone server,
* cluster or clustered instance.
* @return
* a map of all the application configurations keyed by the
* configuration name.
* @throws JBIRemoteException
* if there is a jmx error accessing the instance
*/
public Map getApplicationConfigurations (String componentName, String targetName)
throws JBIRemoteException;
/**
* Retrieves the component specific configuration schema.
*
* @param componentName
* component identification
* @param targetName
* identification of the target. Can be a standalone server,
* cluster or clustered instance.
* @return a String containing the configuration schema.
*/
public String retrieveConfigurationDisplaySchema(String componentName, String targetname)
throws JBIRemoteException;;
/**
* Retrieves the component configuration metadata.
* The XML data conforms to the component
* configuration schema.
*
* @param componentName
* component identification
* @param targetName
* identification of the target. Can be a standalone server,
* cluster or clustered instance.
* @return a String containing the configuration metadata.
*/
public String retrieveConfigurationDisplayData(String componentName, String targetName)
throws JBIRemoteException;
/**
* Detect the components support for component configuration. This method
* returns true if the component has a configuration MBean with configurable
* attributes
*
* @param componentName
* component identification
* @param targetName
* identification of the target. Can be a standalone server,
* cluster or clustered instance.
* @return true if the components configuration MBean has configuration
* attributes
* @throws JBIRemoteException if the component is not installed or is not
* in the Started state.
*
*/
public boolean isComponentConfigSupported(String componentName, String targetName)
throws JBIRemoteException;
/**
* This method is used to provide JBIFramework statistics in the
* given target.
* @param target target name.
* @return TabularData table of framework statistics in the given target.
*
* If the target is a standalone instance the table will have one entry.
* If the target is a cluster the table will have an entry for each instance.
*
* For more information about the type of the entries in table please refer
* to JBIStatisticsMBean
*/
public TabularData getFrameworkStats(String targetName)
throws JBIRemoteException;
/**
* This method is used to provide statistics for the given component
* in the given target
* @param targetName target name
* @param componentName component name
* @return TabularData table of component statistics
*
* If the target is a standalone instance the table will have one entry.
* If the target is a cluster the table will have an entry for each instance.
*
* For more information about the type of the entries in table please refer
* to JBIStatisticsMBean
*
*/
public TabularData getComponentStats(String componentName, String targetName)
throws JBIRemoteException;
/**
* This method is used to provide statistic information about the given
* endpoint in the given target
* @param targetName target name
* @param endpointName the endpoint Name
* @return TabularData table of endpoint statistics
*
* If the target is a standalone instance the table will have one entry.
* If the target is a cluster the table will have an entry for each instance.
*
* For more information about the type of the entries in table please refer
* to JBIStatisticsMBean
*/
public TabularData getEndpointStats(String endpointName, String targetName)
throws JBIRemoteException;
/**
* This method is used to provide statistics about the message service in the
* given target.
* @param target target name.
* @return TabularData table of NMR statistics in the given target.
*
* If the target is a standalone instance the table will have one entry.
* If the target is a cluster the table will have an entry for each instance.
*
* For more information about the type of the entries in table please refer
* to JBIStatisticsMBean
*/
public TabularData getNMRStats(String targetName)
throws JBIRemoteException;
/**
* This method is used to provide statistics about a Service Assembly
* in the given target.
* @param target target name.
* @param saName the service assembly name.
* @return TabularData table of NMR statistics in the given target.
*
* If the target is a standalone instance the table will have one entry.
* If the target is a cluster the table will have an entry for each instance.
*
* For more information about the type of the entries in table please refer
* to JBIStatisticsMBean
*/
public TabularData getServiceAssemblyStats(String saName, String targetName)
throws JBIRemoteException;
/**
* This method is used to provide a list of consuming endpoints for a component.
* @param componentName component name
* @param target target name.
* @return TabularData list of consuming endpoints
* @throws JBIRemoteException if the list of endpoints could not be obtained.
*
* If the target is a standalone instance the table will have one entry.
* If the target is a cluster the table will have an entry for each instance.
*
* Each entry in this tabular data is of the following composite type
*
* String - "InstanceName",
* String[] - "Endpoints",
*/
public TabularData getConsumingEndpointsForComponent(String componentName, String targetName)
throws JBIRemoteException;
/**
* This method is used to provide a list of provisioning endpoints for a component.
* @param componentName component name
* @param target target name.
* @return TabularData list of provisioning endpoints
* @throws JBIRemoteException if the list of endpoints could not be obtained.
*
* If the target is a standalone instance the table will have one entry.
* If the target is a cluster the table will have an entry for each instance.
*
* Each entry in this tabular data is of the following composite type
*
* String - "InstanceName",
* String[] - "Endpoints",
*/
public TabularData getProvidingEndpointsForComponent(String componentName, String targetName)
throws JBIRemoteException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy