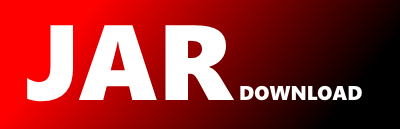
com.sun.jbi.ui.common.JBIArchive Maven / Gradle / Ivy
/*
* BEGIN_HEADER - DO NOT EDIT
*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the "License"). You may not use this file except
* in compliance with the License.
*
* You can obtain a copy of the license at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* HEADER in each file and include the License file at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* If applicable add the following below this CDDL HEADER,
* with the fields enclosed by brackets "[]" replaced with
* your own identifying information: Portions Copyright
* [year] [name of copyright owner]
*/
/*
* @(#)JBIArchive.java
* Copyright 2004-2007 Sun Microsystems, Inc. All Rights Reserved.
*
* END_HEADER - DO NOT EDIT
*/
package com.sun.jbi.ui.common;
import java.io.File;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.jar.JarEntry;
import java.util.jar.JarFile;
/**
* This class reads the jar file and identifies the jbi artifacts
* like jbi descriptors.
*
* @author Sun Microsystems, Inc.
*/
public class JBIArchive
{
/**
* jar file
*/
private JarFile mJarFile;
/**
* jbi descriptor
*/
private JBIDescriptor mJbiDescriptor;
/**
* Creates a new instance of JBIArchive
* @param pathName path name
* @throws IOException on error
*/
public JBIArchive(String pathName) throws IOException
{
this(new File(pathName));
}
/**
* Creates a new instance of JBIArchive
* @param file file
* @throws IOException on error
*/
public JBIArchive(File file) throws IOException
{
this( new JarFile(file));
}
/**
* Creates a new instance of JBIArchive
* @param jarFile jar file
* @throws IOException on error
*/
public JBIArchive(JarFile jarFile) throws IOException
{
this.mJarFile = jarFile;
validate();
}
/**
* validates the archive
* @throws IOException on error
*/
private void validate() throws IOException
{
// TODO schema validate the jbi.xml on tools side in JbiDescriptor
// throws exception if it does not find the jbi.xml in /META-INF or not
// valid according to JBIDescriptor.
try
{
JBIDescriptor jbiDD = getJbiDescriptor();
}
catch (IOException ioEx)
{
// rethrow
throw ioEx;
}
catch (Exception ex)
{
throw new IOException(ex.getMessage());
}
}
/**
* returns JarFile Object for this archive
* @throws IOException on error
* @return jar file
*/
public JarFile getJarFile() throws IOException
{
return this.mJarFile;
}
/**
* returns the jbi descriptor object
* @return jbi descriptor
* @throws Exception on error
*/
public JBIDescriptor getJbiDescriptor() throws Exception
{
if ( this.mJbiDescriptor == null )
{
JarEntry jbiXmlEntry = this.mJarFile.getJarEntry("META-INF/jbi.xml");
if ( jbiXmlEntry != null )
{
InputStreamReader reader = null;
try
{
reader =
new InputStreamReader(this.mJarFile.getInputStream(jbiXmlEntry));
this.mJbiDescriptor = JBIDescriptor.createJBIDescriptor(reader);
}
finally
{
if ( reader != null )
{
try
{
reader.close();
}
catch (Exception ex)
{
// ignore
}
}
try
{
this.mJarFile.close();
}
catch (Exception ex)
{
// ignore
}
}
}
else
{
throw new IOException(
JBIResultXmlBuilder.createFailedJbiResultXml(Util.getCommonI18NBundle(),
"jbi.xml.not.found.in.jbi.archive", null));
}
}
return this.mJbiDescriptor;
}
/**
* checks if it is a shared library archive
* @return true if it is shared library else false
*/
public boolean isSharedLibraryArchive()
{
try
{
JBIDescriptor jbiDesc = getJbiDescriptor();
return ( jbiDesc != null && jbiDesc.isSharedLibraryDescriptor() );
}
catch (Exception ex)
{
return false;
}
}
/**
* checks if it is a service assembly archive
* @return true if it is service assembly else false
*/
public boolean isServiceAssemblyArchive()
{
try
{
JBIDescriptor jbiDesc = getJbiDescriptor();
return ( jbiDesc != null && jbiDesc.isServiceAssemblyDescriptor() );
}
catch (Exception ex)
{
return false;
}
}
/**
* checks if it is a service engine archive
* @return true if it is service engine archive else fasle.
*/
public boolean isServiceEngineArchive()
{
try
{
JBIDescriptor jbiDesc = getJbiDescriptor();
return ( jbiDesc != null && jbiDesc.isServiceEngineDescriptor() );
}
catch (Exception ex)
{
return false;
}
}
/**
* checks it is a binding component archive
* @return true if it is bc archive else false
*/
public boolean isBindingComponentArchive()
{
try
{
JBIDescriptor jbiDesc = getJbiDescriptor();
return ( jbiDesc != null && jbiDesc.isBindingComponentDescriptor() );
}
catch (Exception ex)
{
return false;
}
}
}