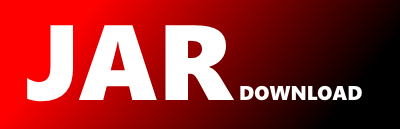
com.sun.jbi.ui.common.JBIComponentInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jbi-admin-common Show documentation
Show all versions of jbi-admin-common Show documentation
The "jbi-admin-common" Common CLI code
The newest version!
/*
* BEGIN_HEADER - DO NOT EDIT
*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the "License"). You may not use this file except
* in compliance with the License.
*
* You can obtain a copy of the license at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* HEADER in each file and include the License file at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* If applicable add the following below this CDDL HEADER,
* with the fields enclosed by brackets "[]" replaced with
* your own identifying information: Portions Copyright
* [year] [name of copyright owner]
*/
/*
* @(#)JBIComponentInfo.java
* Copyright 2004-2007 Sun Microsystems, Inc. All Rights Reserved.
*
* END_HEADER - DO NOT EDIT
*/
package com.sun.jbi.ui.common;
import java.io.PrintWriter;
import java.io.Reader;
import java.io.StringReader;
import java.io.StringWriter;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.List;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.NodeList;
/** This class reads/writes the component info list xml.
*
* @author Sun Microsystems, Inc.
*/
public class JBIComponentInfo
{
/** namespace */
public static final String XMLNS = "http://java.sun.com/xml/ns/jbi/component-info-list";
public static final String XMLNS_VERSION = "http://java.sun.com/xml/ns/jbi/component-info-list/version";
/** unknown type */
public static final String UNKNOWN_TYPE = "unknown";
/** Binding type */
public static final String BINDING_TYPE = "binding-component";
/** Engine Type */
public static final String ENGINE_TYPE = "service-engine";
/** Namespace Type */
public static final String SHARED_LIBRARY_TYPE = "shared-library";
/** state Loaded status. */
public static final String UNKNOWN_STATE = "Unknown";
/** Installed status */
public static final String SHUTDOWN_STATE = "Shutdown";
/** Stopped status */
public static final String STOPPED_STATE = "Stopped";
/** Started status */
public static final String STARTED_STATE = "Started";
/**
* Holds value of property name.
*/
private String mName;
/**
* Holds value of property state.
*/
private String mState;
/**
* Holds value of property description.
*/
private String mDescription;
/**
* Holds value of property type.
*/
private String mType;
/**
* Holds value of property type.
*/
private String mComponentVersion;
/**
* Holds value of property type.
*/
private String mBuildNumber;
/** Creates a new instance of JBIComponentInfo */
public JBIComponentInfo()
{
this.mType = "";
this.mState = "";
this.mName = "";
this.mDescription = "";
this.mComponentVersion = "";
this.mBuildNumber = "";
}
/** Creates a new instance of JBIComponentInfo
* @param type type
* @param state state
* @param name name
* @param description description
* @param componentVersion component version
* @param buildNumber builder number
*/
public JBIComponentInfo(String type, String state,
String name, String description)
{
this.mType = type;
this.mState = state;
this.mName = name;
this.mDescription = description;
}
/**
* Getter for property aliasName.
* @return Value of property aliasName.
*/
public String getName()
{
return this.mName;
}
/**
* Setter for property aliasName.
* @param name New value of property aliasName.
*/
public void setName(String name)
{
this.mName = name;
}
/**
* Getter for property state.
* @return Value of property state.
*/
public String getState()
{
return this.mState;
}
/**
* Setter for property state.
* @param state New value of property state.
*/
public void setState(String state)
{
this.mState = state;
}
/**
* Getter for property description.
* @return Value of property description.
*/
public String getDescription()
{
return this.mDescription;
}
/**
* Setter for property description.
* @param description New value of property description.
*/
public void setDescription(String description)
{
this.mDescription = description;
}
/**
* Getter for property type.
* @return Value of property type.
*/
public String getType()
{
return this.mType;
}
/**
* Setter for property type.
* @param type New value of property type.
*/
public void setType(String type)
{
this.mType = type;
}
/**
* Getter for property component version.
* @return Value of property component version.
*/
public String getComponentVersion()
{
return this.mComponentVersion;
}
/**
* Setter for property version.
* @param type New value of property version.
*/
public void setComponentVersion(String componentVersion)
{
this.mComponentVersion = componentVersion;
}
/**
* Getter for property builder number.
* @return Value of property build number.
*/
public String getBuildNumber()
{
return this.mBuildNumber;
}
/**
* Setter for property build number.
* @param type New value of property build number.
*/
public void setBuildNumber(String buildNumber)
{
this.mBuildNumber = buildNumber;
}
/** Return the localized state value
* @return localized state value
*/
public String getLocalizedState()
{
String localizedState = Util.getCommonI18NBundle().getMessage(this.getState());
return localizedState;
}
/** string value
* @return string value
*/
public String toString()
{
return
"\nType = " +
this.getType() +
"\nName = " +
this.getName() +
"\nDescription = " +
this.getDescription() +
"\nState = " +
this.getState() +
"\nCompoent Version = " +
this.getComponentVersion() +
"\nBuild Number = " +
this.getBuildNumber();
}
/** xml text
* @return xml text
*/
public String toXmlString()
{
String returnStr = "";
returnStr = "\n" +
"" + this.getDescription() + " \n";
if ( ((""+getComponentVersion()).compareTo("") > 0) ||
((""+getBuildNumber()).compareTo("") > 0) )
{
returnStr += " 0)
{
returnStr += " component-version=\"" + getComponentVersion() + "\"";
}
if ((""+getBuildNumber()).compareTo("") > 0)
{
returnStr += " build-number=\"" + getBuildNumber() + "\"";
}
returnStr += "/> \n";
}
returnStr += " ";
return returnStr;
}
/** return component info object
* @param compInfoElement xml element
* @return object
*/
public static JBIComponentInfo createJbiComponentInfo(
Element compInfoElement )
{
JBIComponentInfo info = new JBIComponentInfo();
String type = compInfoElement.getAttribute("type");
info.setType(type);
String name = compInfoElement.getAttribute("name");
info.setName(name);
String state = compInfoElement.getAttribute("state");
info.setState(state);
String desc =
DOMUtil.UTIL.getTextData(
DOMUtil.UTIL.getElement(compInfoElement, "description"));
info.setDescription(desc);
NodeList tmpNodeList =
compInfoElement.getElementsByTagNameNS(
XMLNS_VERSION,
"VersionInfo");
if ((tmpNodeList != null) && (tmpNodeList.getLength()> 0))
{
Element verInfoEle = (Element) tmpNodeList.item(0);
if (verInfoEle != null)
{
String compVer = verInfoEle.getAttribute("component-version");
if ((""+compVer).compareTo("") > 0)
{
info.setComponentVersion(compVer);
}
String buildNumber = verInfoEle.getAttribute("build-number");
if ((""+buildNumber).compareTo("") > 0)
{
info.setBuildNumber(buildNumber);
}
}
}
return info;
}
/** creates list of objects
* @param xmlReader Reader
* @return list of objects
*/
public static List readFromXmlText(Reader xmlReader)
{
ArrayList list = new ArrayList();
Document xmlDoc = null;
try
{
xmlDoc = DOMUtil.UTIL.buildDOMDocument(xmlReader, true);
}
catch (Exception ex)
{
Util.logDebug(ex);
return list;
}
if ( xmlDoc == null )
{
return list; // return empty list
}
// construct the compInfo list
Element compInfoListElement = DOMUtil.UTIL.getElement(xmlDoc, "component-info-list");
if ( compInfoListElement == null )
{
// System.out.println("No Root Element ");
return list; // return empty list
}
NodeList compInfoList =
DOMUtil.UTIL.getChildElements(compInfoListElement, "component-info");
int size = compInfoList.getLength();
// System.out.println("compInfo found in XML Document : " + size);
for ( int i = 0; i < size; ++i )
{
Element compInfoElement = (Element) compInfoList.item(i);
if ( compInfoElement != null )
{
JBIComponentInfo compInfo =
createJbiComponentInfo(compInfoElement);
list.add(compInfo);
}
}
JBIComponentInfo.sort(list);
return list;
}
/** creates list of objects
* @param xmlText xml text
* @return list of objects
*/
public static List readFromXmlText(String xmlText)
{
return readFromXmlText(new StringReader(xmlText));
}
/** write to xml text
* @param compInfoList list of objects
* @return xml text
*/
public static String writeAsXmlText(List compInfoList)
{
JBIComponentInfo.sort(compInfoList);
StringWriter stringWriter = new StringWriter();
PrintWriter writer = new PrintWriter(stringWriter);
// begine xml
writer.println("");
writer.println("");
int size = compInfoList.size();
for (int i=0; i < size; ++i )
{
JBIComponentInfo info = (JBIComponentInfo) compInfoList.get(i);
writer.println(info.toXmlString());
}
writer.println(" ");
// end xml
try
{
writer.close();
stringWriter.close();
}
catch ( Exception ex )
{
// ignore as it will never happen for strings
}
return stringWriter.toString();
}
/**
* sort the list
* @param compInfoList list
*/
public static void sort(List compInfoList )
{
try
{
Collections.sort(compInfoList, new Comparator () {
public int compare(Object o1, Object o2) {
return ((JBIComponentInfo)o1).getName().compareTo(
((JBIComponentInfo)o2).getName() );
}
});
}
catch ( ClassCastException ccEx )
{
// log and
// do nothing.
}
catch ( UnsupportedOperationException unsupEx)
{
// log and
// do nothing.
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy