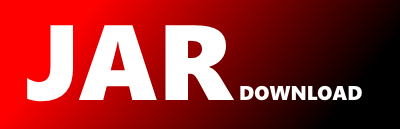
com.sun.jbi.ui.common.JBIJMXObjectNames Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jbi-admin-common Show documentation
Show all versions of jbi-admin-common Show documentation
The "jbi-admin-common" Common CLI code
The newest version!
/*
* BEGIN_HEADER - DO NOT EDIT
*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the "License"). You may not use this file except
* in compliance with the License.
*
* You can obtain a copy of the license at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* HEADER in each file and include the License file at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* If applicable add the following below this CDDL HEADER,
* with the fields enclosed by brackets "[]" replaced with
* your own identifying information: Portions Copyright
* [year] [name of copyright owner]
*/
/*
* @(#)JBIJMXObjectNames.java
* Copyright 2004-2007 Sun Microsystems, Inc. All Rights Reserved.
*
* END_HEADER - DO NOT EDIT
*/
package com.sun.jbi.ui.common;
import javax.management.MalformedObjectNameException;
import javax.management.ObjectName;
/**
* This class provides the JBI MBean ObjectNames
*
* @author Sun Microsystems, Inc.
*/
public final class JBIJMXObjectNames {
/** JMX Domain name for the jbi jmx server */
public static final String JMX_JBI_DOMAIN = "com.sun.jbi";
/** JBI MBean Object type */
public static final String CONTROL_TYPE_KEY = "ControlType";
/** JBI MBean Object type value */
public static final String DEPLOYER_CONTROL_TYPE_VALUE = "Deployer";
/** JBI MBean Object type value */
public static final String INSTALLER_CONTROL_TYPE_VALUE = "Installer";
/** JBI MBean Object type value */
public static final String LIFECYCLE_CONTROL_TYPE_VALUE = "Lifecycle";
/** JBI MBean Object type value */
public static final String CONTROLLER_CONTROL_TYPE_VALUE = "Controller";
/** JBI MBean Object type value */
public static final String ADMIN_SERVICE_CONTROL_TYPE_VALUE = "AdministrationService";
/** JBI MBean Object type */
public static final String COMPONENT_TYPE_KEY = "ComponentType";
/** JBI MBean Object type value */
public static final String SYSTEM_COMPONENT_TYPE_VALUE = "System";
/** JBI MBean Object type value */
public static final String INSTALL_COMPONENT_TYPE_VALUE = "Installed";
/** JBI MBean Object type */
public static final String INSTALLED_TYPE_KEY = "InstalledType";
/** JBI MBean Object type */
public static final String ENGINE_INSTALLED_TYPE_VALUE = "Engine";
/** JBI MBean Object type */
public static final String BINDING_INSTALLED_TYPE_VALUE = "Binding";
/** JBI MBean Object type name */
public static final String COMPONENT_ID_KEY = "ComponentName";
/** JBI Service Names */
/** JBI MBean Object type name */
public static final String SERVICE_NAME_KEY = "ServiceName";
/** Service Names */
public static final String ADMIN_SERVICE = "AdminService";
/** Service Names */
public static final String DEPLOYMENT_SERVICE = "DeploymentService";
/** Service Names */
public static final String INSTALLATION_SERVICE = "InstallationService";
/** Service Names */
public static final String CONFIGURATION_SERVICE = "ConfigurationService";
/** Service Names */
public static final String JBI_ADMIN_UI_SERVICE = "JbiAdminUiService";
/** Service Names */
public static final String JBI_REFERENCE_ADMIN_UI_SERVICE = "JbiReferenceAdminUiService";
/** statistics service */
public static final String JBI_STATISTICS_SERVICE = "JbiStatisticsService";
/** Service Names */
public static final String JAVA_CAPS_MANAGEMENT_ADMINISTRATION_SERVICE = "ESBAdministrationService";
public static final String JAVA_CAPS_MANAGEMENT_CONFIGURATION_SERVICE = "ESBConfigurationService";
public static final String JAVA_CAPS_MANAGEMENT_DEPLOYMENT_SERVICE = "ESBDeploymentService";
public static final String JAVA_CAPS_MANAGEMENT_INSTALLATION_SERVICE = "ESBInstallationService";
public static final String JAVA_CAPS_MANAGEMENT_RUNTIME_MANAGEMENT_SERVICE = "ESBRuntimeManagementService";
public static final String JAVA_CAPS_MANAGEMENT_PERFORMANCE_MEASUREMENT_SERVICE = "ESBPerformanceMeasurementService";
public static final String JAVA_CAPS_MANAGEMENT_NOTIFICATION_SERVICE = "ESBNotificationService";
public static final String TARGET_KEY = "Target";
public static final String SERVICE_TYPE_KEY = "ServiceType";
public static final String COMPONENT_LIFECYCLE_KEY = "ComponentLifeCycle";
/** jbi jmx domain */
private static String sJbiJmxDomain = null;
/** jbi admin object name */
private static ObjectName sAdminMBeanObjectName = null;
/** jbi admin object name */
private static ObjectName sUiMBeanObjectName = null;
/** jbi reference admin object name */
private static ObjectName sReferenceUiMBeanObjectName = null;
/** statistics mbean name */
private static ObjectName sStatisticsMBeanObjectName = null;
/** Java CAPS common management Administration Service object name */
private static ObjectName administrationServiceMBeanObjectName = null;
/** Java CAPS common management Notification Service object name */
private static ObjectName notificationServiceMBeanObjectName = null;
/** Java CAPS common management Configuration Service object name */
private static ObjectName configurationServiceMBeanObjectName = null;
/** Java CAPS common management Deployment Service object name */
private static ObjectName deploymentServiceMBeanObjectName = null;
/** Java CAPS common management Installation Service object name */
private static ObjectName installationServiceMBeanObjectName = null;
/** Java CAPS common management Runtime Management Service object name */
private static ObjectName runtimeManagementServiceMBeanObjectName = null;
/** Java CAPS common management Performance Measurement Service object name */
private static ObjectName performanceMeasurementServiceMBeanObjectName = null;
/**
* gets the jmx domain name
*
* @return domain name
*/
public static String getJbiJmxDomain() {
if (sJbiJmxDomain == null) {
sJbiJmxDomain = System
.getProperty("jbi.jmx.domain", JMX_JBI_DOMAIN);
}
return sJbiJmxDomain;
}
/**
* returns the ObjectName for the installer Mbean.
*
* @return the ObjectName for the installer Mbean.
* @throws MalformedObjectNameException
* if the object name is not formatted according to jmx object
* name
*/
public static ObjectName getJbiAdminUiMBeanObjectName()
throws MalformedObjectNameException {
if (sUiMBeanObjectName == null) {
String mbeanRegisteredName = getJbiJmxDomain() + ":"
+ SERVICE_NAME_KEY + "=" + JBI_ADMIN_UI_SERVICE + ","
+ COMPONENT_TYPE_KEY + "=" + SYSTEM_COMPONENT_TYPE_VALUE;
sUiMBeanObjectName = new ObjectName(mbeanRegisteredName);
}
return sUiMBeanObjectName;
}
/**
* returns the ObjectName for the reference JBI Mbean.
*
* @return the ObjectName for the reference JBI Mbean.
* @throws MalformedObjectNameException
* if the object name is not formatted according to jmx object
* name
*/
public static ObjectName getJbiReferenceAdminUiMBeanObjectName()
throws MalformedObjectNameException {
if (sReferenceUiMBeanObjectName == null) {
String mbeanRegisteredName = getJbiJmxDomain() + ":"
+ SERVICE_NAME_KEY + "=" + JBI_REFERENCE_ADMIN_UI_SERVICE
+ "," + COMPONENT_TYPE_KEY + "="
+ SYSTEM_COMPONENT_TYPE_VALUE;
sReferenceUiMBeanObjectName = new ObjectName(mbeanRegisteredName);
}
return sReferenceUiMBeanObjectName;
}
/**
* returns the ObjectName for the CAPS Management Administration Service
* Mbean.
*
* @return the ObjectName for the CAPS Management Administration Service
* Mbean.
* @throws MalformedObjectNameException
* if the object name is not formatted according to jmx object
* name
*/
public static ObjectName getJavaCapsAdministrationServiceMBeanObjectName()
throws MalformedObjectNameException {
if (administrationServiceMBeanObjectName == null) {
String mbeanRegisteredName = getJbiJmxDomain() + ":"
+ SERVICE_NAME_KEY + "="
+ JAVA_CAPS_MANAGEMENT_ADMINISTRATION_SERVICE + ","
+ COMPONENT_TYPE_KEY + "=" + SYSTEM_COMPONENT_TYPE_VALUE;
administrationServiceMBeanObjectName = new ObjectName(
mbeanRegisteredName);
}
return administrationServiceMBeanObjectName;
}
/**
* returns the ObjectName for the CAPS Management Notification Service
* Mbean.
*
* @return the ObjectName for the CAPS Management Notification Service
* Mbean.
* @throws MalformedObjectNameException
* if the object name is not formatted according to jmx object
* name
*/
public static ObjectName getJavaCapsNotificationServiceMBeanObjectName()
throws MalformedObjectNameException {
if (notificationServiceMBeanObjectName == null) {
String mbeanRegisteredName = getJbiJmxDomain() + ":"
+ SERVICE_NAME_KEY + "="
+ JAVA_CAPS_MANAGEMENT_NOTIFICATION_SERVICE + ","
+ COMPONENT_TYPE_KEY + "=" + SYSTEM_COMPONENT_TYPE_VALUE;
notificationServiceMBeanObjectName = new ObjectName(
mbeanRegisteredName);
}
return notificationServiceMBeanObjectName;
}
/**
* returns the ObjectName for the CAPS Management Configuration Service
* Mbean.
*
* @return the ObjectName for the CAPS Management Configuration Service
* Mbean.
* @throws MalformedObjectNameException
* if the object name is not formatted according to jmx object
* name
*/
public static ObjectName getJavaCapsConfigurationServiceMBeanObjectName()
throws MalformedObjectNameException {
if (configurationServiceMBeanObjectName == null) {
String mbeanRegisteredName = getJbiJmxDomain() + ":"
+ SERVICE_NAME_KEY + "="
+ JAVA_CAPS_MANAGEMENT_CONFIGURATION_SERVICE + ","
+ COMPONENT_TYPE_KEY + "=" + SYSTEM_COMPONENT_TYPE_VALUE;
configurationServiceMBeanObjectName = new ObjectName(
mbeanRegisteredName);
}
return configurationServiceMBeanObjectName;
}
/**
* returns the ObjectName for the CAPS Management Deployment Service Mbean.
*
* @return the ObjectName for the CAPS Management Deployment Service Mbean.
* @throws MalformedObjectNameException
* if the object name is not formatted according to jmx object
* name
*/
public static ObjectName getJavaCapsDeploymentServiceMBeanObjectName()
throws MalformedObjectNameException {
if (deploymentServiceMBeanObjectName == null) {
String mbeanRegisteredName = getJbiJmxDomain() + ":"
+ SERVICE_NAME_KEY + "="
+ JAVA_CAPS_MANAGEMENT_DEPLOYMENT_SERVICE + ","
+ COMPONENT_TYPE_KEY + "=" + SYSTEM_COMPONENT_TYPE_VALUE;
deploymentServiceMBeanObjectName = new ObjectName(
mbeanRegisteredName);
}
return deploymentServiceMBeanObjectName;
}
/**
* returns the ObjectName for the CAPS Management Installation Service
* Mbean.
*
* @return the ObjectName for the CAPS Management Installation Service
* Mbean.
* @throws MalformedObjectNameException
* if the object name is not formatted according to jmx object
* name
*/
public static ObjectName getJavaCapsInstallationServiceMBeanObjectName()
throws MalformedObjectNameException {
if (installationServiceMBeanObjectName == null) {
String mbeanRegisteredName = getJbiJmxDomain() + ":"
+ SERVICE_NAME_KEY + "="
+ JAVA_CAPS_MANAGEMENT_INSTALLATION_SERVICE + ","
+ COMPONENT_TYPE_KEY + "=" + SYSTEM_COMPONENT_TYPE_VALUE;
installationServiceMBeanObjectName = new ObjectName(
mbeanRegisteredName);
}
return installationServiceMBeanObjectName;
}
/**
* returns the ObjectName for the CAPS Runtime Management Service Mbean.
*
* @return the ObjectName for the CAPS Runtime Management Service Mbean.
* @throws MalformedObjectNameException
* if the object name is not formatted according to jmx object
* name
*/
public static ObjectName getJavaCapsRuntimeManagementServiceMBeanObjectName()
throws MalformedObjectNameException {
if (runtimeManagementServiceMBeanObjectName == null) {
String mbeanRegisteredName = getJbiJmxDomain() + ":"
+ SERVICE_NAME_KEY + "="
+ JAVA_CAPS_MANAGEMENT_RUNTIME_MANAGEMENT_SERVICE + ","
+ COMPONENT_TYPE_KEY + "=" + SYSTEM_COMPONENT_TYPE_VALUE;
runtimeManagementServiceMBeanObjectName = new ObjectName(
mbeanRegisteredName);
}
return runtimeManagementServiceMBeanObjectName;
}
/**
* returns the ObjectName for the CAPS Management Performance Measurement
* Service Mbean.
*
* @return the ObjectName for the CAPS Management Performance Measurement
* Service Mbean.
* @throws MalformedObjectNameException
* if the object name is not formatted according to jmx object
* name
*/
public static ObjectName getJavaCapsPerformanceMeasurementServiceMBeanObjectName()
throws MalformedObjectNameException {
if (performanceMeasurementServiceMBeanObjectName == null) {
String mbeanRegisteredName = getJbiJmxDomain() + ":"
+ SERVICE_NAME_KEY + "="
+ JAVA_CAPS_MANAGEMENT_PERFORMANCE_MEASUREMENT_SERVICE
+ "," + COMPONENT_TYPE_KEY + "="
+ SYSTEM_COMPONENT_TYPE_VALUE;
performanceMeasurementServiceMBeanObjectName = new ObjectName(
mbeanRegisteredName);
}
return performanceMeasurementServiceMBeanObjectName;
}
/**
* returns the ObjectName for the deployer Mbean.
*
* @return ObjectName for deployer mbean
* @throws MalformedObjectNameException
* if the object name is not formatted according to jmx object
* name
*/
public static ObjectName getAdminServiceMBeanObjectName()
throws MalformedObjectNameException {
if (sAdminMBeanObjectName == null) {
String mbeanRegisteredName = getJbiJmxDomain() + ":"
+ SERVICE_NAME_KEY + "=" + ADMIN_SERVICE + ","
+ CONTROL_TYPE_KEY + "=" + ADMIN_SERVICE_CONTROL_TYPE_VALUE
+ "," + COMPONENT_TYPE_KEY + "="
+ SYSTEM_COMPONENT_TYPE_VALUE;
sAdminMBeanObjectName = new ObjectName(mbeanRegisteredName);
}
return sAdminMBeanObjectName;
}
/**
* returns the ObjectName Pattern for lifecycle mbeans.
*
* @param componentName
* id of a binding or engine component
* @return the ObjectName Pattern for the lifecycle Mbean.
* @throws MalformedObjectNameException
* if the object name is not formatted according to jmx object
* name
*/
public static ObjectName getComponentLifeCycleMBeanObjectNamePattern(
String componentName) throws MalformedObjectNameException {
// TODO change the COMPONENT_ID_KEY to COMPONENT_NAME
String lifecycleObjectNamePatternString = getJbiJmxDomain() + ":"
+ CONTROL_TYPE_KEY + "=" + LIFECYCLE_CONTROL_TYPE_VALUE + ","
+ COMPONENT_ID_KEY + "=" + componentName + "," + "*";
ObjectName lifecycleObjectNamePattern = new ObjectName(
lifecycleObjectNamePatternString);
return lifecycleObjectNamePattern;
}
/**
* returns the ObjectName Pattern for lifecycle mbeans of the form:
* com.sun.jbi:Target=instance1,ServiceType=ComponentLifecycle,ComponentName=,*
*
* @param componentName
* id of a binding or engine component
* @return the ObjectName Pattern for the lifecycle Mbean.
* @throws MalformedObjectNameException
* if the object name is not formatted according to jmx object
* name
*
*/
public static ObjectName getComponentLifeCycleMBeanObjectNamePattern(
String componentName, String targetName)
throws MalformedObjectNameException {
// TODO change the COMPONENT_ID_KEY to COMPONENT_NAME
String lifecycleObjectNamePatternString = getJbiJmxDomain() + ":"
+ TARGET_KEY + "=" + targetName + "," + SERVICE_TYPE_KEY + "="
+ COMPONENT_LIFECYCLE_KEY + "," + COMPONENT_ID_KEY + "="
+ componentName + "," + "*";
ObjectName lifecycleObjectNamePattern = new ObjectName(
lifecycleObjectNamePatternString);
return lifecycleObjectNamePattern;
}
/**
* returns the ObjectName Pattern for deployer mbeans.
*
* @param componentName
* id of a binding or engine component
* @return the ObjectName Pattern for the deployer Mbean.
* @throws MalformedObjectNameException
* if the object name is not formatted according to jmx object
* name
*/
public static ObjectName getComponentDeployerMBeanObjectNamePattern(
String componentName) throws MalformedObjectNameException {
// TODO change the COMPONENT_ID_KEY to COMPONENT_NAME
String lifecycleObjectNamePatternString = getJbiJmxDomain() + ":"
+ CONTROL_TYPE_KEY + "=" + DEPLOYER_CONTROL_TYPE_VALUE + ","
+ COMPONENT_ID_KEY + "=" + componentName + "," + "*";
ObjectName lifecycleObjectNamePattern = new ObjectName(
lifecycleObjectNamePatternString);
return lifecycleObjectNamePattern;
}
/**
* This method is used to get the ObjectName for the statistics MBean.
* @throws MalformedObjectNameException
* if the object name is not formatted according to jmx object name.
*/
public static ObjectName getJbiStatisticsMBeanObjectName()
throws MalformedObjectNameException
{
if (sStatisticsMBeanObjectName == null)
{
String mbeanRegisteredName =
getJbiJmxDomain() + ":"
+ SERVICE_NAME_KEY + "=" + JBI_STATISTICS_SERVICE
+ "," + COMPONENT_TYPE_KEY + "=" + SYSTEM_COMPONENT_TYPE_VALUE;
sStatisticsMBeanObjectName = new ObjectName(mbeanRegisteredName);
}
return sStatisticsMBeanObjectName;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy