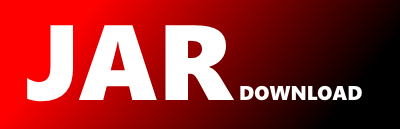
com.sun.jbi.ui.common.JBIResultXmlBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jbi-admin-common Show documentation
Show all versions of jbi-admin-common Show documentation
The "jbi-admin-common" Common CLI code
The newest version!
/*
* BEGIN_HEADER - DO NOT EDIT
*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the "License"). You may not use this file except
* in compliance with the License.
*
* You can obtain a copy of the license at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* HEADER in each file and include the License file at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* If applicable add the following below this CDDL HEADER,
* with the fields enclosed by brackets "[]" replaced with
* your own identifying information: Portions Copyright
* [year] [name of copyright owner]
*/
/*
* @(#)JBIResultXmlBuilder.java
* Copyright 2004-2007 Sun Microsystems, Inc. All Rights Reserved.
*
* END_HEADER - DO NOT EDIT
*/
package com.sun.jbi.ui.common;
import java.io.PrintWriter;
import java.io.StringWriter;
import java.util.ArrayList;
import java.util.List;
/**
* This class is used to create a JBI Management message that contains the
* messages and exception under the framework tags to help the ui messages
* formatted in the standard message format as the any other result messages
* comming from the jbi runtime in the form of jbi manamgment message xml.
*
* @author Sun Microsystems, Inc.
*/
public class JBIResultXmlBuilder {
/**
* success result
*/
public final static boolean SUCCESS_RESULT = true;
/**
* failed result
*/
public final static boolean FAILED_RESULT = false;
/**
* info message type
*/
public final static String INFO_MSG_TYPE = "INFO";
/**
* warning message type
*/
public final static String WARNING_MSG_TYPE = "WARNING";
/**
* error message type
*/
public final static String ERROR_MSG_TYPE = "ERROR";
/**
* default task id
*/
public final static String DEFAULT_TASK_ID = "UI_COMMON_TASK";
/**
* default message code
*/
public final static String DEFAULT_MSG_CODE = "UICMN0000";
/**
* jbi mgmt xml prefix
*/
private final static String JBI_MGMT_XML_BEGIN = ""
+ " "
+ ""
+ ""
+ "" + "";
/**
* jbi mgmt xml end
*/
private final static String JBI_MGMT_XML_END = " "
+ " " + " "
+ "YES "
+ " " + " " + " ";
/**
* static instance of this class
*/
private static JBIResultXmlBuilder sJbiResultXmlBuilder = null;
/** Creates a new instance of EsbResultXmlBuilder */
protected JBIResultXmlBuilder() {
}
/**
* returns the instance of this class
*
* @return instance of this class
*/
public static JBIResultXmlBuilder getInstance() {
if (sJbiResultXmlBuilder == null) {
sJbiResultXmlBuilder = new JBIResultXmlBuilder();
}
return sJbiResultXmlBuilder;
}
/**
* returns the exception cause chain in a list including this exception
*
* @param ex
* exception
* @return list contain the exception chain
*/
protected List /* */flatExceptionChain(Throwable ex) {
List list = new ArrayList();
list.add(ex);
for (Throwable cause = ex.getCause(); cause != null; cause = cause
.getCause()) {
list.add(cause);
}
return list;
}
/**
* return the xml chunk that has task info elements
*
* @param taskId
* task id
* @param successResult
* true for SUCCESS, false for FAILED
* @param msgType
* one of INFO, ERROR, WARNING strings
* @return the xml chunk
*/
protected String getTaskInfoXml(String taskId, boolean successResult,
String msgType) {
String taskResult = (successResult) ? "SUCCESS" : "FAILED";
return "" + taskId + " " + ""
+ taskResult + " " + "" + msgType
+ " ";
}
/**
* return the xml chunk contains the message info elements
*
* @param msgCode
* i18n message code
* @param msg
* l10n message
* @param args
* arguments for i18n message
* @return the xml chunk
*/
protected String getMsgLocInfoXml(String msgCode, String msg, Object[] args) {
StringBuffer buff = new StringBuffer();
buff.append("" + "" + msgCode + " "
+ ""
+ DOMUtil.replaceXmlEscapeCharsToEntityRefereces(msg)
+ " ");
if (args != null) {
for (int i = 0; i < args.length; ++i) {
buff.append("" + args[i] + " ");
}
}
buff.append(" ");
return buff.toString();
}
/**
* return the xml chuck for status message elements
*
* @param msgCode
* i18n message code
* @param msg
* l10n message
* @param args
* arguments for i18n message
* @return the xml chunk
*/
protected String getTaskStatusMsgXml(String msgCode, String msg,
Object[] args) {
return "" + getMsgLocInfoXml(msgCode, msg, args)
+ " ";
}
/**
* return the excpetion stacktrace as a string
*
* @param ex
* exception
* @return string contains the exception stacktrace
*/
protected String getExceptionStackTrace(Throwable ex) {
StringWriter buff = new StringWriter();
PrintWriter out = new PrintWriter(buff);
String stackTrace = "";
StackTraceElement[] stEl = ex.getStackTrace();
if (stEl == null || stEl.length == 0) {
return stackTrace;
}
for (int i = 0; i < stEl.length; ++i) {
out.println(stEl[i].toString());
}
out.close();
stackTrace = buff.getBuffer().toString();
return stackTrace;
}
/**
* returns exceptin info xml chunk
*
* @param msgCode
* i18n message code
* @param nestingLevel
* level in the exception chain
* @param ex
* exception for stacktrace
* @return xml chunk
*/
protected String getExceptionInfoXml(String msgCode, int nestingLevel,
Throwable ex) {
String stackTrace = getExceptionStackTrace(ex);
return "" + "" + nestingLevel
+ " "
+ getMsgLocInfoXml(msgCode, ex.getMessage(), null)
+ ""
+ DOMUtil.replaceXmlEscapeCharsToEntityRefereces(stackTrace)
+ " " + " ";
}
/**
* returns exceptin info xml chunk
*
* @param msgCode
* i18n message code
* @param locMsg
* localized message
* @param nestingLevel
* level in the exception chain
* @param stackTrace
* stacktrace string
* @return xml chunk
*/
protected String getExceptionInfoXml(String msgCode, String locMsg, int nestingLevel,
String stackTrace) {
return "" + "" + nestingLevel
+ " "
+ getMsgLocInfoXml(msgCode, locMsg, null)
+ ""
+ stackTrace
+ " " + " ";
}
/**
* returns the xml chunk contains exception message info for the exception
* chain
*
* @param msgCode
* i18n code
* @param ex
* exception
* @return xml chunk
*/
protected String getChainedExceptionInfoXml(String msgCode, Throwable ex) {
StringBuffer buff = new StringBuffer();
if (msgCode == null) {
msgCode = DEFAULT_MSG_CODE; // UNKNOWN MSG CODE
}
List list = this.flatExceptionChain(ex);
int listSize = list.size();
for (int i = 0; i < listSize; ++i) {
buff.append(getExceptionInfoXml(msgCode, i + 1, (Throwable) list
.get(i)));
}
return buff.toString();
}
/**
* creates the jbi management xml
*
* @param taskId
* task id
* @param successResult
* true for SUCCESS, false for FAILIED message
* @param msgType
* one of INFO, ERROR, WARNING
* @param msgCode
* i18n message code
* @param msg
* message
* @param args
* i18n message arguments
* @param ex
* exception
* @return jbi mgmt xml
*/
public String createJbiResultXml(String taskId, boolean successResult,
String msgType, String msgCode, String msg, Object[] args,
Throwable ex) {
StringBuffer buff = new StringBuffer();
buff.append(JBI_MGMT_XML_BEGIN);
buff.append(getTaskInfoXml(taskId, successResult, msgType));
buff.append(getTaskStatusMsgXml(msgCode, msg, args));
if (ex != null) {
String expMsgCode = DEFAULT_MSG_CODE;
if (msg == null) {
// if msg is null, assume msgCode passed is for expception
expMsgCode = msgCode;
}
buff.append(getChainedExceptionInfoXml(expMsgCode, ex));
}
buff.append(JBI_MGMT_XML_END);
return buff.toString();
}
/**
* creates the jbi management xml
*
* @param taskId
* task id
* @param successResult
* true for SUCCESS, false for FAILIED message
* @param msgType
* one of INFO, ERROR, WARNING
* @param msgCode
* i18n message code
* @param msg
* message
* @param args
* i18n message arguments
* @return jbi mgmt xml
*/
public String createJbiResultXml(String taskId, boolean successResult,
String msgType, String msgCode, String msg, Object[] args) {
return createJbiResultXml(taskId, successResult, msgType, msgCode, msg,
args, null);
}
/**
* creates the jbi management xml
*
* @param taskId
* task id
* @param msgCode
* i18n message code
* @param ex
* exception
* @return jbi mgmt xml
*/
public String createJbiResultXml(String taskId, String msgCode, Throwable ex) {
return createJbiResultXml(taskId, false, "ERROR", msgCode, null, null,
ex);
}
/**
* creates the jbi management xml
*
* @param i18nBundle
* i18n bundle object
* @param i18nKey
* key to look for i18n msg
* @param args
* i18n args
* @param taskId
* task id
* @param successResult
* true or false
* @param msgType
* message type
* @param ex
* exception
* @return the jbi management xml
*/
public static String createJbiResultXml(I18NBundle i18nBundle,
String i18nKey, Object[] args, String taskId,
boolean successResult, String msgType, Throwable ex) {
String msgCode = i18nBundle.getMessage(i18nKey + ".ID");
String msg = i18nBundle.getMessage(i18nKey, args);
String jbiResultXml = JBIResultXmlBuilder.getInstance()
.createJbiResultXml(taskId, successResult, msgType, msgCode,
msg, args, ex);
if (jbiResultXml == null) {
return msg;
} else {
return jbiResultXml;
}
}
/**
* creates the jbi management xml
*
* @param i18nBundle
* i18n bundle object
* @param i18nKey
* i18n key in the bundle
* @param args
* i18n args
* @param ex
* exception
* @return jbi mgmt xml
*/
public static String createJbiResultXml(I18NBundle i18nBundle,
String i18nKey, Object[] args, Throwable ex) {
return createJbiResultXml(i18nBundle, i18nKey, args,
"JBI_UI_COMMON_TASKS", JBIResultXmlBuilder.FAILED_RESULT,
JBIResultXmlBuilder.ERROR_MSG_TYPE, ex);
}
/**
* Creates a XML JBI Management message from the JBI Management Message
*
* @return the jbi management xml
* @param msg - JBIManagementMessage instance to create the xml message from
*/
public String createJbiResultXml(JBIManagementMessage msg)
{
String resultXml = null;
if ( msg != null )
{
JBIManagementMessage.FrameworkTaskResult fwkRslt = msg.getFrameworkTaskResult();
JBIManagementMessage.TaskResultInfo tskRsltInfo = fwkRslt.getTaskResultInfo();
// Header
StringBuffer jbiXmlBuf = new StringBuffer(JBI_MGMT_XML_BEGIN);
// TaskResult
jbiXmlBuf.append(getTaskInfoXml(tskRsltInfo.getTaskId(), msg.isSuccessMsg(),
tskRsltInfo.getMessageType()));
// TaskStatusMsgs
List msgInfos = tskRsltInfo.getStatusMessageInfoList();
for ( JBIManagementMessage.MessageInfo msgInfo : msgInfos )
{
Object[] params = new Object[msgInfo.getl10nMsgParams().size()];
params = msgInfo.getl10nMsgParams().toArray(params);
jbiXmlBuf.append(getTaskStatusMsgXml(msgInfo.getI18nId(),
msgInfo.getLocalizedMsg(), params));
}
// ExceptionInfos
List exInfos = tskRsltInfo.getExceptionInfoList();
for ( JBIManagementMessage.ExceptionInfo exInfo : exInfos )
{
jbiXmlBuf.append(getExceptionInfoXml(
exInfo.getMessageInfo().getI18nId(),
exInfo.getMessageInfo().getLocalizedMsg(),
exInfo.getLevel(),
exInfo.getFormattedStackTrace()));
}
// Trailer
jbiXmlBuf.append(JBI_MGMT_XML_END);
resultXml = jbiXmlBuf.toString();
}
return resultXml;
}
/**
* creates the jbi management xml
*
* @param i18nBundle
* i18n bundle object
* @param i18nKey
* i18n key in the bundle
* @param args
* i18n args
* @return jbi mgmt xml
*/
public static String createFailedJbiResultXml(I18NBundle i18nBundle,
String i18nKey, Object[] args) {
return createJbiResultXml(i18nBundle, i18nKey, args,
"JBI_UI_COMMON_TASKS", JBIResultXmlBuilder.FAILED_RESULT,
JBIResultXmlBuilder.ERROR_MSG_TYPE, null);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy