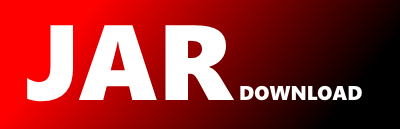
com.sun.jbi.ui.common.ServiceAssemblyDD Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jbi-admin-common Show documentation
Show all versions of jbi-admin-common Show documentation
The "jbi-admin-common" Common CLI code
The newest version!
/*
* BEGIN_HEADER - DO NOT EDIT
*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the "License"). You may not use this file except
* in compliance with the License.
*
* You can obtain a copy of the license at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* HEADER in each file and include the License file at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* If applicable add the following below this CDDL HEADER,
* with the fields enclosed by brackets "[]" replaced with
* your own identifying information: Portions Copyright
* [year] [name of copyright owner]
*/
/*
* @(#)ServiceAssemblyDD.java
* Copyright 2004-2007 Sun Microsystems, Inc. All Rights Reserved.
*
* END_HEADER - DO NOT EDIT
*/
package com.sun.jbi.ui.common;
import java.io.PrintStream;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import org.w3c.dom.Element;
import org.w3c.dom.NodeList;
/**
* This class is reads the jbi.xml as a service assembly descriptor.
*
* @author Sun Microsystems, Inc.
*/
public class ServiceAssemblyDD extends JBIDescriptor
{
/**
* id info
*/
Identification mIdInfo;
/**
* list of su dds
*/
private List mServiceUnitDDList;
/**
* Creates a new instance of JBIDescriptor
* @param idInfo id info.
* @param suDDList list.
*/
protected ServiceAssemblyDD(Identification idInfo, List suDDList)
{
this.mIdInfo = idInfo;
if ( suDDList != null )
{
this.mServiceUnitDDList = Collections.unmodifiableList(suDDList);
}
else
{
this.mServiceUnitDDList = new ArrayList();
}
}
/**
* attribute
* @return value
*/
public String getName()
{
return this.mIdInfo.getName();
}
/**
* attribute
* @return value
*/
public String getDescription()
{
return this.mIdInfo.getDescription();
}
/**
* returns su list
* @return list
*/
public List getServiceUnitDDList()
{
return this.mServiceUnitDDList;
}
/**
* check for shared library descriptor.
* @return true if it is shared library desciptor else false.
*/
public boolean isSharedLibraryDescriptor()
{
return false;
}
/**
* check for ServiceEngine descriptor.
* @return true if it is ServiceEngine desciptor else false.
*/
public boolean isServiceEngineDescriptor()
{
return false;
}
/**
* check for BindingComponen descriptor.
* @return true if it is BindingComponen desciptor else false.
*/
public boolean isBindingComponentDescriptor()
{
return false;
}
/**
* check for service assembly descriptor.
* @return true if it is service assembly desciptor else false.
*/
public boolean isServiceAssemblyDescriptor()
{
return true;
}
/**
* object as string
* @return string
*/
public String toString()
{
// if ( this.mIdInfo == null )
// {
// return "Identification object is null in ServiceAssemblyDD";
// }
// if( this.mServiceUnitDDList == null )
// {
// return "ServiceUnitDDList is null in ServiceAssemblyDD";
// }
return
"Name : " + getName() + "\n" +
"Description : " + getDescription() + "\n" +
"Service Units : " + getServiceUnitDDList().size();
}
/**
* object as string
* @param out print stream.
*/
public void printServiceAssembly(PrintStream out)
{
out.println(this);
List list = this.getServiceUnitDDList();
for ( Iterator itr = list.iterator(); itr.hasNext();)
{
out.println(itr.next());
}
}
/**
* create sa dd.
* @param idInfo id info.
* @param suDDList list.
* @return sa dd .
*/
public static ServiceAssemblyDD createServiceAssemblyDD(Identification idInfo, List suDDList)
{
return new ServiceAssemblyDD(idInfo, suDDList);
}
/**
* creates sa dd.
* @param saEl xml element.
* @return sa dd.
*/
public static ServiceAssemblyDD createServiceAssemblyDD(Element saEl)
{
Identification idInfo = null;
List suDDList = null;
Element idInfoEl =
DOMUtil.UTIL.getChildElement(saEl, "identification");
if ( idInfoEl != null )
{
idInfo = Identification.createIdentification(idInfoEl);
}
suDDList = new ArrayList();
NodeList suNodeList =
DOMUtil.UTIL.getChildElements(saEl, "service-unit");
int size = suNodeList.getLength();
for ( int i = 0; i < size; ++i )
{
Element suEl = (Element) suNodeList.item(i);
if ( suEl != null )
{
ServiceUnitDD suDD = ServiceUnitDD.createServiceUnitDD(suEl);
suDDList.add(suDD);
}
}
return createServiceAssemblyDD(idInfo, suDDList);
}
/**
* Service Unit Deployment Descriptor model
*/
public static class ServiceUnitDD
{
/**
* id info
*/
Identification mIdInfo;
/**
* target name
*/
private String mTargetName;
/**
* SU Zip name
*/
private String mZipName;
/**
* constructor
* @param idInfo id info.
* @param targetName target name.
*/
protected ServiceUnitDD(Identification idInfo, String targetName, String suZipName)
{
this.mIdInfo = idInfo;
this.mTargetName = targetName;
this.mZipName = suZipName;
}
/**
* attribute
* @return value
*/
public String getName()
{
return this.mIdInfo.getName();
}
/**
* attribute
* @return value
*/
public String getDescription()
{
return this.mIdInfo.getDescription();
}
/**
* attribute
* @return value
*/
public String getTargetName()
{
return this.mTargetName;
}
/**
* Getter for the SU zip name
* @return String the zip name for the SU
*/
public String getArtifactZipName()
{
return this.mZipName;
}
/**
* string value of the object
* @return value
*/
public String toString()
{
return
"Name : " + getName() + "\n" +
"Description : " + getDescription() + "\n" +
"TargetName : " + getTargetName();
}
/**
* create su dd.
* @param idInfo id info.
* @param targetName target name.
* @return su dd.
*/
public static ServiceUnitDD createServiceUnitDD(Identification idInfo, String targetName, String suZipName)
{
return new ServiceUnitDD(idInfo, targetName, suZipName);
}
/**
* creates su dd
* @param suEl xml element.
* @return su dd.
*/
public static ServiceUnitDD createServiceUnitDD(Element suEl)
{
Identification idInfo = null;
String targetName = null;
String suZipName = null;
Element idInfoEl =
DOMUtil.UTIL.getElement(suEl, "identification");
if ( idInfoEl != null )
{
idInfo = Identification.createIdentification(idInfoEl);
}
Element targetNameEl =
DOMUtil.UTIL.getElement(suEl, "component-name");
if ( targetNameEl != null )
{
targetName = DOMUtil.UTIL.getTextData(targetNameEl);
}
Element zipEl =
DOMUtil.UTIL.getElement(suEl, "artifacts-zip");
if ( zipEl != null )
{
suZipName = DOMUtil.UTIL.getTextData(zipEl);
}
return createServiceUnitDD(idInfo, targetName, suZipName);
}
}
/**
* Identification model
*/
public static class Identification
{
/**
* name
*/
private String mName;
/**
* descritpion
*/
private String mDesc;
/**
* constructor
* @param name name
* @param desc description
*/
protected Identification(String name, String desc)
{
this.mName = name;
this.mDesc = desc;
}
/**
* attribute
* @return value
*/
public String getName()
{
return this.mName;
}
/**
* attribute
* @return value
*/
public String getDescription()
{
return this.mDesc;
}
/**
* string value of the object
* @return value
*/
public String toString()
{
return
"Name : " + getName() + "\n" +
"Description : " + getDescription();
}
/**
* creates identification object.
* @param name name.
* @param desc descritpion.
* @return id object.
*/
public static Identification createIdentification(String name, String desc)
{
return new Identification(name,desc);
}
/**
* creates id object
* @param idInfoEl xml element
* @return id object
*/
public static Identification createIdentification(Element idInfoEl)
{
String name = null;
String desc = null;
Element nameEl =
DOMUtil.UTIL.getElement(idInfoEl, "name");
if ( nameEl != null )
{
name = DOMUtil.UTIL.getTextData(nameEl);
}
Element descEl =
DOMUtil.UTIL.getElement(idInfoEl, "description");
if ( descEl != null )
{
desc = DOMUtil.UTIL.getTextData(descEl);
}
return createIdentification(name,desc);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy