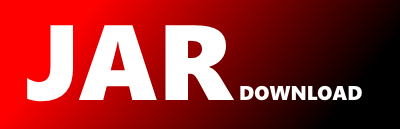
com.sun.jbi.ui.common.ServiceAssemblyInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jbi-admin-common Show documentation
Show all versions of jbi-admin-common Show documentation
The "jbi-admin-common" Common CLI code
The newest version!
/*
* BEGIN_HEADER - DO NOT EDIT
*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the "License"). You may not use this file except
* in compliance with the License.
*
* You can obtain a copy of the license at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* HEADER in each file and include the License file at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* If applicable add the following below this CDDL HEADER,
* with the fields enclosed by brackets "[]" replaced with
* your own identifying information: Portions Copyright
* [year] [name of copyright owner]
*/
/*
* @(#)ServiceAssemblyInfo.java
* Copyright 2004-2007 Sun Microsystems, Inc. All Rights Reserved.
*
* END_HEADER - DO NOT EDIT
*/
package com.sun.jbi.ui.common;
import com.sun.jbi.ui.common.ServiceAssemblyDD.ServiceUnitDD;
import java.io.PrintWriter;
import java.io.Reader;
import java.io.StringReader;
import java.io.StringWriter;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.Iterator;
import java.util.List;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.NodeList;
/** This class reads/writes the service assembly info list xml.
* @author Sun Microsystems, Inc.
*/
public class ServiceAssemblyInfo
{
/** namespace */
public static final String XMLNS = "http://java.sun.com/xml/ns/jbi/service-assembly-info-list";
/**
* state
*/
public static final String STARTED_STATE = "Started";
/**
* state
*/
public static final String STOPPED_STATE = "Stopped";
/**
* state
*/
public static final String SHUTDOWN_STATE = "Shutdown";
/**
* state
*/
public static final String UNKNOWN_STATE = "Unknown";
/**
* state
*/
public static final String ANY_STATE = "any";
/**
* state
*/
public static final String ANY_FW_STATE = "any";
/**
* Holds value of property name.
*/
private String mName;
/**
* Holds value of property name.
*/
private List mSUInfoList;
/**
* Holds value of property description.
*/
private String mDescription;
/**
* Holds value of property state.
*/
private String mState;
/** Creates a new instance of JBIComponentInfo */
public ServiceAssemblyInfo()
{
this("","", UNKNOWN_STATE);
}
/**
* Creates a new instance of JBIComponentInfo
* @param state state
* @param name name
* @param description description
*/
public ServiceAssemblyInfo(String name, String description, String state)
{
this.mName = name;
this.mDescription = description;
this.mState = state;
this.mSUInfoList = new ArrayList();
}
/**
* Getter for property aliasName.
* @return Value of property aliasName.
*/
public String getName()
{
return this.mName;
}
/**
* Setter for property aliasName.
* @param name New value of property aliasName.
*/
public void setName(String name)
{
this.mName = name;
}
/**
* Getter for property description.
* @return Value of property description.
*/
public String getDescription()
{
return this.mDescription;
}
/**
* Setter for property description.
* @param description New value of property description.
*/
public void setDescription(String description)
{
this.mDescription = description;
}
/**
* Getter for property state.
* @return Value of property state.
*/
public String getState()
{
return this.mState;
}
/**
* Setter for property state.
* @param state state
*/
public void setState(String state)
{
this.mState = state;
}
/**
* Getter for property description.
* @return Value of property description.
*/
public List getServiceUnitInfoList()
{
return this.mSUInfoList;
}
/** Return the localized state value
* @return localized state value
*/
public String getLocalizedState()
{
String localizedState = Util.getCommonI18NBundle().getMessage(this.getState());
return localizedState;
}
/** string value
* @return string value
*/
public String toString()
{
return
"Name = " +
this.getName() +
"\nDescription = " +
this.getDescription() +
"\nState = " +
this.getState() +
"\nService Units = " +
this.getServiceUnitInfoList().size();
}
/** xml text
* @return xml text
*/
public String toXmlString()
{
List suInfoList = getServiceUnitInfoList();
String suInfoText = ServiceUnitInfo.writeAsXmlText(suInfoList);
return
"\n" +
"" + this.getDescription() + " \n" +
suInfoText + "\n" +
" ";
}
/**
* return component info object
* @param saInfoEl xml element
* @return ServiceAssemblyInfo.
*/
public static ServiceAssemblyInfo createServiceAssemblyInfo(
Element saInfoEl )
{
ServiceAssemblyInfo info = new ServiceAssemblyInfo();
String name = saInfoEl.getAttribute("name");
String state = saInfoEl.getAttribute("state");
info.setName(name);
info.setState(state);
String desc = null;
Element descEl = DOMUtil.UTIL.getChildElement(saInfoEl, "description");
if ( descEl != null )
{
desc = DOMUtil.UTIL.getTextData(descEl);
}
info.setDescription(desc);
// construct the su info list
NodeList suInfoElList =
DOMUtil.UTIL.getChildElements(saInfoEl, "service-unit-info");
List list = ServiceUnitInfo.createServiceUnitInfoList(suInfoElList);
info.getServiceUnitInfoList().addAll(list);
return info;
}
/**
* creates list of objects
* @return list of objects
* @param saInfoListEl xml element.
*/
public static List createServiceAssemblyInfoList(Element saInfoListEl)
{
ArrayList saInfoList = new ArrayList();
// construct the compInfo list
if ( saInfoListEl == null )
{
// System.out.println("No Root Element ");
return saInfoList; // return empty list
}
String version = saInfoListEl.getAttribute("version");
NodeList saInfoNodeList =
DOMUtil.UTIL.getChildElements(saInfoListEl, "service-assembly-info");
int size = saInfoNodeList.getLength();
// System.out.println("compInfo found in XML Document : " + size);
for ( int i = 0; i < size; ++i )
{
Element saInfoEl = (Element) saInfoNodeList.item(i);
if ( saInfoEl != null )
{
ServiceAssemblyInfo saInfo =
createServiceAssemblyInfo(saInfoEl);
saInfoList.add(saInfo);
}
}
ServiceAssemblyInfo.sort(saInfoList);
return saInfoList;
}
/** creates list of objects
* @param xmlReader xml text reader
* @return list of objects
*/
public static List readFromXmlTextWithProlog(Reader xmlReader)
{
ArrayList saInfoList = new ArrayList();
Document xmlDoc = null;
try
{
xmlDoc = DOMUtil.UTIL.buildDOMDocument(xmlReader);
}
catch (Exception ex)
{
Util.logDebug(ex);
}
if ( xmlDoc == null )
{
return saInfoList; // return empty list
}
// construct the compInfo list
Element saInfoListEl =
DOMUtil.UTIL.getElement(xmlDoc, "service-assembly-info-list");
if ( saInfoListEl == null )
{
// System.out.println("No Root Element ");
return saInfoList; // return empty list
}
return createServiceAssemblyInfoList(saInfoListEl);
}
/** creates list of objects
* @param xmlText xml text
* @return list of objects
*/
public static List readFromXmlTextWithProlog(String xmlText)
{
return readFromXmlTextWithProlog(new StringReader(xmlText));
}
/**
* write to xml text
* @return xml text
* @param saInfoList list.
*/
public static String writeAsXmlText(List saInfoList)
{
ServiceAssemblyInfo.sort(saInfoList);
StringWriter stringWriter = new StringWriter();
PrintWriter writer = new PrintWriter(stringWriter, true);
// begine xml
writer.println(
"");
int size = saInfoList.size();
for (int i=0; i < size; ++i )
{
ServiceAssemblyInfo info = (ServiceAssemblyInfo) saInfoList.get(i);
writer.println(info.toXmlString());
}
// end xml
writer.println(" ");
try
{
writer.close();
stringWriter.close();
}
catch ( Exception ex )
{
// ignore as it will never happen for strings
}
return stringWriter.toString();
}
/**
* write to xml text
* @return xml text
* @param saInfoList list.
*/
public static String writeAsXmlTextWithProlog(List saInfoList)
{
StringBuffer buffer = new StringBuffer();
// begine xml
buffer.append("");
buffer.append("\n");
buffer.append(writeAsXmlText(saInfoList));
buffer.append("\n");
// end xml
return buffer.toString();
}
/**
* creates sa info
* @return ServiceAssemblyINfo
* @param saDD sa descriptor.
*/
public static ServiceAssemblyInfo createFromServiceAssemblyDD(
ServiceAssemblyDD saDD )
{
ServiceAssemblyInfo saInfo = new ServiceAssemblyInfo();
saInfo.setName(saDD.getName());
saInfo.setDescription(saDD.getDescription());
// init su info.
List suDDList = saDD.getServiceUnitDDList();
List suInfoList = new ArrayList();
for ( Iterator itr = suDDList.iterator(); itr.hasNext(); )
{
ServiceUnitInfo suInfo =
ServiceUnitInfo.createFromServiceUnitDD(
(ServiceUnitDD)itr.next());
suInfoList.add(suInfo);
}
saInfo.getServiceUnitInfoList().addAll(suInfoList);
return saInfo;
}
/**
* create sa info
* @param jbiXmlReader reader
* @return sa info object
*/
public static ServiceAssemblyInfo createFromServiceAssemblyDD(Reader jbiXmlReader )
{
try
{
ServiceAssemblyDD dd =
(ServiceAssemblyDD) ServiceAssemblyDD.createJBIDescriptor(jbiXmlReader);
if ( dd != null )
{
return createFromServiceAssemblyDD(dd);
}
}
catch (Exception ex )
{
Util.logDebug(ex);
}
return null;
}
/**
* sorts the list.
* @param saInfoList list.
*/
public static void sort(List saInfoList )
{
try
{
Collections.sort(saInfoList, new Comparator () {
public int compare(Object o1, Object o2) {
return ((ServiceAssemblyInfo)o1).getName().compareTo(
((ServiceAssemblyInfo)o2).getName() );
}
});
}
catch ( ClassCastException ccEx )
{
// log and
// do nothing.
}
catch ( UnsupportedOperationException unsupEx)
{
// log and
// do nothing.
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy