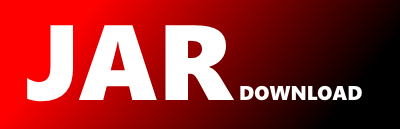
com.sun.jbi.ui.common.ServiceUnitInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jbi-admin-common Show documentation
Show all versions of jbi-admin-common Show documentation
The "jbi-admin-common" Common CLI code
The newest version!
/*
* BEGIN_HEADER - DO NOT EDIT
*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the "License"). You may not use this file except
* in compliance with the License.
*
* You can obtain a copy of the license at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* HEADER in each file and include the License file at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* If applicable add the following below this CDDL HEADER,
* with the fields enclosed by brackets "[]" replaced with
* your own identifying information: Portions Copyright
* [year] [name of copyright owner]
*/
/*
* @(#)ServiceUnitInfo.java
* Copyright 2004-2007 Sun Microsystems, Inc. All Rights Reserved.
*
* END_HEADER - DO NOT EDIT
*/
package com.sun.jbi.ui.common;
import com.sun.jbi.ui.common.ServiceAssemblyDD.ServiceUnitDD;
import java.io.PrintWriter;
import java.io.StringWriter;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.List;
import org.w3c.dom.Element;
import org.w3c.dom.NodeList;
/** This class represents the ServiceUnitInfo in the service assembly info list xml
*
* @author Sun Microsystems, Inc.
*/
public class ServiceUnitInfo
{
/** status Unknown. */
public static final String UNKNOWN_STATE = "Unknown";
/** status Deployed. */
public static final String SHUTDOWN_STATE = "Shutdown";
/** status Deployed. */
public static final String STARTED_STATE = "Started";
/** status Deployed. */
public static final String STOPPED_STATE = "Stopped";
/**
* Holds value of property name.
*/
private String mName;
/**
* Holds value of property description.
*/
private String mDescription;
/**
* Holds value of property targetId.
*/
private String mDeployedOn;
/**
* Holds value of property state.
*/
private String mState;
/** Creates a new instance of JBIComponentInfo */
public ServiceUnitInfo()
{
this("","","", UNKNOWN_STATE );
}
/**
* Creates a new instance of SunAssemblyInfo
* @param deployedOn deployedOn.
* @param state state.
* @param name name
* @param description description
*/
public ServiceUnitInfo(String name, String description,
String deployedOn, String state )
{
this.mName = name;
this.mDescription = description;
this.mDeployedOn = deployedOn;
this.mState = state;
}
/**
* Getter for property aliasName.
* @return Value of property aliasName.
*/
public String getName()
{
return this.mName;
}
/**
* Setter for property aliasName.
* @param name New value of property aliasName.
*/
public void setName(String name)
{
this.mName = name;
}
/**
* Getter for property description.
* @return Value of property description.
*/
public String getDescription()
{
return this.mDescription;
}
/**
* Setter for property description.
* @param description New value of property description.
*/
public void setDescription(String description)
{
this.mDescription = description;
}
/**
* Getter for property targetId.
* @return Value of property targetId.
*/
public String getTargetName()
{
return this.getDeployedOn();
}
/**
* Getter for property targetId.
* @return Value of property targetId.
*/
public String getDeployedOn()
{
return this.mDeployedOn;
}
/**
* Setter for property targetId.
* @param deployedOn deployedOn.
*/
public void setDeployedOn(String deployedOn)
{
this.mDeployedOn = deployedOn;
}
/**
* Getter for property state.
* @return Value of property state.
*/
public String getState()
{
return this.mState;
}
/**
* Setter for property status.
* @param state status
*/
public void setState(String state)
{
this.mState = state;
}
/** Return the localized state value
* @return localized state value
*/
public String getLocalizedState()
{
String localizedState = Util.getCommonI18NBundle().getMessage(this.getState());
return localizedState;
}
/** string value
* @return string value
*/
public String toString()
{
return
"Name = " +
this.getName() +
"\nDescription = " +
this.getDescription() +
"\nState = " +
this.getState() +
"\nDeployed On = " +
this.getDeployedOn();
}
/** xml text
* @return xml text
*/
public String toXmlString()
{
return
"\n" +
"" + this.getDescription() + " \n" +
" ";
}
/**
* return sub assembly info object
* @return object
* @param suInfoEl xml element.
*/
public static ServiceUnitInfo createServiceUnitInfo(
Element suInfoEl )
{
ServiceUnitInfo info = new ServiceUnitInfo();
if ( suInfoEl == null )
{
return null;
}
String name = suInfoEl.getAttribute("name");
info.setName(name);
String state = suInfoEl.getAttribute("state");
info.setState(state);
String deployedOn = suInfoEl.getAttribute("deployed-on");
info.setDeployedOn(deployedOn);
String desc = null;
Element descEl = DOMUtil.UTIL.getElement(suInfoEl, "description");
if ( descEl != null )
{
desc = DOMUtil.UTIL.getTextData(descEl);
}
info.setDescription(desc);
return info;
}
/**
* return component info object
* @return object
* @param suInfoElList xml element.
*/
public static List createServiceUnitInfoList(
NodeList suInfoElList )
{
ArrayList suInfoList = new ArrayList();
if ( suInfoElList == null )
{
return suInfoList; // return empty list
}
int size = suInfoElList.getLength();
for ( int i = 0; i < size; ++i )
{
Element suInfoEl = (Element) suInfoElList.item(i);
if ( suInfoEl != null )
{
ServiceUnitInfo suInfo = createServiceUnitInfo(suInfoEl);
suInfoList.add(suInfo);
}
}
ServiceUnitInfo.sort(suInfoList);
return suInfoList;
}
/**
* write to xml text
* @return xml text
* @param suInfoList xml element.
*/
public static String writeAsXmlText(List suInfoList)
{
StringWriter stringWriter = new StringWriter();
PrintWriter writer = new PrintWriter(stringWriter,true);
if ( suInfoList != null )
{
int size = suInfoList.size();
for (int i=0; i < size; ++i )
{
ServiceUnitInfo info = (ServiceUnitInfo) suInfoList.get(i);
writer.println(info.toXmlString());
}
}
try
{
writer.close();
stringWriter.close();
}
catch ( Exception ex )
{
// ignore as it will never happen for strings unless runtime exp as mem exp
}
return stringWriter.toString();
}
/**
* creates the info from jbi.xml
* @return info object
* @param suDD su dd.
*/
public static ServiceUnitInfo createFromServiceUnitDD(ServiceUnitDD suDD)
{
ServiceUnitInfo suInfo = new ServiceUnitInfo();
suInfo.setName(suDD.getName());
suInfo.setDescription(suDD.getDescription());
suInfo.setDeployedOn(suDD.getTargetName());
return suInfo;
}
/**
* sorts list.
* @param suInfoList su info list.
*/
public static void sort(List suInfoList )
{
try
{
Collections.sort(suInfoList, new Comparator () {
public int compare(Object o1, Object o2) {
return ((ServiceUnitInfo)o1).getName().compareTo(
((ServiceUnitInfo)o2).getName() );
}
});
}
catch ( ClassCastException ccEx )
{
// log and
// do nothing.
}
catch ( UnsupportedOperationException unsupEx)
{
// log and
// do nothing.
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy