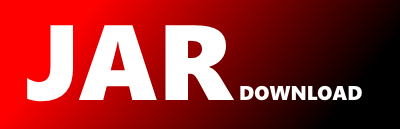
com.sun.jbi.ui.common.Util Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jbi-admin-common Show documentation
Show all versions of jbi-admin-common Show documentation
The "jbi-admin-common" Common CLI code
The newest version!
/*
* BEGIN_HEADER - DO NOT EDIT
*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the "License"). You may not use this file except
* in compliance with the License.
*
* You can obtain a copy of the license at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* HEADER in each file and include the License file at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* If applicable add the following below this CDDL HEADER,
* with the fields enclosed by brackets "[]" replaced with
* your own identifying information: Portions Copyright
* [year] [name of copyright owner]
*/
/*
* @(#)Util.java
* Copyright 2004-2007 Sun Microsystems, Inc. All Rights Reserved.
*
* END_HEADER - DO NOT EDIT
*/
package com.sun.jbi.ui.common;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationTargetException;
import java.net.InetAddress;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
* This object provides utility methods common for client or server side code for tools.
* @author Sun Microsystems, Inc.
*/
public class Util
{
/**
* resource bundle
*/
private static I18NBundle sI18NBundle = null;
/** gives the I18N bundle
*@return I18NBundle object
*/
public static I18NBundle getCommonI18NBundle()
{
// lazzy initialize the JBI Client
if ( sI18NBundle == null )
{
sI18NBundle = new I18NBundle("com.sun.jbi.ui.common");
}
return sI18NBundle;
}
/**
* prints the string to debug output
* @param msg text to print
*/
public static void logDebug(String msg)
{
ToolsLogManager.getCommonLogger().log(Level.FINE,msg);
}
/**
* logs the message
* @param logger logger
* @param ex exception
*/
public static void logDebug(Logger logger, Exception ex)
{
logger.log(Level.FINE, ex.getMessage(), ex);
}
/**
* logs the message
* @param ex exception
*/
public static void logDebug(Exception ex)
{
logDebug(ToolsLogManager.getCommonLogger(), ex);
}
/**
* This method creates a new object of specified type using the reflection apis. It
* instantiate the object using its constructor that takes String as a argument.
* This is mainly used to convert the string value to a specified object type.
* @param type the fully qualified name of the desired class
* @param arg String value to be passed to the class constructor
* @throws java.lang.ClassNotFoundException on error
* @throws java.lang.NoSuchMethodException on error
* @throws java.lang.InstantiationException on error
* @throws java.lang.IllegalAccessException on error
* @throws java.lang.reflect.InvocationTargetException on error
* @throws java.lang.IllegalArgumentException on error
* @return constrcuted object of the type specified with the string value
*/
public static Object newInstance(String type, String arg)
throws ClassNotFoundException, NoSuchMethodException, InstantiationException,
IllegalAccessException, InvocationTargetException, IllegalArgumentException
{
Class objClass = null;
Object instance = null;
// check for the primitive types
// boolean, byte, char, short, int, long, float, double and void
if ( type.equals("boolean"))
{
objClass = Boolean.class;
checkBooleanArgumentString(arg);
}
else if (type.equals("byte"))
{
objClass = Byte.class;
}
else if (type.equals("char"))
{
objClass = Character.class;
}
else if (type.equals("short"))
{
objClass = Short.class;
}
else if (type.equals("int"))
{
objClass = Integer.class;
}
else if (type.equals("long"))
{
objClass = Long.class;
}
else if (type.equals("float"))
{
objClass = Float.class;
}
else if (type.equals("double"))
{
objClass = Double.class;
}
else if (type.equals("void"))
{
objClass = Void.class;
}
else
{
objClass = Class.forName(type);
}
Class[] argClass = new Class[] {String.class};
Constructor objConstructor = objClass.getConstructor(argClass);
String[] argValue = { arg };
try
{
instance = objConstructor.newInstance(argValue);
}
catch(InvocationTargetException ex)
{
String msg = getCommonI18NBundle().getMessage(
"ui.util.invalid.object.value.error",
new Object[]{arg, objClass.getName()});
IllegalArgumentException iaex = new IllegalArgumentException(msg, ex);
throw iaex;
}
return instance;
}
/**
* This method checks that the host name is a localhost or a remote host. This method
* compares the host name on which this method is getting executes with the host name
* that is passed to this method. if the comparison matches it returns true.
* @param host host name to check for local or remote host.
* @throws java.net.UnknownHostException on errror.
* @return true if the host name passed is a localhost else false.
*/
public static boolean isLocalHost(String host) throws java.net.UnknownHostException
{
String localhostName = "localhost";
String loopbackIP = "127.0.0.1";
String loopbackIP6 = "0:0:0:0:0:0:0:1";
String remoteHost = null;
if ( host != null )
{
remoteHost = host.trim().toLowerCase();
}
//logDebug("Checking for Remote HOST " + remoteHost);
if ( remoteHost == null || remoteHost.length() == 0 ||
remoteHost.equalsIgnoreCase(localhostName) ||
remoteHost.equalsIgnoreCase(loopbackIP) ||
remoteHost.equalsIgnoreCase(loopbackIP6))
{
return true;
}
InetAddress localInetAddr = InetAddress.getLocalHost();
String localHostIP = localInetAddr.getHostAddress();
//logDebug("Local Host IP " + localHostIP);
InetAddress[] remoteInetAddrs = InetAddress.getAllByName(remoteHost);
//logDebug("Remote InetAddress Size " + remoteInetAddrs.length);
for ( int i=0; i < remoteInetAddrs.length; ++i )
{
String remoteHostIP = remoteInetAddrs[i].getHostAddress();
//logDebug("Comapring with Remote Host IP : " + remoteHostIP);
if ( localHostIP.equalsIgnoreCase(remoteHostIP) ||
remoteHostIP.equalsIgnoreCase(loopbackIP) ||
remoteHostIP.equalsIgnoreCase(loopbackIP6))
{
return true;
}
}
return false;
}
/**
* This method will return the return the host address information for the given
* host name in the format of / .
* @param host the name of the host (ip address string or name of host).
* @throws java.net.UnknownHostException on error.
* @return the host name in the format of /
*/
public static String getHostName(String aHostName) throws java.net.UnknownHostException
{
InetAddress ia = null;
if ((aHostName.equalsIgnoreCase("localhost")) ||
(aHostName.equals(""))) {
ia = InetAddress.getLocalHost();
}
else {
ia = InetAddress.getByName(aHostName);
}
String hostName = ia.getHostName();
String hostAddress = ia.getHostAddress();
String returnStr = hostName + "/" + hostAddress;
return returnStr;
}
/**
*
* @param arg - boolean argument string
* @throws IllegalArgumentException if the arg string is not a valid boolean
* value string
*/
private static void checkBooleanArgumentString(String arg)
throws java.lang.IllegalArgumentException
{
String msg = getCommonI18NBundle().getMessage(
"ui.util.invalid.boolean.value.error", new Object[]{arg});
if ( arg == null )
{
throw new java.lang.IllegalArgumentException(msg);
}
if ( !(Boolean.FALSE.toString().equalsIgnoreCase(arg)) &&
!(Boolean.TRUE.toString().equalsIgnoreCase(arg)))
{
throw new java.lang.IllegalArgumentException(msg);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy