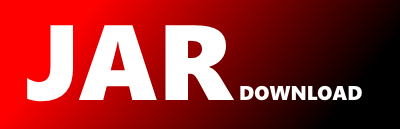
com.sun.jbi.wsdl11wrapper.Wsdl11WrapperHelper Maven / Gradle / Ivy
/*
* BEGIN_HEADER - DO NOT EDIT
*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the "License"). You may not use this file except
* in compliance with the License.
*
* You can obtain a copy of the license at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* HEADER in each file and include the License file at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* If applicable add the following below this CDDL HEADER,
* with the fields enclosed by brackets "[]" replaced with
* your own identifying information: Portions Copyright
* [year] [name of copyright owner]
*/
/*
* @(#)Wsdl11WrapperHelper.java
* Copyright 2004-2007 Sun Microsystems, Inc. All Rights Reserved.
*
* END_HEADER - DO NOT EDIT
*/
package com.sun.jbi.wsdl11wrapper;
import org.w3c.dom.*;
import java.util.*;
import javax.wsdl.*;
import javax.wsdl.extensions.*;
import javax.wsdl.factory.*;
import javax.wsdl.xml.*;
import javax.xml.namespace.QName;
import javax.xml.parsers.*;
import javax.xml.transform.*;
import javax.xml.transform.dom.*;
import javax.xml.transform.stream.*;
/**
* DOCUMENT ME!
*
* @author Sun Microsystems, Inc.
*/
public class Wsdl11WrapperHelper
{
/**
*
*/
private Definition mDefinition;
/**
*
*/
private Document mDoc;
/**
*
*/
public static final String WRAPPER_DEFAULT_NAMESPACE =
"http://java.sun.com/xml/ns/jbi/wsdl-11-wrapper";
/**
*
*/
public static final String WRAPPER_MESSAGE = "jbi:documentroot";
/**
*
*/
public static final String WSDL11 =
"http://schemas.xmlsoap.org/wsdl/";
/**
* Creates a new Wsdl11WrapperHelper object.
*
*/
public Wsdl11WrapperHelper()
{
;
}
/**
* Creates a new Wsdl11WrapperHelper object.
*
* @param wsdlfile
* @param inputfile
*/
public Wsdl11WrapperHelper(
String wsdlfile,
String inputfile)
{
try
{
javax.wsdl.factory.WSDLFactory mFactory = WSDLFactory.newInstance();
javax.wsdl.xml.WSDLReader mReader = mFactory.newWSDLReader();
mDefinition = mReader.readWSDL(null, wsdlfile);
DocumentBuilderFactory docfac =
DocumentBuilderFactory.newInstance();
docfac.setNamespaceAware(true);
mDoc = docfac.newDocumentBuilder().parse(inputfile);
}
catch (Exception e)
{
e.printStackTrace();
}
}
/**
* Creates a new Wsdl11WrapperHelper object.
*
* @param wsdldef
*/
public Wsdl11WrapperHelper(Object wsdldef)
{
mDefinition = (Definition)wsdldef;
}
private Document getDocFromSource(Source src) throws Wsdl11WrapperHelperException
{
Document normaldoc = null;
try
{
DOMResult result = new DOMResult();
TransformerFactory fac = TransformerFactory.newInstance();
Transformer trans = fac.newTransformer();
trans.transform(src, result);
Node node = result.getNode();
if (node.getNodeType() == Node.DOCUMENT_NODE)
{
normaldoc = (Document) node;
}
else if (node.getNodeType() == Node.ELEMENT_NODE)
{
normaldoc = ((Element)(node)).getOwnerDocument();
}
}
catch (Exception e)
{
e.printStackTrace();
throw new Wsdl11WrapperHelperException("Cannot retrieve the DOM from Source", e);
}
return normaldoc;
}
public void parse(String wsdlfile) throws Wsdl11WrapperHelperException
{
try
{
javax.wsdl.factory.WSDLFactory mFactory = WSDLFactory.newInstance();
javax.wsdl.xml.WSDLReader mReader = mFactory.newWSDLReader();
mDefinition = mReader.readWSDL(null, wsdlfile);
}
catch (Exception e)
{
throw new Wsdl11WrapperHelperException("Cannot parse Wsdl 1.1 file", e);
}
}
public boolean isWsdl11() throws Wsdl11WrapperHelperException
{
try
{
String xmlns = mDefinition.getNamespace ("");
if (xmlns.trim ().equals(WSDL11))
{
return true;
}
}
catch (Exception e)
{
throw new Wsdl11WrapperHelperException("Cannot get version", e);
}
return false;
}
public Document wrapMessage(Source src, QName service, String endpoint,
String operation, boolean input) throws Wsdl11WrapperHelperException
{
Document tempdoc = getDocFromSource(src);
return wrapMessage(tempdoc, service, endpoint, operation, input);
}
/**
* DOCUMENT ME!
*
* @param doc
* @param service
* @param enpoint
* @param operation
* @param input
*
* @return
*/
public Document wrapMessage(
Document doc,
QName service,
String enpoint,
String operation,
boolean input) throws Wsdl11WrapperHelperException
{
Document d = null;
if (doc == null)
{
doc = mDoc;
}
try
{
WrapperBuilder builder = HelperFactory.createBuilder();
Message msg = getMessage(service, enpoint, operation, input);
builder.initialize(null, msg, operation);
Map parts = msg.getParts();
Iterator iter = parts.values().iterator();
while (iter.hasNext())
{
Part part = (Part) iter.next();
Element partelement = findpart(doc, part);
if (partelement != null)
{
builder.addPart(part.getName(), partelement);
}
}
d = builder.getResult();
}
catch (Exception e)
{
e.printStackTrace();
throw new Wsdl11WrapperHelperException("Cannot create Wsdl 1.1 Wrapper", e);
}
return d;
}
public Document unwrapFault(
Source wrappeddoc,
QName service,
String endpoint,
String operation) throws Wsdl11WrapperHelperException
{
return unwrapFault(getDocFromSource(wrappeddoc), service, endpoint, operation);
}
public Document wrapFault(
Source doc,
QName service,
String endpoint,
String operation,
String fault) throws Wsdl11WrapperHelperException
{
return wrapFault(getDocFromSource(doc), service, endpoint, operation, fault);
}
/**
*
*
* @param doc
* @param service
* @param endpoint
* @param operation
* @param fault
*
* @return
*/
public Document wrapFault(
Document doc,
QName service,
String endpoint,
String operation,
String fault) throws Wsdl11WrapperHelperException
{
Document d = null;
try
{
WrapperBuilder builder = HelperFactory.createBuilder();
Message msg = getFaultMessage(service, endpoint, operation, fault);
builder.initialize(null, msg, operation);
Map parts = msg.getParts();
Iterator iter = parts.values().iterator();
while (iter.hasNext())
{
Part part = (Part) iter.next();
Element partelement = findpart(doc, part);
if (partelement != null)
{
builder.addPart(part.getName(), partelement);
}
}
d = builder.getResult();
}
catch (Exception e)
{
e.printStackTrace();
throw new Wsdl11WrapperHelperException("Cannot create Wsdl 1.1 wrapper for Fault", e);
}
return d;
}
public Document unwrapMessage(
Source wrappeddoc,
QName service,
String endpoint,
String operation,
boolean input) throws Wsdl11WrapperHelperException
{
return unwrapMessage(getDocFromSource(wrappeddoc), service, endpoint, operation, input);
}
/**
*
*
* @param wrappeddoc
* @param service
* @param endpoint
* @param operation
*
* @return
*/
public Document unwrapFault(
Document wrappeddoc,
QName service,
String endpoint,
String operation) throws Wsdl11WrapperHelperException
{
Document d = null;
try
{
d = DocumentBuilderFactory.newInstance().newDocumentBuilder()
.newDocument();
WrapperParser parser = HelperFactory.createParser();
parser.parse(wrappeddoc, mDefinition);
String msgname = parser.getMessageName();
QName msgtype = parser.getMessageType();
Message msg = mDefinition.getMessage(msgtype);
Map parts = msg.getParts();
Iterator iter = parts.values().iterator();
Element docroot = null;
if (parts.size() > 1)
{
docroot =
d.createElementNS(WRAPPER_DEFAULT_NAMESPACE, WRAPPER_MESSAGE);
}
while (iter.hasNext())
{
Part part = (Part) iter.next();
if (parser.hasPart(part.getName()))
{
NodeList partnodes = parser.getPartNodes(part.getName());
for (int i = 0; i < partnodes.getLength(); i++)
{
Node temp = (Node) partnodes.item(i);
if ((temp == null) || (temp.getLocalName() == null))
{
continue;
}
Node node = d.importNode(temp, true);
if (docroot != null)
{
docroot.appendChild(node);
}
else
{
d.appendChild(node);
}
}
}
}
d.normalize();
}
catch (Exception e)
{
e.printStackTrace();
throw new Wsdl11WrapperHelperException("Cannot un-wrap fault message", e);
}
return d;
}
/**
*
*
* @param wrappeddoc
* @param service
* @param endpoint
* @param operation
* @param input
*
* @return
*/
public Document unwrapMessage(
Document wrappeddoc,
QName service,
String endpoint,
String operation,
boolean input) throws Wsdl11WrapperHelperException
{
Document d = null;
if (wrappeddoc == null)
{
wrappeddoc = mDoc;
}
try
{
d = DocumentBuilderFactory.newInstance().newDocumentBuilder()
.newDocument();
WrapperParser parser = HelperFactory.createParser();
Message msg = getMessage(service, endpoint, operation, input);
parser.parse(wrappeddoc, msg);
Map parts = msg.getParts();
Iterator iter = parts.values().iterator();
Element docroot = null;
if (parts.size() > 1)
{
docroot =
d.createElementNS(WRAPPER_DEFAULT_NAMESPACE, WRAPPER_MESSAGE);
}
while (iter.hasNext())
{
Part part = (Part) iter.next();
if (parser.hasPart(part.getName()))
{
NodeList partnodes = parser.getPartNodes(part.getName());
for (int i = 0; i < partnodes.getLength(); i++)
{
Node temp = (Node) partnodes.item(i);
if ((temp == null) || (temp.getLocalName() == null))
{
continue;
}
Node node = d.importNode(temp, true);
if (docroot != null)
{
docroot.appendChild(node);
}
else
{
d.appendChild(node);
}
}
}
}
d.normalize();
}
catch (Exception e)
{
e.printStackTrace();
throw new Wsdl11WrapperHelperException("Cannot un-wrap output message", e);
}
return d;
}
/**
* DOCUMENT ME!
*
* @param doc
* @param part
*
* @return
*/
private Element findpart(
Document doc,
Part part)
{
Element ele = null;
try
{
QName partelement = part.getElementName();
QName parttype = part.getTypeName();
NodeList nl = null;
if (partelement != null)
{
nl = doc.getElementsByTagNameNS("*", partelement.getLocalPart());
}
if (parttype != null)
{
nl = doc.getElementsByTagNameNS("*",
part.getName());
}
if ((nl != null) && (nl.getLength() > 0))
{
ele = (Element) nl.item(0);
}
}
catch (Exception e)
{
e.printStackTrace();
}
return ele;
}
/**
* DOCUMENT ME!
*
* @param service
* @param portstring
* @param oper
* @param input
*
* @return
*/
public Message getMessage(
QName service,
String portstring,
String oper,
boolean input)
{
Message msg = null;
try
{
Service ser = mDefinition.getService(service);
//Port port = ser.getPort(\");
Port port = ser.getPort(portstring);
List l = port.getBinding().getPortType().getOperations();
Iterator iter = l.iterator();
while (iter.hasNext())
{
Operation operation = (Operation) iter.next();
if (operation.getName().equals(oper))
{
if (input)
{
return operation.getInput().getMessage();
}
else
{
return operation.getOutput().getMessage();
}
}
}
}
catch (Exception e)
{
e.printStackTrace();
}
return null;
}
/**
* DOCUMENT ME!
*
* @param service
* @param portstring
* @param oper
* @param fault
*
* @return
*/
public Message getFaultMessage(
QName service,
String portstring,
String oper,
String fault)
{
Message msg = null;
try
{
Service ser = mDefinition.getService(service);
Port port = ser.getPort(portstring);
List l = port.getBinding().getPortType().getOperations();
Iterator iter = l.iterator();
while (iter.hasNext())
{
Operation operation = (Operation) iter.next();
if (operation.getName().equals(oper))
{
if (fault != null)
{
return operation.getFault(fault).getMessage();
}
else
{
Map faults = operation.getFaults();
Iterator faultiter = faults.values().iterator();
Fault defaultflt = (Fault) faultiter.next();
return defaultflt.getMessage();
}
}
}
}
catch (Exception e)
{
e.printStackTrace();
}
return null;
}
/**
* DOCUMENT ME!
*
* @param args
*/
/*
public static void main(String [] args)
{
if (args.length != 2)
{
System.out.println("Takes wsdl file name and the document to wrap");
System.exit(1);
}
Wsdl11WrapperHelper wrapper = new Wsdl11WrapperHelper(args[0], args[1]);
QName service =
new QName("http://sun.com/jbi/binding/soap/stockquote/wsdl",
"QuoteService");
Document d = wrapper.wrapMessage(null, service, "QuotePort", "getQuote", true);
try
{
TransformerFactory tFactory = TransformerFactory.newInstance();
Transformer trans = tFactory.newTransformer();
StreamResult result = new StreamResult(System.out);
trans.transform(new DOMSource(d), result);
}
catch (Exception e)
{
e.printStackTrace();
}
Document d1 =
wrapper.unwrapMessage(null, service, "QuotePort", "getQuote", false);
try
{
TransformerFactory tFactory = TransformerFactory.newInstance();
Transformer trans = tFactory.newTransformer();
StreamResult result = new StreamResult(System.out);
trans.transform(new DOMSource(d1), result);
}
catch (Exception e)
{
e.printStackTrace();
}
}
*/
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy