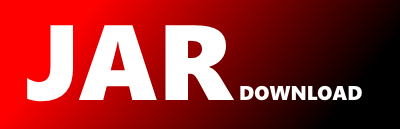
com.sun.jbi.wsdl11wrapper.impl.WrapperBuilderImpl Maven / Gradle / Ivy
/*
* BEGIN_HEADER - DO NOT EDIT
*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the "License"). You may not use this file except
* in compliance with the License.
*
* You can obtain a copy of the license at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* HEADER in each file and include the License file at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* If applicable add the following below this CDDL HEADER,
* with the fields enclosed by brackets "[]" replaced with
* your own identifying information: Portions Copyright
* [year] [name of copyright owner]
*/
/*
* @(#)WrapperBuilderImpl.java
* Copyright 2004-2007 Sun Microsystems, Inc. All Rights Reserved.
*
* END_HEADER - DO NOT EDIT
*/
package com.sun.jbi.wsdl11wrapper.impl;
import com.sun.jbi.wsdl11wrapper.HelperFactory;
import com.sun.jbi.wsdl11wrapper.WrapperBuilder;
import com.sun.jbi.wsdl11wrapper.WrapperProcessingException;
import com.sun.jbi.wsdl11wrapper.util.WrapperUtil;
import java.util.Map;
import java.util.HashMap;
import java.util.List;
import java.util.Iterator;
import javax.wsdl.Definition;
import javax.wsdl.Message;
import javax.wsdl.Part;
import javax.wsdl.WSDLException;
import javax.wsdl.factory.WSDLFactory;
import javax.xml.namespace.QName;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.w3c.dom.Document;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
/**
* Assists in building the WSDL 1.1 wrapper for normalized messages specified by JBI
*
* Usage sequence:
* initialize()
* addParts() or multiple addPart() calls
* getResult()
*
* The same instance should not be used by multiple threads concurrently as it is
* not guaranteed to be thread safe - and maintains state
*
* The re-use of the same instance however is encouraged.
*
* @author aegloff
*/
public class WrapperBuilderImpl implements WrapperBuilder {
DocumentBuilder mBuilder;
Document normalDoc;
Element jbiMessageWrapper;
Message messageDefinition;
WSDLInfo info;
boolean isResultPrepared;
Map mPartNameToPartNodes;
/**
* Creates a new instance
*
* The preferred way to create and use this builder implementation is to use
* the HelperFactory
instead.
*/
public WrapperBuilderImpl() throws WrapperProcessingException {
}
/**
* Re-sets the result document, sets the WSDL message definition of the message to normalize
*/
public void initialize(Document docToPopulate, Message wsdlMessageDefinition, String operationBindingMessageName) throws WrapperProcessingException {
isResultPrepared = false;
mPartNameToPartNodes = null;
this.normalDoc = docToPopulate;
this.messageDefinition = wsdlMessageDefinition;
if (docToPopulate == null) {
try {
normalDoc = newDocument();
} catch (ParserConfigurationException ex) {
throw new WrapperProcessingException("Failed to create an empty target document for building the wrapped normalized message: " + ex.getMessage(), ex);
}
}
info = WSDLInfo.getInstance(wsdlMessageDefinition);
// Add a message wrapper
jbiMessageWrapper = WrapperUtil.createJBIMessageWrapper(normalDoc, info.getMessageType(), operationBindingMessageName);
normalDoc.appendChild(jbiMessageWrapper);
}
/**
* Add a part in the right position (wrapped in a JBI part wrapper) to the JBI message wrapper element
* @param partName the name of the message part
* @param partNode the part node (payload)
* The part node does not have to be associated with the normalDoc yet, it will be imported
*/
public void addPart(String partName, org.w3c.dom.NodeList partNodes) throws WrapperProcessingException {
List partsOrder = info.getPartsOrder();
int partPos = partsOrder.indexOf(partName);
if (partPos > -1) {
if (mPartNameToPartNodes == null) {
mPartNameToPartNodes = new HashMap();
}
mPartNameToPartNodes.put(partName, partNodes);
} else {
throw new WrapperProcessingException("Unknown part " + partName + " is not defined in the WSDL message definition for " + info.getMessageType() + ". Cannot add part to normalized message.");
}
}
/**
* Add a part in the right position (wrapped in a JBI part wrapper) to the JBI message wrapper element
* @param partName the name of the message part
* @param partNode the part node (payload)
* The part node does not have to be associated with the normalDoc yet, it will be imported
*/
public void addPart(String partName, Element partNode) throws WrapperProcessingException {
addPart(partName, new NodeListImpl(partNode));
/*
List partsOrder = info.getPartsOrder();
int partPos = partsOrder.indexOf(partName);
if (partPos > -1) {
if (mPartNameToPartNodes == null) {
mPartNameToPartNodes = new HashMap();
}
mPartNameToPartNodes.put(partName, partNode);
} else {
throw new WrapperProcessingException("Unknown part " + partName + " is not defined in the WSDL message definition for " + info.getMessageType() + ". Cannot add part to normalized message.");
}
*/
}
/**
* Add parts in the right order (each wrapped in a JBI part wrapper) to the passed in JBI message wrapper element
* The jbiMessageWrapper must be a node of the normalDoc.
* @param normalDoc The target document of the normalization
* @param jbiMessageWrapper The message wrapper element to add the jbi part wrappers and part payload to
* @param messageDefinintion the WSDL message definition
* @param partNameToPartNodes a mapping from the part name to the part NodeList (payload).
* The part node does not have to be associated with the normalDoc yet, it will be imported
*/
public void addParts(Map partNameToPartNodes) {
mPartNameToPartNodes = partNameToPartNodes;
}
/**
* Retrieve the Normalized Message with the parts added (addPart(s)) since the initialize() call
* Make sure to only retrieve the result after all desired parts have been added.
*
* Parts added after the result of a build squence has been retrieved already
* (meaning without starting a new build squence by calling initialize) will NOT be included
* in subsequent retrievals of Result
*/
public Document getResult() throws WrapperProcessingException {
if (!isResultPrepared) {
Iterator partsIter = info.getOrderedMessageParts().iterator();
while (partsIter.hasNext()) {
Part aPart = (Part)partsIter.next();
String partName = aPart.getName();
NodeList nodes = null;
if (mPartNameToPartNodes != null) {
Object payload = mPartNameToPartNodes.get(partName);
if (payload instanceof NodeList) {
nodes = (NodeList) payload;
} else {
throw new WrapperProcessingException("Part payload added is not a NodeList: " + (payload == null ? "payload is null" : payload.getClass().getName()));
}
}
Element wrapperElem = WrapperUtil.importJBIWrappedPart(normalDoc, nodes);
jbiMessageWrapper.appendChild(wrapperElem);
}
isResultPrepared = true;
}
return normalDoc;
}
public Message getStatusMessage() throws WrapperProcessingException {
Message msg = null;
try {
WSDLFactory factory = javax.wsdl.factory.WSDLFactory.newInstance();
Definition def = factory.newDefinition();
msg = def.createMessage();
msg.setQName(new QName(WrapperBuilder.STATUS_TAG));
Part part = def.createPart();
part.setName(WrapperBuilder.RESULT_TAG);
msg.addPart(part);
} catch (WSDLException ex) {
throw new WrapperProcessingException(ex);
}
return msg;
}
public Document getStatusDocument(String statusMessage) throws WrapperProcessingException {
Document res = null;
try {
Document doc = DocumentBuilderFactory.newInstance().newDocumentBuilder().newDocument();
WrapperBuilder builder = HelperFactory.createBuilder();
builder.initialize(doc, getStatusMessage(), null);
// add the "wrapper" elements for "normalizing" doc/lit
Element statusElem = doc.createElement(WrapperBuilder.STATUS_TAG);
statusElem.appendChild(doc.createTextNode(statusMessage));
builder.addPart(WrapperBuilder.RESULT_TAG, statusElem);
res = builder.getResult();
} catch (ParserConfigurationException ex) {
throw new WrapperProcessingException(ex);
}
return res;
}
private Document newDocument() throws ParserConfigurationException {
if (mBuilder == null) {
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
mBuilder = factory.newDocumentBuilder();
}
return mBuilder.newDocument();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy