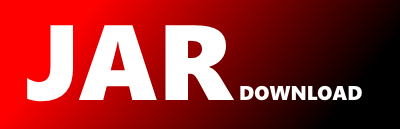
com.sun.jbi.wsdl2.impl.Binding Maven / Gradle / Ivy
/*
* BEGIN_HEADER - DO NOT EDIT
*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the "License"). You may not use this file except
* in compliance with the License.
*
* You can obtain a copy of the license at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* HEADER in each file and include the License file at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* If applicable add the following below this CDDL HEADER,
* with the fields enclosed by brackets "[]" replaced with
* your own identifying information: Portions Copyright
* [year] [name of copyright owner]
*/
/*
* @(#)Binding.java
* Copyright 2004-2007 Sun Microsystems, Inc. All Rights Reserved.
*
* END_HEADER - DO NOT EDIT
*/
package com.sun.jbi.wsdl2.impl;
import javax.xml.namespace.QName;
import org.w3c.dom.DocumentFragment;
import org.w3.ns.wsdl.BindingType;
/**
* Abstract implementation of
* WSDL 2.0 Binding component.
*
* @author Sun Microsystems, Inc.
*/
abstract class Binding extends ExtensibleDocumentedComponent
implements com.sun.jbi.wsdl2.Binding
{
/**
* Get the BindingType XML bean behind this Binding component.
*
* @return The BindingType XML bean behind this component.
*/
protected final BindingType getBean()
{
return (BindingType) this.mXmlObject;
}
/**
* Construct an abstract Binding implementation base component.
*
* @param bean The XML bean for this Binding component
*/
Binding(BindingType bean)
{
super(bean);
}
/**
* Get local name of this binding component.
*
* @return Local name of this binding component
*/
public String getName()
{
return getBean().getName();
}
/**
* Set local name of this binding component.
*
* @param theName Local name of this binding component
*/
public void setName(String theName)
{
getBean().setName(theName);
}
/**
* Create a new operation, appending it to this binding's operations
* list.
*
* @return Newly created operation, appended to this binding's operation
* list.
*/
public abstract com.sun.jbi.wsdl2.BindingOperation addNewOperation();
/**
* Create a new binding fault, appending it to this binding's faults
* list.
*
* @param ref Interface fault to which the new binding fault adds binding
* information
* @return Newly created binding fault, appended to the faults list.
*/
public abstract com.sun.jbi.wsdl2.BindingFault addNewBindingFault(
com.sun.jbi.wsdl2.InterfaceFault ref);
/**
* Get URI indicating the type of this binding.
*
* @return URI indicating the type of this binding
*/
public String getType()
{
return getBean().getType();
}
/**
* Set URI indicating the type of this binding
*
* @param theType URI indicating the type of this binding
*/
public void setType(String theType)
{
getBean().setType(theType);
}
/**
* Return this WSDL binding as an XML string.
*
* @return This binding, serialized as an XML string.
*/
public abstract String toXmlString();
/**
* Return this binding as a DOM document fragment. The DOM subtree is a
* copy; altering it will not affect this binding.
*
* @return This binding, as a DOM document fragment.
*/
public abstract DocumentFragment toXmlDocumentFragment();
}
// End-of-file: Binding.java
© 2015 - 2025 Weber Informatics LLC | Privacy Policy