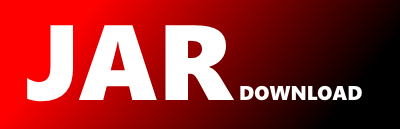
com.sun.jbi.wsdl2.impl.BindingFaultImpl Maven / Gradle / Ivy
/*
* BEGIN_HEADER - DO NOT EDIT
*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the "License"). You may not use this file except
* in compliance with the License.
*
* You can obtain a copy of the license at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* HEADER in each file and include the License file at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* If applicable add the following below this CDDL HEADER,
* with the fields enclosed by brackets "[]" replaced with
* your own identifying information: Portions Copyright
* [year] [name of copyright owner]
*/
/*
* @(#)BindingFaultImpl.java
* Copyright 2004-2007 Sun Microsystems, Inc. All Rights Reserved.
*
* END_HEADER - DO NOT EDIT
*/
package com.sun.jbi.wsdl2.impl;
import javax.xml.namespace.QName;
import org.w3.ns.wsdl.BindingFaultType;
/**
* Implementation of WSDL 2.0 Binding Fault component.
*
* @author Sun Microsystems, Inc.
*/
final class BindingFaultImpl extends BindingFault
{
/** The definitions component this binding belongs to */
private DescriptionImpl mContainer;
/**
* Get the container for this component.
*
* @return The component for this component
*/
protected DescriptionImpl getContainer()
{
return this.mContainer;
}
/**
* Construct a binding fault component implementation object from the given
* XML bean.
*
* @param bean The binding XML bean to use to construct this component.
* @param defs The container for this binding fault component.
*/
private BindingFaultImpl(BindingFaultType bean, DescriptionImpl defs)
{
super(bean);
this.mContainer = defs;
}
/**
* Get interface fault that is bound to this binding fault.
*
* @return Interface fault that is bound to this binding fault
*/
public QName getRef()
{
return getBean().getRef();
}
/**
* Set interface fault that is bound to this binding fault.
*
* @param theRef Interface fault that is bound to this binding fault
*/
public void setRef(QName theRef)
{
getBean().setRef(theRef);
}
/** Map of WSDL-defined attribute QNames. Keyed by QName.toString value */
private static java.util.Map sWsdlAttributeQNames = null;
/**
* Worker class method for {@link #getWsdlAttributeNameMap()}.
*
* @return Map of WSDL-defined attribute QNames for this component,
* indexed by QName.toString()
*/
static synchronized java.util.Map getAttributeNameMap()
{
if (sWsdlAttributeQNames == null)
{
sWsdlAttributeQNames = XmlBeansUtil.getAttributesMap(BindingFaultType.type);
}
return sWsdlAttributeQNames;
}
/**
* Get map of WSDL-defined attribute QNames for this component, indexed by
* canonical QName string (see {@link javax.xml.namespace.QName#toString()}.
*
* Implementation note: since this method is declared in the public API
* interface
, it has to be a member, not static. We delegate
* to a class method to do the actual work.
*
* @return Map of WSDL-defined attribute QNames for this component,
* indexed by QName.toString()
*/
public java.util.Map getWsdlAttributeNameMap()
{
return getAttributeNameMap();
}
/**
* A factory class for creating / finding components for given XML beans.
*
* This factory guarantees that there will only be one component for each
* XML bean instance.
*/
static class Factory
{
/**
* Find the WSDL binding component associated with the given XML
* bean, creating a new component if necessary.
*
* This is thread-safe.
*
* @param bean The XML bean to find the component for.
* @param defs The container for the component.
* @return The WSDL binding fault component for the given
* bean
(null if the bean
is null).
*/
static BindingFaultImpl getInstance(
BindingFaultType bean, DescriptionImpl defs)
{
BindingFaultImpl result = null;
if (bean != null)
{
java.util.Map map = defs.getBindingFaultMap();
synchronized (map)
{
result = (BindingFaultImpl) map.get(bean);
if (result == null)
{
result = new BindingFaultImpl(bean, defs);
map.put(bean, result);
}
}
}
return result;
}
}
}