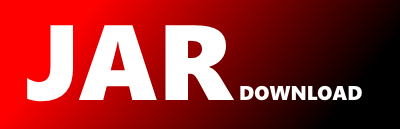
com.sun.jbi.wsdl2.impl.BindingOperationImpl Maven / Gradle / Ivy
/*
* BEGIN_HEADER - DO NOT EDIT
*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the "License"). You may not use this file except
* in compliance with the License.
*
* You can obtain a copy of the license at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* HEADER in each file and include the License file at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* If applicable add the following below this CDDL HEADER,
* with the fields enclosed by brackets "[]" replaced with
* your own identifying information: Portions Copyright
* [year] [name of copyright owner]
*/
/*
* @(#)BindingOperationImpl.java
* Copyright 2004-2007 Sun Microsystems, Inc. All Rights Reserved.
*
* END_HEADER - DO NOT EDIT
*/
package com.sun.jbi.wsdl2.impl;
import java.util.Map;
import javax.xml.namespace.QName;
import org.w3.ns.wsdl.BindingOperationMessageType;
import org.w3.ns.wsdl.BindingOperationType;
/**
* Implementation of WSDL 2.0 Binding Operation Component.
*
* @author Sun Microsystems, Inc.
*/
final class BindingOperationImpl extends BindingOperation
{
/** Container for this component */
private DescriptionImpl mContainer;
/**
* Get the container for this component.
*
* @return The component for this component
*/
protected DescriptionImpl getContainer()
{
return this.mContainer;
}
/**
* Construct a binding operation component implementation object from the
* given XML bean.
* @param type The binding operation XML bean to use to construct this
* component.
* @param defs Container for this component.
*/
private BindingOperationImpl(BindingOperationType type,
DescriptionImpl defs)
{
super(type);
this.mContainer = defs;
}
/** Map of WSDL-defined attribute QNames. Keyed by QName.toString value */
private static java.util.Map sWsdlAttributeQNames = null;
/**
* Worker class method for {@link #getWsdlAttributeNameMap()}.
*
* @return Map of WSDL-defined attribute QNames for this component,
* indexed by QName.toString()
*/
static synchronized java.util.Map getAttributeNameMap()
{
if (sWsdlAttributeQNames == null)
{
sWsdlAttributeQNames = XmlBeansUtil.getAttributesMap(
BindingOperationType.type);
}
return sWsdlAttributeQNames;
}
/**
* Get map of WSDL-defined attribute QNames for this component, indexed by
* canonical QName string (see {@link javax.xml.namespace.QName#toString()}.
*
* @return Map of WSDL-defined attribute QNames for this component,
* indexed by QName.toString()
*/
public java.util.Map getWsdlAttributeNameMap()
{
return getAttributeNameMap();
}
/**
* Get the qualified name of the Interface Operation being bound.
*
* @return Qualified name of the Interface Operation being bound
*/
public QName getInterfaceOperation()
{
return getBean().getRef();
}
/**
* Set operation being bound.
*
* @param theInterfaceOperation Qualified name of the Interface Operation
* being bound
*/
public void setInterfaceOperation(QName theInterfaceOperation)
{
getBean().setRef(theInterfaceOperation);
}
/**
* Get the number of BindingMessageReference items in inputs.
*
* @return The number of BindingMessageReference items in inputs
*/
public int getInputsLength()
{
return getBean().sizeOfInputArray();
}
/**
* Get input binding message references by indexed position.
*
* @param index Indexed position value 0..length-1
* @return Input binding message references at given index
position.
*/
public com.sun.jbi.wsdl2.BindingMessageReference getInput(int index)
{
return BindingMessageReferenceImpl.Factory.getInstance(
getBean().getInputArray(index),
mContainer);
}
/**
* Set input binding message references by indexed position.
*
* @param index Indexed position value (0..length-1) of the item to set
* @param theInput Item to add at position index
.
*/
public void setInput(int index, com.sun.jbi.wsdl2.BindingMessageReference theInput)
{
getBean().setInputArray(
index,
theInput != null ? ((BindingMessageReferenceImpl) theInput).getBean()
: null);
}
/**
* Append an item to input binding message references.
*
* @param theInput Item to append to inputs
*/
public void appendInput(com.sun.jbi.wsdl2.BindingMessageReference theInput)
{
final int length = getInputsLength();
setInput(length, theInput);
}
/**
* Remove input binding message references by index position.
*
* @param index The index position of the input to remove
* @return The BindingMessageReference removed, if any.
*/
public com.sun.jbi.wsdl2.BindingMessageReference removeInput(int index)
{
com.sun.jbi.wsdl2.BindingMessageReference result = getInput(index);
getBean().removeInput(index);
return result;
}
/**
* Get the number of BindingMessageReference items in outputs.
*
* @return The number of BindingMessageReference items in outputs
*/
public int getOutputsLength()
{
return getBean().sizeOfOutputArray();
}
/**
* Get output binding message references by indexed position.
*
* @param index Indexed position value 0..length-1
* @return Output binding message references at given index
position.
*/
public com.sun.jbi.wsdl2.BindingMessageReference getOutput(int index)
{
return BindingMessageReferenceImpl.Factory.getInstance(
getBean().getOutputArray(index),
this.mContainer);
}
/**
* Set output binding message references by indexed position.
*
* @param index Indexed position value (0..length-1) of the item to set
* @param theOutput Item to add at position index
.
*/
public void setOutput(int index, com.sun.jbi.wsdl2.BindingMessageReference theOutput)
{
getBean().setOutputArray(
index,
theOutput != null ? ((BindingMessageReferenceImpl) theOutput).getBean()
: null);
}
/**
* Append an item to output binding message references.
*
* @param theOutput Item to append to outputs
*/
public void appendOutput(com.sun.jbi.wsdl2.BindingMessageReference theOutput)
{
final int length = getOutputsLength();
setOutput(length, theOutput);
}
/**
* Remove output binding message references by index position.
*
* @param index The index position of the output to remove
* @return The BindingMessageReference removed, if any.
*/
public com.sun.jbi.wsdl2.BindingMessageReference removeOutput(int index)
{
com.sun.jbi.wsdl2.BindingMessageReference result = getOutput(index);
getBean().removeOutput(index);
return result;
}
/**
* Get the target namespace of this operation
*
* @return Target namespace of this operation
*/
public String getTargetNamespace()
{
return this.mContainer.getTargetNamespace();
}
/**
* Create a new message reference for this operation, appending it to this
* operation's input list.
*
* @param messageLabel NC role name of the message for which binding details
* are provided
* @return The newly created input message reference, appended to this
* operation.
*/
public com.sun.jbi.wsdl2.BindingMessageReference addNewInput(
String messageLabel)
{
BindingOperationMessageType msgBean;
synchronized (getBean().monitor())
{
msgBean = getBean().addNewInput();
msgBean.setMessageLabel(messageLabel);
}
return BindingMessageReferenceImpl.Factory.getInstance(
msgBean,
mContainer);
}
/**
* Create a new message reference for this operation, appending it this
* operation's output list.
*
* @param messageLabel NC role name of the message for which binding details
* are provided.
* @return The newly created output message reference, appended to this
* operation.
*/
public com.sun.jbi.wsdl2.BindingMessageReference addNewOutput(
String messageLabel)
{
BindingOperationMessageType msgBean;
synchronized (getBean().monitor())
{
msgBean = getBean().addNewOutput();
msgBean.setMessageLabel(messageLabel);
}
return BindingMessageReferenceImpl.Factory.getInstance(
msgBean,
mContainer);
}
/**
* A factory class for creating / finding components for given XML beans.
*
* This factory guarantees that there will only be one component for each
* XML bean instance.
*/
static class Factory
{
/**
* Find the binding operation component associated with the given XML
* bean, creating a new component if necessary.
*
* This is thread-safe.
*
* @param bean The XML bean to find the component for.
* @param defs The container for the component.
* @return The binding operation component for the given bean.
*/
static BindingOperationImpl getInstance(BindingOperationType bean,
DescriptionImpl defs)
{
BindingOperationImpl result;
if (bean != null)
{
Map map = defs.getBindingOperationMap();
synchronized (map)
{
result = (BindingOperationImpl) map.get(bean);
if (result == null)
{
result = new BindingOperationImpl(bean, defs);
map.put(bean, result);
}
}
}
else
{
result = null;
}
return result;
}
}
}
// End-of-file: BindingOperationImpl.java