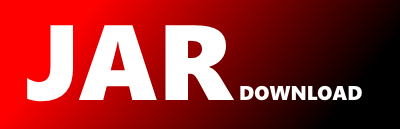
com.sun.jbi.wsdl2.impl.DOMUtilities Maven / Gradle / Ivy
/*
* BEGIN_HEADER - DO NOT EDIT
*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the "License"). You may not use this file except
* in compliance with the License.
*
* You can obtain a copy of the license at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* HEADER in each file and include the License file at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* If applicable add the following below this CDDL HEADER,
* with the fields enclosed by brackets "[]" replaced with
* your own identifying information: Portions Copyright
* [year] [name of copyright owner]
*/
/*
* @(#)DOMUtilities.java
* Copyright 2004-2007 Sun Microsystems, Inc. All Rights Reserved.
*
* END_HEADER - DO NOT EDIT
*/
package com.sun.jbi.wsdl2.impl;
import com.sun.jbi.wsdl2.WsdlException;
import javax.xml.namespace.QName;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import org.w3c.dom.Attr;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.xml.sax.InputSource;
/**
* This class contains a set of utilities for dealing with DOM trees.
*
* @author Sun Microsystems, Inc.
*/
class DOMUtilities
{
/**
* Resolve the given attribute value, which is assumed to contain a prefixed
* name value into a proper QName.
*
* @param attr Attribute containing the prefixed name value
* (e.g., "foo:bar") to be resolved.
* @param documentURI The URI of the containing document.
* @return The qualified name for the attribute value; null if it cannot be
* resolved.
* @exception WsdlException ILLEGAL_QNAME_ATTR_VALUE when the QName value in
* the attribute is illegal.
*/
public static QName resolveNamespace(Attr attr, String documentURI)
throws WsdlException
{
QName result = null;
if (attr != null)
{
Element element = attr.getOwnerElement();
String value = attr.getValue();
try
{
result = resolveNamespace(value, element, documentURI);
}
catch (WsdlException ex)
{
throw new WsdlException(
"Illegal qualified name {0} in value of attribute {2} in "
+ "document {1}",
new Object[] {value, documentURI, attr.getName()});
}
}
return result;
}
/**
* Resolve the given attribute value, which is assumed to contain a prefixed
* name value into a proper QName. The context for the namespace is the
* parent element given.
*
* @param value The attribute value to resolve to a QName.
* @param element The parent element to the attribute value given.
* @param documentURI The URI of the containing document.
* @return The qualified name for the attribute value; null if it cannot be
* resolved.
* @exception WsdlException ILLEGAL_QNAME_VALUE when the QName value in
* the attribute is illegal.
*/
public static QName resolveNamespace(String value, Element element,
String documentURI) throws WsdlException
{
QName result = null;
if (value != null)
{
int valueLength = value.length();
int colonIndex = value.indexOf(':');
String targetNsDecl;
if (colonIndex > 0 && colonIndex < valueLength - 1)
{
targetNsDecl = "xmlns:" + value.substring(0, colonIndex);
}
else if (colonIndex < 0 && valueLength > 0)
{
targetNsDecl = "xmlns";
}
else
{
throw new WsdlException(
"Illegal qualified name {0} in element {2} in document {1}",
new Object[] {value, documentURI, element.getNodeName()});
}
Attr nsDeclAttr = findAttribute(element, targetNsDecl);
if (nsDeclAttr != null)
{
result = new QName(
nsDeclAttr.getValue(),
value.substring(colonIndex + 1));
}
}
return result;
}
/**
* Starting at the given element, search for the named attribute. The
* attribute is searched for from child to parent, up to (and including the
* root document element. This search order conforms to the XML
* name space scoping rules.
Note: this function uses recursion to search
* up the parent edges of the node graph.
*
* @param element The element to search from.
* @param targetName The name of the attribute to search for.
* @return The target attribute; null if not found.
*/
private static Attr findAttribute(Element element, String targetName)
{
Attr result = null;
if (element != null)
{
result = element.getAttributeNode(targetName);
if (result == null)
{
Node parent = element.getParentNode();
if (parent != null && parent.getNodeType() == Node.ELEMENT_NODE)
{
result = findAttribute((Element) parent, targetName);
}
}
}
return result;
}
/**
* Get the document from the given input source.
*
* @param documentBaseURI The base URI for the document to be read.
* @param inputSource The input source for the document.
* @return A DOM document representation of the well-formed XML document
* from the input stream.
* @exception WsdlException if an XML parsing error occurs.
*/
public static Document getDocument(String documentBaseURI,
InputSource inputSource) throws WsdlException
{
Document result;
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
factory.setNamespaceAware(true);
factory.setValidating(false);
try
{
DocumentBuilder builder = factory.newDocumentBuilder();
result = builder.parse(inputSource);
}
catch (Exception ex)
{
throw new WsdlException("Problem parsing '{0}.\n"
+ "\tReason = {0}\n",
new Object[] {documentBaseURI, ex.getMessage()});
}
return result;
}
}