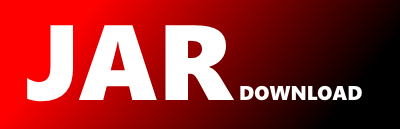
com.sun.jbi.wsdl2.impl.DescriptionImpl Maven / Gradle / Ivy
/*
* BEGIN_HEADER - DO NOT EDIT
*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the "License"). You may not use this file except
* in compliance with the License.
*
* You can obtain a copy of the license at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* HEADER in each file and include the License file at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* If applicable add the following below this CDDL HEADER,
* with the fields enclosed by brackets "[]" replaced with
* your own identifying information: Portions Copyright
* [year] [name of copyright owner]
*/
/*
* @(#)DescriptionImpl.java
* Copyright 2004-2007 Sun Microsystems, Inc. All Rights Reserved.
*
* END_HEADER - DO NOT EDIT
*/
package com.sun.jbi.wsdl2.impl;
import java.io.StringWriter;
import java.lang.ref.WeakReference;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.WeakHashMap;
import javax.xml.namespace.QName;
import org.apache.xmlbeans.XmlOptions;
import org.w3.ns.wsdl.BindingType;
import org.w3.ns.wsdl.DescriptionDocument;
import org.w3.ns.wsdl.DescriptionType;
import org.w3.ns.wsdl.ImportType;
import org.w3.ns.wsdl.IncludeType;
import org.w3.ns.wsdl.InterfaceType;
import org.w3.ns.wsdl.ServiceType;
/**
* Implementation of WSDL 2.0 Description container.
*
* @author Sun Microsystems, Inc.
*/
final class DescriptionImpl extends Description
{
/** The base URI for the document this wsdl:definitions component came from */
private String mDocumentBaseUri;
/** The document bean for this wsdl:definitions component */
private DescriptionDocument mDocBean;
/**
* Construct a definitions component implementation object from the given
* XML beans.
*
* @param docBean The Description XML bean to use to construct this
* component.
* @param documentBaseUri The base URI for the document this definitions
* component is based on.
*/
private DescriptionImpl(DescriptionDocument docBean, String documentBaseUri)
{
super(docBean.getDescription());
this.mDocumentBaseUri = documentBaseUri;
this.mDocBean = docBean;
}
/**
* Get the document bean for this component.
*
* @return The document bean for this component.
*/
DescriptionDocument getDocBean()
{
return this.mDocBean;
}
/**
* Get the base document URI for this definitions component.
*
* @return The base URI for the document this definitions component is associated
* with.
*/
public String getDocumentBaseUri()
{
return this.mDocumentBaseUri;
}
/**
* Set the base document URI for this wsdl:definitions component.
*
* @param theDocumentBaseUri The base document URI for this component
*/
public void setDocumentBaseUri(String theDocumentBaseUri)
{
if (theDocumentBaseUri != null)
{
this.mDocumentBaseUri = theDocumentBaseUri;
// $TBD -- invalidate all import / include definitions loaded, if they
// use relative location URIs?
}
}
/** Map of WSDL-defined attribute QNames. Keyed by QName.toString value */
private static java.util.Map sWsdlAttributeQNames = null;
/**
* Worker class-method for {@link #getWsdlAttributeNameMap()}.
*
* @return Map of WSDL-defined attribute QNames for this component,
* indexed by QName.toString()
*/
static synchronized java.util.Map getAttributeNameMap()
{
if (sWsdlAttributeQNames == null)
{
sWsdlAttributeQNames = XmlBeansUtil.getAttributesMap(
DescriptionType.type);
}
return sWsdlAttributeQNames;
}
/**
* Get map of WSDL-defined attribute QNames for this component, indexed by
* canonical QName string (see {@link javax.xml.namespace.QName#toString()}.
*
* @return Map of WSDL-defined attribute QNames for this component,
* indexed by QName.toString()
*/
public java.util.Map getWsdlAttributeNameMap()
{
return getAttributeNameMap();
}
/**
* Get namespace for components in this container.
*
* @return Namespace for components in this container.
*/
public String getTargetNamespace()
{
return getBean().getTargetNamespace();
}
/**
* Set namespace for components in this container.
*
* @param theTargetNamespace Namespace for components in this container.
*/
public void setTargetNamespace(String theTargetNamespace)
{
getBean().setTargetNamespace(theTargetNamespace);
}
/**
* Get the number of Types items in types.
*
* @return The number of Types items in types
*/
public int getTypesLength()
{
return getBean().sizeOfTypesArray();
}
/**
* Get types (in schema or whatever) by indexed position.
*
* @param index Indexed position value 0..length-1
* @return Types (in schema or whatever) at given index
* position.
*/
public com.sun.jbi.wsdl2.Types getType(int index)
{
return TypesImpl.Factory.getInstance(
getBean().getTypesArray(index),
this);
}
/**
* Set types (in schema or whatever) by indexed position.
*
* @param index Indexed position value (0..length-1) of the item to set
* @param theType Item to add at position index
.
*/
public void setType(int index, com.sun.jbi.wsdl2.Types theType)
{
getBean().setTypesArray(
index,
theType != null ? ((TypesImpl) theType).getBean()
: null);
}
/**
* Append an item to types (in schema or whatever).
*
* @param theType Item to append to types
*/
public void appendType(com.sun.jbi.wsdl2.Types theType)
{
synchronized (getBean().monitor())
{
getBean().setTypesArray(
getTypesLength(),
theType != null ? ((TypesImpl) theType).getBean()
: null);
}
}
/**
* Remove types (in schema or whatever) by index position.
*
* @param index The index position of the type to remove
* @return The Types removed, if any.
*/
public com.sun.jbi.wsdl2.Types removeType(int index)
{
com.sun.jbi.wsdl2.Types result;
synchronized (getBean().monitor())
{
result = getType(index);
getBean().removeTypes(index);
}
return result;
}
/**
* Get the number of Import items in imports.
*
* @return The number of Import items in imports
*/
public int getImportsLength()
{
return getBean().sizeOfImportArray();
}
/**
* Get definitions imported into this container by indexed position.
*
* @param index Indexed position value 0..length-1
* @return Description imported into this container. at given index
* position.
*/
public com.sun.jbi.wsdl2.Import getImport(int index)
{
ImportType theImport = getBean().getImportArray(index);
return ImportImpl.Factory.getInstance(theImport, this);
}
/**
* Set definitions imported into this container, by indexed position.
*
* @param index Indexed position value (0..length-1) of the item to set
* @param theImport Item to add at position index
.
*/
public void setImport(int index, com.sun.jbi.wsdl2.Import theImport)
{
getBean().setImportArray(
index,
theImport != null ? ((Import) theImport).getBean() : null);
}
/**
* Append an item to definitions imported into this container.
*
* @param theImport Item to append to imports
*/
public void appendImport(com.sun.jbi.wsdl2.Import theImport)
{
final int nImports = this.getImportsLength();
setImport(nImports, theImport);
}
/**
* Remove definitions imported into this container, by index position.
*
* @param index The index position of the import to remove
* @return The Import removed, if any.
*/
public com.sun.jbi.wsdl2.Import removeImport(int index)
{
com.sun.jbi.wsdl2.Import result = this.getImport(index);
getBean().removeImport(index);
return result;
}
/**
* Get the number of Include items in includes.
*
* @return The number of Include items in includes
*/
public int getIncludesLength()
{
return getBean().sizeOfIncludeArray();
}
/**
* Get definitions included in this container, by indexed position.
*
* @param index Indexed position value 0..length-1
* @return Description included in this container. at given index
* position.
*/
public com.sun.jbi.wsdl2.Include getInclude(int index)
{
IncludeType type = getBean().getIncludeArray(index);
return IncludeImpl.Factory.getInstance(type, this);
}
/**
* Set definitions included in this container, by indexed position.
*
* @param index Indexed position value (0..length-1) of the item to set
* @param theInclude Item to add at position index
.
*/
public void setInclude(int index, com.sun.jbi.wsdl2.Include theInclude)
{
getBean().setIncludeArray(
index,
theInclude != null ? ((IncludeImpl) theInclude).getBean() : null);
}
/**
* Append an item to definitions included in this container.
*
* @param theInclude Item to append to includes
*/
public void appendInclude(com.sun.jbi.wsdl2.Include theInclude)
{
final int length = getIncludesLength();
setInclude(length, theInclude);
}
/**
* Remove definitions included in this container, by index position.
*
* @param index The index position of the include to remove
* @return The Include removed, if any.
*/
public com.sun.jbi.wsdl2.Include removeInclude(int index)
{
com.sun.jbi.wsdl2.Include result = getInclude(index);
getBean().removeInclude(index);
return result;
}
/**
* Get the number of Interface items in interfaces.
*
* @return The number of Interface items in interfaces
*/
public int getInterfacesLength()
{
return getBean().sizeOfInterfaceArray();
}
/**
* Get interfaces for this component, by indexed position.
*
* @param index Indexed position value 0..length-1
* @return Interfaces for this component. at given index
position.
*/
public com.sun.jbi.wsdl2.Interface getInterface(int index)
{
return InterfaceImpl.Factory.getInstance(
getBean().getInterfaceArray(index),
this);
}
/**
* Set interfaces for this component, by indexed position.
*
* @param index Indexed position value (0..length-1) of the item to set
* @param theInterface Item to add at position index
.
*/
public void setInterface(
int index,
com.sun.jbi.wsdl2.Interface theInterface)
{
getBean().setInterfaceArray(
index,
theInterface != null ? ((Interface) theInterface).getBean() : null);
}
/**
* Append an item to interfaces for this component.
*
* @param theInterface Item to append to interfaces
*/
public void appendInterface(com.sun.jbi.wsdl2.Interface theInterface)
{
final int length = getInterfacesLength();
setInterface(length, theInterface);
}
/**
* Remove interfaces for this component, by index position.
*
* @param index The index position of the interface to remove
* @return The Interface removed, if any.
*/
public com.sun.jbi.wsdl2.Interface removeInterface(int index)
{
com.sun.jbi.wsdl2.Interface result = getInterface(index);
getBean().removeInterface(index);
return result;
}
/**
* Get the number of Binding items in bindings.
*
* @return The number of Binding items in bindings
*/
public int getBindingsLength()
{
return getBean().sizeOfBindingArray();
}
/**
* Get bindings for this component, by indexed position.
*
* @param index Indexed position value 0..length-1
* @return Bindings for this component. at given index
position.
*/
public com.sun.jbi.wsdl2.Binding getBinding(int index)
{
return BindingImpl.Factory.getInstance(
getBean().getBindingArray(index),
this);
}
/**
* Set bindings for this component, by indexed position.
*
* @param index Indexed position value (0..length-1) of the item to set
* @param theBinding Item to add at position index
.
*/
public void setBinding(int index, com.sun.jbi.wsdl2.Binding theBinding)
{
getBean().setBindingArray(
index,
theBinding != null ? ((BindingImpl) theBinding).getBean() : null);
}
/**
* Append an item to bindings for this component..
*
* @param theBinding Item to append to bindings
*/
public void appendBinding(com.sun.jbi.wsdl2.Binding theBinding)
{
final int length = getBindingsLength();
setBinding(length, theBinding);
}
/**
* Remove bindings for this component, by index position.
*
* @param index The index position of the binding to remove
* @return The Binding removed, if any.
*/
public com.sun.jbi.wsdl2.Binding removeBinding(int index)
{
com.sun.jbi.wsdl2.Binding result = getBinding(index);
getBean().removeBinding(index);
return result;
}
/**
* Get the number of Service items in services.
*
* @return The number of Service items in services
*/
public int getServicesLength()
{
return getBean().sizeOfServiceArray();
}
/**
* Get services for this component, by indexed position.
*
* @param index Indexed position value 0..length-1
* @return Services for this component. at given index
position.
*/
public com.sun.jbi.wsdl2.Service getService(int index)
{
return ServiceImpl.Factory.getInstance(
getBean().getServiceArray(index),
this);
}
/**
* Set services for this component, by indexed position.
*
* @param index Indexed position value (0..length-1) of the item to set
* @param theService Item to add at position index
.
*/
public void setService(int index, com.sun.jbi.wsdl2.Service theService)
{
getBean().setServiceArray(
index,
theService != null ? ((ServiceImpl) theService).getBean() : null);
}
/**
* Append an item to services for this component..
*
* @param theService Item to append to services
*/
public void appendService(com.sun.jbi.wsdl2.Service theService)
{
final int length = getServicesLength();
setService(length, theService);
}
/**
* Remove services for this component, by index position.
*
* @param index The index position of the service to remove
* @return The Service removed, if any.
*/
public com.sun.jbi.wsdl2.Service removeService(int index)
{
com.sun.jbi.wsdl2.Service result = getService(index);
getBean().removeService(index);
return result;
}
/**
* Find named binding in this definition or the imported/included bindings.
*
* This implementation assumes that the import/include structure does not
* introduce a circular search path.
*
* @param name Name of binding to find.
* @return Named Binding; null if none found.
*/
public com.sun.jbi.wsdl2.Binding findBinding(QName name)
{
com.sun.jbi.wsdl2.Binding result = null;
if (name != null)
{
String namespace = name.getNamespaceURI();
if (getTargetNamespace().equals(namespace))
{
result = findLocalBinding(name.getLocalPart());
}
if (result == null && namespace == null)
{
result = findIncludedBinding(name);
}
if (result == null && namespace == null)
{
result = findImportedBinding(name);
}
}
return result;
}
/**
* Find binding with given local name in the bindings from this definition
* only.
*
* @param localName Local name of the binding to search for.
* @return Binding found matching the local name given; null if not found.
*/
protected com.sun.jbi.wsdl2.Binding findLocalBinding(String localName)
{
com.sun.jbi.wsdl2.Binding result = null;
BindingType[] bindings = getBean().getBindingArray();
for (BindingType type : bindings) {
if (type != null && localName.equals(type.getName()))
{
result = BindingImpl.Factory.getInstance(type, this);
}
}
return result;
}
/**
* Find binding with given qualified name in the bindings included in
* this defintions component.
*
* @param name Qualified name of binding to look for
* @return Binding with given qualified name; null if not found
*/
protected com.sun.jbi.wsdl2.Binding findIncludedBinding(QName name)
{
com.sun.jbi.wsdl2.Binding result = null;
IncludeType[] imports = getBean().getIncludeArray();
final int nIncludes = imports != null ? imports.length : 0;
String tns = name.getNamespaceURI();
for (int idx = 0; result == null && idx < nIncludes; idx++)
{
IncludeType include = imports[idx];
IncludeImpl includeImpl = IncludeImpl.Factory.getInstance(include, this);
if (includeImpl != null)
{
DescriptionImpl defs = (DescriptionImpl) includeImpl.getDescription();
result = defs != null ? defs.findBinding(name) : null;
}
}
return result;
}
/**
* Find binding with given qualified name in the bindings imported in
* this definitions component.
*
* @param name Qualified name of binding to look for
* @return Binding with given qualified name; null if not found
*/
protected com.sun.jbi.wsdl2.Binding findImportedBinding(QName name)
{
com.sun.jbi.wsdl2.Binding result = null;
ImportType[] imports = getBean().getImportArray();
final int nImports = imports != null ? imports.length : 0;
String tns = name.getNamespaceURI();
for (int idx = 0; result == null && idx < nImports; idx++)
{
ImportType imp = imports[idx];
ImportImpl impImpl = ImportImpl.Factory.getInstance(imp, this);
if (impImpl != null && tns.equals(imp.getNamespace()))
{
DescriptionImpl defs = (DescriptionImpl) impImpl.getDescription();
result = defs != null ? defs.findBinding(name) : null;
}
}
return result;
}
/**
* Find named interface in this definition or the imported/included interfaces.
*
* @param name Name of interface to find.
* @return Named Interface; null if none found.
*/
public com.sun.jbi.wsdl2.Interface findInterface(QName name)
{
com.sun.jbi.wsdl2.Interface result = null;
if (name != null)
{
String namespace = name.getNamespaceURI();
if (getTargetNamespace().equals(namespace))
{
result = findLocalInterface(name.getLocalPart());
}
if (result == null && namespace != null)
{
result = findIncludedInterface(name);
}
if (result == null && namespace != null)
{
result = findImportedInterface(name);
}
}
return result;
}
/**
* Find interface with given local name in the interfaces from this definition
* only.
*
* @param localName Local name of the interface to search for.
* @return Interface found matching the local name given; null if not found.
*/
protected com.sun.jbi.wsdl2.Interface findLocalInterface(String localName)
{
com.sun.jbi.wsdl2.Interface result = null;
InterfaceType[] interfaces = getBean().getInterfaceArray();
for (InterfaceType type : interfaces) {
if (type != null && localName.equals(type.getName()))
{
result = InterfaceImpl.Factory.getInstance(type, this);
}
}
return result;
}
/**
* Find interface with given qualified name in the interfaces included in
* this defintions component.
*
* @param name Qualified name of interface to look for
* @return Interface with given qualified name; null if not found
*/
protected com.sun.jbi.wsdl2.Interface findIncludedInterface(QName name)
{
com.sun.jbi.wsdl2.Interface result = null;
IncludeType[] imports = getBean().getIncludeArray();
final int nIncludes = imports != null ? imports.length : 0;
String tns = name.getNamespaceURI();
for (int idx = 0; result == null && idx < nIncludes; idx++)
{
IncludeType include = imports[idx];
IncludeImpl includeImpl = IncludeImpl.Factory.getInstance(include, this);
if (includeImpl != null)
{
DescriptionImpl defs = (DescriptionImpl) includeImpl.getDescription();
result = defs != null ? defs.findInterface(name) : null;
}
}
return result;
}
/**
* Find interface with given qualified name in the interfaces imported in
* this definitions component.
*
* @param name Qualified name of interface to look for
* @return Interface with given qualified name; null if not found
*/
protected com.sun.jbi.wsdl2.Interface findImportedInterface(QName name)
{
com.sun.jbi.wsdl2.Interface result = null;
ImportType[] imports = getBean().getImportArray();
final int nImports = imports != null ? imports.length : 0;
String tns = name.getNamespaceURI();
for (int idx = 0; result == null && idx < nImports; idx++)
{
ImportType imp = imports[idx];
ImportImpl impImpl = ImportImpl.Factory.getInstance(imp, this);
if (impImpl != null && tns.equals(imp.getNamespace()))
{
DescriptionImpl defs = (DescriptionImpl) impImpl.getDescription();
result = defs != null ? defs.findInterface(name) : null;
}
}
return result;
}
/**
* Find named service in this definition or the imported/included services.
*
* @param name Name of service to find.
* @return Named Service; null if none found.
*/
public com.sun.jbi.wsdl2.Service findService(QName name)
{
com.sun.jbi.wsdl2.Service result = null;
if (name != null)
{
String namespace = name.getNamespaceURI();
if (getTargetNamespace().equals(namespace))
{
result = findLocalService(name.getLocalPart());
}
if (result == null && namespace != null)
{
result = findIncludedService(name);
}
if (result == null && namespace != null)
{
result = findImportedService(name);
}
}
return result;
}
/**
* Find interface with given local name in the interfaces from this definition
* only.
*
* @param localName Local name of the interface to search for.
* @return Service found matching the local name given; null if not found.
*/
protected com.sun.jbi.wsdl2.Service findLocalService(String localName)
{
com.sun.jbi.wsdl2.Service result = null;
ServiceType[] interfaces = getBean().getServiceArray();
for (ServiceType type : interfaces) {
if (type != null && localName.equals(type.getName()))
{
result = ServiceImpl.Factory.getInstance(type, this);
}
}
return result;
}
/**
* Find interface with given qualified name in the interfaces included in
* this defintions component.
*
* @param name Qualified name of interface to look for
* @return Service with given qualified name; null if not found
*/
protected com.sun.jbi.wsdl2.Service findIncludedService(QName name)
{
com.sun.jbi.wsdl2.Service result = null;
IncludeType[] imports = getBean().getIncludeArray();
final int nIncludes = imports != null ? imports.length : 0;
String tns = name.getNamespaceURI();
for (int idx = 0; result == null && idx < nIncludes; idx++)
{
IncludeType include = imports[idx];
IncludeImpl includeImpl = IncludeImpl.Factory.getInstance(include, this);
if (includeImpl != null)
{
DescriptionImpl defs = (DescriptionImpl) includeImpl.getDescription();
result = defs != null ? defs.findService(name) : null;
}
}
return result;
}
/**
* Find an interface with given qualified name in the interfaces imported in
* this definitions component.
*
* @param name Qualified name of interface to look for
* @return Service with given qualified name; null if not found
*/
protected com.sun.jbi.wsdl2.Service findImportedService(QName name)
{
com.sun.jbi.wsdl2.Service result = null;
ImportType[] imports = getBean().getImportArray();
final int nImports = imports != null ? imports.length : 0;
String tns = name.getNamespaceURI();
for (int idx = 0; result == null && idx < nImports; idx++)
{
ImportType imp = imports[idx];
ImportImpl impImpl = ImportImpl.Factory.getInstance(imp, this);
if (impImpl != null && tns.equals(imp.getNamespace()))
{
DescriptionImpl defs = (DescriptionImpl) impImpl.getDescription();
result = defs != null ? defs.findService(name) : null;
}
}
return result;
}
/**
* Create a new binding component, appended to this definition's binding list.
*
* @param name Name of binding to create.
* @return Newly created binding, appended to the bindings list.
*/
public com.sun.jbi.wsdl2.Binding addNewBinding(String name)
{
BindingType bindingBean = getBean().addNewBinding();
bindingBean.setName(name);
return BindingImpl.Factory.getInstance(bindingBean, this);
}
/**
* Create a new import component, appended to this definition's import list.
*
* @return Newly created import, appended to the imports list.
*/
public com.sun.jbi.wsdl2.Import addNewImport()
{
return ImportImpl.Factory.getInstance(
getBean().addNewImport(),
this);
}
/**
* Create a new include, appended to this definition's include list.
*
* @return Newly created include, appended to the includes list.
*/
public com.sun.jbi.wsdl2.Include addNewInclude()
{
return IncludeImpl.Factory.getInstance(
getBean().addNewInclude(),
this);
}
/**
* Create a new interface component, appended to this definition's interface list.
*
* @param name Name of interface to create.
* @return Newly created interface, appended to interfaces list.
*/
public com.sun.jbi.wsdl2.Interface addNewInterface(String name)
{
InterfaceType ifaceBean = getBean().addNewInterface();
ifaceBean.setName(name);
return InterfaceImpl.Factory.getInstance(ifaceBean, this);
}
/**
* Create a new service component, appended to this definition's service list.
*
* @param name Name of service to create.
* @return Newly created service, appended to the services list.
*/
public com.sun.jbi.wsdl2.Service addNewService(String name)
{
ServiceType serviceBean = getBean().addNewService();
serviceBean.setName(name);
return ServiceImpl.Factory.getInstance(serviceBean, this);
}
/**
* Create a new types component, replacing the existing types component of
* this definition, if necessary.
*
* @return Newly created Types component.
*/
public com.sun.jbi.wsdl2.Types newTypes()
{
return TypesImpl.Factory.getInstance(
getBean().addNewTypes(),
this);
}
/*
* The following maps are used to map XML Bean instances (of various types)
* to component objects. These maps are used by the component factories.
*/
/** Map of known BindingType beans to Binding components */
private final Map mBindingMap = new HashMap();
/**
* Get the BindingType to BindingImpl object map for this definitions
* component.
*
* @return The binding map for this definition.
*/
public Map getBindingMap()
{
return this.mBindingMap;
}
/** Map of known BindingFaultType beans to BindingFault components */
private final Map mBindingFaultMap = new HashMap();
/**
* Get the BindingFaultType to BindingFaultImpl object map for this
* definitions component.
*
* @return The binding fault map for this definition.
*/
public Map getBindingFaultMap()
{
return this.mBindingFaultMap;
}
/**
* Map of known BindingFaultType beans to BindingFaultReference
* components
*/
private final Map mBindingFaultReferenceMap = new HashMap();
/**
* Get the BindingFaultType to BindingFaultReferenceImpl map
* for this definitions component.
*
* @return The Binding Fault Reference map for this definition.
*/
public Map getBindingFaultReferenceMap()
{
return this.mBindingFaultReferenceMap;
}
/**
* Map of known BindingOperationFaultType beans to BindingOperationFault
* components
*/
private final Map mBindingOperationFaultMap = new HashMap();
/**
* Get the BindingOperationFault to BindingOperationFaultImpl map
* for this definitions component.
*
* @return The Binding Operation Fault map for this definition.
*/
public Map getBindingOperationFaultMap()
{
return this.mBindingOperationFaultMap;
}
/**
* Map of known BindingOperationMessageType beans to
* BindingMessageReferenceImpl components.
*/
private final Map mBindingMessageReferenceMap = new HashMap();
/**
* Get the BindingOperationMessageType to BindingMessageReferenceImpl
* map for this definitions component.
*
* @return The Binding Operation Message Type map for this definition.
*/
public Map getBindingMessageReferenceMap()
{
return this.mBindingMessageReferenceMap;
}
/**
* Map of known BindingOperationType beans to BindingOperationImpl
* components
*/
private final Map mBindingOperationMap = new HashMap();
/**
* Get the BindingOperationType to BindingOperationImpl map for this
* definitions component.
*
* @return The Binding Operation map for this component.
*/
public Map getBindingOperationMap()
{
return this.mBindingOperationMap;
}
/** Map of known DocumentationType beans to DocumentImpl components */
private final Map mDocumentMap = new HashMap();
/**
* Get the DocumentationType to DocumentImpl map for this definitions
* component.
*
* @return The Document map for this component.
*/
public Map getDocumentMap()
{
return this.mDocumentMap;
}
/** Map of known EndpointType beans to EndpointImpl components */
private final Map mEndpointMap = new HashMap();
/**
* Get the EndpointType to EndpointImpl map for this definitions
* component.
*
* @return The Endpoint map for this component.
*/
public Map getEndpointMap()
{
return this.mEndpointMap;
}
/** Map of known ImportType beans to ImportImpl components */
private final Map mImportMap = new HashMap();
/**
* Get the ImportType to ImportImpl map for this definitions
* component.
*
* @return The Import map for this component.
*/
public Map getImportMap()
{
return this.mImportMap;
}
/** Map of known IncludeType beans to IncludeImpl components */
private final Map mIncludeMap = new HashMap();
/**
* Get the IncludeType to IncludeImpl map for this definitions
* component.
*
* @return The Include map for this component.
*/
public Map getIncludeMap()
{
return this.mIncludeMap;
}
/** Map of known InterfaceType beans to InterfaceImpl components */
private final Map mInterfaceMap = new HashMap();
/**
* Get the InterfaceType to InterfaceImpl map for this component.
*
* @return The Interface map for this component.
*/
public Map getInterfaceMap()
{
return this.mInterfaceMap;
}
/** Map of known InterfaceFaultType beans to InterfaceFault components */
private final Map mInterfaceFaultMap = new HashMap();
/**
* Get the InterfaceFaultType to InterfaceFaultImpl object map for this
* definitions component.
*
* @return The interface fault map for this definition.
*/
public Map getInterfaceFaultMap()
{
return this.mInterfaceFaultMap;
}
/**
* Map of known InterfaceOperationType beans to InterfaceOperationImpl
* components
*
*/
private final Map mInterfaceOperationMap = new HashMap();
/**
* Get the InterfaceOperationType to InterfaceOperationImpl map for
* this component.
*
* @return The Interface Operation map for this component.
*/
public Map getInterfaceOperationMap()
{
return this.mInterfaceOperationMap;
}
/**
* Map of known MessageRefFaultType beans to MessageFaultReferenceImpl
* components
*/
private final Map mMessageFaultReferenceMap = new HashMap();
/**
* Get the MessageRefFaultType to MessageFaultReferenceImpl map for
* this component.
*
* @return The Message Fault Reference map for this compoent.
*/
public Map getMessageFaultReferenceMap()
{
return this.mMessageFaultReferenceMap;
}
/** Map of known MessageRefType beans to MessageReferenceImpl components */
private final Map mMessageReferenceMap = new HashMap();
/**
* Get the MessageRefType to MessageReferenceImpl map for this
* component.
*
* @return The Message Reference map for this component.
*/
public Map getMessageReferenceMap()
{
return this.mMessageReferenceMap;
}
/** Map of known ServiceType beans to ServiceImpl components */
private final Map mServiceMap = new HashMap();
/**
* Get the ServiceType to ServiceImpl map for this component.
*
* @return The Service map for this component.
*/
public Map getServiceMap()
{
return this.mServiceMap;
}
/** Map of known TypesType beans to TypesImpl components */
private final Map mTypesMap = new HashMap();
/**
* Get the TypesType to TypesImpl map for this component.
*
* @return The Types map for this component.
*/
public Map getTypesMap()
{
return this.mTypesMap;
}
/**
* Get the container for this component.
*
* @return The component for this component
*/
protected DescriptionImpl getContainer()
{
return this;
}
/**
* Return this WSDL definition as an XML string.
*
* @return This definition, serialized as an XML string.
*/
public String toXmlString()
{
String result;
StringWriter sw = new StringWriter();
XmlOptions options = new XmlOptions();
options.setSavePrettyPrint();
options.setSavePrettyPrintIndent(Constants.XML_PRETTY_PRINT_INDENT);
options.setSaveOuter();
try
{
getBean().save(sw, options);
sw.close();
}
catch (java.io.IOException ex)
{
sw.write("\n\n");
// $TODO: log error
}
return sw.getBuffer().toString();
}
/**
* Return this document as a DOM document.
*
* @return This definition, as a DOM document.
*/
public org.w3c.dom.Document toXmlDocument()
{
return (org.w3c.dom.Document) getDocBean().newDomNode();
}
/**
* This class serves as the external API for creating (or recalling)
* DescriptionImpl objects. This allows a simple caching mechanism that
* permits us to find the DescriptionImpl object associated with a
* particular XmlBeans WSDL Description object, maintaining a one-to-one
* relationship between the Impl object and the XML bean instance.
*/
static class Factory
{
/**
* The association map between all known DefinitionType objects (the key)
* and the corresponding DescriptionImpl objects. This is a WeakHashMap
* to permit reclamation of the definition by the GC.
*/
private static Map sDescriptionMap = Collections.synchronizedMap(
new WeakHashMap());
/**
* Get (or create if necessary) the instance of DescriptionImpl that is
* associated with the given XML Bean object, type
.
*
* This is thread-safe.
*
* @param docBean The XML Bean object to get the definitions
* component of.
* @param documentBaseUri The base URI to be associated with the result when
* handled as a document.
* @return The definitions component implementation object for
* the bean
supplied.
*/
static DescriptionImpl getInstance(
DescriptionDocument docBean,
String documentBaseUri)
{
DescriptionImpl result = null;
if (docBean != null)
{ //
// Since the values we put in the WeakHashMap have strong
// references to the key, we must wrap the value in a weak
// reference to allow the GC to reclaim old definitions out of
// our cache.
//
synchronized (sDescriptionMap)
{
WeakReference ref = (WeakReference) sDescriptionMap.get(docBean);
if (ref != null)
{
result = (DescriptionImpl) ref.get();
}
if (result == null)
{
result = new DescriptionImpl(docBean, documentBaseUri);
sDescriptionMap.put(docBean, new WeakReference(result));
}
}
}
return result;
}
/**
* Create a new definitions component implementation. This is for the
* programmatic creation of WSDL.
*
* @param documentBaseUri The base URI to be associated with the result when
* handled as a document.
* @return A new definitions component implementation object.
*/
static DescriptionImpl newInstance(String documentBaseUri)
{
DescriptionDocument docBean = DescriptionDocument.Factory.newInstance();
DescriptionType bean = docBean.addNewDescription();
return getInstance(docBean, documentBaseUri);
}
} // end inner class Factory
}
// End-of-file: DescriptionImpl.java