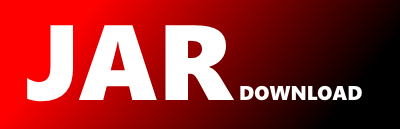
com.sun.jbi.wsdl2.impl.DocumentImpl Maven / Gradle / Ivy
/*
* BEGIN_HEADER - DO NOT EDIT
*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the "License"). You may not use this file except
* in compliance with the License.
*
* You can obtain a copy of the license at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* HEADER in each file and include the License file at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* If applicable add the following below this CDDL HEADER,
* with the fields enclosed by brackets "[]" replaced with
* your own identifying information: Portions Copyright
* [year] [name of copyright owner]
*/
/*
* @(#)DocumentImpl.java
* Copyright 2004-2007 Sun Microsystems, Inc. All Rights Reserved.
*
* END_HEADER - DO NOT EDIT
*/
package com.sun.jbi.wsdl2.impl;
import java.util.Map;
import java.util.List;
import java.util.ArrayList;
import org.apache.xmlbeans.XmlException;
import org.apache.xmlbeans.XmlOptions;
import org.w3.ns.wsdl.DocumentationType;
import org.w3c.dom.DocumentFragment;
import org.w3c.dom.Element;
/**
* Implementation of Documentation for a WSDL, component.
*
* @author Sun Microsystems, Inc.
*/
final class DocumentImpl extends Document
{
/** Documentation Xml bean. */
private DocumentationType mBean;
/** The component which is documented by this component. */
private ExtensibleDocumentedComponent mParent;
/** Container for this component */
private DescriptionImpl mContainer;
/**
* Fetch the Xml bean for this component.
*
* @return The XML Bean for this documentation component.
*/
DocumentationType getBean()
{
return this.mBean;
}
/**
* Construct a Document component from the given XmlBean.
*
* @param bean The XmlBean for this document component.
* @param parent The parent for which this component serves as
* documentation.
* @param defs The container for this component.
*/
private DocumentImpl(
DocumentationType bean,
ExtensibleDocumentedComponent parent,
DescriptionImpl defs)
{
this.mBean = bean;
this.mParent = parent;
this.mContainer = defs;
}
/**
* Get DOM document fragment containing documentation.
*
* @return DOM document fragment containing documentation
*/
public DocumentFragment getDocumentation()
{
XmlOptions opts = new XmlOptions()
.setSaveOuter()
.setSaveAggresiveNamespaces();
DocumentFragment result = (DocumentFragment) getBean().newDomNode(opts);
return result;
}
/**
* Set DOM element containing documentation.
*
* @param theDocumentFragment DOM element containing documentation
*/
public void setDocumentation(
DocumentFragment theDocumentFragment)
{
if (theDocumentFragment != null)
{
DocumentationType newBean = null;
try
{
newBean = DocumentationType.Factory.parse(theDocumentFragment);
}
catch (XmlException ex)
{
; // Keep checkstyle happy.
// Retry after wrapping the fragment in a documentation element
// (below).
}
if (newBean == null)
{
// Try wrapping in a wsdl documentation element.
org.w3c.dom.Document doc = theDocumentFragment.getOwnerDocument();
Element elm = doc.createElementNS(
Constants.WSDL_NAMESPACE_NAME,
"documentation");
elm.appendChild(theDocumentFragment);
try
{
newBean = DocumentationType.Factory.parse(elm);
}
catch (XmlException ex)
{
System.err.println( "Error parsing documentation fragment" );
System.err.println( ex.getMessage() );
System.err.println( ex.getError().toString() );
ex.printStackTrace(System.err);
}
}
if (newBean != null)
{
DocumentationType oldBean = getBean();
Map map = mContainer.getDocumentMap();
synchronized (map)
{
map.put(newBean, map.remove(oldBean));
DocumentationType [] docTypeArray = mParent.getExBean().getDocumentationArray();
ArrayList theList = new ArrayList();
if (docTypeArray != null)
{
for (int i=0; i < docTypeArray.length; ++i)
{
theList.add(i, docTypeArray[i]);
}
}
int theIndex = theList.indexOf(oldBean);
if (theIndex != -1)
theList.set(theIndex, newBean);
else
theList.add(newBean);
mParent.getExBean().setDocumentationArray((DocumentationType[]) theList.toArray());
}
}
}
return; // $$TODO -- need to verify insertion point of theDocumentFragment
}
/**
* A factory class for creating / finding components for given XML beans.
*
* This factory guarantees that there will only be one component for each
* XML bean instance.
*/
static class Factory
{
/**
* Find the WSDL documentation component associated with the given XML
* bean, creating a new component if necessary.
*
* This is thread-safe.
*
* @param bean The XML bean to find the component for.
* @param parent The parent for which this component serves as
* documentation.
* @param defs The container for the component.
* @return The WSDL documentation component for the given bean
* (null if the bean
is null).
*/
static DocumentImpl getInstance(
DocumentationType bean,
ExtensibleDocumentedComponent parent,
DescriptionImpl defs)
{
DocumentImpl result;
if (bean != null)
{
Map map = defs.getDocumentMap();
synchronized (map)
{
result = (DocumentImpl) map.get(bean);
if (result == null)
{
result = new DocumentImpl(bean, parent, defs);
map.put(bean, result);
}
}
}
else
{
result = null;
}
return result;
}
}
}
// End-of-file: DocumentImpl.java