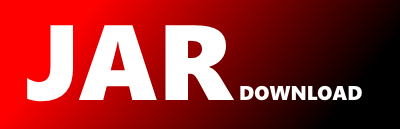
com.sun.jbi.wsdl2.impl.IncludeImpl Maven / Gradle / Ivy
/*
* BEGIN_HEADER - DO NOT EDIT
*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the "License"). You may not use this file except
* in compliance with the License.
*
* You can obtain a copy of the license at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* HEADER in each file and include the License file at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* If applicable add the following below this CDDL HEADER,
* with the fields enclosed by brackets "[]" replaced with
* your own identifying information: Portions Copyright
* [year] [name of copyright owner]
*/
/*
* @(#)IncludeImpl.java
* Copyright 2004-2007 Sun Microsystems, Inc. All Rights Reserved.
*
* END_HEADER - DO NOT EDIT
*/
package com.sun.jbi.wsdl2.impl;
import com.sun.jbi.wsdl2.WsdlException;
import java.io.IOException;
import java.util.Map;
import org.w3.ns.wsdl.IncludeType;
/**
* Implementation of WSDL 2.0 Include component.
*
* @author Sun Microsystems, Inc.
*/
final class IncludeImpl extends Include
{
/** The container for this import component */
private DescriptionImpl mContainer;
/** The result of reading this component */
private DescriptionImpl mIncludeResult = null;
/**
* Get the container for this component.
*
* @return The component for this component
*/
protected DescriptionImpl getContainer()
{
return this.mContainer;
}
/**
* Construct an Include component implementation from an Include XML Bean.
* @param bean The Include XML bean to use to construct this component.
* @param defs The container for this component.
*/
private IncludeImpl(IncludeType bean, DescriptionImpl defs)
{
super(bean);
this.mContainer = defs;
}
/** Map of WSDL-defined attribute QNames. Keyed by QName.toString value */
private static java.util.Map sWsdlAttributeQNames = null;
/**
* Worker class method for {@link #getWsdlAttributeNameMap()}.
*
* @return Map of WSDL-defined attribute QNames for this component,
* indexed by QName.toString()
*/
static synchronized java.util.Map getAttributeNameMap()
{
if (sWsdlAttributeQNames == null)
{
sWsdlAttributeQNames = XmlBeansUtil.getAttributesMap(
IncludeType.type);
}
return sWsdlAttributeQNames;
}
/**
* Get map of WSDL-defined attribute QNames for this component, indexed by
* canonical QName string (see {@link javax.xml.namespace.QName#toString()}.
*
* @return Map of WSDL-defined attribute QNames for this component,
* indexed by QName.toString()
*/
public java.util.Map getWsdlAttributeNameMap()
{
return getAttributeNameMap();
}
/**
* Get location hint for the included definitions.
*
* @return Location hint for the included definitions
*/
public String getLocation()
{
return getBean().getLocation();
}
/**
* Set location hint for the included definitions.
*
* @param theLocation Location hint for the included definitions
*/
public synchronized void setLocation(String theLocation)
{
getBean().setLocation(theLocation);
this.mIncludeResult = null;
}
/**
* Get the description included by this component
*
* @return Description from included by this component, if any
*/
public synchronized com.sun.jbi.wsdl2.Description getDescription()
{
if (this.mIncludeResult == null)
{
this.mIncludeResult = includeTarget();
}
return this.mIncludeResult;
}
/**
* Get the definitions included by this component
*
* @deprecated - replaced by getDescription
* @return Definitions from included by this component, if any
*/
public com.sun.jbi.wsdl2.Definitions getDefinitions()
{
return (com.sun.jbi.wsdl2.Definitions) getDescription();
}
/**
* Read the target of this include into its own Description component.
*
* @return The WSDL definitions component pointed to by this include.
*/
private DescriptionImpl includeTarget()
{
WsdlReader reader = new WsdlReader();
com.sun.jbi.wsdl2.Description defs = null;
try
{
defs = reader.readDescription(
mContainer.getDocumentBaseUri(),
getLocation());
}
catch (IOException ex)
{
System.err.println("WSDL include IO error reading " +
getLocation() + " (relative to) " +
mContainer.getDocumentBaseUri() + ":" );
if (ex.getMessage() != null)
{
System.err.println(ex.getMessage());
}
ex.printStackTrace(System.err);
}
catch (WsdlException ex)
{
System.err.println("WSDL include error reading " +
getLocation() + " (relative to) " +
mContainer.getDocumentBaseUri() + ":" );
if (ex.getMessage() != null)
{
System.err.println(ex.getMessage());
}
ex.printStackTrace(System.err);
}
return defs != null ? (DescriptionImpl) defs : null;
}
/**
* A factory class for creating / finding components for given XML beans.
*
* This factory guarantees that there will only be one component for each
* XML bean instance.
*/
static class Factory
{
/**
* Find the WSDL include component associated with the given XML
* bean, creating a new component if necessary.
*
* This is thread-safe.
*
* @param bean The XML bean to find the component for.
* @param defs The container for the component.
* @return The WSDL include component for the given bean
* (null if the bean
is null).
*/
static IncludeImpl getInstance(IncludeType bean, DescriptionImpl defs)
{
IncludeImpl result;
if (bean != null)
{
Map map = defs.getIncludeMap();
synchronized (map)
{
result = (IncludeImpl) map.get(bean);
if (result == null)
{
result = new IncludeImpl(bean, defs);
map.put(bean, result);
}
}
}
else
{
result = null;
}
return result;
}
}
}
// End-of-file: IncludeImpl.java