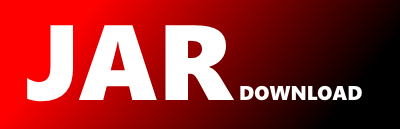
com.sun.jbi.wsdl2.impl.InterfaceImpl Maven / Gradle / Ivy
/*
* BEGIN_HEADER - DO NOT EDIT
*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the "License"). You may not use this file except
* in compliance with the License.
*
* You can obtain a copy of the license at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* HEADER in each file and include the License file at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* If applicable add the following below this CDDL HEADER,
* with the fields enclosed by brackets "[]" replaced with
* your own identifying information: Portions Copyright
* [year] [name of copyright owner]
*/
/*
* @(#)InterfaceImpl.java
* Copyright 2004-2007 Sun Microsystems, Inc. All Rights Reserved.
*
* END_HEADER - DO NOT EDIT
*/
package com.sun.jbi.wsdl2.impl;
import java.net.URI;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import javax.xml.namespace.QName;
import org.w3.ns.wsdl.InterfaceType;
/**
* Implementation of WSDL 2.0 Interface Component.
*
* @author Sun Microsystems, Inc.
*/
final class InterfaceImpl extends Interface
{
/** The container for this component */
private DescriptionImpl mContainer;
/**
* Get the container for this component.
*
* @return The component for this component
*/
protected DescriptionImpl getContainer()
{
return this.mContainer;
}
/**
* Construct an Interface component implementation from an Interface XML bean.
* @param bean The XML bean to construct the component from.
* @param defs The container for this component.
*/
private InterfaceImpl(InterfaceType bean, DescriptionImpl defs)
{
super(bean);
this.mContainer = defs;
}
/** Map of WSDL-defined attribute QNames. Keyed by QName.toString value */
private static java.util.Map sWsdlAttributeQNames = null;
/**
* Worker class method for {@link #getWsdlAttributeNameMap()}.
*
* @return Map of WSDL-defined attribute QNames for this component,
* indexed by QName.toString()
*/
static synchronized java.util.Map getAttributeNameMap()
{
if (sWsdlAttributeQNames == null)
{
sWsdlAttributeQNames = XmlBeansUtil.getAttributesMap(
InterfaceType.type);
}
return sWsdlAttributeQNames;
}
/**
* Get map of WSDL-defined attribute QNames for this component, indexed by
* canonical QName string (see {@link javax.xml.namespace.QName#toString()}.
*
* @return Map of WSDL-defined attribute QNames for this component,
* indexed by QName.toString()
*/
public java.util.Map getWsdlAttributeNameMap()
{
return getAttributeNameMap();
}
/**
* Get the target namespace of this interface's.
*
* @return The target namespace of this interface's container.
*/
public String getTargetNamespace()
{
return this.mContainer.getTargetNamespace();
}
/**
* Get the qualified name of this binding component.
*
* @return The qualified name of this binding component.
*/
public QName getQName()
{
return new QName(this.mContainer.getTargetNamespace(), getName());
}
/**
* Get local name of this interface component.
*
* @return Local name of this interface component
*/
public String getName()
{
return getBean().getName();
}
/**
* Set local name of this interface component.
*
* @param theName Local name of this interface component
*/
public void setName(String theName)
{
getBean().setName(theName);
}
/**
* Get the number of Interface items in interfaces.
*
* @return The number of Interface items in interfaces
*/
public int getInterfacesLength()
{
List extendsList = getBean().getExtends();
return extendsList != null ? extendsList.size() : 0;
}
/**
* Get interfaces this interface subclasses, if any by indexed position.
*
* @param index Indexed position value 0..length-1
* @return Interfaces this interface subclasses, if any at given
* index
position.
*/
public com.sun.jbi.wsdl2.Interface getInterface(int index)
{
com.sun.jbi.wsdl2.Interface result = null;
List extendsList = getBean().getExtends();
if (extendsList != null && index < extendsList.size())
{
QName ifaceName = (QName) extendsList.get(index);
result = this.mContainer.findInterface(ifaceName);
}
return result;
}
/**
* Set interfaces this interface subclasses, if any by indexed position.
*
* @param index Indexed position value (0..length-1) of the item to set.
* @param theInterface Item to add at position index
.
*/
public void setInterface(
int index,
com.sun.jbi.wsdl2.Interface theInterface)
{
synchronized (getBean().monitor())
{
List extendsList = getBean().getExtends();
extendsList.set(
index,
theInterface != null ? theInterface.getQName() : null);
getBean().setExtends(extendsList);
}
}
/**
* Append an item to interfaces this interface subclasses, if any.
*
* @param theInterface Item to append to interfaces
*/
public void appendInterface(
com.sun.jbi.wsdl2.Interface theInterface)
{
synchronized (getBean().monitor())
{
List extendsList = getBean().getExtends();
extendsList.set(
extendsList.size(),
theInterface != null ? theInterface.getQName() : null);
getBean().setExtends(extendsList);
}
}
/**
* Remove interfaces this interface subclasses, if any by index position.
*
* @param index The index position of the interface to remove
* @return The Interface removed, if any.
*/
public com.sun.jbi.wsdl2.Interface removeInterface(int index)
{
com.sun.jbi.wsdl2.Interface result = null;
List extendsList;
QName ifaceName;
synchronized (getBean().monitor())
{
extendsList = getBean().getExtends();
ifaceName = (QName) extendsList.remove(index);
}
if (ifaceName != null)
{
result = this.mContainer.findInterface(ifaceName);
}
return result;
}
/**
* Get the default style for the message prop of operations contained in
* this interface.
*
* @return The default style for the message prop of operations contained
* in this interface
*/
public java.net.URI[] getStyleDefault()
{
List list = getBean().getStyleDefault();
URI[] result = new URI[list != null ? list.size() : 0];
for (int i = 0; i < result.length; i++)
{
result[i] = (URI) list.get(i);
}
return result;
}
/**
* Set the default style for the message prop of operations contained
* in this interface.
*
* @param theStyleDefault The default styles for the message prop of
* operations contained in this interface.
*/
public void setStyleDefault(java.net.URI[] theStyleDefault)
{
List list = new ArrayList();
if (theStyleDefault != null)
{
for (URI theStyleDefault1 : theStyleDefault) {
list.add(theStyleDefault1);
}
}
}
/**
* Get the number of InterfaceFault items in faults.
*
* @return The number of InterfaceFault items in faults
*/
public int getFaultsLength()
{
return getBean().sizeOfFaultArray();
}
/**
* Get faults that may occur executing operations of this interface by
* indexed position.
*
* @param index Indexed position value 0..length-1
* @return Faults that may occur executing operations of this interface
* at given index
position.
*/
public com.sun.jbi.wsdl2.InterfaceFault getFault(int index)
{
return InterfaceFaultImpl.Factory.getInstance(
getBean().getFaultArray(index),
this.mContainer);
}
/**
* Set faults that may occur executing operations of this interface by
* indexed position.
*
* @param index Indexed position value (0..length-1) of the item to set
* @param theFault Item to add at position index
.
*/
public void setFault(int index, com.sun.jbi.wsdl2.InterfaceFault theFault)
{
getBean().setFaultArray(
index,
theFault != null ? ((InterfaceFaultImpl) theFault).getBean()
: null);
}
/**
* Append an item to faults that may occur executing operations of this
* interface.
*
* @param theFault Item to append to faults
*/
public void appendFault(com.sun.jbi.wsdl2.InterfaceFault theFault)
{
synchronized (getBean().monitor())
{
setFault(getFaultsLength(), theFault);
}
}
/**
* Remove faults that may occur executing operations of this interface by
* index position.
*
* @param index The index position of the fault to remove
* @return The InterfaceFault removed, if any.
*/
public com.sun.jbi.wsdl2.InterfaceFault removeFault(int index)
{
com.sun.jbi.wsdl2.InterfaceFault result;
synchronized (getBean().monitor())
{
result = getFault(index);
getBean().removeFault(index);
}
return result;
}
/**
* Get the number of InterfaceOperation items in operations.
*
* @return The number of InterfaceOperation items in operations
*/
public int getOperationsLength()
{
return getBean().sizeOfOperationArray();
}
/**
* Get operations defined by this interface only, by indexed position.
*
* @param index Indexed position value 0..length-1
* @return Operations defined by this interface only. at given
* index
position.
*/
public com.sun.jbi.wsdl2.InterfaceOperation getOperation(int index)
{
return InterfaceOperationImpl.Factory.getInstance(
getBean().getOperationArray(index),
this.mContainer);
}
/**
* Set operations defined by this interface only, by indexed position.
*
* @param index Indexed position value (0..length-1) of the item to set
* @param theOperation Item to add at position index
.
*/
public void setOperation(
int index,
com.sun.jbi.wsdl2.InterfaceOperation theOperation)
{
getBean().setOperationArray(
index,
theOperation != null
? ((InterfaceOperationImpl) theOperation).getBean()
: null);
}
/**
* Append an item to operations defined by this interface only.
*
* @param theOperation Item to append to operations
*/
public void appendOperation(
com.sun.jbi.wsdl2.InterfaceOperation theOperation)
{
synchronized (getBean().monitor())
{
setOperation(getOperationsLength(), theOperation);
}
}
/**
* Remove operations defined by this interface only, by index position.
*
* @param index The index position of the operation to remove
* @return The InterfaceOperation removed, if any.
*/
public com.sun.jbi.wsdl2.InterfaceOperation removeOperation(
int index)
{
com.sun.jbi.wsdl2.InterfaceOperation result;
synchronized (getBean().monitor())
{
result = getOperation(index);
getBean().removeOperation(index);
}
return result;
}
/**
* Get the interfaces extended by this interface, including those extended by
* this interface's super-interfaces.
*
* @return Extended interfaces, including those extended by this interface's
* super-interfaces
*/
public com.sun.jbi.wsdl2.Interface[] getExtendedInterfaces()
{
com.sun.jbi.wsdl2.Interface[] result;
Map map = new HashMap();
Object[] interfaces;
synchronized (getBean().monitor())
{
List names = getBean().getExtends();
getSuperInterfaces(names, map);
interfaces = map.values().toArray();
}
result = new com.sun.jbi.wsdl2.Interface[interfaces.length];
System.arraycopy(interfaces, 0, result, 0, interfaces.length);
return result;
}
/**
* Get the interfaces corresponding to each the given QNames, placing
* them into the given map, key by QName string value.
*
* @param names A list of QNames of interfaces to find.
* @param map A map to place the (QName, Interface) pairs into
*/
private void getSuperInterfaces(List names, Map map)
{
final Iterator eachName = names != null ? names.iterator() : null;
while (eachName != null && eachName.hasNext())
{
QName qName = (QName) eachName.next();
String name = qName.toString();
if (!map.containsKey(name)) // Avoid circular or duplicate inheritance
{
com.sun.jbi.wsdl2.Interface iface = mContainer.findInterface(qName);
if (iface != null)
{
map.put(name, iface);
// Recurse up the inheritance tree.
getSuperInterfaces(
((InterfaceImpl) iface).getBean().getExtends(),
map);
}
}
}
}
/**
* Get the operations defined by this interface, and all its super-interfaces
*
* @return Operations defined by this interface, and all super-interfaces
*/
public com.sun.jbi.wsdl2.InterfaceOperation[] getExtendedOperations()
{
ArrayList result = new ArrayList();
Map ifaceMap = new HashMap();
List names;
synchronized (getBean().monitor())
{
names = getBean().getExtends();
addInterfaceOperations(this, result); // include self-defined operations
getSuperInterfaces(names, ifaceMap);
Iterator eachIface = ifaceMap.values().iterator();
while (eachIface.hasNext())
{
addInterfaceOperations(
(com.sun.jbi.wsdl2.Interface) eachIface.next(),
result);
}
}
com.sun.jbi.wsdl2.InterfaceOperation[] retVal =
new com.sun.jbi.wsdl2.InterfaceOperation[result.size()];
for (int idx = 0; idx < retVal.length; idx++)
{
retVal[idx] = (com.sun.jbi.wsdl2.InterfaceOperation) result.get(idx);
}
return retVal;
}
/**
* Add the operations for the given interface to the given operations list.
*
* This is a gathering function used by {@link #getExtendedOperations()} to
* build the full operations list for all interfaces it is interested in.
*
* @param iface The interface whose operations are to be added to the given
* list. This may be null.
* @param opsList The list to which to add the operations found.
*/
private static void addInterfaceOperations(
com.sun.jbi.wsdl2.Interface iface, List opsList)
{
if (iface != null)
{
final int nOps = iface.getOperationsLength();
for (int idx = 0; idx < nOps; idx++)
{
com.sun.jbi.wsdl2.InterfaceOperation op =
(com.sun.jbi.wsdl2.InterfaceOperation) iface.getOperation(idx);
if (op != null)
{
opsList.add(op);
}
}
}
}
/**
* Create a new operation, appending it to this interface's operations list.
*
* @return Newly created operation, appended to the operations list.
*/
public com.sun.jbi.wsdl2.InterfaceOperation addNewOperation()
{
return InterfaceOperationImpl.Factory.getInstance(
getBean().addNewOperation(),
this.mContainer);
}
/**
* Create a new fault, and append it to this interface's faults list.
*
* @param name Name of the new fault
* @return Newly created interface, appended to the faults list.
*/
public com.sun.jbi.wsdl2.InterfaceFault addNewFault(String name)
{
return null; // $$TOD
}
/**
* A factory class for creating / finding components for given XML beans.
*
* This factory guarantees that there will only be one component for each
* XML bean instance.
*/
static class Factory
{
/**
* Find the WSDL interface component associated with the given XML
* bean, creating a new component if necessary.
*
* This is thread-safe.
*
* @param bean The XML bean to find the component for.
* @param defs The container for the component.
* @return The WSDL interface component for the given bean
* (null if the bean
is null).
*/
static InterfaceImpl getInstance(InterfaceType bean, DescriptionImpl defs)
{
InterfaceImpl result;
if (bean != null)
{
Map map = defs.getInterfaceMap();
synchronized (map)
{
result = (InterfaceImpl) map.get(bean);
if (result == null)
{
result = new InterfaceImpl(bean, defs);
map.put(bean, result);
}
}
}
else
{
result = null;
}
return result;
}
} // end inner class Factory
}
// End-of-file: InterfaceImpl.java