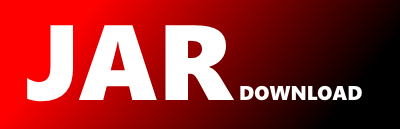
com.sun.jbi.wsdl2.impl.InterfaceOperationImpl Maven / Gradle / Ivy
/*
* BEGIN_HEADER - DO NOT EDIT
*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the "License"). You may not use this file except
* in compliance with the License.
*
* You can obtain a copy of the license at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* HEADER in each file and include the License file at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* If applicable add the following below this CDDL HEADER,
* with the fields enclosed by brackets "[]" replaced with
* your own identifying information: Portions Copyright
* [year] [name of copyright owner]
*/
/*
* @(#)InterfaceOperationImpl.java
* Copyright 2004-2007 Sun Microsystems, Inc. All Rights Reserved.
*
* END_HEADER - DO NOT EDIT
*/
package com.sun.jbi.wsdl2.impl;
import java.io.StringWriter;
import java.util.Map;
import javax.xml.namespace.QName;
import org.apache.xmlbeans.XmlOptions;
import org.w3.ns.wsdl.InterfaceOperationType;
import org.w3.ns.wsdl.MessageRefFaultType;
import org.w3c.dom.DocumentFragment;
/**
* Implementation of WSDL 2.0 interface operation component.
*
* @author Sun Microsystems, Inc.
*/
final class InterfaceOperationImpl extends InterfaceOperation
{
/** Container for this component */
private DescriptionImpl mContainer;
/**
* Get the container for this component.
*
* @return The component for this component
*/
protected DescriptionImpl getContainer()
{
return this.mContainer;
}
/**
* Returns true if the operation is safe, as defined by the W3C Web Services
* Architecture document.
*
* @return True if the operation is safe, as defined by the W3C Web
* Services Architecture document
*/
public boolean getSafe()
{
return getBean().getSafe();
}
/**
* Set true if the operation is safe, as defined by the W3C Web Services
* Architecture document.
*
* @param isSafe True if the operation is safe, as defined by the W3C
* Web Services Architecture document
*/
public void setSafe(boolean isSafe)
{
getBean().setSafe(isSafe);
}
/**
* Construct an interface operation component implementation object from the
* given XML bean.
* @param bean The interface operation XML bean to use to construct this
* component.
* @param defs The definitions container for the interface operation.
*/
private InterfaceOperationImpl(InterfaceOperationType bean,
DescriptionImpl defs)
{
super(bean);
this.mContainer = defs;
}
/** Map of WSDL-defined attribute QNames. Keyed by QName.toString value */
private static java.util.Map sWsdlAttributeQNames = null;
/**
* Worker class method for {@link #getWsdlAttributeNameMap()}.
*
* @return Map of WSDL-defined attribute QNames for this component,
* indexed by QName.toString()
*/
static synchronized java.util.Map getAttributeNameMap()
{
if (sWsdlAttributeQNames == null)
{
sWsdlAttributeQNames = XmlBeansUtil.getAttributesMap(
InterfaceOperationType.type);
}
return sWsdlAttributeQNames;
}
/**
* Get map of WSDL-defined attribute QNames for this component, indexed by
* canonical QName string (see {@link javax.xml.namespace.QName#toString()}.
*
* @return Map of WSDL-defined attribute QNames for this component,
* indexed by QName.toString()
*/
public java.util.Map getWsdlAttributeNameMap()
{
return getAttributeNameMap();
}
/**
* Get name of this interface operation component.
*
* @return Name of this interface operation component
*/
public String getName()
{
return getBean().getName();
}
/**
* Set name of this interface operation component.
*
* @param theName Name of this interface operation component
*/
public void setName(String theName)
{
getBean().setName(theName);
}
/**
* Get message exchange pattern URI for this operation.
*
* @return Message exchange pattern URI for this operation
*/
public String getPattern()
{
return getBean().getPattern();
}
/**
* Set message exchange pattern URI for this operation.
*
* @param thePattern Message exchange pattern URI for this operation
*/
public void setPattern(String thePattern)
{
getBean().setPattern(thePattern);
}
/**
* Get a URI identifying the message construction rules for a message reference.
*
* @return A URI identifying the message construction rules for a message reference
*/
public String getStyle()
{
return getBean().getStyle();
}
/**
* Set a URI identifying the message construction rules for a message reference.
*
* @param theStyle A URI identifying the message construction rules for a message
* reference
*/
public void setStyle(String theStyle)
{
getBean().setStyle(theStyle);
}
/**
* Get the number of MessageReference items in inputs.
*
* @return The number of MessageReference items in inputs
*/
public int getInputsLength()
{
return getBean().sizeOfInputArray();
}
/**
* Get input message references by indexed position.
*
* @param index Indexed position value 0..length-1
* @return Input message references at given index
position.
*/
public com.sun.jbi.wsdl2.MessageReference getInput(int index)
{
return MessageReferenceImpl.Factory.getInstance(
getBean().getInputArray(index),
this.mContainer);
}
/**
* Set input message references by indexed position.
*
* @param index Indexed position value (0..length-1) of the item to set
* @param theInput Item to add at position index
.
*/
public void setInput(int index,
com.sun.jbi.wsdl2.MessageReference theInput)
{
getBean().setInputArray(
index,
theInput != null ? ((MessageReferenceImpl) theInput).getBean() : null );
}
/**
* Append an item to input message references.
*
* @param theInput Item to append to inputs
*/
public void appendInput(com.sun.jbi.wsdl2.MessageReference theInput)
{
synchronized (getBean().monitor())
{
setInput(getInputsLength(), theInput);
}
}
/**
* Remove input message references by index position.
*
* @param index The index position of the input to remove
* @return The MessageReference removed, if any.
*/
public com.sun.jbi.wsdl2.MessageReference removeInput(int index)
{
com.sun.jbi.wsdl2.MessageReference result;
synchronized (getBean().monitor())
{
result = getInput(index);
getBean().removeInput(index);
}
return result;
}
/**
* Get the number of MessageReference items in outputs.
*
* @return The number of MessageReference items in outputs
*/
public int getOutputsLength()
{
return getBean().sizeOfOutputArray();
}
/**
* Get output message references by indexed position.
*
* @param index Indexed position value 0..length-1
* @return Output message references at given index
position.
*/
public com.sun.jbi.wsdl2.MessageReference getOutput(int index)
{
return MessageReferenceImpl.Factory.getInstance(
getBean().getOutputArray(index),
this.mContainer);
}
/**
* Set output message references by indexed position.
*
* @param index Indexed position value (0..length-1) of the item to set
* @param theOutput Item to add at position index
.
*/
public void setOutput(
int index,
com.sun.jbi.wsdl2.MessageReference theOutput)
{
getBean().setOutputArray(
index,
theOutput != null ? ((MessageReferenceImpl) theOutput).getBean() : null);
}
/**
* Append an item to output message references.
*
* @param theOutput Item to append to outputs
*/
public void appendOutput(com.sun.jbi.wsdl2.MessageReference theOutput)
{
synchronized (getBean().monitor())
{
setOutput(getOutputsLength(), theOutput);
}
}
/**
* Remove output message references by index position.
*
* @param index The index position of the output to remove
* @return The MessageReference removed, if any.
*/
public com.sun.jbi.wsdl2.MessageReference removeOutput(int index)
{
com.sun.jbi.wsdl2.MessageReference result;
synchronized (getBean().monitor())
{
result = getOutput(index);
getBean().removeOutput(index);
}
return result;
}
/**
* Get the number of MessageFaultReference items in inFaults.
*
* @return The number of MessageFaultReference items in inFaults
*/
public int getInFaultsLength()
{
return getBean().sizeOfInfaultArray();
}
/**
* Get in fault message references by indexed position.
*
* @param index Indexed position value 0..length-1
* @return In fault message references at given index
position.
*/
public com.sun.jbi.wsdl2.MessageFaultReference getInFault(int index)
{
return MessageFaultReferenceImpl.Factory.getInstance(
getBean().getInfaultArray(index),
this.mContainer);
}
/**
* Set in fault message references by indexed position.
*
* @param index Indexed position value (0..length-1) of the item to set
* @param theInFault Item to add at position index
.
*/
public void setInFault(
int index,
com.sun.jbi.wsdl2.MessageFaultReference theInFault)
{
getBean().setInfaultArray(
index,
theInFault != null
? ((MessageFaultReferenceImpl) theInFault).getBean()
: null);
}
/**
* Append an item to in fault message references.
*
* @param theInFault Item to append to inFaults
*/
public void appendInFault(
com.sun.jbi.wsdl2.MessageFaultReference theInFault)
{
synchronized (getBean().monitor())
{
setInFault(getInFaultsLength(), theInFault);
}
}
/**
* Remove in fault message references by index position.
*
* @param index The index position of the inFault to remove
* @return The MessageFaultReference removed, if any.
*/
public com.sun.jbi.wsdl2.MessageFaultReference removeInFault(int index)
{
com.sun.jbi.wsdl2.MessageFaultReference result;
synchronized (getBean().monitor())
{
result = getInFault(index);
getBean().removeInfault(index);
}
return result;
}
/**
* Get the number of MessageFaultReference items in outFaults.
*
* @return The number of MessageFaultReference items in outFaults
*/
public int getOutFaultsLength()
{
return getBean().sizeOfOutfaultArray();
}
/**
* Get out fault message references by indexed position.
*
* @param index Indexed position value 0..length-1
* @return Out fault message references at given index
position.
*/
public com.sun.jbi.wsdl2.MessageFaultReference getOutFault(int index)
{
return MessageFaultReferenceImpl.Factory.getInstance(
getBean().getOutfaultArray(index),
this.mContainer);
}
/**
* Set out fault message references by indexed position.
*
* @param index Indexed position value (0..length-1) of the item to set
* @param theOutFault Item to add at position index
.
*/
public void setOutFault(
int index,
com.sun.jbi.wsdl2.MessageFaultReference theOutFault)
{
getBean().setOutfaultArray(
index,
theOutFault != null
? ((MessageFaultReferenceImpl) theOutFault).getBean()
: null);
}
/**
* Append an item to out fault message references.
*
* @param theOutFault Item to append to outFaults
*/
public void appendOutFault(
com.sun.jbi.wsdl2.MessageFaultReference theOutFault)
{
synchronized (getBean().monitor())
{
setOutFault(getOutFaultsLength(), theOutFault);
}
}
/**
* Remove out fault message references by index position.
*
* @param index The index position of the outFault to remove
* @return The MessageFaultReference removed, if any.
*/
public com.sun.jbi.wsdl2.MessageFaultReference removeOutFault(int index)
{
com.sun.jbi.wsdl2.MessageFaultReference result;
synchronized (getBean().monitor())
{
result = getOutFault(index);
getBean().removeOutfault(index);
}
return result;
}
/**
* Create a new input message reference for this operation, and append
* it to this operation's input list.
*
* @return The newly created input message reference.
*/
public com.sun.jbi.wsdl2.MessageReference addNewInput()
{
return MessageReferenceImpl.Factory.getInstance(
getBean().addNewInput(),
this.mContainer);
}
/**
* Create a new output message reference for this operation, and append
* it to this operation's output list.
*
* @return The newly created input message reference.
*/
public com.sun.jbi.wsdl2.MessageReference addNewOutput()
{
return MessageReferenceImpl.Factory.getInstance(
getBean().addNewOutput(),
this.mContainer);
}
/**
* Create a new messsage in-fault reference for this operation, and
* append it to the operation's in-fault list.
*
* @param fault Fault to add to this operation.
* @return The newly created in-fault reference.
*/
public com.sun.jbi.wsdl2.MessageFaultReference addNewInFault(
com.sun.jbi.wsdl2.InterfaceFault fault)
{
MessageRefFaultType faultBean = getBean().addNewInfault();
if (fault != null)
{
faultBean.setRef(fault.getQualifiedName());
}
return MessageFaultReferenceImpl.Factory.getInstance(
faultBean,
this.mContainer);
}
/**
* Create a new messsage out-fault reference for this operation, and
* append it to the operation's out-fault list.
*
* @param fault Fault to add to this operation.
* @return The newly created out-fault reference.
*/
public com.sun.jbi.wsdl2.MessageFaultReference addNewOutFault(
com.sun.jbi.wsdl2.InterfaceFault fault)
{
MessageRefFaultType faultBean = getBean().addNewOutfault();
if (fault != null)
{
faultBean.setRef(fault.getQualifiedName());
}
return MessageFaultReferenceImpl.Factory.getInstance(
faultBean,
this.mContainer);
}
/**
* Return this WSDL interface operation as an XML string.
*
* @return This operation, serialized as an XML string.
*/
public String toXmlString()
{
String result;
StringWriter sw = new StringWriter();
XmlOptions options = new XmlOptions();
options.setSavePrettyPrint();
options.setSavePrettyPrintIndent(Constants.XML_PRETTY_PRINT_INDENT);
options.setSaveOuter();
try
{
getBean().save(sw, options);
sw.close();
}
catch (java.io.IOException ex)
{
sw.write( "\n\n" );
// $TODO: log error
}
return sw.getBuffer().toString();
}
/**
* Return this interface operation as a DOM document fragment. The DOM
* subtree is a copy; altering it will not affect this service.
*
* @return This operation, as a DOM document fragment.
*/
public DocumentFragment toXmlDocumentFragment()
{
XmlOptions options = new XmlOptions();
options.setSaveOuter();
return (DocumentFragment) getBean().newDomNode(options);
}
/**
* Get qualified name of this interface operation.
*
* @return Qualified name of this interface operation
*/
public QName getQualifiedName()
{
return new QName(
this.mContainer.getTargetNamespace(),
getBean().getName());
}
/**
* A factory class for creating / finding components for given XML beans.
*
* This factory guarantees that there will only be one component for each
* XML bean instance.
*/
static class Factory
{
/**
* Find the WSDL interface operation component associated with the given XML
* bean, creating a new component if necessary.
*
* This is thread-safe.
*
* @param bean The XML bean to find the component for.
* @param defs The container for the component.
* @return The WSDL interface operation component for the given bean
* (null if the bean
is null).
*/
static InterfaceOperationImpl getInstance(InterfaceOperationType bean,
DescriptionImpl defs)
{
InterfaceOperationImpl result;
if (bean != null)
{
Map map = defs.getInterfaceOperationMap();
synchronized (map)
{
result = (InterfaceOperationImpl) map.get(bean);
if (result == null)
{
result = new InterfaceOperationImpl(bean, defs);
map.put(bean, result);
}
}
}
else
{
result = null;
}
return result;
}
}
}
// End-of-file: InterfaceOperationImpl.java