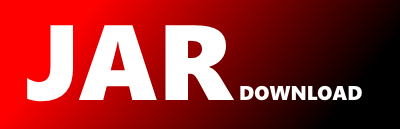
com.sun.jbi.wsdl2.impl.WsdlWriter Maven / Gradle / Ivy
/*
* BEGIN_HEADER - DO NOT EDIT
*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the "License"). You may not use this file except
* in compliance with the License.
*
* You can obtain a copy of the license at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* HEADER in each file and include the License file at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* If applicable add the following below this CDDL HEADER,
* with the fields enclosed by brackets "[]" replaced with
* your own identifying information: Portions Copyright
* [year] [name of copyright owner]
*/
/*
* @(#)WsdlWriter.java
* Copyright 2004-2007 Sun Microsystems, Inc. All Rights Reserved.
*
* END_HEADER - DO NOT EDIT
*/
package com.sun.jbi.wsdl2.impl;
import com.sun.jbi.wsdl2.Description;
import com.sun.jbi.wsdl2.Definitions;
import com.sun.jbi.wsdl2.WsdlException;
import java.io.IOException;
import java.io.OutputStream;
import java.io.Writer;
import org.apache.xmlbeans.XmlOptions;
import org.w3c.dom.Document;
import org.w3c.dom.Node;
/**
* This class supplies a WSDL serializer, where the serialization is
* WSDL 2.0 compliant XML.
*
* @author Sun Microsystems, Inc.
*/
final class WsdlWriter implements com.sun.jbi.wsdl2.WsdlWriter
{
/**
* Return a document generated from the specified WSDL model.
*
* @param model The WSDL definitions component to be written
* @return A DOM document that reflects the contents of the given model
* @exception WsdlException When a problem reading the model
* occurs.
*/
public Document getDocument(Description model) throws WsdlException
{
Document result = null;
DescriptionImpl defs = (DescriptionImpl) model;
if (defs != null)
{
Node node = defs.getDocBean().newDomNode();
if (node != null && node.getNodeType() == Node.DOCUMENT_NODE)
{
result = (Document) node;
}
}
return result;
}
/**
*
* @deprecated - replaced by getDocument(Description model)
*
*/
public Document getDocument(Definitions model) throws WsdlException
{
return getDocument((Description) model);
}
/**
* Write the specified WSDL definition to the specified Writer.
*
* @param model The WSDL definitions component to be written
* @param sink The Writer to write the xml to
* @exception WsdlException When a problem reading the model
* occurs.
* @exception IOException When a problem writing to sink
* occurs.
*/
public void writeDescription(Description model, Writer sink)
throws WsdlException, IOException
{
DescriptionImpl defs = (DescriptionImpl) model;
if (defs != null && sink != null)
{
XmlOptions opts = new XmlOptions();
opts.setSavePrettyPrint();
opts.setSavePrettyPrintIndent(Constants.XML_PRETTY_PRINT_INDENT);
defs.getDocBean().save(sink, opts);
}
return;
}
/**
* @deprecated - replaced by writeDescription
*/
public void writeWsdl(Definitions model, Writer sink)
throws WsdlException, IOException
{
writeDescription((Description) model, sink);
}
/**
* Write the specified WSDL definition to the specified OutputStream.
*
* @param model The WSDL definitions component to be written
* @param sink The OutputStream to write the XML to
* @exception WsdlException When a problem reading the model
* occurs.
* @exception IOException When a problem writing to sink
* occurs.
*/
public void writeDescription(Description model, OutputStream sink)
throws WsdlException, IOException
{
DescriptionImpl defs = (DescriptionImpl) model;
if (defs != null && sink != null)
{
XmlOptions opts = new XmlOptions();
opts.setSavePrettyPrint();
opts.setSavePrettyPrintIndent(Constants.XML_PRETTY_PRINT_INDENT);
defs.getDocBean().save(sink);
}
return;
}
/**
* @deprecated - replaced by writeDescription
*/
public void writeWsdl(Definitions model, OutputStream sink)
throws WsdlException, IOException
{
writeDescription((Description) model, sink);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy