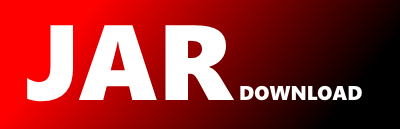
com.sun.jbi.wsdl2.impl.XmlBeansUtil Maven / Gradle / Ivy
/*
* BEGIN_HEADER - DO NOT EDIT
*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the "License"). You may not use this file except
* in compliance with the License.
*
* You can obtain a copy of the license at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* HEADER in each file and include the License file at
* https://open-esb.dev.java.net/public/CDDLv1.0.html.
* If applicable add the following below this CDDL HEADER,
* with the fields enclosed by brackets "[]" replaced with
* your own identifying information: Portions Copyright
* [year] [name of copyright owner]
*/
/*
* @(#)XmlBeansUtil.java
* Copyright 2004-2007 Sun Microsystems, Inc. All Rights Reserved.
*
* END_HEADER - DO NOT EDIT
*/
package com.sun.jbi.wsdl2.impl;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import javax.xml.namespace.QName;
import org.apache.xmlbeans.SchemaAttributeModel;
import org.apache.xmlbeans.SchemaLocalAttribute;
import org.apache.xmlbeans.SchemaType;
/**
* This class collects a set of XmlBeans utilities.
*
* @author Sun Microsystems, Inc.
*/
class XmlBeansUtil
{
/**
* Get the qualified names of all attributes given in the attribute model
* of the given schema type. This is handy for distinguishing extension
* attributes in the empty namespace.
*
* @param type The schema type to analyze.
* @return A possibly empty list of attribute QNames.
*/
static List getAttributes(SchemaType type)
{
ArrayList result = new ArrayList();
SchemaAttributeModel model;
if (type != null && (model = type.getAttributeModel()) != null)
{
SchemaLocalAttribute[] attrs = model.getAttributes();
final int nAttrs = attrs != null ? attrs.length : 0;
for (int idx = 0; idx < nAttrs; idx++)
{
result.add(attrs[idx].getName());
}
}
return result;
}
/**
* Get a map of the qualified names of all attributes given in the attribute
* model of the given schema type. This is handy for distinguishing extension
* attributes in the empty namespace.
*
* @param type The schema type to analyze.
* @return A possibly empty map of attribute QNames, keyed on the QName
* canonical name string.
*/
static Map getAttributesMap(SchemaType type)
{
HashMap result = new HashMap();
List attrs = getAttributes(type);
Iterator eachAttr = attrs.iterator();
while (eachAttr.hasNext())
{
QName attrName = (QName) eachAttr.next();
result.put(attrName.toString(), attrName);
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy