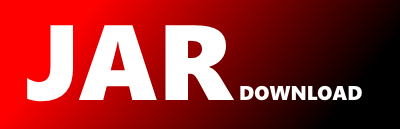
net.openhft.chronicle.map.ReaderWithSize Maven / Gradle / Ivy
/*
* Copyright (C) 2015 higherfrequencytrading.com
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*/
package net.openhft.chronicle.map;
import net.openhft.chronicle.hash.serialization.BytesReader;
import net.openhft.chronicle.hash.serialization.SizeMarshaller;
import net.openhft.lang.io.Bytes;
import net.openhft.lang.threadlocal.Provider;
import net.openhft.lang.threadlocal.ThreadLocalCopies;
import org.jetbrains.annotations.Nullable;
final class ReaderWithSize {
private final SizeMarshaller sizeMarshaller;
private final BytesReader originalReader;
private final Provider> readerProvider;
ReaderWithSize(SerializationBuilder serializationBuilder) {
sizeMarshaller = serializationBuilder.sizeMarshaller();
originalReader = serializationBuilder.reader();
readerProvider = (Provider>) Provider.of(originalReader.getClass());
}
public ThreadLocalCopies getCopies(ThreadLocalCopies copies) {
return readerProvider.getCopies(copies);
}
public T read(Bytes in, @Nullable ThreadLocalCopies copies, @Nullable T using) {
long size = sizeMarshaller.readSize(in);
copies = readerProvider.getCopies(copies);
BytesReader reader = readerProvider.get(copies, originalReader);
return reader.read(in, size, using);
}
public T readNullable(Bytes in, @Nullable ThreadLocalCopies copies, T using) {
if (in.readBoolean())
return null;
return read(in, copies, using);
}
public BytesReader readerForLoop(@Nullable ThreadLocalCopies copies) {
copies = readerProvider.getCopies(copies);
return readerProvider.get(copies, originalReader);
}
public T readInLoop(Bytes in, BytesReader reader) {
long size = sizeMarshaller.readSize(in);
return reader.read(in, size);
}
public T readNullableInLoop(Bytes in, BytesReader reader) {
if (in.readBoolean())
return null;
return readInLoop(in, reader);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy