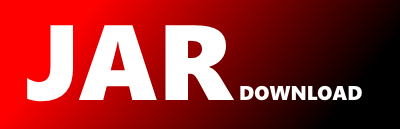
net.openhft.chronicle.map.ChronicleMap Maven / Gradle / Ivy
/*
* Copyright (C) 2015 higherfrequencytrading.com
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*/
package net.openhft.chronicle.map;
import net.openhft.chronicle.hash.ChronicleHash;
import net.openhft.chronicle.hash.serialization.BytesReader;
import net.openhft.lang.io.Bytes;
import net.openhft.lang.io.serialization.BytesMarshaller;
import net.openhft.lang.model.Byteable;
import org.jetbrains.annotations.NotNull;
import java.io.Closeable;
import java.io.File;
import java.io.IOException;
import java.util.Map;
import java.util.concurrent.ConcurrentMap;
/**
* Extension of {@link ConcurrentMap} interface, stores the data off-heap.
*
* For information on
- how to construct a {@code ChronicleMap}
- {@code
* ChronicleMap} flavors and properties
- available configurations
see {@link
* ChronicleMapBuilder} documentation.
*
* Functionally this interface defines some methods supporting garbage-free off-heap programming:
* {@link #getUsing(Object, Object)}, {@link #acquireUsing(Object, Object)}.
*
*
Roughly speaking, {@code ChronicleMap} compares keys and values by their binary serialized
* form, that shouldn't necessary be the same equality relation as defined by built-in {@link
* Object#equals(Object)} method, which is prescribed by general {@link Map} contract.
*
*
Note that {@code ChronicleMap} extends {@link Closeable}, don't forget to {@linkplain #close()
* close} map when it is no longer needed.
*
* @param the map key type
* @param the map value type
*/
public interface ChronicleMap extends ConcurrentMap, ChronicleHash {
/**
* Returns the number of entries in this map.
*
* @return number of entries in this map
* @see Map#size()
*/
long longSize();
/**
* Returns the value to which the specified key is mapped, or {@code null} if this map contains
* no mapping for the key.
*
* If the value class allows reusing, particularly if it is a {@link Byteable} subclass,
* consider {@link #getUsing(Object, Object)} method instead of this to reduce garbage
* creation.
*
* @param key the key whose associated value is to be returned
* @return the value to which the specified key is mapped after this method call, or {@code
* null} if no value is mapped
* @see #getUsing(Object, Object)
*/
@Override
V get(Object key);
/**
* Returns the value to which the specified key is mapped, read to the provided {@code value}
* object, if possible, or returns {@code null}, if this map contains no mapping for the key.
*
*
If the specified key is present in the map, the value data is read to the provided {@code
* value} object via value marshaller's {@link BytesMarshaller#read(Bytes, Object) read(Bytes,
* value)} or value reader's {@link BytesReader#read(Bytes, long, Object) read(Bytes, size,
* value)} method, depending on what deserialization strategy is configured on the builder,
* using which this map was constructed. If the value deserializer is able to reuse the given
* {@code value} object, calling this method instead of {@link #get(Object)} could help to
* reduce garbage creation.
*
*
The provided {@code value} object is allowed to be {@code null}, in this case {@code
* map.getUsing(key, null)} call is semantically equivalent to simple {@code map.get(key)}
* call.
*
* @param key the key whose associated value is to be returned
* @param usingValue the object to read value data in, if possible
* @return the value to which the specified key is mapped, or {@code null} if this map contains
* no mapping for the key
* @see #get(Object)
* @see #acquireUsing(Object, Object)
* @see ChronicleMapBuilder#valueMarshaller(BytesMarshaller)
*/
V getUsing(K key, V usingValue);
/**
* The method is similar {@link V getUsing(K key, V usingValue);} but in addition read locks
* the map segment to operations to be performed atomically. ( see the example below )
*
* Returns the ReadContext which holds a map segment lock and provides method to get the value
* ( to which the specified key is mapped) atomically ( see example below for an explanation ),
* read to the provided {@code value} object, if possible, or returns {@code null}, if this map
* contains no mapping for the key.
*
*
If the specified key is present in the map, the readContext.value() uses the provided
* {@code usingValue} object via value marshaller's {@link BytesMarshaller#read(Bytes, Object)
* read(Bytes, value)} or value reader's {@link BytesReader#read(Bytes, long, Object)
* read(Bytes, size, value)} method, depending on what deserialization strategy is configured on
* the builder, using which this map was constructed. If the value deserializer is able to reuse
* the given {@code usingValue} object, calling this method instead of {@link #get(Object)}
* could help to reduce garbage creation.
*
{@code
* try (ReadContext rc = map.getUsingLocked(key, bond)) {
* if (rc.present ()) { // check whether the key was present
* long issueDate = bond.getIssueDate();
* String symbol = bond.getSymbol();
* // add your logic here ( the lock will ensure this bond can not be changed by another thread
* )
* }
* } // the read lock is released here
* }
* To ensure that you can read the 'issueDate' and 'symbol' can be read atomically, these values
* must be read while the segment lock is in place.
*
*
* The provided {@code value} object is allowed to be {@code null}, in this case
*
* @param key the key whose associated value is to be returned
* @param usingValue the object to read value data in, if possible
* @return the read context containing the value to which the specified key is mapped
*
* no mapping for the key
* @see #get(Object)
* @see #getUsing(Object, Object)
* @see ChronicleMapBuilder#valueMarshaller(BytesMarshaller)
*/
@NotNull
ReadContext getUsingLocked(@NotNull K key, @NotNull V usingValue);
/**
* Acquire a value for a key, creating if absent.
*
* If the specified key is absent in the map, {@linkplain ChronicleMapBuilder#defaultValue(Object)
* default value} is taken or {@linkplain ChronicleMapBuilder#defaultValueProvider(DefaultValueProvider)
* default value provider} is called. Then this object is put to this map for the specified
* key.
*
*
Then, either if the key was initially absent in the map or already present, the value is
* deserialized just as during {@link #getUsing(Object, Object) getUsing(key, usingValue)} call,
* passed the same {@code key} and {@code usingValue} as into this method call. This means, as
* in {@link #getUsing}, {@code usingValue} could safely be {@code null}, in this case a new
* value instance is created to deserialize the data.
*
* In code, {@code acquireUsing} is specified as :
*
{@code
* V acquireUsing(K key, V usingValue) {
* if (!containsKey(key))
* put(key, defaultValue(key));
* return getUsing(key, usingValue);
* }}
*
*
* Where {@code defaultValue(key)} returns either {@linkplain ChronicleMapBuilder#defaultValue(Object)
* default value} or {@link ChronicleMapBuilder#defaultValueProvider(DefaultValueProvider)
* defaultValueProvider.}
*
* If the {@code ChronicleMap} is off-heap updatable, i. e. created via {@link
* ChronicleMapBuilder} builder (values are {@link Byteable}), there is one more option of what
* to do if the key is absent in the map. By default, value bytes are just zeroed out, no
* default value, either provided for key or constant, is put for the absent key.
*
* @param key the key whose associated value is to be returned
* @param usingValue the object to read value data in, if present. Can not be null
* @return value to which the given key is mapping after this call, either found or created
* @see #getUsing(Object, Object)
*/
V acquireUsing(@NotNull K key, V usingValue);
@NotNull
WriteContext acquireUsingLocked(@NotNull K key, @NotNull V usingValue);
/**
* Apply a mapping to the value returned by a key and return a result. A read lock is assumed.
*
* @param key to apply the mapping to
* @param function to calculate a result
* @param return type.
* @return the result of the function, or null if there is no entry for the key.
*/
R getMapped(K key, @NotNull Function super V, R> function);
/**
* Apply a unaryOperator to the value for a key and return a result. A write lock is assumed.
* If there is no entry for this key null will be returned
*
* @param key to apply the mapping to
* @param unaryOperator to alter the value and calculate a result
* @return the result of the function.
*/
V putMapped(K key, @NotNull UnaryOperator unaryOperator);
/**
* A special operation, similar to {@link #replace(Object, Object, Object)
* replace(key, value, value)}, but puts the entry even if it was absent in the map.
*/
UpdateResult update(K key, V value);
/**
* Exports all the entries to a {@link java.io.File} storing them in JSON format, an attempt is
* made where possible to use standard java serialisation and keep the data human readable, data
* serialized using the custom serialises are converted to a binary format which is not human
* readable but this is only done if the Keys or Values are not {@link java.io.Serializable}.
* This method can be used in conjunction with {@link ChronicleMap#putAll(java.io.File)} and is
* especially useful if you wish to import/export entries from one chronicle map into another.
* This import and export of the entries can be performed even when the versions of ChronicleMap
* differ. This method is not performant and as such we recommend it is not used in performance
* sensitive code.
*
* @param toFile the file to store all the entries to, the entries will be stored in JSON
* format
* @throws IOException its not possible store the data to {@code toFile}
* @see ChronicleMap#putAll(java.io.File)
*/
void getAll(File toFile) throws IOException;
/**
* Imports all the entries from a {@link java.io.File}, the {@code fromFile} must be created
* using or the same format as {@link ChronicleMap#get(java.lang.Object)}, this method behaves
* similar to {@link java.util.Map#put(java.lang.Object, java.lang.Object)} where existing
* entries are overwritten. A write lock is only held while each individual entry is inserted
* into the map, not over all the entries in the {@link java.io.File}
*
* @param fromFile the file containing entries ( in JSON format ) which will be deserialized and
* {@link java.util.Map#put(java.lang.Object, java.lang.Object)} into the map
* @throws IOException its not possible read the {@code fromFile}
* @see ChronicleMap#getAll(java.io.File)
*/
void putAll(File fromFile) throws IOException;
/**
* Creates an empty value instance, which can be used with the
* following methods :
*
* {@link ChronicleMap#getUsing(java.lang.Object, java.lang.Object) }
* {@link ChronicleMap#getUsingLocked(java.lang.Object, java.lang.Object) }
* {@link ChronicleMap#acquireUsing(java.lang.Object, java.lang.Object) }
* {@link ChronicleMap#acquireUsingLocked(java.lang.Object, java.lang.Object) }
*
* for example like this :
*
* {@code
* V value = map.newValueInstance();
* try (ReadContext rc = map.getUsingLocked(key, value)) {
* // add your logic here
* } // the read lock is released here
* }
*
*
* @return a new empty instance based on the Value type
* @see ChronicleMap#getUsing(java.lang.Object, java.lang.Object)
* @see ChronicleMap#getUsingLocked(java.lang.Object, java.lang.Object)
* @see ChronicleMap#acquireUsing(java.lang.Object, java.lang.Object)
* @see ChronicleMap#acquireUsingLocked(java.lang.Object, java.lang.Object)
*/
V newValueInstance();
/**
* Creates an empty value instance, which can be used with the
* following methods :
*
* {@link ChronicleMap#getUsing(java.lang.Object, java.lang.Object) }
* {@link ChronicleMap#getUsingLocked(java.lang.Object, java.lang.Object) }
* {@link ChronicleMap#acquireUsing(java.lang.Object, java.lang.Object) }
* {@link ChronicleMap#acquireUsingLocked(java.lang.Object, java.lang.Object) }
*
* for example like this :
*
* {@code
* K key = map.newKeyInstance();
* key.setMyStringField("some key");
*
* try (ReadContext rc = map.getUsingLocked(key, value)) {
* // add your logic here
* } // the read lock is released here
* }
*
*
* @return a new empty instance based on the Key type
* @see ChronicleMap#getUsing(java.lang.Object, java.lang.Object)
* @see ChronicleMap#getUsingLocked(java.lang.Object, java.lang.Object)
* @see ChronicleMap#acquireUsing(java.lang.Object, java.lang.Object)
* @see ChronicleMap#acquireUsingLocked(java.lang.Object, java.lang.Object)
*/
K newKeyInstance();
K readKey(Bytes entry, long keyPos);
V readValue(Bytes entry, long valuePos);
/**
* @return the class of {@code }
*/
Class keyClass();
/**
* @return the class of {@code }
*/
Class valueClass();
}