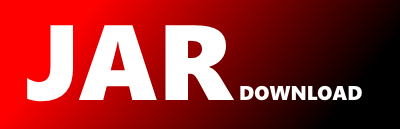
net.openhft.chronicle.map.SerializationBuilder Maven / Gradle / Ivy
/*
* Copyright (C) 2015 higherfrequencytrading.com
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*/
package net.openhft.chronicle.map;
import net.openhft.chronicle.hash.serialization.*;
import net.openhft.chronicle.hash.serialization.internal.*;
import net.openhft.chronicle.hash.serialization.internal.ByteBufferMarshaller;
import net.openhft.lang.io.ByteBufferBytes;
import net.openhft.lang.io.Bytes;
import net.openhft.lang.io.serialization.BytesMarshallable;
import net.openhft.lang.io.serialization.BytesMarshaller;
import net.openhft.lang.io.serialization.ObjectFactory;
import net.openhft.lang.io.serialization.ObjectSerializer;
import net.openhft.lang.io.serialization.impl.*;
import net.openhft.lang.model.*;
import net.openhft.lang.threadlocal.Provider;
import net.openhft.lang.threadlocal.ThreadLocalCopies;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import java.io.*;
import java.lang.reflect.Modifier;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.net.URL;
import java.nio.ByteBuffer;
import java.util.Arrays;
import java.util.List;
import static net.openhft.chronicle.hash.serialization.SizeMarshallers.constant;
import static net.openhft.chronicle.hash.serialization.SizeMarshallers.stopBit;
import static net.openhft.chronicle.map.Objects.hash;
final class SerializationBuilder implements Cloneable, Serializable {
private static final long serialVersionUID = 0L;
private static final Bytes EMPTY_BYTES = new ByteBufferBytes(ByteBuffer.allocate(0));
private static boolean concreteClass(Class c) {
return !c.isInterface() && !Modifier.isAbstract(c.getModifiers());
}
private static boolean marshallerUseFactory(Class c) {
return Byteable.class.isAssignableFrom(c) ||
BytesMarshallable.class.isAssignableFrom(c) ||
Externalizable.class.isAssignableFrom(c);
}
private static final List knownJDKImmutableClasses = Arrays.asList(
String.class, Byte.class, Short.class, Character.class, Integer.class,
Float.class, Long.class, Double.class, BigDecimal.class, BigInteger.class, URL.class
);
private static boolean instancesAreMutable(Class c) {
return !knownJDKImmutableClasses.contains(c);
}
enum Role {KEY, VALUE}
private enum CopyingInterop {FROM_MARSHALLER, FROM_WRITER}
private final Role role;
final Class eClass;
private boolean instancesAreMutable;
private SizeMarshaller sizeMarshaller = stopBit();
private BytesReader reader;
private Object interop;
private CopyingInterop copyingInterop = null;
private MetaBytesInterop metaInterop;
private MetaProvider metaInteropProvider;
private long maxSize;
final boolean sizeIsStaticallyKnown;
boolean serializesGeneratedClasses = false;
@SuppressWarnings("unchecked")
SerializationBuilder(Class eClass, Role role) {
this.role = role;
this.eClass = eClass;
configureByDefault(eClass, role);
sizeIsStaticallyKnown = constantSizeMarshaller();
}
private void configureByDefault(Class eClass, Role role) {
instancesAreMutable = instancesAreMutable(eClass);
if (eClass.isInterface()) {
try {
BytesReader reader = DataValueBytesMarshallers.acquireBytesReader(eClass);
BytesWriter writer = DataValueBytesMarshallers.acquireBytesWriter(eClass);
DataValueModel model = DataValueModels.acquireModel(eClass);
int size = DataValueGenerator.computeNonScalarOffset(model, eClass);
reader(reader);
copyingInterop(null);
setInterop(writer);
metaInterop((MetaBytesInterop) DataValueMetaBytesInterop.forIdentity(role));
metaInteropProvider(DataValueMetaBytesInterop.interopProvider(eClass));
sizeMarshaller(constant((long) size));
serializesGeneratedClasses = true;
return;
} catch (Exception e) {
try {
eClass = DataValueClasses.directClassFor(eClass);
serializesGeneratedClasses = true;
} catch (Exception ex) {
// ignore, fall through
}
// ignore, fall through
}
}
ObjectFactory factory = concreteClass(eClass) && marshallerUseFactory(eClass) ?
new NewInstanceObjectFactory(eClass) :
NullObjectFactory.of();
if (concreteClass(eClass) && Byteable.class.isAssignableFrom(eClass)) {
ByteableMarshaller byteableMarshaller = ByteableMarshaller.of((Class) eClass);
sizeMarshaller(byteableMarshaller);
reader(byteableMarshaller);
interop(byteableMarshaller);
} else if (eClass == CharSequence.class || eClass == String.class) {
reader((BytesReader) CharSequenceReader.of());
writer((BytesWriter) CharSequenceWriter.instance());
} else if (eClass == StringBuilder.class) {
reader((BytesReader) CharSequenceReader.ofStringBuilder());
writer((BytesWriter) CharSequenceWriter.instance());
} else if (eClass == Void.class) {
sizeMarshaller(VoidMarshaller.INSTANCE);
reader((BytesReader) VoidMarshaller.INSTANCE);
interop((BytesInterop) VoidMarshaller.INSTANCE);
} else if (eClass == Long.class) {
sizeMarshaller(LongMarshaller.INSTANCE);
reader((BytesReader) LongMarshaller.INSTANCE);
interop((BytesInterop) LongMarshaller.INSTANCE);
} else if (eClass == Double.class) {
sizeMarshaller(DoubleMarshaller.INSTANCE);
reader((BytesReader) DoubleMarshaller.INSTANCE);
interop((BytesInterop) DoubleMarshaller.INSTANCE);
} else if (eClass == Integer.class) {
sizeMarshaller(IntegerMarshaller.INSTANCE);
reader((BytesReader) IntegerMarshaller.INSTANCE);
interop((BytesInterop) IntegerMarshaller.INSTANCE);
} else if (eClass == byte[].class) {
reader((BytesReader) ByteArrayMarshaller.INSTANCE);
interop((BytesInterop) ByteArrayMarshaller.INSTANCE);
} else if (eClass == char[].class) {
reader((BytesReader) CharArrayMarshaller.INSTANCE);
interop((BytesInterop) CharArrayMarshaller.INSTANCE);
} else if (eClass == ByteBuffer.class) {
reader((BytesReader) ByteBufferMarshaller.INSTANCE);
writer((BytesWriter) ByteBufferMarshaller.INSTANCE);
} else if (concreteClass(eClass)) {
BytesMarshaller marshaller = chooseMarshaller(eClass, eClass);
if (marshaller != null)
marshaller(marshaller);
}
if (concreteClass(eClass) && marshallerUseFactory(eClass)) {
factory(factory);
}
}
private void writeObject(ObjectOutputStream out) throws IOException {
out.writeBoolean(serializesGeneratedClasses);
if (serializesGeneratedClasses) {
out.writeObject(eClass);
out.writeObject(role);
}
out.defaultWriteObject();
}
private void readObject(ObjectInputStream in) throws IOException, ClassNotFoundException {
boolean generatedClassesSerialized = in.readBoolean();
if (generatedClassesSerialized) {
Class eClass = (Class) in.readObject();
Role role = (Role) in.readObject();
new SerializationBuilder(eClass, role);
}
in.defaultReadObject();
}
boolean possibleOffHeapReferences() {
if (reader instanceof CharSequenceReader)
return false;
if (reader instanceof VoidMarshaller)
return false;
// exclude some known classes, most notably boxed primitive types
if (!instancesAreMutable(eClass))
return false;
if (eClass.isArray() && (eClass.getComponentType().isPrimitive() ||
instancesAreMutable(eClass.getComponentType())))
return false;
BytesMarshaller marshallerAsReader = BytesReaders.getBytesMarshaller(reader);
if (marshallerAsReader instanceof SerializableMarshaller ||
marshallerAsReader instanceof ExternalizableMarshaller)
return false;
// otherwise reader could possibly keep the given bytes addresses and update off-heap memory
return true;
}
@SuppressWarnings("unchecked")
private BytesMarshaller chooseMarshaller(Class eClass, Class classForMarshaller) {
if (BytesMarshallable.class.isAssignableFrom(eClass))
return new BytesMarshallableMarshaller(classForMarshaller);
if (Externalizable.class.isAssignableFrom(eClass))
return new ExternalizableMarshaller(classForMarshaller);
return null;
}
private SerializationBuilder copyingInterop(CopyingInterop copyingInterop) {
this.copyingInterop = copyingInterop;
return this;
}
public SerializationBuilder interop(BytesInterop interop) {
return copyingInterop(null)
.setInterop(interop)
.metaInterop(DelegatingMetaBytesInterop.>instance())
.metaInteropProvider(DelegatingMetaBytesInteropProvider
.>instance());
}
public SerializationBuilder writer(BytesWriter writer) {
if (writer instanceof BytesInterop)
return interop((BytesInterop) writer);
return copyingInterop(CopyingInterop.FROM_WRITER)
.setInterop(writer)
.metaInterop(CopyingMetaBytesInterop
.>forBytesWriter(role));
}
public SerializationBuilder marshaller(BytesMarshaller super E> marshaller) {
return copyingInterop(CopyingInterop.FROM_MARSHALLER)
.reader(BytesReaders.fromBytesMarshaller(marshaller))
.setInterop(marshaller)
.metaInterop(CopyingMetaBytesInterop
.>forBytesMarshaller(role));
}
public SerializationBuilder maxSize(long maxSize) {
if (copyingInterop == CopyingInterop.FROM_MARSHALLER) {
this.maxSize = maxSize;
metaInteropProvider(CopyingMetaBytesInterop
.>providerForBytesMarshaller(
instancesAreMutable, maxSize));
} else if (copyingInterop == CopyingInterop.FROM_WRITER) {
this.maxSize = maxSize;
metaInteropProvider(CopyingMetaBytesInterop
.>providerForBytesWriter(instancesAreMutable));
}
return this;
}
public SerializationBuilder constantSizeBySample(E sampleObject) {
Object originalInterop = this.interop;
Provider interopProvider = Provider.of(originalInterop.getClass());
ThreadLocalCopies copies = interopProvider.getCopies(null);
Object interop = interopProvider.get(copies, originalInterop);
copies = metaInteropProvider.getCopies(copies);
MetaBytesWriter metaInterop;
if (maxSize > 0) {
// this loop is very dumb: tries to find buffer size, sufficient to serialize
// the object, by trying to serialize to small buffers first and doubling the buffer
// size on _any_ Exception (it may be IllegalArgument, IndexOutOfBounds, IllegalState,
// see JLANG-17 issue).
// TODO The question is: couldn't this cause JVM crash without throwing an exception?
findSufficientSerializationSize:
while (true) {
MetaProvider metaInteropProvider = this.metaInteropProvider;
try {
metaInterop = metaInteropProvider.get(
copies, this.metaInterop, interop, sampleObject);
break findSufficientSerializationSize;
} catch (Exception e) {
CopyingMetaBytesInterop.checkMaxSizeStillReasonable(maxSize, e);
maxSize(maxSize * 2);
}
}
} else {
MetaProvider metaInteropProvider = this.metaInteropProvider;
metaInterop = metaInteropProvider.get(copies, this.metaInterop, interop, sampleObject);
}
long constantSize = metaInterop.size(interop, sampleObject);
if (sizeIsStaticallyKnown) {
long expectedConstantSize = pseudoReadConstantSize();
if (constantSize != expectedConstantSize) {
throw new IllegalStateException("Although configuring constant size by sample " +
"is not forbidden for types which size we already know statically, they " +
"should be the same. For " + eClass + " we know constant size is " +
expectedConstantSize + " statically, configured sample is " + sampleObject +
" which size in serialized form is " + constantSize);
}
}
// set max size once more, because current maxSize could be x64 or x2 larger than needed
maxSize(constantSize);
sizeMarshaller(net.openhft.chronicle.hash.serialization.SizeMarshallers.constant(constantSize));
return this;
}
public SerializationBuilder objectSerializer(ObjectSerializer serializer) {
if (reader == null || interop == null) {
marshaller(new SerializableMarshaller(serializer, eClass));
}
return this;
}
public SerializationBuilder instancesAreMutable(boolean mutable) {
this.instancesAreMutable = mutable;
return this;
}
public SizeMarshaller sizeMarshaller() {
return sizeMarshaller;
}
boolean constantSizeMarshaller() {
try {
return sizeMarshaller().sizeEncodingSize(1L) == 0;
} catch (Exception e) {
// size marshaller thrown likely IllegalArgumentException, that means it doesn't
// expect size 1. marshaller of constant size shouldn't do that, it's body should be
// just `return 0;`
return false;
}
}
boolean constantSizeEncodingSizeMarshaller() {
return sizeMarshaller().minSizeEncodingSize() == sizeMarshaller().maxSizeEncodingSize();
}
long pseudoReadConstantSize() {
return sizeMarshaller().readSize(EMPTY_BYTES);
}
public SerializationBuilder sizeMarshaller(SizeMarshaller sizeMarshaller) {
this.sizeMarshaller = sizeMarshaller;
return this;
}
public BytesReader reader() {
return reader;
}
public SerializationBuilder reader(BytesReader reader) {
this.reader = reader;
return this;
}
public Object interop() {
return interop;
}
private SerializationBuilder setInterop(Object interop) {
this.interop = interop;
return this;
}
public MetaBytesInterop metaInterop() {
return metaInterop;
}
private SerializationBuilder metaInterop(MetaBytesInterop metaInterop) {
this.metaInterop = metaInterop;
return this;
}
@SuppressWarnings("unchecked")
public MetaProvider> metaInteropProvider() {
return (MetaProvider>) metaInteropProvider;
}
private SerializationBuilder metaInteropProvider(
MetaProvider metaInteropProvider) {
this.metaInteropProvider = metaInteropProvider;
return this;
}
@SuppressWarnings("unchecked")
public SerializationBuilder factory(ObjectFactory factory) {
if (reader instanceof DeserializationFactoryConfigurableBytesReader) {
DeserializationFactoryConfigurableBytesReader newReader =
((DeserializationFactoryConfigurableBytesReader) reader)
.withDeserializationFactory(factory);
reader(newReader);
if (newReader instanceof BytesInterop)
interop((BytesInterop) newReader);
if (newReader instanceof SizeMarshaller)
sizeMarshaller((SizeMarshaller) newReader);
return this;
}
if (!marshallerUseFactory(eClass)) {
throw new IllegalStateException("Default marshaller for " + eClass +
" value don't use object factory");
} else if (interop instanceof BytesMarshallableMarshaller) {
if (factory instanceof AllocateInstanceObjectFactory) {
interop = new BytesMarshallableMarshaller(
((AllocateInstanceObjectFactory) factory).allocatedClass());
} else {
interop = new BytesMarshallableMarshallerWithCustomFactory(
((BytesMarshallableMarshaller) interop).marshaledClass(),
factory
);
}
} else if (interop instanceof ExternalizableMarshaller) {
if (factory instanceof AllocateInstanceObjectFactory) {
interop = new ExternalizableMarshaller(
((AllocateInstanceObjectFactory) factory).allocatedClass());
} else {
interop = new ExternalizableMarshallerWithCustomFactory(
((ExternalizableMarshaller) interop).marshaledClass(),
factory
);
}
} else {
// interop is custom, it is user's responsibility to use the same factory inside
// marshaller and standalone
String role = this.role.toString().toLowerCase();
throw new IllegalStateException(
role + "DeserializationFactory() should be called " +
"only if the Map " + role + " type is Byteable, BytesMarshallable " +
"or Externalizable subtype and no custom marshallers were configured.");
}
return this;
}
@Override
public SerializationBuilder clone() {
try {
return (SerializationBuilder) super.clone();
} catch (CloneNotSupportedException e) {
throw new AssertionError(e);
}
}
private static class SerializableMarshaller implements BytesMarshaller {
private static final long serialVersionUID = 0L;
private final ObjectSerializer serializer;
private final Class expectedClass;
private SerializableMarshaller(ObjectSerializer serializer, Class expectedClass) {
this.serializer = serializer;
this.expectedClass = expectedClass;
}
@Override
public void write(Bytes bytes, Object obj) {
try {
serializer.writeSerializable(bytes, obj, expectedClass);
} catch (IOException e) {
throw new IllegalStateException(e);
}
}
@Nullable
@Override
public Object read(Bytes bytes) {
try {
return serializer.readSerializable(bytes, null, null);
} catch (IOException e) {
throw new IllegalStateException(e);
} catch (ClassNotFoundException e) {
throw new IllegalStateException(e);
}
}
@Nullable
@Override
public Object read(Bytes bytes, @Nullable Object obj) {
try {
return serializer.readSerializable(bytes, null, obj);
} catch (IOException e) {
throw new IllegalStateException(e);
} catch (ClassNotFoundException e) {
throw new IllegalStateException(e);
}
}
}
private static class BytesMarshallableMarshallerWithCustomFactory
extends BytesMarshallableMarshaller {
private static final long serialVersionUID = 0L;
@NotNull
private final ObjectFactory factory;
BytesMarshallableMarshallerWithCustomFactory(@NotNull Class tClass,
@NotNull ObjectFactory factory) {
super(tClass);
this.factory = factory;
}
@NotNull
@Override
protected T getInstance() throws Exception {
return factory.create();
}
@Override
public boolean equals(Object obj) {
if (obj == null || obj.getClass() != getClass())
return false;
BytesMarshallableMarshallerWithCustomFactory that =
(BytesMarshallableMarshallerWithCustomFactory) obj;
return that.marshaledClass() == marshaledClass() && that.factory.equals(this.factory);
}
@Override
public int hashCode() {
return hash(marshaledClass(), factory);
}
@Override
public String toString() {
return getClass().getSimpleName() + "{marshaledClass=" + marshaledClass() +
",factory=" + factory + "}";
}
}
private static class ExternalizableMarshallerWithCustomFactory
extends ExternalizableMarshaller {
private static final long serialVersionUID = 0L;
@NotNull
private final ObjectFactory factory;
ExternalizableMarshallerWithCustomFactory(@NotNull Class tClass,
@NotNull ObjectFactory factory) {
super(tClass);
this.factory = factory;
}
@NotNull
@Override
protected T getInstance() throws Exception {
return factory.create();
}
@Override
public boolean equals(Object obj) {
if (obj == null || obj.getClass() != getClass())
return false;
ExternalizableMarshallerWithCustomFactory that =
(ExternalizableMarshallerWithCustomFactory) obj;
return that.marshaledClass() == marshaledClass() && that.factory.equals(this.factory);
}
@Override
public int hashCode() {
return hash(marshaledClass(), factory);
}
@Override
public String toString() {
return getClass().getSimpleName() + "{marshaledClass=" + marshaledClass() +
",factory=" + factory + "}";
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy