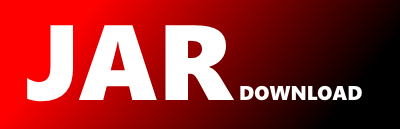
net.openhft.chronicle.set.SetFromMap Maven / Gradle / Ivy
/*
* Copyright (C) 2015 higherfrequencytrading.com
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*/
package net.openhft.chronicle.set;
import net.openhft.chronicle.map.ChronicleMap;
import java.io.File;
import java.io.IOException;
import java.io.Serializable;
import java.util.AbstractSet;
import java.util.Collection;
import java.util.Iterator;
import java.util.Set;
import static net.openhft.chronicle.set.DummyValue.DUMMY_VALUE;
class SetFromMap extends AbstractSet
implements ChronicleSet, Serializable {
private final ChronicleMap m; // The backing map
private transient Set s; // Its keySet
SetFromMap(ChronicleMap map) {
m = map;
s = map.keySet();
}
public void clear() {
m.clear();
}
public int size() {
return m.size();
}
public boolean isEmpty() {
return m.isEmpty();
}
public boolean contains(Object o) {
return m.containsKey(o);
}
public boolean remove(Object o) {
return m.remove(o, DUMMY_VALUE);
}
public boolean add(E e) {
return m.putIfAbsent(e, DUMMY_VALUE) == null;
}
public Iterator iterator() {
return s.iterator();
}
public Object[] toArray() {
return s.toArray();
}
public T[] toArray(T[] a) {
return s.toArray(a);
}
public String toString() {
return s.toString();
}
public int hashCode() {
return s.hashCode();
}
public boolean equals(Object o) {
return o == this || s.equals(o);
}
// TODO optimize in case of stateless clients -- because bulk ops are optimized on maps
// but on key set they delegate to individual element queries => individual remote calls
public boolean containsAll(Collection> c) {
return s.containsAll(c);
}
public boolean removeAll(Collection> c) {
return s.removeAll(c);
}
public boolean retainAll(Collection> c) {
return s.retainAll(c);
}
// addAll is the only inherited implementation
private static final long serialVersionUID = 2454657854757543876L;
private void readObject(java.io.ObjectInputStream stream)
throws IOException, ClassNotFoundException {
stream.defaultReadObject();
s = m.keySet();
}
@Override
public long longSize() {
return m.longSize();
}
@Override
public File file() {
return m.file();
}
@Override
public void close() {
m.close();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy