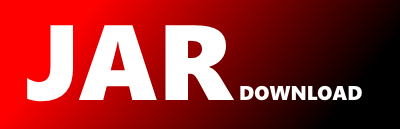
net.openhft.lang.io.RandomDataInput Maven / Gradle / Ivy
Show all versions of lang Show documentation
/*
* Copyright (C) 2015 higherfrequencytrading.com
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*/
package net.openhft.lang.io;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import java.io.ObjectInput;
import java.io.StreamCorruptedException;
import java.nio.ByteBuffer;
import java.util.Collection;
import java.util.Map;
import java.util.RandomAccess;
/**
* @author peter.lawrey
*/
public interface RandomDataInput extends ObjectInput, RandomAccess, BytesCommon {
/**
* Reads some bytes from an input stream and stores them into the buffer array b
. The number of bytes
* read is equal to the length of b
.
*
* This method blocks until one of the following conditions occurs:
*
*
* b.length
bytes of input data are available, in which case a normal return is made.
* - End of file is detected, in which case an
EOFException
is thrown.
* - An I/O error occurs, in which case an
IOException
other than EOFException
is thrown.
*
*
* If bytes
is null
, a NullPointerException
is thrown. If bytes.length
* is zero, then no bytes are read. Otherwise, the first byte read is stored into element bytes[0]
, the
* next one into bytes[1]
, and so on. If an exception is thrown from this method, then it may be that some
* but not all bytes of bytes
have been updated with data from the input stream.
*
*
* @param bytes the buffer into which the data is read.
*/
@Override
void readFully(@NotNull byte[] bytes);
/**
* Reads len
bytes from an input stream.
*
* This method blocks until one of the following conditions occurs:
*
*
* len
bytes of input data are available, in which case a normal return is made.
* - End of file is detected, in which case an
EOFException
is thrown.
* - An I/O error occurs, in which case an
IOException
other than EOFException
is thrown.
*
*
* If bytes
is null
, a NullPointerException
is thrown. If off
is
* negative, or len
is negative, or off+len
is greater than the length of the array
* bytes
, then an IndexOutOfBoundsException
is thrown. If len
is zero, then no
* bytes are read. Otherwise, the first byte read is stored into element bytes[off]
, the next one into
* bytes[off+1]
, and so on. The number of bytes read is, at most, equal to len
.
*
*
* @param bytes the buffer into which the data is read.
* @param off an int specifying the offset into the data.
* @param len an int specifying the number of bytes to read.
*/
@Override
void readFully(@NotNull byte[] bytes, int off, int len);
void readFully(long offset, @NotNull byte[] bytes, int off, int len);
void readFully(@NotNull char[] data);
void readFully(@NotNull char[] data, int off, int len);
/**
* Makes an attempt to skip over n
bytes of data from the input stream, discarding the skipped bytes.
* However, it may skip over some smaller number of bytes, possibly zero. This may result from any of a number of
* conditions; reaching end of file before n
bytes have been skipped is only one possibility. This
* method never throws an EOFException
. The actual number of bytes skipped is returned.
*
* @param n the number of bytes to be skipped.
* @return the number of bytes actually skipped.
*/
@Override
int skipBytes(int n);
/**
* Reads one input byte and returns true
if that byte is nonzero, false
if that byte is
* zero. This method is suitable for reading the byte written by the writeBoolean
method of interface
* DataOutput
.
*
* @return the boolean
value read.
*/
@Override
boolean readBoolean();
/**
* Reads one input byte and returns true
if that byte is nonzero, false
if that byte is
* zero. This method is suitable for reading the byte written by the writeBoolean
method of interface
* RandomDataOutput
.
*
* @param offset to read byte translated into a boolean
* @return the boolean
value read.
*/
boolean readBoolean(long offset);
/**
* Reads and returns one input byte. The byte is treated as a signed value in the range -128
through
* 127
, inclusive. This method is suitable for reading the byte written by the writeByte
* method of interface DataOutput
.
*
* @return the 8-bit value read.
*/
@Override
byte readByte();
/**
* Reads and returns one input byte. The byte is treated as a signed value in the range -128
through
* 127
, inclusive. This method is suitable for reading the byte written by the writeByte
* method of interface RandomDataOutput
.
*
* @param offset of byte to read.
* @return the 8-bit value read.
*/
byte readByte(long offset);
/**
* Reads one input byte, zero-extends it to type int
, and returns the result, which is therefore in the
* range 0
through 255
. This method is suitable for reading the byte written by the
* writeByte
method of interface DataOutput
if the argument to writeByte
was
* intended to be a value in the range 0
through 255
.
*
* @return the unsigned 8-bit value read.
*/
@Override
int readUnsignedByte();
/**
* Reads one input byte, zero-extends it to type int
, and returns the result, which is therefore in the
* range 0
through 255
. This method is suitable for reading the byte written by the
* writeByte
method of interface RandomDataOutput
if the argument to
* writeByte
was intended to be a value in the range 0
through 255
.
*
* @param offset of byte to read
* @return the unsigned 8-bit value read.
*/
int readUnsignedByte(long offset);
/**
* Reads two input bytes and returns a short
value. Let a
be the first byte read and
* b
be the second byte on big endian machines, and the opposite on little endian machines. The value
* returned is:
* (short)((a << 8) | (b & 0xff))
*
* This method is suitable for reading the bytes written by the writeShort
method of interface
* DataOutput
.
*
* @return the 16-bit value read.
*/
@Override
short readShort();
/**
* Reads two input bytes and returns a short
value. Let a
be the first byte read and
* b
be the second byte on big endian machines, and the opposite on little endian machines. The value
* returned is:
*
* (short)((a << 8) | (b & 0xff))
*
* This method is suitable for reading the bytes written by the writeShort
method of interface
* RandomDataOutput
.
*
* @param offset of short to read.
* @return the 16-bit value read.
*/
short readShort(long offset);
/**
* Reads two input bytes and returns an int
value in the range 0
through
* 65535
. Let a
be the first byte read and b
be the second byte on big endian
* machines, and the opposite on little endian machines. The value returned is:
*
* (((a & 0xff) << 8) | (b & 0xff))
*
* This method is suitable for reading the bytes written by the writeUnsignedShort
method of interface
* DataOutput
if the argument to writeUnsignedShort
was intended to be a value in the
* range 0
through 65535
.
*
* @return the unsigned 16-bit value read.
*/
@Override
int readUnsignedShort();
/**
* Reads two input bytes and returns an int
value in the range 0
through
* 65535
. Let a
be the first byte read and b
be the second byte on big endian
* machines, and the opposite on little endian machines. The value returned is:
*
* (((a & 0xff) << 8) | (b & 0xff))
*
* This method is suitable for reading the bytes written by the writeShort
method of interface
* RandomDataOutput
if the argument to writeUnsignedShort
was intended to be a value in
* the range 0
through 65535
.
*
* @param offset of short to read.
* @return the unsigned 16-bit value read.
*/
int readUnsignedShort(long offset);
/**
* Reads one or three input bytes and returns a short
value. Let a
be the first byte read.
* This mapped as follows; Byte.MIN_VALUE => Short.MIN_VALUE, Byte.MAX_VALUE => Short.MAX_VALUE, Byte.MIN_VALUE+2 to
* Byte.MAX_VALUE-1 => same as short value, Byte.MIN_VALUE+1 => readShort().
*
* This method is suitable for reading the bytes written by the writeCompactShort
method of interface
* RandomDataOutput
.
*
* @return the 16-bit value read.
*/
short readCompactShort();
/**
* Reads one or three input bytes and returns a short
value. Let a
be the first byte read.
* This mapped as follows; -1 => readUnsignedShort(), default => (a & 0xFF)
*
*
This method is suitable for reading the bytes written by the writeCompactUnsignedShort
method of
* interface RandomDataOutput
.
*
* @return the unsigned 16-bit value read.
*/
int readCompactUnsignedShort();
/**
* Reads two input bytes and returns a char
value. Let a
be the first byte read and
* b
be the second byte on big endian machines, and the opposite on little endian machines. The value
* returned is:
*
(char)((a << 8) | (b & 0xff))
*
* This method is suitable for reading bytes written by the writeChar
method of interface
* DataOutput
.
*
* @return the char
value read.
*/
@Override
char readChar();
/**
* Reads two input bytes and returns a char
value. Let a
be the first byte read and
* b
be the second byte on big endian machines, and the opposite on little endian machines. The value
* returned is:
* (char)((a << 8) | (b & 0xff))
*
* This method is suitable for reading bytes written by the writeChar
method of interface
* RandomDataOutput
.
*
* @param offset of the char to read.
* @return the char
value read.
*/
char readChar(long offset);
/**
* Reads three input bytes and returns a 24-bit int
value. Let a-c
be the first through
* third bytes read on big endian machines, and the opposite on little endian machines. The value returned is:
*
*
* ((((a & 0xff) << 24) | ((b & 0xff) << 16) | ((c & 0xff) << 8))) >> 8
*
* This method is suitable for reading bytes written by the writeInt24
method of interface
* RandomDataOutput
.
*
* @return the int
value read.
*/
int readInt24();
/**
* Reads three input bytes and returns a 24-bit int
value. Let a-c
be the first through
* third bytes read on big endian machines, and the opposite on little endian machines. The value returned is:
*
*
* ((((a & 0xff) << 24) | ((b & 0xff) << 16) | ((c & 0xff) << 8))) >> 8
*
* This method is suitable for reading bytes written by the writeInt24
method of interface
* RandomDataOutput
.
*
* @param offset to read from
* @return the int
value read.
*/
int readInt24(long offset);
/**
* Reads four input bytes and returns an int
value. Let a-d
be the first through fourth
* bytes read on big endian machines, and the opposite on little endian machines. The value returned is:
*
*
* (((a & 0xff) << 24) | ((b & 0xff) << 16) | ((c & 0xff) << 8) | (d & 0xff))
*
* This method is suitable for reading bytes written by the writeInt
method of interface
* DataOutput
.
*
* @return the int
value read.
*/
@Override
int readInt();
/**
* Reads four input bytes and returns an int
value. Let a-d
be the first through fourth
* bytes read on big endian machines, and the opposite on little endian machines. The value returned is:
*
*
* (((a & 0xff) << 24) | ((b & 0xff) << 16) | ((c & 0xff) << 8) | (d & 0xff))
*
* This method is suitable for reading bytes written by the writeInt
method of interface
* RandomDataOutput
.
*
* @param offset to read from
* @return the int
value read.
*/
int readInt(long offset);
/**
* This is the same as readInt() except a read barrier is performed first. Reads four input bytes and returns
* an int
value. Let a-d
be the first through fourth bytes read on big endian machines,
* and the opposite on little endian machines. The value returned is:
*
*
* (((a & 0xff) << 24) | ((b & 0xff) << 16) | ((c & 0xff) << 8) | (d & 0xff))
*
* This method is suitable for reading bytes written by the writeOrderedInt
or
* writeVolatileInt
method of interface RandomDataOutput
.
*
* @return the int
value read.
*/
int readVolatileInt();
/**
* This is the same as readInt() except a read barrier is performed first. Reads four input bytes and returns
* an int
value. Let a-d
be the first through fourth bytes read on big endian machines,
* and the opposite on little endian machines. The value returned is:
*
*
* (((a & 0xff) << 24) | ((b & 0xff) << 16) | ((c & 0xff) << 8) | (d & 0xff))
*
* This method is suitable for reading bytes written by the writeOrderedInt
or
* writeVolatileInt
method of interface RandomDataOutput
.
*
* @param offset to read from
* @return the int
value read.
*/
int readVolatileInt(long offset);
/**
* Reads four input bytes and returns an int
value. Let a-d
be the first through fourth
* bytes read on big endian machines, and the opposite on little endian machines. The value returned is:
*
*
* ((((long) a & 0xff) << 24) | ((b & 0xff) << 16) | ((c & 0xff) << 8) | (d &
* 0xff))
*
* This method is suitable for reading bytes written by the writeUnsignedInt
method of interface
* RandomDataOutput
.
*
* @return the unsigned int
value read.
*/
long readUnsignedInt();
/**
* Reads four input bytes and returns an int
value. Let a-d
be the first through fourth
* bytes read on big endian machines, and the opposite on little endian machines. The value returned is:
*
*
* ((((long) a & 0xff) << 24) | ((b & 0xff) << 16) | ((c & 0xff) << 8) | (d &
* 0xff))
*
* This method is suitable for reading bytes written by the writeUnsignedInt
method of interface
* RandomDataOutput
.
*
* @param offset to read from
* @return the unsigned int
value read.
*/
long readUnsignedInt(long offset);
/**
* Reads two or six input bytes and returns an int
value. Let a
be the first short read
* with readShort(). This mapped as follows; Short.MIN_VALUE => Integer.MIN_VALUE, Short.MAX_VALUE =>
* Integer.MAX_VALUE, Short.MIN_VALUE+2 to Short.MAX_VALUE-1 => same as short value, Short.MIN_VALUE+1 =>
* readInt().
*
* This method is suitable for reading the bytes written by the writeCompactInt
method of interface
* RandomDataOutput
.
*
* @return the 32-bit value read.
*/
int readCompactInt();
/**
* Reads two or six input bytes and returns an int
value. Let a
be the first short read
* with readShort(). This mapped as follows; -1 => readUnsignedInt(), default => (a & 0xFFFF)
*
*
This method is suitable for reading the bytes written by the writeCompactUnsignedInt
method of
* interface RandomDataOutput
.
*
* @return the unsigned 32-bit value read.
*/
long readCompactUnsignedInt();
/**
* Reads eight input bytes and returns a long
value. Let a-h
be the first through eighth
* bytes read on big endian machines, and the opposite on little endian machines. The value returned is:
*
* (((long)(a & 0xff) << 56) |
* ((long)(b & 0xff) << 48) |
* ((long)(c & 0xff) << 40) |
* ((long)(d & 0xff) << 32) |
* ((long)(e & 0xff) << 24) |
* ((long)(f & 0xff) << 16) |
* ((long)(g & 0xff) << 8) |
* ((long)(h & 0xff)))
*
*
* This method is suitable for reading bytes written by the writeLong
method of interface
* DataOutput
.
*
* @return the long
value read.
*/
@Override
long readLong();
/**
* Same as readLong except the remaining() can be less than 8.
*
* @param offset base
* @return long.
*/
long readIncompleteLong(long offset);
/**
* Reads eight input bytes and returns a long
value. Let a-h
be the first through eighth
* bytes read on big endian machines, and the opposite on little endian machines. The value returned is:
*
* (((long)(a & 0xff) << 56) |
* ((long)(b & 0xff) << 48) |
* ((long)(c & 0xff) << 40) |
* ((long)(d & 0xff) << 32) |
* ((long)(e & 0xff) << 24) |
* ((long)(f & 0xff) << 16) |
* ((long)(g & 0xff) << 8) |
* ((long)(h & 0xff)))
*
*
* This method is suitable for reading bytes written by the writeLong
method of interface
* RandomDataOutput
.
*
* @param offset of the long to read
* @return the long
value read.
*/
long readLong(long offset);
/**
* This is the same readLong() except a dread barrier is performed first
*
*
Reads eight input bytes and returns a long
value. Let a-h
be the first through eighth
* bytes read on big endian machines, and the opposite on little endian machines. The value returned is:
*
* (((long)(a & 0xff) << 56) |
* ((long)(b & 0xff) << 48) |
* ((long)(c & 0xff) << 40) |
* ((long)(d & 0xff) << 32) |
* ((long)(e & 0xff) << 24) |
* ((long)(f & 0xff) << 16) |
* ((long)(g & 0xff) << 8) |
* ((long)(h & 0xff)))
*
*
* This method is suitable for reading bytes written by the writeOrderedLong
or
* writeVolatileLong
method of interface RandomDataOutput
.
*
* @return the long
value read.
*/
long readVolatileLong();
/**
* This is the same readLong() except a dread barrier is performed first
*
*
Reads eight input bytes and returns a long
value. Let a-h
be the first through eighth
* bytes read on big endian machines, and the opposite on little endian machines. The value returned is:
*
* (((long)(a & 0xff) << 56) |
* ((long)(b & 0xff) << 48) |
* ((long)(c & 0xff) << 40) |
* ((long)(d & 0xff) << 32) |
* ((long)(e & 0xff) << 24) |
* ((long)(f & 0xff) << 16) |
* ((long)(g & 0xff) << 8) |
* ((long)(h & 0xff)))
*
*
* This method is suitable for reading bytes written by the writeOrderedLong
or
* writeVolatileLong
method of interface RandomDataOutput
.
*
* @param offset of the long to read
* @return the long
value read.
*/
long readVolatileLong(long offset);
/**
* Reads six input bytes and returns a long
value. Let a-f
be the first through sixth
* bytes read on big endian machines, and the opposite on little endian machines. The value returned is:
*
* (((long)(a & 0xff) << 56) |
* ((long)(b & 0xff) << 48) |
* ((long)(c & 0xff) << 40) |
* ((long)(d & 0xff) << 32) |
* ((long)(e & 0xff) << 24) |
* ((long)(f & 0xff) << 16)) >> 16
*
*
* This method is suitable for reading bytes written by the writeInt48
method of interface
* RandomDataOutput
.
*
* @return the long
value read.
*/
long readInt48();
/**
* Reads six input bytes and returns a long
value. Let a-f
be the first through sixth
* bytes read on big endian machines, and the opposite on little endian machines. The value returned is:
*
* (((long)(a & 0xff) << 56) |
* ((long)(b & 0xff) << 48) |
* ((long)(c & 0xff) << 40) |
* ((long)(d & 0xff) << 32) |
* ((long)(e & 0xff) << 24) |
* ((long)(f & 0xff) << 16)) >> 16
*
*
* This method is suitable for reading bytes written by the writeInt48
method of interface
* RandomDataOutput
.
*
* @param offset of the long to read
* @return the long
value read.
*/
long readInt48(long offset);
/**
* Reads four or twelve input bytes and returns a long
value. Let a
be the first int read
* with readInt(). This mapped as follows; Integer.MIN_VALUE => Long.MIN_VALUE, Integer.MAX_VALUE => Long.MAX_VALUE,
* Integer.MIN_VALUE+2 to Integer.MAX_VALUE-1 => same as short value, Integer.MIN_VALUE+1 => readLong().
*
*
This method is suitable for reading the bytes written by the writeCompactLong
method of interface
* RandomDataOutput
.
*
* @return the 64-bit value read.
*/
long readCompactLong();
/**
* Reads between one and ten bytes with are stop encoded with support for negative numbers
*
* long l = 0, b;
* int count = 0;
* while ((b = readByte()) < 0) {
* l |= (b & 0x7FL) << count;
* count += 7;
* }
* if (b == 0 && count > 0)
* return ~l;
* return l | (b << count);
*
*
* @return a stop bit encoded number as a long.
*/
long readStopBit();
/**
* Reads four input bytes and returns a float
value. It does this by first constructing an
* int
value in exactly the manner of the readInt
method, then converting this
* int
value to a float
in exactly the manner of the method
* Float.intBitsToFloat
. This method is suitable for reading bytes written by the
* writeFloat
method of interface DataOutput
.
*
* @return the float
value read.
*/
@Override
float readFloat();
/**
* Reads four input bytes and returns a float
value. It does this by first constructing an
* int
value in exactly the manner of the readInt
method, then converting this
* int
value to a float
in exactly the manner of the method
* Float.intBitsToFloat
. This method is suitable for reading bytes written by the
* writeFloat
method of interface DataOutput
.
*
* @param offset to read from
* @return the float
value read.
*/
float readFloat(long offset);
/**
* This is the same as readFloat() except a read barrier is performed first. Reads four input bytes and returns
* a float
value.
*
*
This method is suitable for reading bytes written by the writeOrderedFloat
method of interface RandomDataOutput
.
*
* @param offset to read from
* @return the int
value read.
*/
float readVolatileFloat(long offset);
/**
* Reads eight input bytes and returns a double
value. It does this by first constructing a
* long
value in exactly the manner of the readLong
method, then converting this
* long
value to a double
in exactly the manner of the method
* Double.longBitsToDouble
. This method is suitable for reading bytes written by the
* writeDouble
method of interface DataOutput
.
*
* @return the double
value read.
*/
@Override
double readDouble();
/**
* Reads eight input bytes and returns a double
value. It does this by first constructing a
* long
value in exactly the manner of the readLong
method, then converting this
* long
value to a double
in exactly the manner of the method
* Double.longBitsToDouble
. This method is suitable for reading bytes written by the
* writeDouble
method of interface DataOutput
.
*
* @param offset to read from
* @return the double
value read.
*/
double readDouble(long offset);
/**
* Reads the first four bytes as readFloat(). If this is Float.NaN, the next eight bytes are read as readDouble()
*
* @return the double
value read.
*/
double readCompactDouble();
/**
* This is the same as readDouble() except a read barrier is performed first.
Reads four input bytes and returns
* a float
value.
*
*
This method is suitable for reading bytes written by the writeOrderedFloat
method of interface RandomDataOutput
.
*
* @param offset to read from
* @return the int
value read.
*/
double readVolatileDouble(long offset);
/**
* Reads the next line of text from the input stream. It reads successive bytes, converting each byte separately
* into a character, until it encounters a line terminator or end of file; the characters read are then returned as
* a String
. Note that because this method processes bytes, it does not support input of the full
* Unicode character set.
*
*
If end of file is encountered before even one byte can be read, then null
is returned. Otherwise,
* each byte that is read is converted to type char
by zero-extension. If the character
* '\n'
is encountered, it is discarded and reading ceases. If the character '\r'
is
* encountered, it is discarded and, if the following byte converts to the character '\n'
, then
* that is discarded also; reading then ceases. If end of file is encountered before either of the characters
* '\n'
and '\r'
is encountered, reading ceases. Once reading has ceased, a
* String
is returned that contains all the characters read and not discarded, taken in order. Note
* that every character in this string will have a value less than \u0100
, that is,
* (char)256
.
*
* @return the next line of text from the input stream, or null
if the end of file is encountered
* before a byte can be read.
*/
@Override
@Nullable
String readLine();
/**
* Reads in a string that has been encoded using a modified UTF-8 format. The general
* contract of readUTF
is that it reads a representation of a Unicode character string encoded in
* modified UTF-8 format; this string of characters is then returned as a String
.
*
*
First, two bytes are read and used to construct an unsigned 16-bit integer in exactly the manner of the
* readUnsignedShort
method . This integer value is called the UTF length and specifies the
* number of additional bytes to be read. These bytes are then converted to characters by considering them in
* groups. The length of each group is computed from the value of the first byte of the group. The byte following a
* group, if any, is the first byte of the next group.
*
*
If the first byte of a group matches the bit pattern 0xxxxxxx
(where x
means "may be
* 0
or 1
"), then the group consists of just that byte. The byte is zero-extended to form
* a character.
*
*
If the first byte of a group matches the bit pattern 110xxxxx
, then the group consists of that byte
* a
and a second byte b
. If there is no byte b
(because byte a
* was the last of the bytes to be read), or if byte b
does not match the bit pattern
* 10xxxxxx
, then a UTFDataFormatException
is thrown. Otherwise, the group is converted to
* the character:
*
(char)(((a& 0x1F) << 6) | (b & 0x3F))
*
* If the first byte of a group matches the bit pattern 1110xxxx
, then the group consists of that byte
* a
and two more bytes b
and c
. If there is no byte c
(because
* byte a
was one of the last two of the bytes to be read), or either byte b
or byte
* c
does not match the bit pattern 10xxxxxx
, then a UTFDataFormatException
* is thrown. Otherwise, the group is converted to the character:
*
* (char)(((a & 0x0F) << 12) | ((b & 0x3F) << 6) | (c & 0x3F))
*
* If the first byte of a group matches the pattern 1111xxxx
or the pattern 10xxxxxx
, then
* a UTFDataFormatException
is thrown.
*
* If end of file is encountered at any time during this entire process, then an EOFException
is
* thrown.
*
*
After every group has been converted to a character by this process, the characters are gathered, in the same
* order in which their corresponding groups were read from the input stream, to form a String
, which
* is returned.
*
*
The writeUTF
method of interface DataOutput
may be used to write data that is suitable
* for reading by this method.
*
* @return a Unicode string.
* @throws IllegalStateException if the bytes do not represent a valid modified UTF-8 encoding of a string.
*/
@Override
@NotNull
String readUTF();
/**
* The same as readUTF() except the length is stop bit encoded. This saves one byte for strings shorter than 128
* chars. null
values are also supported
*
* @return a Unicode string or null
if writeUTFΔ(null)
was called
*/
@Nullable
String readUTFΔ();
/**
* The same as readUTFΔ() except an offset is given.
*
* @param offset to read from
* @return a Unicode string or null
if writeUTFΔ(null)
was called
* @throws IllegalStateException if the length to be read is out of range.
*/
@Nullable
String readUTFΔ(long offset) throws IllegalStateException;
/**
* The same as readUTFΔ() except the chars are copied to a truncated StringBuilder.
*
* @param stringBuilder to copy chars to
* @return true
if there was a String, or false
if it was null
*/
boolean readUTFΔ(@NotNull StringBuilder stringBuilder);
boolean read8bitText(@NotNull StringBuilder stringBuilder) throws StreamCorruptedException;
/**
* Copy bytes into a ByteBuffer to the minimum of the length remaining()
in the ByteBuffer or the
* Excerpt.
*
* @param bb to copy into
*/
void read(@NotNull ByteBuffer bb);
/**
* Copy bytes into a ByteBuffer to the minimum of the length in the ByteBuffer or the
* Excerpt.
*
* @param bb to copy into
* @param length number of bytes to copy
*/
void read(@NotNull ByteBuffer bb, int length);
/**
* Read a String with readUTFΔ
which is converted to an enumerable type. i.e. where there is a one to
* one mapping between an object and it's toString().
*
*
This is suitable to read an object written using writeEnum()
in the RandomDataOutput
* interface
*
* @param the enum class
* @param eClass to decode the String as
* @return the decoded value. null
with be return if null was written.
*/
@Nullable
E readEnum(@NotNull Class eClass);
/**
* Read a stop bit encoded length and populates this Collection after zeroOut()ing it.
*
* This is suitable to reading a list written using writeList()
in the RandomDataOutput
* interface
*
* @param the list element class
* @param eClass the list element class
* @param list to populate
*/
void readList(@NotNull Collection list, @NotNull Class eClass);
/**
* Read a stop bit encoded length and populates this Map after zeroOut()ing it.
*
* This is suitable to reading a list written using writeMap()
in the RandomDataOutput
* interface
*
* @param the map key class
* @param the map value class
* @param kClass the map key class
* @param vClass the map value class
* @param map to populate
* @return the map
*/
Map readMap(@NotNull Map map, @NotNull Class kClass, @NotNull Class vClass);
// ObjectInput
/**
* Read and return an object. The class that implements this interface defines where the object is "read" from.
*
* @return the object read from the stream
* @throws IllegalStateException the class of a serialized object cannot be found or any of the usual Input/Output
* related exceptions occur.
*/
@Nullable
@Override
Object readObject() throws IllegalStateException;
/**
* Read and return an object. The class that implements this interface defines where the object is "read" from.
*
* @param the class of the object to read
* @param tClass the class of the object to read
* @return the object read from the stream
* @throws IllegalStateException the class of a serialized object cannot be found or any of the usual Input/Output
* related exceptions occur.
* @throws ClassCastException The class cannot be cast or converted to the type given.
*/
@Nullable
T readObject(Class tClass) throws IllegalStateException;
/**
* Read an instance of a class assuming objClass was provided when written.
*
* @param the class of the object to read
* @param objClass class to write
* @param obj to reuse or null if a new object is needed
* @return the object read from the stream
*/
@Nullable
T readInstance(@NotNull Class objClass, T obj);
/**
* Reads a byte of data. This method is non blocking.
*
* @return the byte read, or -1 if the end of the stream is reached.
*/
@Override
int read();
/**
* Reads into an array of bytes. This method will block until some input is available.
*
* @param bytes the buffer into which the data is read
* @return the actual number of bytes read, -1 is returned when the end of the stream is reached.
*/
@Override
int read(@NotNull byte[] bytes);
/**
* Reads into an array of bytes. This method will block until some input is available.
*
* @param bytes the buffer into which the data is read
* @param off the start offset of the data
* @param len the maximum number of bytes read
* @return the actual number of bytes read, -1 is returned when the end of the stream is reached.
*/
@Override
int read(@NotNull byte[] bytes, int off, int len);
/**
* Read the object from start to end bytes
*
* @param object to read into
* @param start byte inclusive
* @param end byte exclusive
*/
void readObject(Object object, int start, int end);
/**
* Skips n bytes of input.
*
* @param n the number of bytes to be skipped
* @return the actual number of bytes skipped.
*/
@Override
long skip(long n);
/**
* @return remaining() or Integer.MAX_VALUE if larger.
*/
@Override
int available();
/**
* Finishes the excerpt entry if not finished()
*/
@Override
void close();
boolean startsWith(RandomDataInput input);
boolean compare(long offset, RandomDataInput input, long inputOffset, long len);
E readEnum(long offset, int maxSize, Class eClass);
/**
* From a given bit index, find the next bit with is set.
*
* @param fromIndex first bit to scan.
* @return first bit equals or after it which is set.
*/
long nextSetBit(long fromIndex);
}