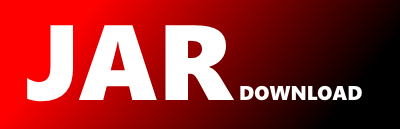
net.optionfactory.pebbel.execution.ExpressionEvaluator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pebbel Show documentation
Show all versions of pebbel Show documentation
An expression language designed for embedding in java projects
package net.optionfactory.pebbel.execution;
import java.util.Arrays;
import net.optionfactory.pebbel.ast.BooleanExpression;
import net.optionfactory.pebbel.ast.Expression;
import net.optionfactory.pebbel.ast.FunctionCall;
import net.optionfactory.pebbel.ast.NumberExpression;
import net.optionfactory.pebbel.ast.NumberLiteral;
import net.optionfactory.pebbel.ast.ShortCircuitExpression;
import net.optionfactory.pebbel.ast.StringExpression;
import net.optionfactory.pebbel.ast.StringLiteral;
import net.optionfactory.pebbel.ast.Variable;
import net.optionfactory.pebbel.execution.Function.ExecutionException;
import net.optionfactory.pebbel.loading.Symbols;
import net.optionfactory.pebbel.results.Result;
/**
* An AST visitor evaluating an expression.
*/
public class ExpressionEvaluator implements Expression.Visitor
© 2015 - 2025 Weber Informatics LLC | Privacy Policy