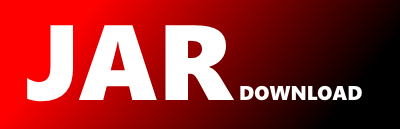
com.simple.orm.dao.Query Maven / Gradle / Ivy
package com.simple.orm.dao;
import java.io.Serializable;
import java.sql.SQLException;
import java.util.List;
public interface Query {
/**
* 根据主键属性查询一条数据
* @param id 主键的值
* @return 返回数据表的映射对象
* @throws SQLException 抛出数据库异常
*/
M find(Serializable id) throws SQLException;
/**
* 根据属性值查询一条数据
* @param property 属性名称
* @param value 对应的属性值
* @return 返回数据表的映射对象
* @throws SQLException 抛出数据库异常
*/
M find(String property,Object value) throws SQLException;
/**
* 根据属性值查询
* @param property 属性名称
* @param value 对应的属性值
* @return 返回数据表的映射对象列表
* @throws SQLException 抛出数据库异常
*/
List query(String property,Object value) throws SQLException;
/**
* 根据属性值分页查询
* @param property 属性名称
* @param value 对应的属性值
* @param start 开始索引(limit start,num)
* @param num 最大查询数量
* @return 返回数据表的映射对象列表
* @throws SQLException 抛出数据库异常
*/
List query(String property,Object value,Integer start,Integer num) throws SQLException;
/**
* 查询所有
* @return 返回数据表的映射对象列表
* @throws SQLException 抛出数据库异常
*/
List query() throws SQLException;
/**
* 根据对象分页查询
* @param m 映射的对象
* @param start 开始索引(limit start,num)
* @param num 最大查询数量
* @return 返回数据表的映射对象列表
* @throws SQLException 抛出数据库异常
*/
List query(M m,Integer start,Integer num) throws SQLException;
/**
* 根据分页查询所有
* @param start 开始索引(limit start,num)
* @param num 最大查询数量
* @return 返回数据表的映射对象列表
* @throws SQLException 抛出数据库异常
*/
List query(Integer start,Integer num) throws SQLException;
/**
* 根据对象设置的属性查询
* @param m 映射的对象
* @return 返回数据表的映射对象列表
* @throws SQLException 抛出数据库异常
*/
List query(M m) throws SQLException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy