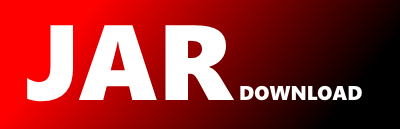
com.simple.orm.service.BaseService Maven / Gradle / Ivy
package com.simple.orm.service;
import java.io.Serializable;
import java.sql.SQLException;
import java.util.List;
import java.util.Map;
import org.springframework.dao.DataAccessException;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import com.simple.orm.dao.Query;
public interface BaseService {
/**
* 设置某个属性排序
* @param property 属性名称
* @param by ASC|DESC
* @return 返回查询对象
* @throws SQLException 抛出数据库异常
*/
Query setOrderBy(String property,String by) throws SQLException;
/**
* 根据主键属性查询一条数据
* @param id 主键的值
* @return 返回数据表的映射对象
* @throws SQLException 抛出数据库异常
*/
M find(Serializable id) throws SQLException;
/**
* 根据属性值查询一条数据
* @param property 属性名称
* @param value 对应的属性值
* @return 返回数据表的映射对象
* @throws SQLException 抛出数据库异常
*/
M find(String property,Object value) throws SQLException;
/**
* 根据属性值查询
* @param property 属性名称
* @param value 对应的属性值
* @return 返回数据表的映射对象列表
* @throws SQLException 抛出数据库异常
*/
List query(String property,Object value) throws SQLException;
/**
* 根据条件分页查询
* @param property 属性名称
* @param value 对应的属性值
* @param pageable 分页对象
* @return 分页后的数据对象
* @throws SQLException 抛出数据库异常
*/
Page query(String property,Object value,Pageable pageable) throws SQLException;
/**
* 查询所有
* @return 返回数据表的映射对象列表
* @throws SQLException 抛出数据库异常
*/
List query() throws SQLException;
/**
* 查询所有分页
* @param pageable 分页对象
* @return 分页后的数据对象
* @throws SQLException 抛出数据库异常
*/
Page query(Pageable pageable) throws SQLException;
/**
* 根据映射的对象设置的属性和值分页查询
* @param m 映射的对象
* @param pageable 分页对象
* @return 分页后的数据对象
* @throws SQLException 抛出数据库异常
*/
Page query(M m,Pageable pageable) throws SQLException;
/**
* 根据对象设置的属性查询
* @param m 映射的对象
* @return 返回数据表的映射对象列表
* @throws SQLException 抛出数据库异常
*/
List query(M m) throws SQLException;
/**
* 模拟持久化,有主键值调用更新操作,没有主键值 调用添加操作
* @param m 映射的对象
* @return 返回数据表的映射对象
* @throws SQLException 抛出数据库异常
*/
M save(M m) throws SQLException;
/**
* 根据映射对象设置的属性条件统计数量
* @param m 映射的对象
* @return 返回数字
* @throws SQLException 抛出数据库异常
*/
Integer count(M m) throws SQLException;
/**
* 根据属性名称和值统计数量
* @param property 属性名称
* @param value 属性值
* @return 返回数字
* @throws SQLException 抛出数据库异常
*/
Integer count(String property,Object value) throws SQLException;
/**
* 统计表中的数量
* @return 返回数字
* @throws SQLException 抛出数据库异常
*/
Integer count() throws SQLException;
/**
* 根据M主键值更新其他属性
* @param m 映射的对象
* @return 返回当前的映射对象
* @throws SQLException 抛出数据库异常
*/
M update(M m) throws SQLException;
/**
* 更新操作
* @param setValues 更新的属性与对应的值
* @param condValues 更新的条件属性和对应的值
* @return 返回执行的行数
* @throws SQLException 抛出数据库异常
*/
Integer update(Map setValues,Map condValues) throws SQLException;
/**
* 更新
* @param setProperty 更新的属性
* @param setValue 更新的值
* @param condProperty 条件属性
* @param condValue 条件值
* @return 返回执行的行数
* @throws SQLException 抛出数据库异常
*/
Integer update(String setProperty,Object setValue,String condProperty,Object condValue) throws SQLException;
/**
* 更新
* @param setProperty 更新的属性
* @param setValue 更新的值
* @param condValues 更新的条件属性和对应的值
* @return 返回执行的行数
* @throws SQLException 抛出数据库异常
*/
Integer update(String setProperty,Object setValue,Map condValues) throws SQLException;
/**
* 更新
* @param setValues 更新的属性与对应的值
* @param condProperty 条件属性
* @param condValue 条件值
* @return 返回执行的行数
* @throws SQLException 抛出数据库异常
*/
Integer update(Map setValues,String condProperty,Object condValue) throws SQLException;
/**
* 根据主键Id删除
* @param id 主键的值
* @return 返回影响的行数
* @throws SQLException 抛出数据库异常
*/
Integer deleteById(Serializable id)throws SQLException;
/**
* 根据M实例的对象属性删除
* @param m 映射的对象
* @return 返回影响的行数
* @throws SQLException 抛出数据库异常
*/
Integer delete(M m) throws SQLException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy