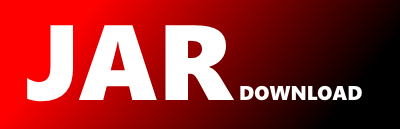
com.simple.orm.dao.impl.ConditionBuilderImpl Maven / Gradle / Ivy
package com.simple.orm.dao.impl;
import java.lang.reflect.Array;
import java.lang.reflect.Field;
import java.sql.SQLException;
import java.util.LinkedList;
import java.util.List;
import javax.persistence.Column;
import javax.persistence.EnumType;
import javax.persistence.Enumerated;
import org.springframework.util.StringUtils;
import com.simple.orm.dao.ConditionBuilder;
/**
* 条件构建器
*
* @author Administrator
*
* @param 实体M类
*/
public class ConditionBuilderImpl implements ConditionBuilder {
private OrmInfo ormInfo;
private Conditioner conditioner = new Conditioner();
public ConditionBuilderImpl(OrmInfo ormInfo) {
this.ormInfo = ormInfo;
}
public ConditionBuilder likeProperty(String property, Object value) throws SQLException {
String column = ormInfo.getColumnName(property);
return likeColumn(column, value);
}
public ConditionBuilder leftLikeProperty(String property, Object value) throws SQLException {
String column = ormInfo.getColumnName(property);
return leftLikeColumn(column, value);
}
public ConditionBuilder rightLikeProperty(String property, Object value) throws SQLException {
String column = ormInfo.getColumnName(property);
return rightLikeColumn(column, value);
}
public ConditionBuilder equalEntity(M m) throws SQLException {
try {
Field[] fields = ormInfo.getFields();
for (Field field : fields) {
field.setAccessible(true);
Object v = field.get(m);
if (v != null) {
Column column = field.getAnnotation(Column.class);
String columnLabel;
if (column == null || (columnLabel = column.name()).equals("")) {
continue;
}
if (field.getType().isEnum()) { // 如果是枚举类型
Enum em = (Enum) v;
Enumerated enumerated = field.getAnnotation(Enumerated.class);
EnumType enumType;
if (enumerated != null && (enumType = enumerated.value()) != null
&& enumType.equals(EnumType.ORDINAL)) {
v = em.ordinal();
} else {
v = em.name();
}
}
String condition = " m." + columnLabel + "= ";
conditioner.addCondition(condition, v);
}
}
} catch (Exception e) {
throw new SQLException(e);
}
return this;
}
public ConditionBuilder equalProperty(String property, Object value) throws SQLException {
String column = ormInfo.getColumnName(property);
return equalColumn(column, value);
}
public ConditionBuilder gtProperty(String property, Object value) throws SQLException {
String column = ormInfo.getColumnName(property);
return gtColumn(column, value);
}
public ConditionBuilder ltProperty(String property, Object value) throws SQLException {
String column = ormInfo.getColumnName(property);
return ltColumn(column, value);
}
public ConditionBuilder inProperty(String property, Object... values) throws SQLException {
String column = ormInfo.getColumnName(property);
return inColumn(column, values);
}
public ConditionBuilder likeColumn(String column, Object value) throws SQLException {
if (StringUtils.isEmpty(column)) {
throw new SQLException("查询条件 like 列名为空");
}
if (value == null) {
throw new SQLException("查询条件 like 列名[ " + column + " ] 值为空");
}
String condition = " m." + column + " like ";
String start = "CONCAT('%',";
String end = "'%')";
conditioner.addCondition(condition, start, end, value);
return this;
}
public ConditionBuilder leftLikeColumn(String column, Object value) throws SQLException {
if (StringUtils.isEmpty(column)) {
throw new SQLException("查询条件 like 列名为空");
}
if (value == null) {
throw new SQLException("查询条件 like 列名[ " + column + " ] 值为空");
}
String condition = " m." + column + " like ";
String start = "CONCAT('%',";
String end = ")";
conditioner.addCondition(condition, start, end, value);
return this;
}
public ConditionBuilder rightLikeColumn(String column, Object value) throws SQLException {
if (StringUtils.isEmpty(column)) {
throw new SQLException("查询条件 like 列名为空");
}
if (value == null) {
throw new SQLException("查询条件 like 列名[ " + column + " ] 值为空");
}
String condition = " m." + column + " like ";
String start = "CONCAT(";
String end = "'%')";
conditioner.addCondition(condition, start, end, value);
return this;
}
public ConditionBuilder equalColumn(String column, Object value) throws SQLException {
if (StringUtils.isEmpty(column)) {
throw new SQLException("查询条件 = 列名为空");
}
if (value == null) {
throw new SQLException("查询条件 = 列名[ " + column + " ] 值为空");
}
String condition = " m." + column + " = ";
conditioner.addCondition(condition, value);
return this;
}
public ConditionBuilder gtColumn(String column, Object value) throws SQLException {
if (StringUtils.isEmpty(column)) {
throw new SQLException("查询条件 > 列名为空");
}
if (value == null) {
throw new SQLException("查询条件 > 列名[ " + column + " ] 值为空");
}
String condition = "m." + column + " > ";
conditioner.addCondition(condition, value);
return this;
}
public ConditionBuilder ltColumn(String column, Object value) throws SQLException {
if (StringUtils.isEmpty(column)) {
throw new SQLException("查询条件 < 列名为空");
}
if (value == null) {
throw new SQLException("查询条件 < 列名[ " + column + " ] 值为空");
}
String condition = " m." + column + " < ";
conditioner.addCondition(condition, value);
return this;
}
public ConditionBuilder inColumn(String column, Object... values) throws SQLException {
if (StringUtils.isEmpty(column)) {
throw new SQLException("查询条件 in 列名为空");
}
if (values == null || values.length == 0) {
throw new SQLException(column + " in " + values + " is empty");
}
String condition = " m." + column + " in ";
conditioner.addCondition(condition, "(", ")", values);
return this;
}
public String bulidConditionSql() {
if (conditioner.getConditionSql().equals("")) {
return "";
}
StringBuffer buffer = new StringBuffer();
// 条件
buffer.append(" where ");
buffer.append(conditioner.getConditionSql());
return buffer.toString();
}
public Object[] getConditionvValues() {
return conditioner.getConditionValues();
}
/**
* 条件内部类
*
* @author Administrator
*
*/
private class Conditioner {
private StringBuffer conditionSql = new StringBuffer();
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy