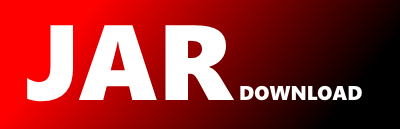
com.simple.orm.dao.impl.EntityRowMapper Maven / Gradle / Ivy
package com.simple.orm.dao.impl;
import java.lang.reflect.Field;
import java.sql.ResultSet;
import java.sql.ResultSetMetaData;
import java.sql.SQLException;
import java.util.HashMap;
import java.util.Map;
import javax.persistence.Column;
import org.springframework.jdbc.core.RowMapper;
public final class EntityRowMapper implements RowMapper {
private Class entityClass=null;
private Field[] fields= {};
@SuppressWarnings("unchecked")
public EntityRowMapper(Class> entityClass, Field[] fields) {
this.entityClass = (Class) entityClass;
this.fields = fields;
}
public M mapRow(ResultSet rs, int rowNum) throws SQLException {
M m = null;
try {
ResultSetMetaData rsmd = rs.getMetaData();
int count = rsmd.getColumnCount();
Map columnMap=new HashMap();
for (int i = 1; i <=count; i++) {
String columnName=rsmd.getColumnLabel(i);
columnMap.put(columnName, i);
}
m = entityClass.newInstance();
for (Field field : fields) {
Column column = field.getAnnotation(Column.class);
int columnIndex=columnMap.get(column.name());
if(columnIndex<=0) {
continue;
}
Object value = rs.getObject(columnIndex);
field.setAccessible(true);
field.set(m, value);
}
} catch (InstantiationException e) {
e.printStackTrace();
} catch (IllegalAccessException e) {
e.printStackTrace();
} catch (SecurityException e) {
e.printStackTrace();
} catch (IllegalArgumentException e) {
e.printStackTrace();
}
return m;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy