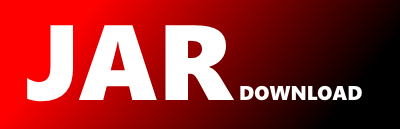
com.simple.orm.dao.impl.OrmInfo Maven / Gradle / Ivy
package com.simple.orm.dao.impl;
import java.lang.reflect.Field;
import java.sql.SQLException;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import java.util.StringJoiner;
import javax.persistence.Column;
import javax.persistence.Id;
import javax.persistence.OrderBy;
import javax.persistence.Table;
import org.springframework.jdbc.core.RowMapper;
import org.springframework.util.StringUtils;
import com.simple.orm.annotation.TargetDataSource;
/**
* 实体与表的信息类
* @author Administrator
* @param
*
*/
public class OrmInfo {
private Class entityClass;
/**
* 表面
*/
private String tableName;
private String targetDataSourceName;
/**
* 属性名称
*/
private String pkPropertyName;
/**
* 属性与列名 k:属性名,v:列名
*/
private Map ormMap=new HashMap();
/**
* 排序
*/
private String orderBy="";
//默认的查询列名
private String selectColumnNames;
/**
* 加载类中所有的属相和方法
* @param entityClass 映射对象的CLASS
*/
public OrmInfo(Class entityClass) {
this.entityClass=entityClass;
init();
}
/**
* 加载类中所有的属相和方法
*/
private void init() {
//获取表的名称
Table table = this.entityClass.getAnnotation(Table.class);
tableName = table.name();
//获取目标数据源
TargetDataSource targetDataSource = entityClass.getAnnotation(TargetDataSource.class);
if(targetDataSource!=null && !StringUtils.isEmpty(targetDataSource.name())) {
targetDataSourceName=targetDataSource.name();
}
//加载属性
Class> searchType = entityClass;
StringJoiner stringJoiner=new StringJoiner(",");
while (!Object.class.equals(searchType) && searchType != null) {
Field[] temp = searchType.getDeclaredFields();
for(Field field:temp){
//获取主键位置
if(StringUtils.isEmpty(pkPropertyName)) {
Id pk = field.getAnnotation(Id.class);
if (pk != null) {
pkPropertyName=field.getName();
}
}
//获取列信息
Column column = field.getAnnotation(Column.class);
String columnName;
if (column == null || (columnName = column.name()).equals("")) {
continue;
}
String propertyName=field.getName();
ormMap.put(propertyName, field);
stringJoiner.add("m."+columnName);
//加载默认排序字段和方式
OrderBy ob=field.getAnnotation(OrderBy.class);
if(ob!=null){
orderBy+=columnName+" "+(StringUtils.isEmpty(ob.value())?"ASC":ob.value());
}
}
searchType = searchType.getSuperclass();
}
selectColumnNames=stringJoiner.toString();
}
/**
* 获取主键列名
* @return 主键列名
* @throws SQLException 抛出数据库异常
*/
public String getPkColumnName() throws SQLException {
Field field=ormMap.get(pkPropertyName);
if(field!=null) {
return field.getAnnotation(Column.class).name();
}
throw new SQLException("在" + getEntityClass() + "中没有发现 @Id 主键标识");
}
/**
* 获取主键属性名
* @return 主键属性名
* @throws SQLException 抛出数据库异常
*/
public String getPkPropertyName() throws SQLException {
if(StringUtils.isEmpty(pkPropertyName)) {
throw new SQLException("在" + getEntityClass() + "中没有发现 @Id 主键标识");
};
return pkPropertyName;
}
/**
* 根据属性名称获取属性对象
* @param propertyName 属性名称
* @return 属性对象
*/
public Field getField(String propertyName) throws SQLException {
Field field=ormMap.get(propertyName);
if(field==null) {
throw new SQLException("在" + getEntityClass() + "中没有发现 属性为" + propertyName + " @column 标识");
}
return field;
}
/**
* 获取列名
* @param propertyName 属性名称
* @return 列名
* @throws SQLException 抛出数据库异常
*/
public String getColumnName(String propertyName) throws SQLException {
Field field=ormMap.get(propertyName);
if(field!=null) {
return field.getAnnotation(Column.class).name();
}
throw new SQLException("在" + getEntityClass() + "中没有发现 属性为" + propertyName + " @column 标识");
}
/**
* 获取fields
* @return 属性集合
*/
public Field[] getFields() {
Collection fields=ormMap.values();
return ormMap.values().toArray(new Field[fields.size()]);
}
public Class> getEntityClass() {
return entityClass;
}
public String getTableName() throws SQLException {
if (StringUtils.isEmpty(tableName)) {
throw new SQLException("在" + getEntityClass() + "上没有发现 @Table 标识");
}
return tableName;
}
public String getTargetDataSourceName() throws SQLException {
if (StringUtils.isEmpty(tableName)) {
throw new SQLException("在" + getEntityClass() + "上没有发现 @targetDataSourceName 标识");
}
return targetDataSourceName;
}
public String getOrderBy() {
return orderBy;
}
public String getSelectColumnNames() {
if (StringUtils.isEmpty(selectColumnNames)) {
return " * ";
}
return selectColumnNames;
}
public final RowMapper getRowMapper(){
Field[] fields=this.getFields();
return new EntityRowMapper(this.getEntityClass(), fields);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy