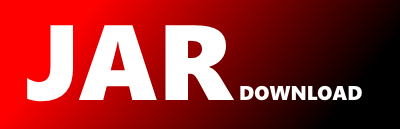
com.simple.orm.dao.impl.QueryerImpl Maven / Gradle / Ivy
package com.simple.orm.dao.impl;
import java.sql.SQLException;
import java.util.LinkedList;
import java.util.List;
import java.util.StringJoiner;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.util.StringUtils;
import com.simple.orm.dao.ConditionBuilder;
import com.simple.orm.dao.Queryer;
public class QueryerImpl implements Queryer {
private JdbcTemplate jdbcTemplate;
private OrmInfo ormInfo;
private ConditionBuilder conditionBuilder;
private List orderBys = new LinkedList();
private String defaultOrderBy,selectColumnNames,limit="";
public QueryerImpl(OrmInfo ormInfo,JdbcTemplate jdbcTemplate) {
this.ormInfo = ormInfo;
this.defaultOrderBy=ormInfo.getOrderBy();
this.selectColumnNames=ormInfo.getSelectColumnNames();
conditionBuilder=new ConditionBuilderImpl(ormInfo);
this.jdbcTemplate=jdbcTemplate;
}
public Queryer selectProperty(String... properties) throws SQLException {
if (properties == null || properties.length == 0) {
throw new SQLException("指定查询的属性不可以为空!");
}
StringJoiner sj = new StringJoiner(",");
for (String property : properties) {
String columnLabel = ormInfo.getColumnName(property);
sj.add("m."+columnLabel);
}
selectColumnNames = sj.toString();
return this;
}
public Queryer orderByProperty(String property,String by) throws SQLException{
String column=ormInfo.getColumnName(property);
return orderByColumn(column, by);
}
public Queryer orderByColumn(String column,String by) throws SQLException{
if(StringUtils.isEmpty(column)) {
throw new SQLException("order by 列名为空");
}
String order=" m."+column+" "+by;
orderBys.add(order);
return this;
}
public Queryer limit(int start,int size){
limit=" limit "+start+","+size;
return this;
}
public Queryer likeProperty(String property,Object value) throws SQLException{
conditionBuilder.likeProperty(property, value);
return this;
}
public Queryer leftLikeProperty(String property,Object value) throws SQLException{
conditionBuilder.leftLikeProperty(property, value);
return this;
}
public Queryer rightLikeProperty(String property,Object value) throws SQLException{
conditionBuilder.rightLikeProperty(property, value);
return this;
}
public Queryer equalEntity(M m) throws SQLException{
conditionBuilder.equalEntity(m);
return this;
}
public Queryer equalProperty(String property,Object value) throws SQLException{
conditionBuilder.equalProperty(property, value);
return this;
}
public Queryer gtProperty(String property,Object value) throws SQLException{
conditionBuilder.gtProperty(property, value);
return this;
}
public Queryer ltProperty(String property,Object value) throws SQLException{
conditionBuilder.ltProperty(property, value);
return this;
}
public Queryer inProperty(String property,Object... values) throws SQLException{
conditionBuilder.inProperty(property, values);
return this;
}
public Queryer likeColumn(String column,Object value) throws SQLException{
conditionBuilder.likeColumn(column, value);
return this;
}
public Queryer leftLikeColumn(String column,Object value) throws SQLException{
conditionBuilder.leftLikeColumn(column, value);
return this;
}
public Queryer rightLikeColumn(String column,Object value) throws SQLException{
conditionBuilder.rightLikeColumn(column, value);
return this;
}
public Queryer equalColumn(String column,Object value) throws SQLException{
conditionBuilder.equalColumn(column, value);
return this;
}
public Queryer gtColumn(String column,Object value) throws SQLException{
conditionBuilder.gtColumn(column, value);
return this;
}
public Queryer ltColumn(String column,Object value) throws SQLException{
conditionBuilder.ltColumn(column, value);
return this;
}
public Queryer inColumn(String column,Object... values) throws SQLException{
conditionBuilder.inColumn(column, values);
return this;
}
public M get() throws SQLException {
this.limit=" limit 0,1";
String sql = buildSelectSql();
List list=jdbcTemplate.query(sql, ormInfo.getRowMapper(),getConditionvValues());
if(list==null || list.size()==0){
return null;
}
return list.get(0);
}
public List list() throws SQLException {
String sql = buildSelectSql();
return jdbcTemplate.query(sql, ormInfo.getRowMapper(), getConditionvValues());
}
public Long count() throws SQLException {
String sql = buildCountSql();
return jdbcTemplate.queryForObject(sql, Long.class,getConditionvValues());
}
private String buildSelectSql() throws SQLException {
StringBuffer buffer=new StringBuffer();
buffer.append("select ");
buffer.append(selectColumnNames);
buffer.append(" from ");
buffer.append(ormInfo.getTableName());
buffer.append(" m ");
//条件
buffer.append(conditionBuilder.bulidConditionSql());
//order by
if(orderBys.size()>0) {
buffer.append(" order by ");
for(String orderBy:orderBys) {
buffer.append(orderBy);
buffer.append(" ");
}
}else if(defaultOrderBy!=null && !"".equals(defaultOrderBy.trim())){
buffer.append(" order by ");
buffer.append(defaultOrderBy);
buffer.append(" ");
}
//limt
buffer.append(limit);
return buffer.toString();
}
private String buildCountSql() throws SQLException {
StringBuffer buffer=new StringBuffer();
buffer.append("select count(*)");
buffer.append(" from ");
buffer.append(ormInfo.getTableName());
buffer.append(" m ");
//条件
buffer.append(conditionBuilder.bulidConditionSql());
return buffer.toString();
}
private Object[] getConditionvValues() {
return conditionBuilder.getConditionvValues();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy