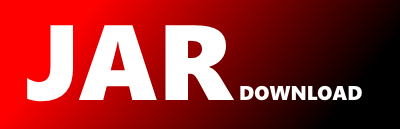
com.simple.orm.domain.PageImpl Maven / Gradle / Ivy
package com.simple.orm.domain;
import java.util.ArrayList;
import java.util.List;
/**
* 分页内容实现类
* @param M 数据的实体对象
*/
public class PageImpl implements Page {
private final Pageable pageable;
private final long total;
private List contents = new ArrayList();
private static final int MAX_DISPLAY_PAGE_NUM = 5; //一页最大显示页码数
//总页数
private int pages=0;
/**
* 分页构造
* @param contents 页面中的内容
* @param pageable 页码分页构造器
* @param total 总条数
*/
public PageImpl(List contents, Pageable pageable, long total) {
this.pageable = pageable;
this.total = total;
this.contents = contents;
this.pages=(int) Math.ceil((double) total / (double) pageable.getPageSize());
}
/**
* 获取下一页页码
* @return 返回下一页页码
*/
public Integer getNextPageNum() {
return getCurrentPageNum() < getPages() ? getCurrentPageNum() + 1 : -1;
}
/**
* 上一页页码
* @return 上一页页码
*/
public Integer getPrevPageNum() {
return getCurrentPageNum() > 1 ? getCurrentPageNum() - 1 : -1;
}
/**
* 获取当前的页码
* @return 返回当前的页码数
*/
public Integer getCurrentPageNum() {
return pageable.getCurrentPageNum();
}
/**
* 获取页码列表
* @return 返回页码列表
*/
public int[] getPageNums() {
int start;
int end;
int mid = (int) Math.ceil((double) MAX_DISPLAY_PAGE_NUM / 2);
if (getCurrentPageNum() <= mid) {
start = 1;
end = getPages() > MAX_DISPLAY_PAGE_NUM ? MAX_DISPLAY_PAGE_NUM : getPages();
} else if (getCurrentPageNum() >= getPages() - mid) {
start = getPages() - MAX_DISPLAY_PAGE_NUM + 1;
end = getPages();
} else {
start = getCurrentPageNum() - 2;
int temp = getCurrentPageNum() + 2;
end = temp > getPages() ? getPages() : temp;
}
int[] pageNums = new int[end - start + 1];
for (int i = 0; start <= end; start++, i++) {
pageNums[i] = start;
}
return pageNums;
}
/**
* 获取总条数
* @return 返回总条数
*/
public long getTotal() {
return this.total;
}
/**
* 获取页码总数
* @return 返回总页数
*/
public int getPages() {
return this.pages;
}
/**
* 返回页码里面的元素
*
* @return 返回页码里面的元素
*/
public List getContents() {
return this.contents;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy