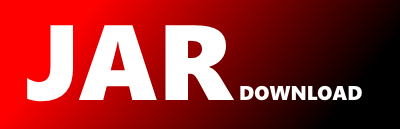
com.ld.zxw.service.LuceneServiceImpl Maven / Gradle / Ivy
The newest version!
package com.ld.zxw.service;
import java.io.IOException;
import java.util.List;
import org.apache.lucene.document.Document;
import org.apache.lucene.index.Term;
import org.apache.lucene.search.Query;
import org.apache.lucene.search.Sort;
import org.apache.lucene.search.highlight.InvalidTokenOffsetsException;
import com.ld.zxw.config.LuceneDataSource;
import com.ld.zxw.config.LucenePlusConfig;
import com.ld.zxw.index.IndexDao;
import com.ld.zxw.page.Page;
import com.ld.zxw.util.CommonUtil;
public class LuceneServiceImpl implements LuceneService {
private LucenePlusConfig lucenePlusConfig = null;
private IndexDao indexDao;
public LuceneServiceImpl(String sourceKey){
lucenePlusConfig = LuceneDataSource.build().dataSource.get(sourceKey);
this.indexDao = IndexDao.build(this.lucenePlusConfig);
}
@Override
public void saveObj(List objs) throws IOException {
indexDao.saveIndex(CommonUtil.turnDoc(objs, this.lucenePlusConfig));
}
@Override
public void saveObj(T objs) throws IOException {
indexDao.saveIndex(CommonUtil.turnDoc(objs, this.lucenePlusConfig));
}
@Override
public void saveDocument(List documents) throws IOException {
indexDao.saveIndex(documents);
}
@Override
public void saveDocument(Document document) throws IOException {
indexDao.saveIndex(document);
}
@Override
public void delAll() throws IOException {
indexDao.delAll();
}
@Override
public void delKey(Term term) throws IOException {
indexDao.deletekey(term);
}
@Override
public void delKey(Query query) throws IOException {
indexDao.deletekey(query);
}
@Override
public void updateObj(List objs, Term term) throws IOException {
indexDao.updateIndex(CommonUtil.turnDoc(objs, this.lucenePlusConfig), term);
}
@Override
public void updateObj(T obj, Term term) throws IOException {
indexDao.updateIndex(CommonUtil.turnDoc(obj, this.lucenePlusConfig), term);
}
@Override
public void updateDocument(List docs, Term term) throws IOException {
indexDao.updateIndex(docs, term);
}
@Override
public void updateDocument(Document doc, Term term) throws IOException {
indexDao.updateIndex(doc, term);
}
@Override
public List findList(Query query, Class obj, int num, Sort sort) throws IOException, InvalidTokenOffsetsException {
return indexDao.findList(query, obj, num, sort);
}
@Override
public Page findList(Query query, int pageNumber, int pageSize, Class obj, Sort sort) throws IOException, InvalidTokenOffsetsException {
return indexDao.findList(query, pageNumber, pageSize, obj, sort);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy