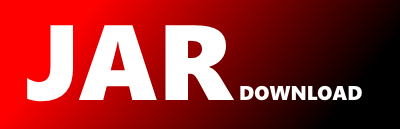
cicada.core.FileUtil Maven / Gradle / Ivy
package cicada.core;
import java.io.BufferedOutputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
public class FileUtil
{
/**
* 获取项目根目录 默认返回根目录 例如"/tmp/123/ 但有可能存在空格 " 如果运行在jar包里面 返回""
*
* @return
*/
public static String rootPath()
{
try
{
String path = FileUtil.class.getClassLoader().getResource("").getPath();
return path;
}
catch (Exception e)
{
return "";
}
}
/**
* 文件转成bytes[]
*
* @param filePath
* @return
* @throws FileNotFoundException
* @throws IOException
*/
public static byte[] file2bytes(final String filePath) throws FileNotFoundException, IOException
{
File file = new File(filePath);
if (file != null && file.isFile())
{
byte[] bytes = file2bytes(file);
return bytes;
}
return null;
}
/**
* File 读取byte[]
*
* @param file
* @return
* @throws FileNotFoundException
* @throws IOException
*/
public static byte[] file2bytes(final File file) throws FileNotFoundException, IOException
{
try (FileInputStream fileInputStream = new FileInputStream(file))
{
try (ByteArrayOutputStream byteArrayOutputStream = new ByteArrayOutputStream(1000))
{
byte[] bytes = new byte[1000];
int n = -1;
while ((n = fileInputStream.read(bytes)) != -1)
{
byteArrayOutputStream.write(bytes, 0, n);
}
byte[] buffer = byteArrayOutputStream.toByteArray();
return buffer;
}
}
}
/**
* 读取文件内容
*
* @param filePath
* @return
* @throws FileNotFoundException
* @throws IOException
*/
public static String file2string(final String filePath) throws FileNotFoundException, IOException
{
File file = new File(filePath);
if (file != null && file.isFile())
{
byte[] bytes = file2bytes(file);
return new String(bytes);
}
return null;
}
/**
* @param file
* @return
* @throws FileNotFoundException
* @throws IOException
*/
public static String file2String(final File file) throws FileNotFoundException, IOException
{
if (file != null && file.isFile())
{
byte[] bytes = file2bytes(file);
String result = new String(bytes);
return result;
}
return null;
}
/**
* byte[] 写入文件
*
* @param bytes
* @param filePath
* @return
* @throws FileNotFoundException
* @throws IOException
*/
public static boolean byte2file(byte[] bytes, final String filePath) throws FileNotFoundException, IOException
{
File file = new File(filePath);
boolean result = byte2file(bytes, file);
return result;
}
/**
* 字符串写入文件
*
* @param content
* @param file
* @return
* @throws FileNotFoundException
* @throws IOException
*/
public static boolean string2file(String content, File file) throws FileNotFoundException, IOException
{
byte[] bytes = content.getBytes();
boolean result = byte2file(bytes, file);
return result;
}
private static boolean byte2file(byte[] bytes, File file) throws FileNotFoundException, IOException
{
try (OutputStream outputStream = new FileOutputStream(file))
{
try (BufferedOutputStream bufferedOutputStream = new BufferedOutputStream(outputStream))
{
bufferedOutputStream.write(bytes);
return true;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy