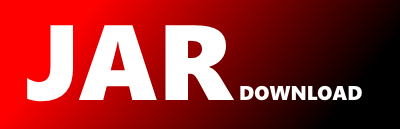
cicada.filesystem.FileSystemImpl Maven / Gradle / Ivy
package cicada.filesystem;
import java.io.IOException;
import java.util.Map;
import org.csource.common.MyException;
import org.springframework.stereotype.Component;
import cicada.core.PropertyResolverCustom;
import cicada.core.config.ConfigManager;
import cicada.filesystem.distributed.FileSystemImplDistributed;
import cicada.filesystem.local.FileSystemImplLocal;
@Component
public class FileSystemImpl implements FileSystem
{
private final String typeConfigName = "Cicada.FileSystem.Type";
private FileSystem _fileSystem = null;
public FileSystemImpl() throws Exception
{
String cicadaPath=ConfigManager.getCicadaConfigPath();
Map configurationDataRespository = PropertyResolverCustom.getConfigProperties(cicadaPath);
String text = configurationDataRespository.get(typeConfigName);
if (text == null || text.isEmpty())
{
text = "local";
}
text = text.toLowerCase();
if (text.equals("local"))
{
_fileSystem = new FileSystemImplLocal(configurationDataRespository);
return;
}
else if (text.equals("distributed"))
{
_fileSystem = new FileSystemImplDistributed(configurationDataRespository);
return;
}
throw new Exception(String.format("您配置的文件系统类型无效,请配置%s节点", typeConfigName));
}
@Override
public String upload(byte[] data, String fileExt) throws Exception
{
return this._fileSystem.upload(data, fileExt, 0);
}
@Override
public String upload1(byte[] data, String fileName) throws IOException, MyException, Exception
{
return this._fileSystem.upload1(data, fileName, 0);
}
@Override
public String upload(byte[] data, String fileExt, int resultPathType) throws Exception
{
return this._fileSystem.upload(data, fileExt, resultPathType);
}
@Override
public String upload1(byte[] data, String fileName, int resultPathType) throws IOException, MyException, Exception
{
return this._fileSystem.upload1(data, fileName, resultPathType);
}
@Override
public byte[] download(String fileName) throws IOException, MyException, Exception
{
return this._fileSystem.download(fileName);
}
@Override
public boolean remove(String fileName) throws Exception
{
return this._fileSystem.remove(fileName);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy