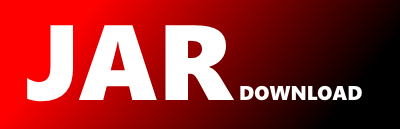
cicada.filesystem.distributed.FileSystemImplDistributed Maven / Gradle / Ivy
package cicada.filesystem.distributed;
import java.io.IOException;
import java.util.Map;
import org.csource.common.MyException;
import org.csource.fastdfs.ClientGlobal;
import org.csource.fastdfs.StorageClient;
import org.csource.fastdfs.StorageServer;
import org.csource.fastdfs.TrackerClient;
import org.csource.fastdfs.TrackerServer;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import cicada.core.Guard;
import cicada.filesystem.FileSystem;
public class FileSystemImplDistributed implements FileSystem
{
private static final Logger log = LoggerFactory.getLogger(FileSystemImplDistributed.class);
private final String serverConfigName = "Cicada.FileSystem.Distributed.Server";
private final String groupNameConfigName = "Cicada.FileSystem.Distributed.GroupName";
private final String pathUrlPrefix = "Cicada.FileSystem.UrlPrefix";
private Map _configurationDataRespository;
private String _groupName;
public FileSystemImplDistributed(Map configurationDataRespository) throws Exception
{
_configurationDataRespository = configurationDataRespository;
initFastDfs();
_groupName = configurationDataRespository.get(groupNameConfigName);
if (_groupName == null || _groupName.isEmpty())
{
throw new Exception(String.format("请为分布式文件系统设置组名称,请配置%s节点", groupNameConfigName));
}
}
@Override
public String upload(byte[] data, String fileExt) throws Exception
{
String result = this.upload(data, fileExt, 0);
return result;
}
@Override
public String upload(byte[] data, String fileExt, int resultPathType) throws Exception
{
Guard.ThrowIfArgumentIsNull(data, "data");
Guard.ThrowIfArgumentIsNull(fileExt, "fileExt");
TrackerClient tracker = new TrackerClient();
TrackerServer trackerServer = tracker.getConnection();
StorageServer storageServer = null;
StorageClient client = new StorageClient(trackerServer, storageServer);
String[] fileInfo = client.upload_file(this._groupName, data, fileExt, null);
if (fileInfo == null) return null;
String result = String.format("%s/%s", fileInfo[0], fileInfo[1]);
if (resultPathType != 0)// 相对路径
{
String temp = _configurationDataRespository.get(pathUrlPrefix);
if (temp == null || temp.isEmpty())
{
log.error(String.format("将文件路径转换为绝对路径时出现错误,错误原因是没有配置Url前缀,请通过配置文件配置%s节点", pathUrlPrefix));
}
else
{
result = String.format("%s/%s", temp, result);
}
}
return result;
}
@Override
public byte[] download(String fileName) throws Exception
{
Guard.ThrowIfArgumentIsNullOrEmpty(fileName, "fileName");
FilePath filePath = new FilePath(fileName);
TrackerClient tracker = new TrackerClient();
TrackerServer trackerServer = tracker.getConnection();
StorageServer storageServer = null;
StorageClient storageClient = new StorageClient(trackerServer, storageServer);
String groupName = filePath.getGroupName();
String storageFileName = filePath.getStorageFileName();
byte[] result = storageClient.download_file(groupName, storageFileName);
return result;
}
@Override
public boolean remove(String fileName) throws Exception
{
Guard.ThrowIfArgumentIsNullOrEmpty(fileName, "fileName");
FilePath filePath = new FilePath(fileName);
TrackerClient tracker = new TrackerClient();
TrackerServer trackerServer = tracker.getConnection();
StorageServer storageServer = null;
String groupName = filePath.getGroupName();
String storageFileName = filePath.getStorageFileName();
StorageClient storageClient = new StorageClient(trackerServer, storageServer);
int result = storageClient.delete_file(groupName, storageFileName);
if (result == 0) return true;
else
{
log.info(String.format("删除失败:状态码为%s", result));
return false;
}
}
private void initFastDfs() throws Exception
{
String text = _configurationDataRespository.get(serverConfigName);
if (text == null || text.isEmpty())
{
throw new Exception(String.format("请为分布式文件系统设置服务器路径,请配置%s节点", serverConfigName));
}
try
{
ClientGlobal.initByTrackers(text);
}
catch (Exception e)
{
throw new Exception(String.format("您配置的分布式文件系统服务器路径无效,请配置%s节点", serverConfigName));
}
}
private class FilePath
{
private String groupName;
private String storageFileName;
public String getGroupName()
{
return groupName;
}
public void setGroupName(String groupName)
{
this.groupName = groupName;
}
public String getStorageFileName()
{
return storageFileName;
}
public void setStorageFileName(String storageFileName)
{
this.storageFileName = storageFileName;
}
public FilePath(String fileName)
{
int num = fileName.indexOf('/');
groupName = fileName.substring(0, num);
storageFileName = fileName.substring(num + 1);
}
@Override
public String toString()
{
return this.groupName + '/' + this.storageFileName;
}
}
@Override
public String upload1(byte[] data, String fileName) throws IOException, MyException, Exception
{
return null;
}
@Override
public String upload1(byte[] data, String fileName, int resultPathType) throws IOException, MyException, Exception
{
return null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy