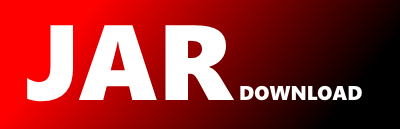
cicada.filesystem.local.FileSystemImplLocal Maven / Gradle / Ivy
package cicada.filesystem.local;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.Map;
import java.util.UUID;
import org.csource.common.MyException;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import cicada.core.Guard;
import cicada.filesystem.FileSystem;
public class FileSystemImplLocal implements FileSystem
{
private static final Logger log = LoggerFactory.getLogger(FileSystemImplLocal.class);
private final String rootConfigName = "Cicada.FileSystem.Local.Root";
private String _root;
public FileSystemImplLocal(Map configurationDataRespository) throws Exception
{
this._root = configurationDataRespository.get(rootConfigName);
if (_root == null || _root.isEmpty())
{
throw new Exception(String.format("请为本地文件系统设置根目录,请配置%s节点", rootConfigName));
}
String projectRoot = cicada.core.FileUtil.rootPath();
StringBuffer sb = new StringBuffer();
_root = sb.append(projectRoot).append(_root).toString();
}
@Override
public String upload(byte[] data, String fileExt) throws Exception
{
String result = this.upload(data, fileExt, 0);
return result;
}
@Override
public String upload1(byte[] data, String fileName) throws IOException, MyException, Exception
{
String result = this.upload(data, fileName, 0);
return result;
}
@Override
public String upload(byte[] data, String fileExt, int resultPathType) throws Exception
{
Guard.ThrowIfArgumentIsNull(data, "data");
Guard.ThrowIfArgumentIsNullOrEmpty(fileExt, "fileExt");
SimpleDateFormat sdf = new SimpleDateFormat(String.format("yyyy%sMM%sdd", File.separator, File.separator));
String path = sdf.format(new Date());
path = _root + File.separator + path;
File filePath = new File(path);
if (!filePath.exists())
{
if (!filePath.mkdirs()) return null;
}
String uuid = UUID.randomUUID().toString().replace("-", "");
String fileName = uuid + "." + fileExt;
fileName = path + File.separator + fileName;
File temp = new File(fileName);
if (temp.exists())
{
temp.delete();
}
boolean result = temp.createNewFile();
if (!result) return null;
BufferedOutputStream stream = null;
try
{
stream = new BufferedOutputStream(new FileOutputStream(temp));
stream.write(data);
}
catch (Exception e)
{
log.error("文件上传错误", e);
}
finally
{
stream.close();
}
if (resultPathType == 0) return fileName.replace(_root, "");
return fileName;
}
@Override
public String upload1(byte[] data, String fileName, int resultPathType) throws IOException, MyException, Exception
{
Guard.ThrowIfArgumentIsNull(data, "data");
Guard.ThrowIfArgumentIsNullOrEmpty(fileName, "fileName");
SimpleDateFormat sdf = new SimpleDateFormat(String.format("yyyy%sMM%sdd", File.separator, File.separator));
String path = sdf.format(new Date());
path = _root + File.separator + path;
File filePath = new File(path);
if (!filePath.exists())
{
if (!filePath.mkdirs()) return null;
}
fileName = path + File.separator + fileName;
File temp = new File(fileName);
if (temp.exists())
{
temp.delete();
}
boolean result = temp.createNewFile();
if (!result) return null;
BufferedOutputStream stream = null;
try
{
stream = new BufferedOutputStream(new FileOutputStream(temp));
stream.write(data);
}
catch (Exception e)
{
log.error("文件上传错误", e);
e.printStackTrace();
}
finally
{
stream.close();
}
if (resultPathType == 0) return fileName.replace(_root, "");
return fileName;
}
@Override
public byte[] download(String fileName) throws Exception
{
Guard.ThrowIfArgumentIsNull(fileName, "fileName");
String physicsPath = this._root + File.separator + fileName;
File file = new File(physicsPath);
if (file.exists())
{
ByteArrayOutputStream bos = new ByteArrayOutputStream((int) file.length());
BufferedInputStream in = null;
try
{
in = new BufferedInputStream(new FileInputStream(file));
int buf_size = 1024;
byte[] buffer = new byte[buf_size];
int len = 0;
while (-1 != (len = in.read(buffer, 0, buf_size)))
{
bos.write(buffer, 0, len);
}
return bos.toByteArray();
}
catch (IOException e)
{
e.printStackTrace();
}
finally
{
if (in != null) in.close();
if (bos != null) bos.close();
}
}
return null;
}
@Override
public boolean remove(String fileName) throws Exception
{
Guard.ThrowIfArgumentIsNull(fileName, "fileName");
String physicsPath = this._root + File.separator + fileName;
File file = new File(physicsPath);
if (!file.exists())
{
return true;
}
return file.delete();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy