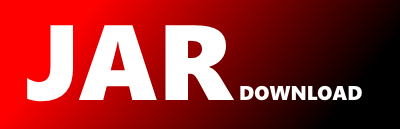
net.ossindex.common.VulnerabilityDescriptor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ossindex-api Show documentation
Show all versions of ossindex-api Show documentation
OSS Index REST API access library
/**
* Copyright (c) 2017 Vör Security Inc.
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
* * Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* * Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
* * Neither the name of the nor the
* names of its contributors may be used to endorse or promote products
* derived from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
* ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
* WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL BE LIABLE FOR ANY
* DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
* (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
* LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
* ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
* SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package net.ossindex.common;
import java.util.Date;
import java.util.Iterator;
import java.util.List;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlElementWrapper;
/** Represents a vulnerability from OSS Index
*
* @author Ken Duck
*
*/
public class VulnerabilityDescriptor {
@XmlElement(name = "id")
private Long id = 0L;
@XmlElement(name = "title")
private String title;
@XmlElement(name = "description")
private String description;
@XmlElementWrapper(name="versions")
@XmlElement(name = "version")
private List versions;
@XmlElementWrapper(name="references")
@XmlElement(name = "reference")
private List references;
@XmlElement(name = "published")
private long published;
@XmlElement(name = "updated")
private long updated;
@XmlElement(name = "cve")
private String cve;
/**
* Get the OSS Index ID
* @return the ID
*/
public long getId() {
return id;
}
/**
* Get the title
* @return the title
*/
public String getTitle() {
return title;
}
/**
* Get the description
* @return the description
*/
public String getDescription() {
return description;
}
/**
* Get the versions
* @return the versions
*/
public List getVersions() {
return versions;
}
/**
* Get the references
* @return The references
*/
public List getReferences() {
return references;
}
/**
* Get the published timestamp
* @return published timestamp
*/
public long getPublished() {
return published;
}
/**
* Get the published date
* @return published date
*/
public Date getPublishedDate() {
return new Date(published);
}
/**
* Get the updated timestamp
* @return updated timestamp
*/
public long getUpdated() {
return updated;
}
/**
* Get the updated date
* @return updated date
*/
public Date getUpdatedDate() {
return new Date(updated);
}
/**
* Get the OSS Index URL through which to find more information
* @return URL for finding more information
*/
public String getUriString() {
if (isCve()) {
return "https://ossindex.sonatype.org/resource/cve/" + id;
}
return "https://ossindex.sonatype.org/resource/vulnerability/" + id;
}
/** Is this vulnerability a CVE?
*
* @return The CVE
*/
public boolean isCve() {
if (cve != null) {
return true;
}
return false;
}
@Override
public int hashCode() {
return id.hashCode();
}
@Override
public boolean equals(Object o) {
if (o instanceof VulnerabilityDescriptor) {
return id.equals(((VulnerabilityDescriptor)o).id);
}
return false;
}
/*
* (non-Javadoc)
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("[").append(id).append("] ").append(title).append(" (");
if (versions != null) {
for (Iterator it = versions.iterator(); it.hasNext();) {
String version = it.next();
sb.append(version);
if (it.hasNext()) {
sb.append(" || ");
}
}
}
sb.append(")");
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy