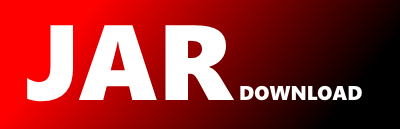
net.ossindex.common.request.FromTillRequest Maven / Gradle / Ivy
package net.ossindex.common.request;
import java.io.IOException;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
import java.util.Spliterator;
import java.util.function.Consumer;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import net.ossindex.common.IFromTillRequest;
import net.ossindex.common.PackageDescriptor;
/** Implements vulnerability queries
*
* @author Ken Duck
*
*/
public class FromTillRequest implements Iterator>, Iterable>, IFromTillRequest {
private Gson gson = new GsonBuilder().disableHtmlEscaping().create();
private String pm;
private long from;
private long till;
private final OssIndexHttpClient client;
/**
* From beginning Till end
* @param pm
*/
public FromTillRequest(OssIndexHttpClient client, String pm) {
this.client = client;
this.pm = pm;
this.from = 0;
this.till = Long.MAX_VALUE;
}
public FromTillRequest(OssIndexHttpClient client, String pm, long from, long till) {
this.client = client;
this.pm = pm;
this.from = from;
if (till < 0) {
this.till = Long.MAX_VALUE;
} else {
this.till = till;
}
}
/*
* (non-Javadoc)
* @see java.lang.Iterable#iterator()
*/
@Override
public Iterator> iterator() {
return this;
}
@Override
public boolean hasNext() {
return from < till;
}
@Override
public Collection next() {
// Perform the OSS Index query
String query = "vulnerability/pm/" + pm + "/fromtill/" + from + "/" + till;
try {
String json = client.performGetRequest(query);
// System.err.println(json);
FromTillResponse response = gson.fromJson(json, FromTillResponse.class);
System.err.println("FROMTILL:");
System.err.println(" REQUESTED FROM: " + response.getRequestedFrom());
System.err.println(" REQUESTED TILL: " + response.getRequestedTill());
System.err.println(" ACTUAL FROM: " + response.getActualFrom());
System.err.println(" NEXT: " + response.getNext());
till = response.getActualFrom() - 1;
return response.getPackages();
} catch (IOException e) {
throw new AssertionError(e);
}
}
@Override
public void remove() {
throw new UnsupportedOperationException();
}
}
class FromTillResponse {
private List packages;
private Long requestedFrom;
private Long requestedTill;
private Long actualFrom;
private String next;
public List getPackages() {
return packages;
}
/**
* @return the requestedFrom
*/
public Long getRequestedFrom() {
return requestedFrom;
}
/**
* @return the requestedTill
*/
public Long getRequestedTill() {
return requestedTill;
}
/**
* @return the actualFrom
*/
public Long getActualFrom() {
return actualFrom;
}
/**
* @return the next
*/
public String getNext() {
return next;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy