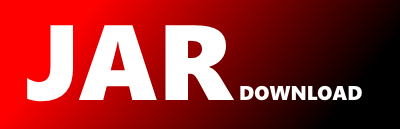
net.ossindex.common.request.OssIndexHttpClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ossindex-api Show documentation
Show all versions of ossindex-api Show documentation
OSS Index REST API access library
package net.ossindex.common.request;
import java.io.IOException;
import java.net.ConnectException;
import java.util.Arrays;
import java.util.LinkedList;
import java.util.List;
import org.apache.http.HttpHost;
import org.apache.http.ParseException;
import org.apache.http.client.config.AuthSchemes;
import org.apache.http.client.config.RequestConfig;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
public class OssIndexHttpClient
{
private List proxies = new LinkedList();
private static String SCHEME = "https";
private static String HOST = "ossindex.sonatype.org";
private static String VERSION = "v2.0";
private static String BASE_URL = SCHEME + "://" + HOST + "/" + VERSION + "/";
static {
String scheme = System.getProperty("OSSINDEX_SCHEME");
if (scheme != null && !scheme.trim().isEmpty()) {
SCHEME = scheme.trim();
}
String host = System.getProperty("OSSINDEX_HOST");
if (host != null && !host.trim().isEmpty()) {
HOST = host.trim();
}
String version = System.getProperty("OSSINDEX_VERSION");
if (version != null && !version.trim().isEmpty()) {
VERSION = version.trim();
}
BASE_URL = SCHEME + "://" + HOST + "/" + VERSION + "/";
}
private static int PROXY_SOCKET_TIMEOUT = 60000;
private static int PROXY_CONNECT_TIMEOUT = 10000;
private static int PROXY_CONNECTION_REQUEST_TIMEOUT = 10000;
static {
// Define the default proxy settings using environment variables
String tmp = System.getenv("OSSINDEX_PROXY_SOCKET_TIMEOUT");
if (tmp != null && !tmp.trim().isEmpty()) {
PROXY_SOCKET_TIMEOUT = Integer.parseInt(tmp.trim());
}
tmp = System.getenv("OSSINDEX_PROXY_CONNECT_TIMEOUT");
if (tmp != null && !tmp.trim().isEmpty()) {
PROXY_CONNECT_TIMEOUT = Integer.parseInt(tmp.trim());
}
tmp = System.getenv("OSSINDEX_PROXY_CONNECTION_REQUEST_TIMEOUT");
if (tmp != null && !tmp.trim().isEmpty()) {
PROXY_CONNECTION_REQUEST_TIMEOUT = Integer.parseInt(tmp.trim());
}
}
static {
// Define the default proxy settings using properties
String tmp = System.getProperty("OSSINDEX_PROXY_SOCKET_TIMEOUT");
if (tmp != null && !tmp.trim().isEmpty()) {
PROXY_SOCKET_TIMEOUT = Integer.parseInt(tmp.trim());
}
tmp = System.getProperty("OSSINDEX_PROXY_CONNECT_TIMEOUT");
if (tmp != null && !tmp.trim().isEmpty()) {
PROXY_CONNECT_TIMEOUT = Integer.parseInt(tmp.trim());
}
tmp = System.getProperty("OSSINDEX_PROXY_CONNECTION_REQUEST_TIMEOUT");
if (tmp != null && !tmp.trim().isEmpty()) {
PROXY_CONNECTION_REQUEST_TIMEOUT = Integer.parseInt(tmp.trim());
}
}
private static final String USER = System.getProperty("OSSINDEX_USER");
private static final String TOKEN = System.getProperty("OSSINDEX_TOKEN");
/**
* Override the server location.
*/
static void setHost(String scheme, String host, int port) {
BASE_URL = scheme + "://" + host + ":" + port + "/" + VERSION + "/";
}
/** Perform the request with the given URL and JSON data.
*
* @param requestString Server request relative URL
* @param data JSON data for the request
* @return JSON results of the request
* @throws IOException On query problems
*/
public String performPostRequest(String requestString, String data) throws IOException {
HttpPost request = new HttpPost(getBaseUrl() + requestString);
String json = null;
CloseableHttpClient httpClient = HttpClients.createDefault();
if (proxies.size() > 0) {
// We only check the first proxy for now
Proxy myProxy = proxies.get(0);
HttpHost proxy = myProxy.getHttpHost();
RequestConfig config = RequestConfig.custom()
.setProxy(proxy)
.setSocketTimeout(myProxy.getProxySocketTimeout())
.setConnectTimeout(myProxy.getProxyConnectTimeout())
.setConnectionRequestTimeout(myProxy.getProxyConnectionRequestTimeout())
.setProxyPreferredAuthSchemes(Arrays.asList(AuthSchemes.BASIC))
.build();
request.setConfig(config);
}
try {
request.setEntity(new StringEntity(data));
CloseableHttpResponse response = httpClient.execute(request);
int code = response.getStatusLine().getStatusCode();
if(code < 200 || code > 299) {
throw new ConnectException(response.getStatusLine().getReasonPhrase() + " (" + code + ")");
}
json = EntityUtils.toString(response.getEntity(), "UTF-8");
} catch(ParseException e) {
throw new IOException(e);
} finally {
httpClient.close();
}
return json;
}
/** Perform a get request
*
* @param requestString
* @return
* @throws IOException
*/
protected String performGetRequest(String requestString) throws IOException {
HttpGet request = new HttpGet(getBaseUrl() + requestString);
if (USER != null && !USER.isEmpty()) {
request.setHeader("Authorization", USER + ":" + TOKEN);
}
String json = null;
CloseableHttpClient httpClient = HttpClients.createDefault();
try {
CloseableHttpResponse response = httpClient.execute(request);
int code = response.getStatusLine().getStatusCode();
if(code < 200 || code > 299) {
throw new ConnectException(response.getStatusLine().getReasonPhrase() + " (" + code + ")");
}
json = EntityUtils.toString(response.getEntity(), "UTF-8");
} catch(ParseException e) {
throw new IOException(e);
} finally {
httpClient.close();
}
return json;
}
/** Get the base URL for requests
*
* @return The base URL
*/
private String getBaseUrl() {
return BASE_URL;
}
/** Add a proxy server through which to make requests
*/
public void addProxy(String protocol, String host, int port, String username, String password) {
Proxy proxy = new Proxy(protocol, host, port, username, password);
proxies.add(proxy);
}
/** Add a proxy server through which to make requests
*/
public void addProxy(String protocol, String host, int port, String username, String password,
int socketTimeout, int connectTimeout, int connectionRequestTimeout) {
Proxy proxy = new Proxy(protocol, host, port, username, password, socketTimeout, connectTimeout, connectionRequestTimeout);
proxies.add(proxy);
}
/**
* Simple POJO for proxy information.
*/
class Proxy {
private String protocol;
private String host;
private int port;
private String username;
private String password;
private int proxySocketTimeout = PROXY_SOCKET_TIMEOUT;
private int proxyConnectTimeout = PROXY_CONNECT_TIMEOUT;
private int proxyConnectionRequestTimeout = PROXY_CONNECTION_REQUEST_TIMEOUT;
public Proxy(String protocol, String host, int port, String username, String password) {
this.protocol = protocol;
this.host = host;
this.port = port;
this.username = username;
this.password = password;
}
public int getProxyConnectionRequestTimeout() {
return proxyConnectionRequestTimeout;
}
public int getProxyConnectTimeout() {
return proxyConnectTimeout;
}
public int getProxySocketTimeout() {
return proxySocketTimeout;
}
public Proxy(String protocol, String host, int port, String username, String password,
int socketTimeout, int connectTimeout, int connectionRequestTimeout) {
this.protocol = protocol;
this.host = host;
this.port = port;
this.username = username;
this.password = password;
proxySocketTimeout = socketTimeout;
proxyConnectTimeout = connectTimeout;
proxyConnectionRequestTimeout = connectionRequestTimeout;
}
public HttpHost getHttpHost() {
return new HttpHost(host, port, protocol);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy