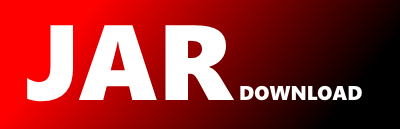
net.ossindex.common.request.PackageRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ossindex-api Show documentation
Show all versions of ossindex-api Show documentation
OSS Index REST API access library
/**
* Copyright (c) 2017 Vör Security Inc.
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
* * Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* * Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
* * Neither the name of the nor the
* names of its contributors may be used to endorse or promote products
* derived from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
* ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
* WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL BE LIABLE FOR ANY
* DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
* (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
* LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
* ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
* SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package net.ossindex.common.request;
import java.io.IOException;
import java.lang.reflect.Type;
import java.util.Collection;
import java.util.Collections;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.List;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.reflect.TypeToken;
import net.ossindex.common.IPackageRequest;
import net.ossindex.common.PackageCoordinate;
import net.ossindex.common.PackageDescriptor;
import net.ossindex.common.VulnerabilityDescriptor;
import net.ossindex.common.filter.IVulnerabilityFilter;
import net.ossindex.common.filter.VulnerabilityFilterFactory;
/**
* Perform a package request.
*
* @author Ken Duck
*/
public class PackageRequest implements IPackageRequest
{
private final Gson gson = new GsonBuilder().disableHtmlEscaping().create();
private final OssIndexHttpClient client;
public PackageRequest(OssIndexHttpClient client) {
this.client = client;
}
/**
* Packages returned from the server are in the same order as the packages SENT to the server.
*/
private List packages = new LinkedList();
/**
* We assume that the results for a path will be returned in the same order that they are requested from the server.
* In other words there will be one path per package (see packages above).
*/
private List> paths = new LinkedList<>();
/**
* List of all filters to apply
*/
private List filters = new LinkedList<>();
public void addVulnerabilityFilter(IVulnerabilityFilter filter) {
filters.add(filter);
}
/*
* (non-Javadoc)
* @see net.ossindex.common.IPackageRequest#add(java.lang.String, java.lang.String, java.lang.String, java.lang.String)
*/
@Override
public PackageDescriptor add(String pm, String groupId, String artifactId, String version) {
PackageCoordinate pkg = PackageCoordinate.newBuilder()
.withFormat(pm)
.withNamespace(groupId)
.withName(artifactId)
.withVersion(version)
.build();
return add(Collections.singletonList(pkg));
}
@Override
public PackageDescriptor add(List path) {
if (path != null && !path.isEmpty()) {
PackageCoordinate pkg = path.get(path.size() - 1);
// Build the descriptor for the query
PackageDescriptor desc = new PackageDescriptor(pkg.getFormat(), pkg.getNamespace(), pkg.getName(),
pkg.getVersion());
// Add the package path to the packages and path lists, in the same order
packages.add(desc);
paths.add(path);
return desc;
}
return null;
}
/*
* (non-Javadoc)
* @see net.ossindex.common.IPackageRequest#run()
*/
@Override
public Collection run() throws IOException {
String data = gson.toJson(packages);
// Perform the OSS Index query
String response = client.performPostRequest("package", data);
// Convert the results to Java objects
Type listType = new TypeToken>() { }.getType();
Collection results = gson.fromJson(response, listType);
filterResults(results); // This will remove vulnerabilities from packages
return results;
}
private void filterResults(final Collection pkgs) {
if (!filters.isEmpty()) {
Iterator pkgIt = pkgs.iterator();
Iterator> pathIt = paths.iterator();
while (pkgIt.hasNext()) {
if (!pathIt.hasNext()) {
throw new IllegalArgumentException("Server results do not match request");
}
PackageDescriptor pkg = pkgIt.next();
List path = pathIt.next();
filterPackage(pkg, path);
}
}
}
private void filterPackage(final PackageDescriptor pkg,
final List path)
{
List vulns = pkg.getVulnerabilities();
if (vulns != null && !vulns.isEmpty()) {
// Build a new list of vulnerabilities
List filteredVulns = new LinkedList<>();
// See if any vulnerabilities are filtered
for (VulnerabilityDescriptor vuln : vulns) {
String vid = Long.toString(vuln.getId());
for (IVulnerabilityFilter filter : filters) {
if (!VulnerabilityFilterFactory.shouldFilter(filter, path, vid)) {
filteredVulns.add(vuln);
}
}
}
pkg.setVulnerabilities(filteredVulns);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy