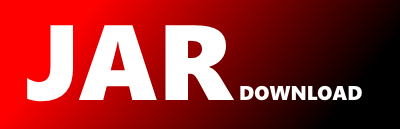
net.ozwolf.mongo.migrations.MongoTrekState Maven / Gradle / Ivy
package net.ozwolf.mongo.migrations;
import net.ozwolf.mongo.migrations.internal.domain.Migration;
import java.util.*;
import static java.util.stream.Collectors.toList;
/**
* Mongo Trek State
*
* This class provides the collection of migrations and an ability to query the overall state of the process.
*
* This includes what the current version is, what the pending state is as well as a list of failed and applied migrations.
*/
public class MongoTrekState {
private final Map migrations;
public MongoTrekState(Collection migrations) {
this.migrations = new HashMap<>();
migrations.forEach(m -> this.migrations.put(m.getVersion(), m));
}
/**
* Get the currently applied migration version.
*
* @return The currently applied version
*/
public String getCurrentVersion() {
return getLastSuccessfulMigration().map(Migration::getVersion).orElse("N/A");
}
/**
* Get the full list of migrations. This includes the entire history of applied migrations, even if the command source has since been removed from the project.
*
* @return The full list of migrations, both applied history and pending commands.
*/
public List getMigrations() {
return migrations.values().stream().sorted(Migration.sortByVersionAscending()).collect(toList());
}
/**
* Return the pending migrations state.
*
* @return The pending migration state
*/
public Pending getPending() {
List migrations = this.migrations.values()
.stream()
.filter(m -> m.isPending() || m.isFailed())
.collect(toList());
return new Pending(migrations);
}
/**
* Get the list of migrations that have failed.
*
* @return The failed migrations
*/
public List getFailed() {
return this.migrations.values()
.stream()
.filter(Migration::isFailed)
.collect(toList());
}
/**
* Get the list of successfully applied migrations
*
* @return The applied migrations
*/
public List getApplied() {
return this.migrations.values()
.stream()
.filter(Migration::isSuccessful)
.collect(toList());
}
private Optional getLastSuccessfulMigration() {
return migrations.values()
.stream()
.filter(Migration::isSuccessful)
.min(Migration.sortByVersionDescending());
}
/**
* Migrations State - Pending State
*
* This class provides the collection of migrations pending application to the system.
*/
public static class Pending {
private final List migrations;
private Pending(List migrations) {
this.migrations = migrations;
}
/**
* Flag to determine if there is any pending migrations
*
* @return true if there are pending migrations
*/
public boolean hasPendingMigrations() {
return !this.migrations.isEmpty();
}
/**
* The next migration version to be applied
*
* @return the next pending migration version or {@code N/A} if none to be applied
*/
public String getNextPendingVersion() {
return migrations.stream().min(Migration.sortByVersionAscending()).map(Migration::getVersion).orElse("N/A");
}
/**
* The last migration version to be applied
*
* @return the next pending migration version or {@code N/A} if none to be applied
*/
public String getLastPendingVersion() {
return migrations.stream().min(Migration.sortByVersionDescending()).map(Migration::getVersion).orElse("N/A");
}
/**
* The list of pending migrations
*
* @return The list of pending migrations
*/
public List getMigrations() {
return migrations.stream().sorted(Migration.sortByVersionAscending()).collect(toList());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy