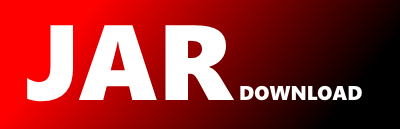
pascal.taie.analysis.dataflow.inter.AbstractInterDataflowAnalysis Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tai-e Show documentation
Show all versions of tai-e Show documentation
An easy-to-learn/use static analysis framework for Java
The newest version!
/*
* Tai-e: A Static Analysis Framework for Java
*
* Copyright (C) 2022 Tian Tan
* Copyright (C) 2022 Yue Li
*
* This file is part of Tai-e.
*
* Tai-e is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License
* as published by the Free Software Foundation, either version 3
* of the License, or (at your option) any later version.
*
* Tai-e is distributed in the hope that it will be useful,but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY
* or FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General
* Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with Tai-e. If not, see .
*/
package pascal.taie.analysis.dataflow.inter;
import pascal.taie.World;
import pascal.taie.analysis.ProgramAnalysis;
import pascal.taie.analysis.dataflow.fact.DataflowResult;
import pascal.taie.analysis.graph.icfg.CallEdge;
import pascal.taie.analysis.graph.icfg.CallToReturnEdge;
import pascal.taie.analysis.graph.icfg.ICFG;
import pascal.taie.analysis.graph.icfg.ICFGBuilder;
import pascal.taie.analysis.graph.icfg.ICFGEdge;
import pascal.taie.analysis.graph.icfg.NormalEdge;
import pascal.taie.analysis.graph.icfg.ReturnEdge;
import pascal.taie.config.AnalysisConfig;
/**
* Provides common functionalities for {@link InterDataflowAnalysis} implementations.
*
* @param type of ICFG edges
* @param type of ICFG nodes
* @param type of data-flow facts
*/
public abstract class AbstractInterDataflowAnalysis
extends ProgramAnalysis>
implements InterDataflowAnalysis {
protected ICFG icfg;
protected InterSolver solver;
public AbstractInterDataflowAnalysis(AnalysisConfig config) {
super(config);
}
/**
* If the concrete analysis needs to perform some initialization before
* the solver starts, then it can overwrite this method.
*/
protected void initialize() {
}
/**
* If the concrete analysis needs to perform some finishing work after
* the solver finishes, then it can overwrite this method.
*/
protected void finish() {
}
/**
* Dispatches {@code Node} to specific node transfer functions for
* call nodes and non-call nodes.
*/
@Override
public boolean transferNode(Node node, Fact in, Fact out) {
if (icfg.isCallSite(node)) {
return transferCallNode(node, in, out);
} else {
return transferNonCallNode(node, in, out);
}
}
/**
* Transfer function for call node.
*/
protected abstract boolean transferCallNode(Node node, Fact in, Fact out);
/**
* Transfer function for non-call node.
*/
protected abstract boolean transferNonCallNode(Node node, Fact in, Fact out);
/**
* Dispatches {@link ICFGEdge} to specific edge transfer functions
* according to the concrete type of {@link ICFGEdge}.
*/
@Override
public Fact transferEdge(ICFGEdge edge, Fact out) {
if (edge instanceof NormalEdge) {
return transferNormalEdge((NormalEdge) edge, out);
} else if (edge instanceof CallToReturnEdge) {
return transferCallToReturnEdge((CallToReturnEdge) edge, out);
} else if (edge instanceof CallEdge) {
return transferCallEdge((CallEdge) edge, out);
} else {
return transferReturnEdge((ReturnEdge) edge, out);
}
}
// ---------- transfer functions for specific ICFG edges ----------
protected abstract Fact transferNormalEdge(NormalEdge edge, Fact out);
protected abstract Fact transferCallToReturnEdge(CallToReturnEdge edge, Fact out);
protected abstract Fact transferCallEdge(CallEdge edge, Fact callSiteOut);
protected abstract Fact transferReturnEdge(ReturnEdge edge, Fact returnOut);
// ----------------------------------------------------------------
@Override
public DataflowResult analyze() {
icfg = World.get().getResult(ICFGBuilder.ID);
initialize();
solver = new InterSolver<>(this, icfg);
DataflowResult result = solver.solve();
finish();
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy