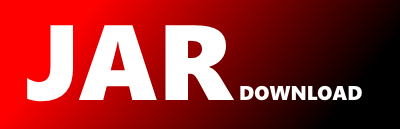
pascal.taie.analysis.graph.callgraph.Edge Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tai-e Show documentation
Show all versions of tai-e Show documentation
An easy-to-learn/use static analysis framework for Java
The newest version!
/*
* Tai-e: A Static Analysis Framework for Java
*
* Copyright (C) 2022 Tian Tan
* Copyright (C) 2022 Yue Li
*
* This file is part of Tai-e.
*
* Tai-e is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License
* as published by the Free Software Foundation, either version 3
* of the License, or (at your option) any later version.
*
* Tai-e is distributed in the hope that it will be useful,but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY
* or FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General
* Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with Tai-e. If not, see .
*/
package pascal.taie.analysis.graph.callgraph;
import pascal.taie.util.Hashes;
/**
* Represents call edges in the call graph.
*
* @param type of call sites
* @param type of methods
*/
public class Edge {
private final CallKind kind;
private final CallSite callSite;
private final Method callee;
private final int hashCode;
public Edge(CallKind kind, CallSite callSite, Method callee) {
this.kind = kind;
this.callSite = callSite;
this.callee = callee;
hashCode = Hashes.hash(kind, callSite, callee);
}
/**
* @return kind of the call edge.
*/
public CallKind getKind() {
return kind;
}
/**
* @return String representation of information for this edge.
* By default, the information represents the {@link CallKind},
* and other subclasses of {@link Edge} may contain additional content.
*/
public String getInfo() {
return kind.name();
}
/**
* @return the call site (i.e., the source) of the call edge.
*/
public CallSite getCallSite() {
return callSite;
}
/**
* @return the callee method (i.e., the target) of the call edge.
*/
public Method getCallee() {
return callee;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Edge, ?> edge = (Edge, ?>) o;
return kind == edge.kind &&
callSite.equals(edge.callSite) &&
callee.equals(edge.callee);
}
@Override
public int hashCode() {
return hashCode;
}
@Override
public String toString() {
return "[" + getInfo() + "]" + callSite + " -> " + callee;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy